What is Arduino interfacing?
Arduino interfacing refers to the process of connecting and communicating with external devices and sensors using an Arduino board. An Arduino board is a microcontroller-based platform that can be programmed to control and interact with a wide variety of electronic components and systems.
How Can Arduino interface with MATLAB?
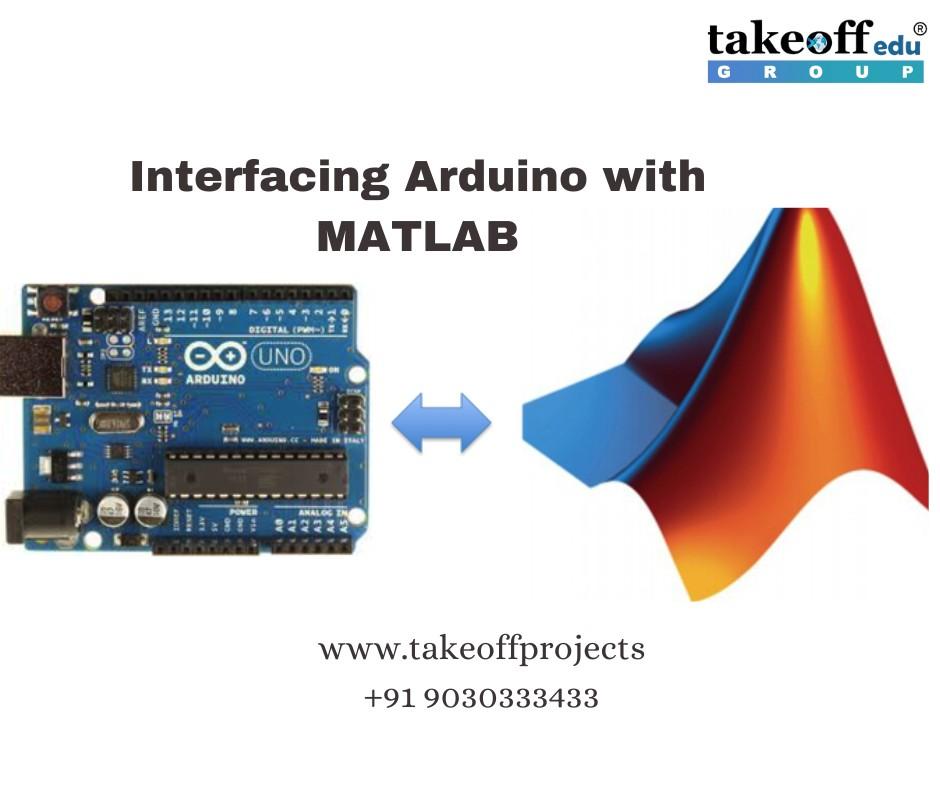
Arduino and MATLAB can be interfaced with each other in several ways. One of the most common ways is by using the MATLAB Support Package for Arduino Hardware, which allows you to communicate with your Arduino board from within MATLAB. Here are the steps to get started:
Install the MATLAB Support Package for Arduino Hardware from the MATLAB Add-Ons Explorer or MathWorks website.
Connect your Arduino board to your computer via USB.
Open MATLAB and create a new script.
In the script, use the "arduino" function to create an Arduino object:
less Copy code
a = arduino('COM3', 'Uno');
Replace "COM3" with the port your Arduino board is connected to, and "Uno" with the board type you are using.
Use MATLAB commands to interact with your Arduino board. For example, you can turn on an LED connected to pin 13 by running: scss
Copy code
writeDigitalPin(a, 'D13', 1);
This sends a digital high signal to pin 13.
You can also read from sensors connected to your Arduino board using MATLAB commands.
There are many more functions and capabilities provided by the MATLAB Support Package for Arduino Hardware, so be sure to check out the documentation for more information.
What are the 3 types of interfaces?
The three types of interfaces are:
Command-Line Interface (CLI)
Graphical User Interface (GUI)
Application Programming Interface (API)
How to integrate MATLAB code with Arduino?
Integrating MATLAB with Arduino can be done using the following steps:
Install the MATLAB Support Package for Arduino: The first step is to install the MATLAB Support Package for Arduino, which allows MATLAB to communicate with Arduino over a serial port. To install the support package, go to the "Add-Ons" menu in MATLAB, search for "Arduino," and follow the installation instructions.
Connect the Arduino: Connect your Arduino to your computer using a USB cable.
Write the Arduino code: Write the Arduino code using the Arduino IDE or any other text editor. The code should include the necessary input and output pins, as well as any necessary libraries. The code should also include serial communication instructions to allow communication with MATLAB.
Upload the Arduino code: Upload the Arduino code to the board using the Arduino IDE.
Write the MATLAB code: Write the MATLAB code that will communicate with the Arduino. The MATLAB code should include instructions for opening the serial port and sending and receiving data to and from the Arduino.
Run the MATLAB code: Run the MATLAB code to communicate with the Arduino.
Here's an example code snippet in MATLAB to communicate with Arduino:
MATLAB
Copy code
% Define serial port s = serialport("COM3", 9600);
% Send data to Arduino
write(s, "Hello Arduino!");
% Receive data from Arduino
data = readline(s);
% Close serial port
clear s;
And here's an example code snippet for the Arduino:
C++
Copy code
void setup() {
// Initialize serial communication
Serial.begin(9600);
} void loop() {
// Wait for incoming serial data
while (Serial.available() == 0) {}
// Read incoming serial data
String data = Serial.readString();
// Send data back to MATLAB
Serial.println("Received data: " + data);
Note that this is just a basic example, and the actual code will depend on your specific project requirements.
Here Takeoff Projects will help you get a wide range of Projects
We also give Project Assistance for your project Ideas. Call/whatsapp -9030333433 for a free demo OR Visit: https://takeoffprojects.com/