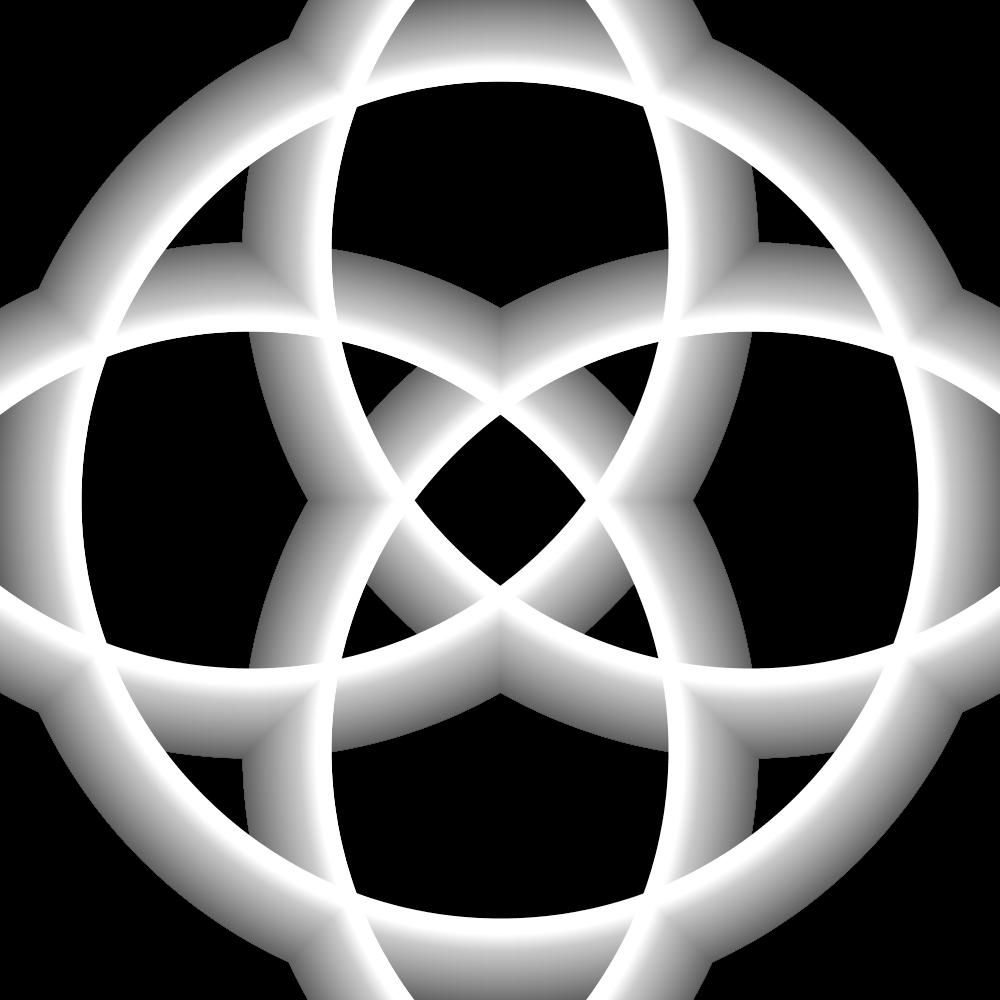
3 minute read
GlobalVariable, localVariable
Organizing code into setup () and draw () function blocks.
This chapter introduces the Processing programming language. To start with, let‘s try to analyse this program.
Advertisement
int _diam = 10; float _centX, _centY;
This is the initialisation of the variable _diam. I assume this stands for a diameter of 10 pixels. That in itself sounds very basic but I have a general comment about using underscores in global variables. I have tried to use it as Matt Pearson suggests. But I find it unclear. Underscores disappear in the text. In contrast, I like Andrew Glassner‘s suggestion better. He says: type global variables with an initial capital and start local variables with underscores. Two examples: GlobalVariable, localVariable. I think that, to me, is clear enough.
Initialisation of the variables _centX and _centY frameRate (24);
The frame rate is 24 frames per second.
_centX = width / 2;
_centX is the horizontal point (250) at the centre of the width.
_centY = height / 2; stroke (0); strokeWeight (5); fill (255, 50); if (_diam <= 400){ background (180);
_centY is the vertical point (150) at the centre of the height.
The colour of the line is black.
The thickness of the line is 5 pixels.
Each circle is filled with white and an alpha of 50.
If _diam is less than or equal to 400, the program running.
On every run through draw, the background is refreshed with a grey tone of 180. ellipse (_centX, _centY, _diam, _diam);
The first ellipse is placed in the centre with a diameter (_diam) of 10 pixels.
_diam += 10;
After each run through the draw () function block, _diam is incremented by 10 pixels. Until _diam is less than or equal to 400 pixels. Then the program stops.
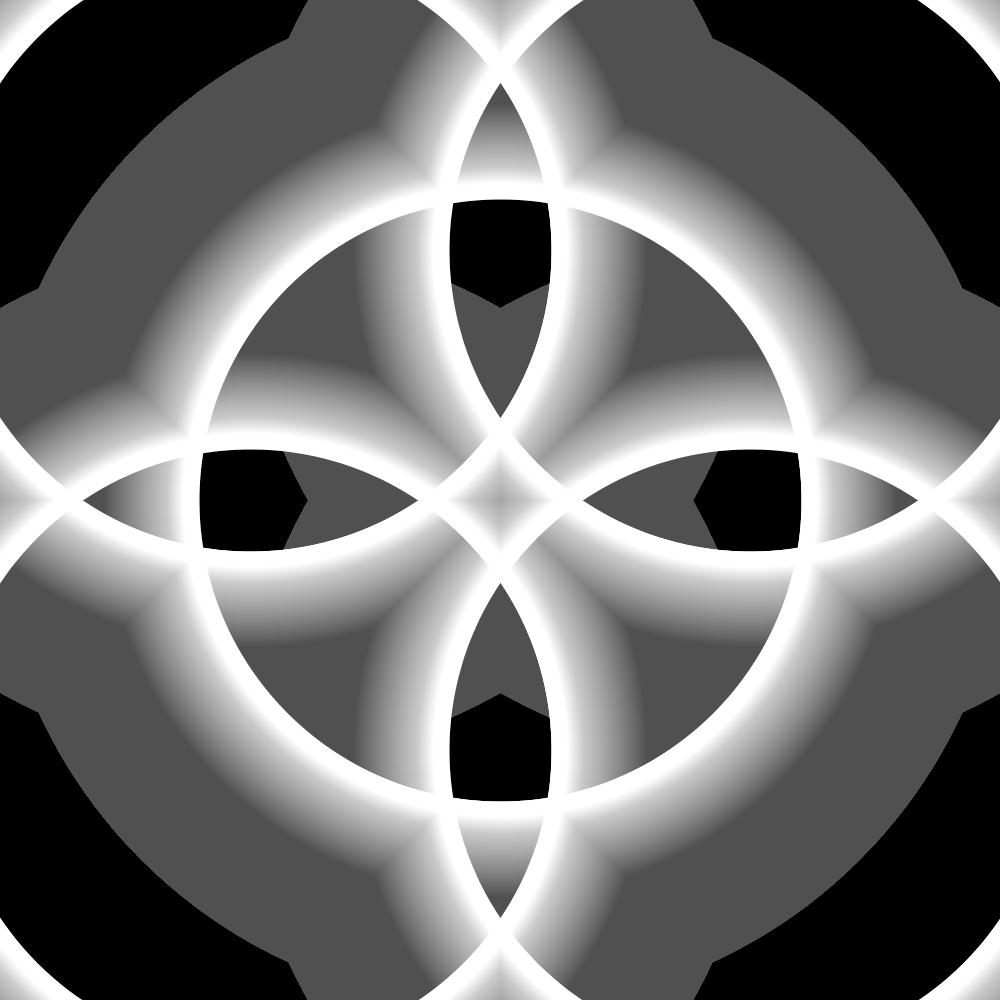
Variations
Organizing code into setup () and draw () function blocks. LFMP_02_01_01 int DiaMeter = 1000; Because I changed the size (), the initialisation of DiaMeter was also changed. size (1000, 1000); Defines the dimension of the display window width (1000) and height (1000) in units of pixels. fill (0, 2); noStroke (); rect (0, 0, width, height); noFill (); stroke (255); We only use white lines. strokeWeight (16); Lines 16 pixels thick. if (DiaMeter >= 0){ ellipse (CenterX, CenterY, DiaMeter, DiaMeter); The first ellipse is placed in the centre (500, 500). ellipse (CenterX / 2, CenterY / 2, DiaMeter, DiaMeter); The second ellipse is placed at the top left (250, 250). ellipse ((CenterX / 2) * 3, CenterY / 2, DiaMeter, DiaMeter); The third ellipse is placed at the top right (750, 250).
I‘m not sure if that was Matt Pearson‘s intention. His version had a more educational function. But I‘m sure he could also have made variations if he had wanted to. In this program, we are dealing with three global variables: _diam, _centX and _centY. As I explained on the previous textpage, I have changed these into the following variable names: DiaMeter, CenterX and CenterY. I also changed the size ().
CenterX = width / 2; CenterX is the width divided by two. CenterX determines the horizontal centre of the display window (500).
CenterY = height / 2; CentreY is the height divided by two. CentreY defines the vertical centre of the display window (500).
In this animation, I wanted very slow-flowing graphics. A well-known method to do this is to fill the total window with a rectangle of high transparency at each run through draw ().
You don‘t want an outline at the rectangles to be placed in the display window.
A rect is inserted and refreshed with each frame. This leaves a grey trace of all the pictured circles. The disadvantage is that the black background eventually changes to grey. This produces this a quasi-3D image. Black is apparently not possible. unless you lower the transparency even more.
After the square is placed, you don‘t want to fill any more surfaces.
If DiaMeter is greater than or equal to 0, the image is refreshed. DiaMeter at the first pass is 1000. So the objects become smaller.
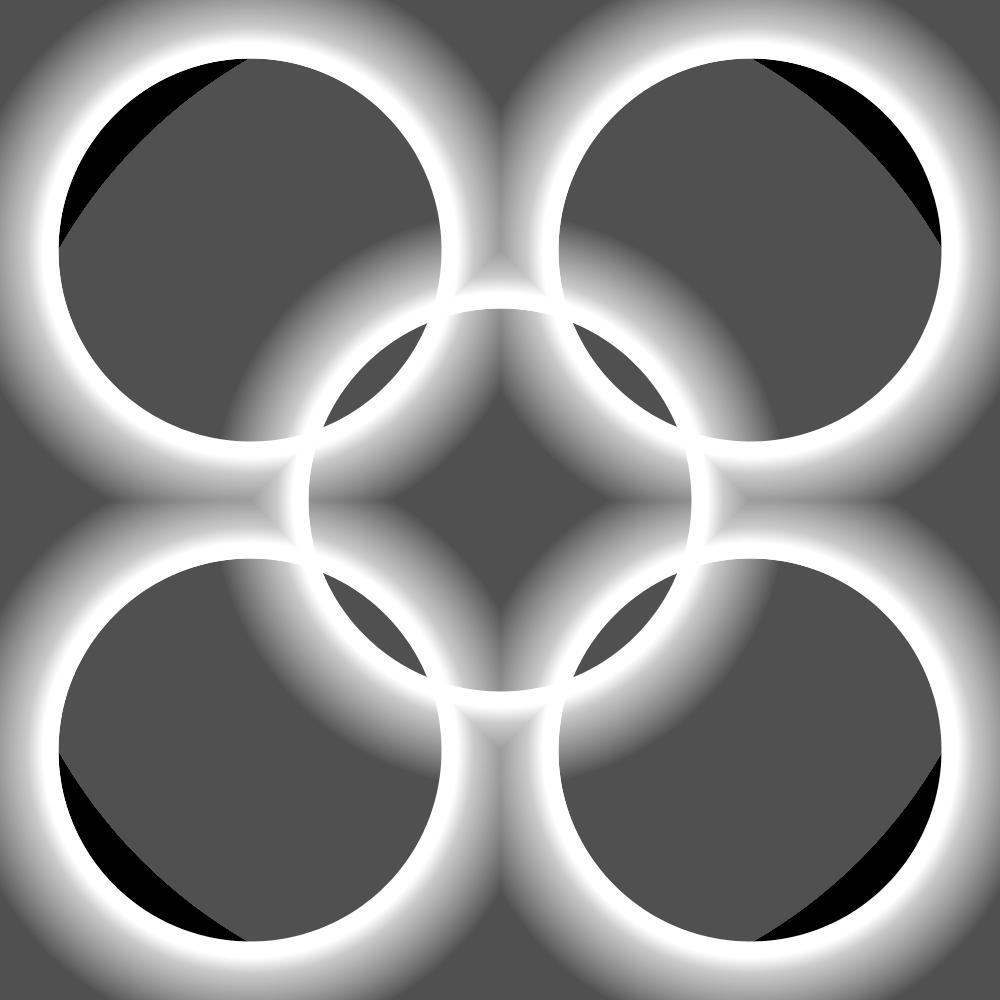
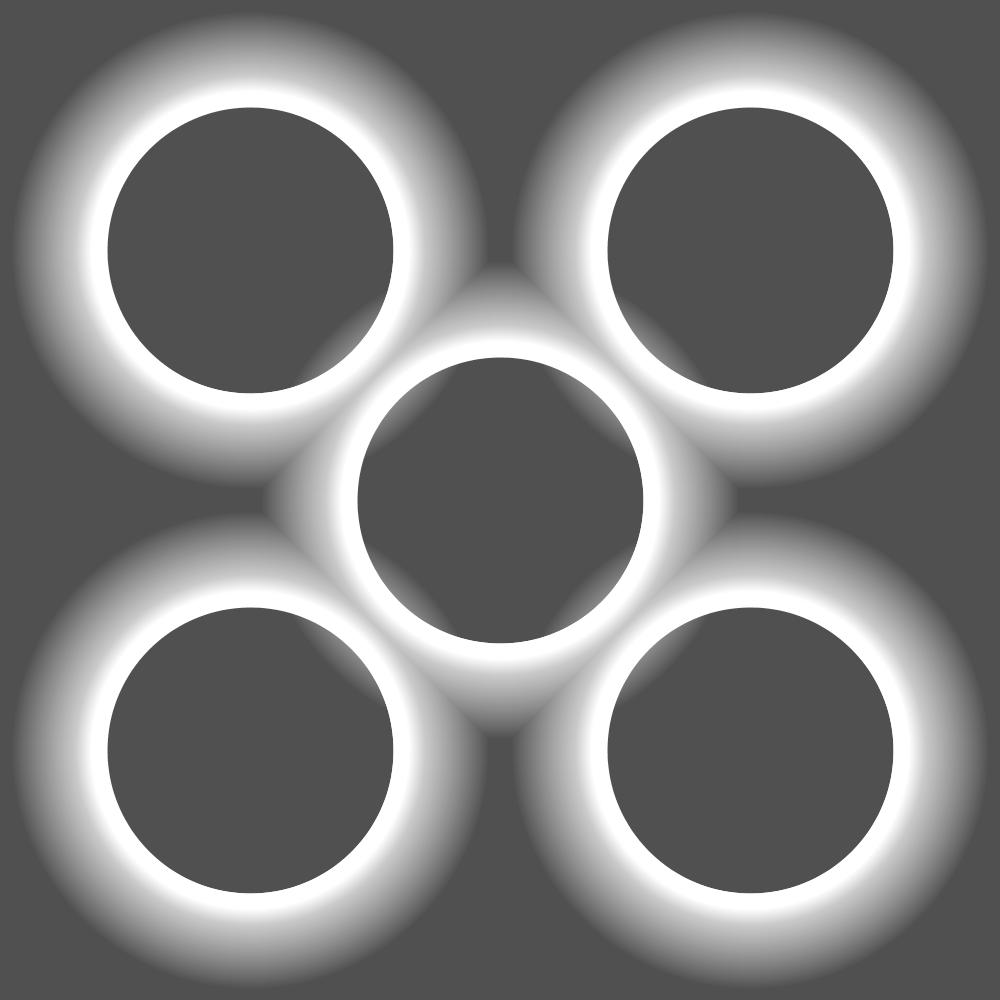
Variations ellipse (CenterX / 2,(CenterY / 2) * 3, DiaMeter, DiaMeter); The fourth ellipse is placed at the bottom left (250, 750). ellipse ((CenterX / 2) * 3, (CenterY / 2) * 3, DiaMeter, DiaMeter); The fifth ellipse is placed at the bottom right (750, 750).
Organizing code into setup () and draw () function blocks.
DiaMeter -= 1; After each run through the if loop, one pixel is subtracted from the 1000 and the loop starts again until the variable DiaMeter is less than or equal to 0. Then the program stops.
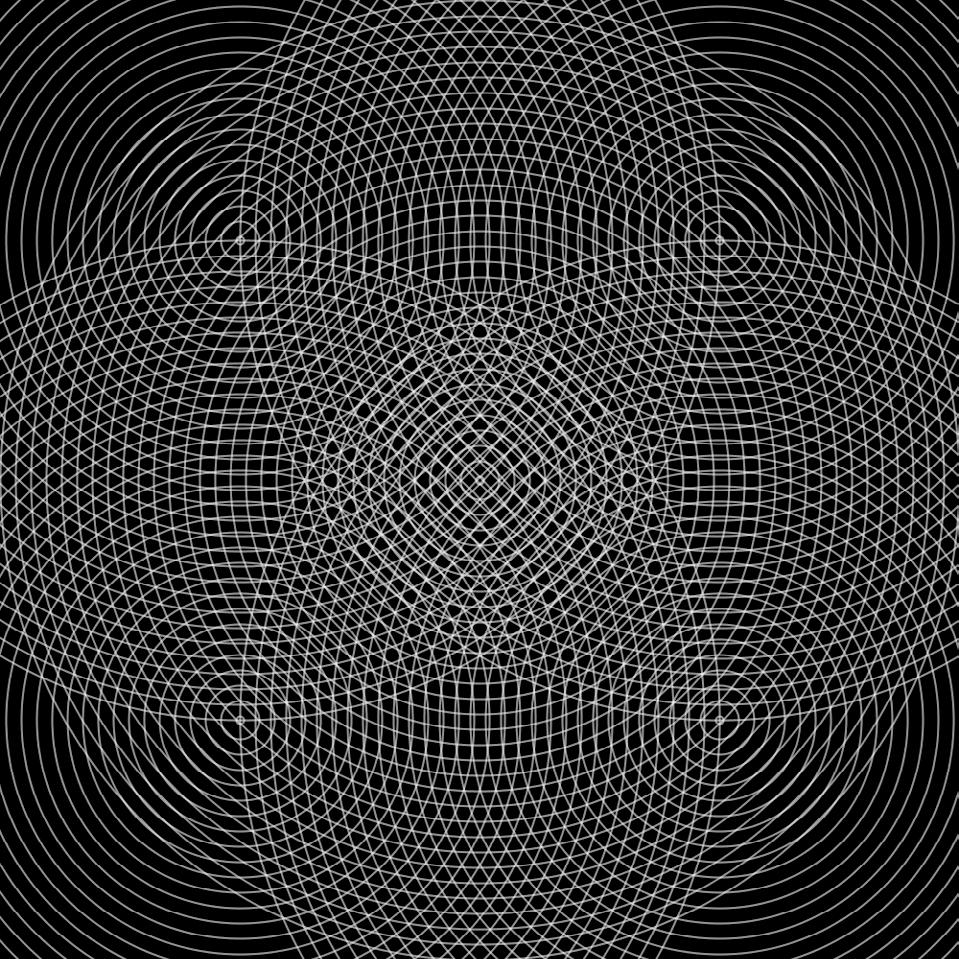
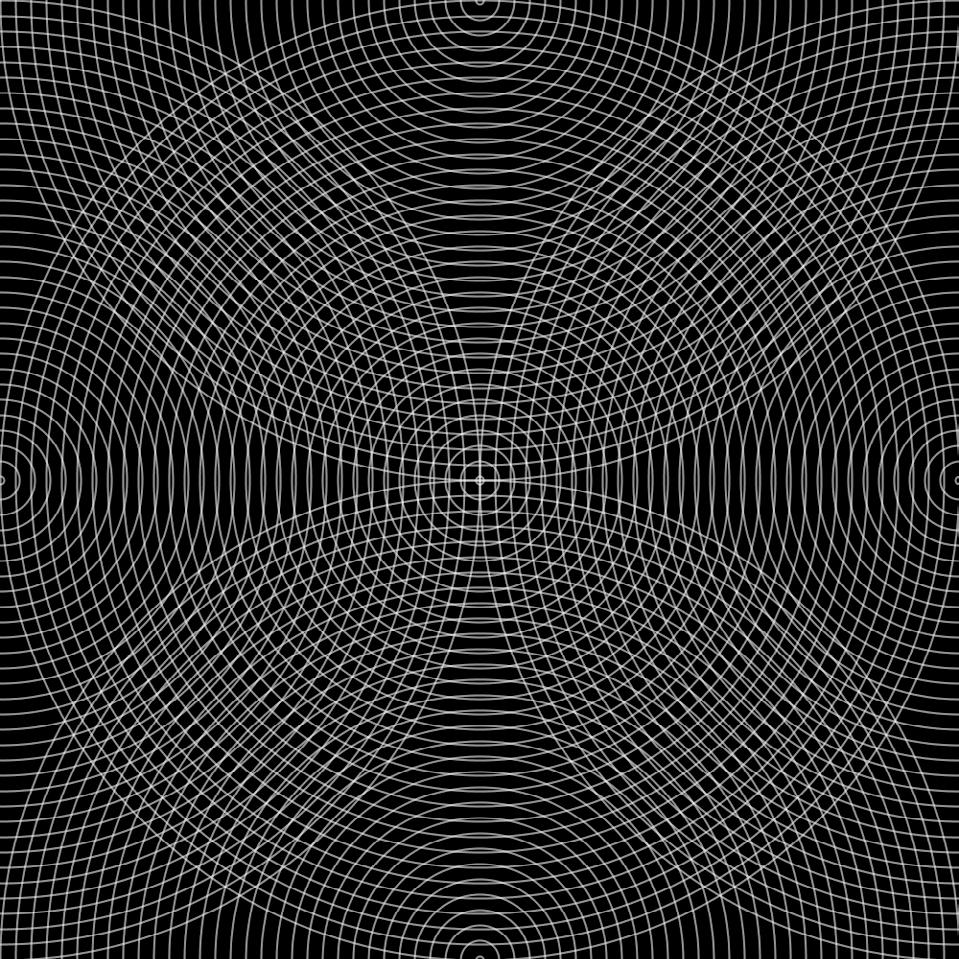
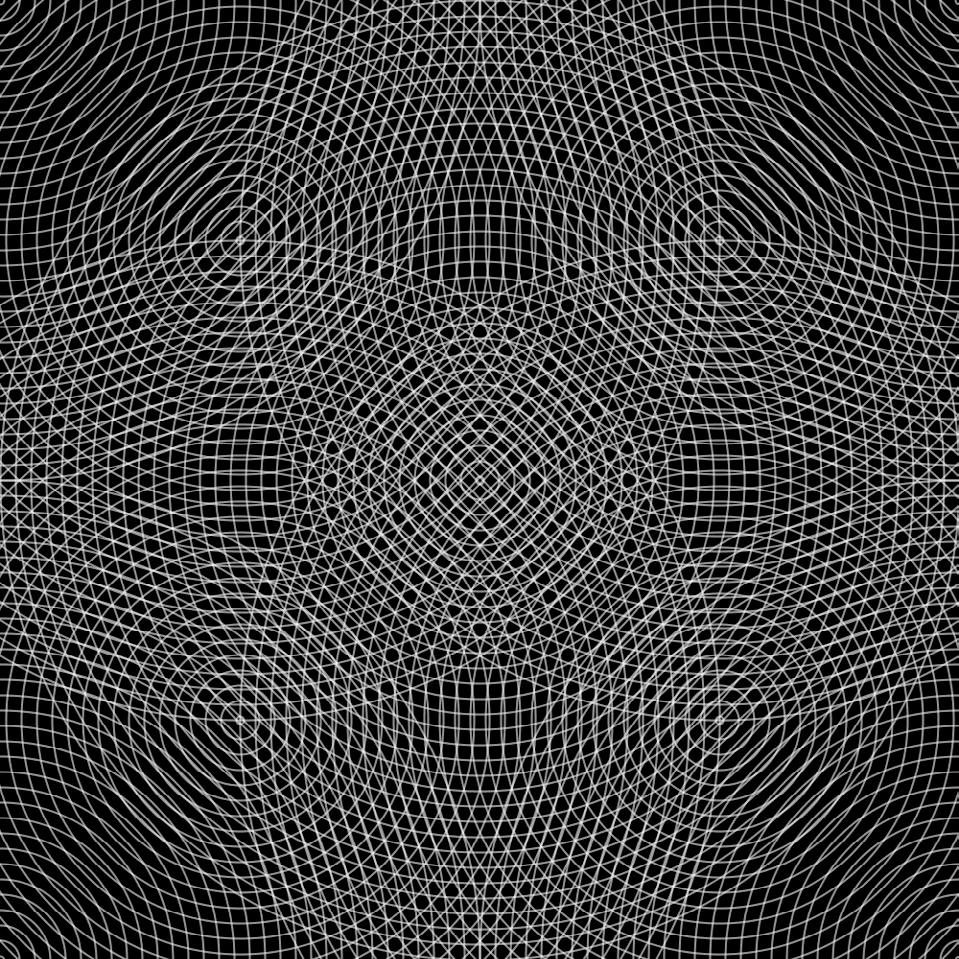
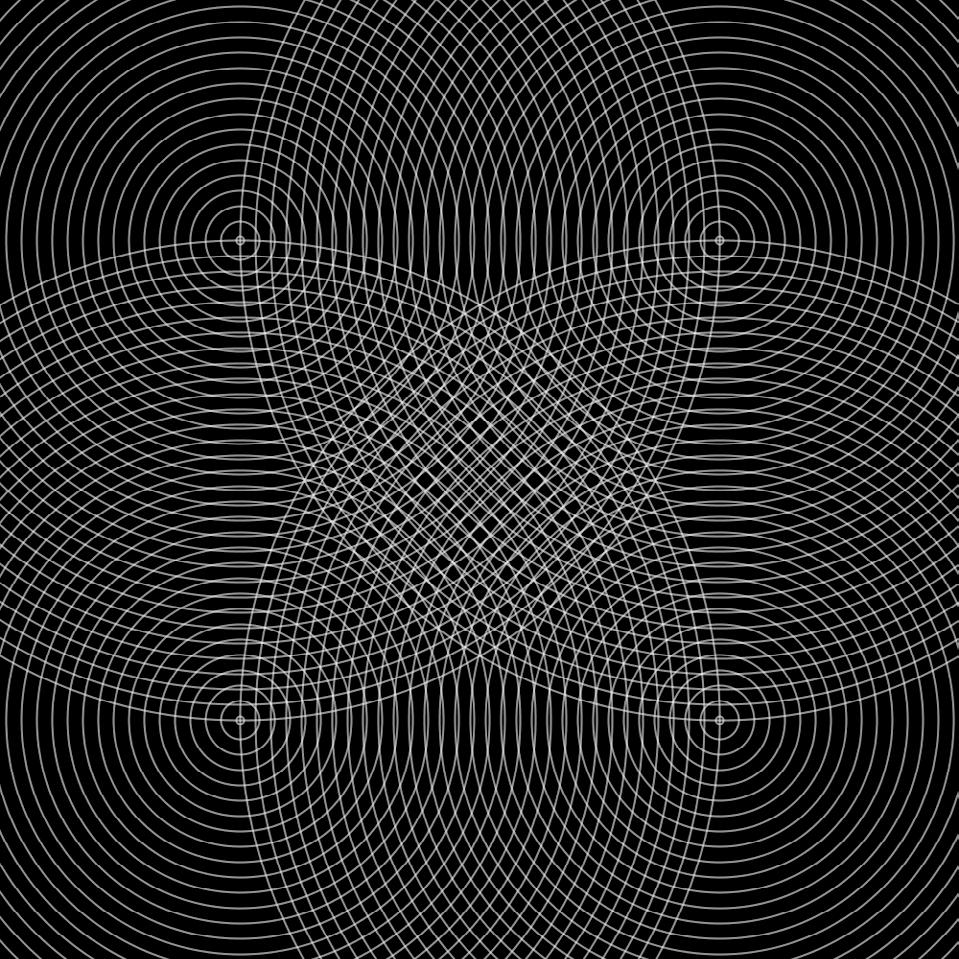
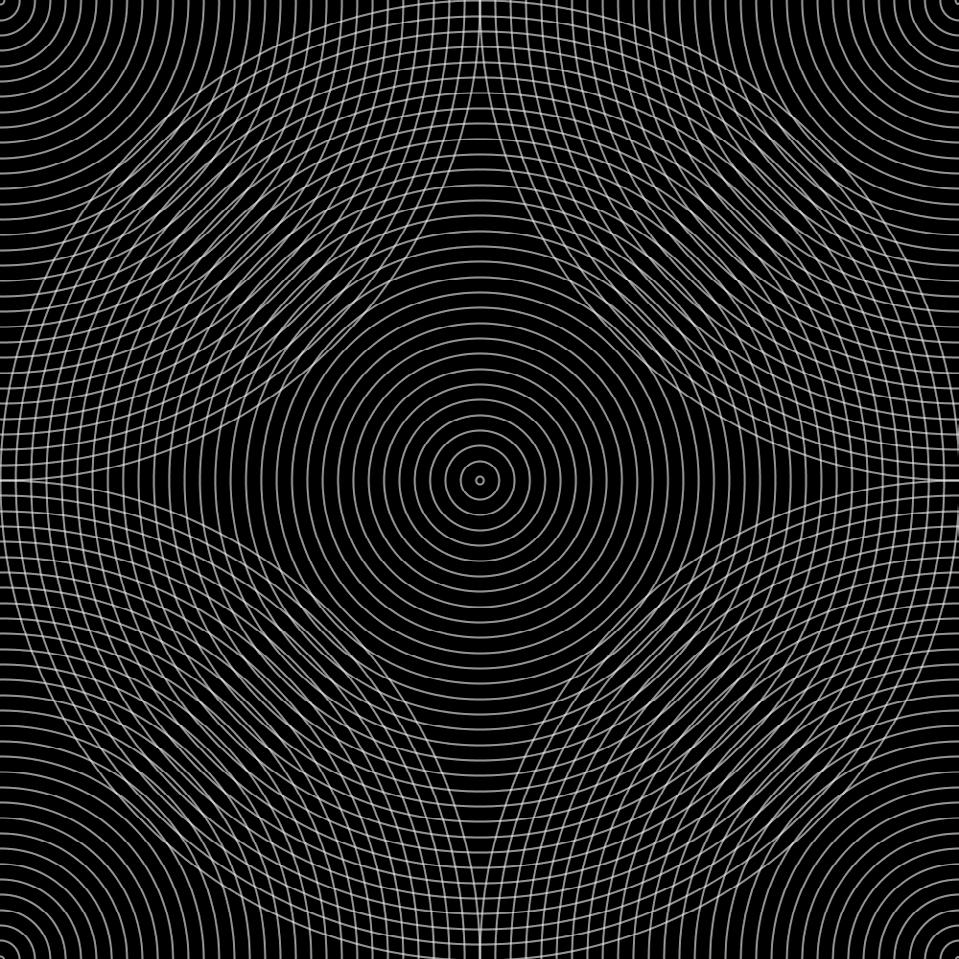
Variations
Organizing code into setup () and draw () function blocks.