WHAT ARE SPACE FILLING CURVES?
In mathematical analysis, a space-filling curve is a curve whose range contains the entire 2-dimensional unit square (or more generally an n-dimensional unit hypercube).
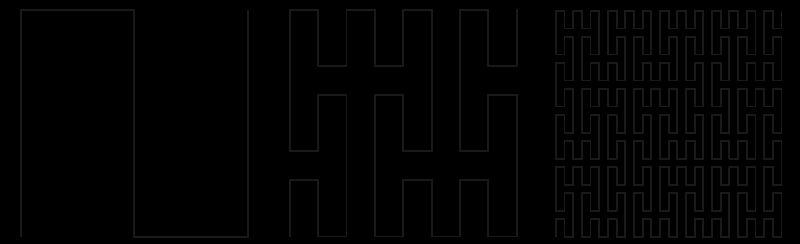
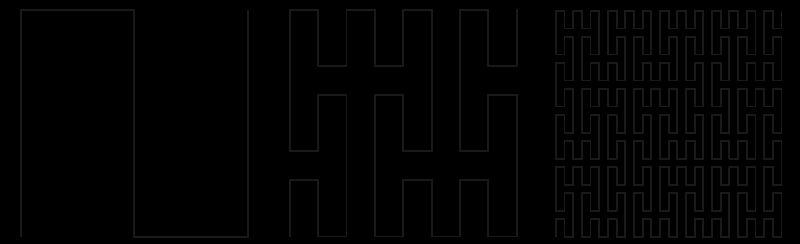
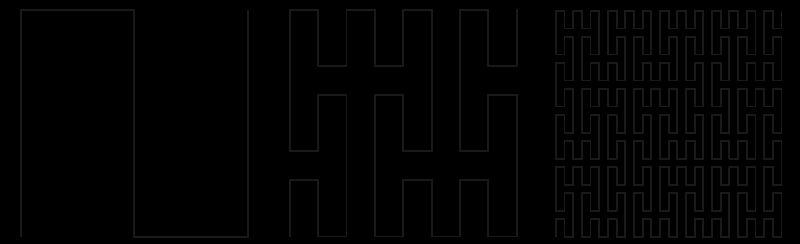
Giuseppe Peano (1858–1932) was the first to discover space-filling curves in the 2-dimensional plane and hence they are sometimes called Peano curves, but that phrase also refers to the Peano curve, the specific example of a space-filling curve found by Peano.
The concept of space-filling curves can be also be implemented in a 3-dimensional space.
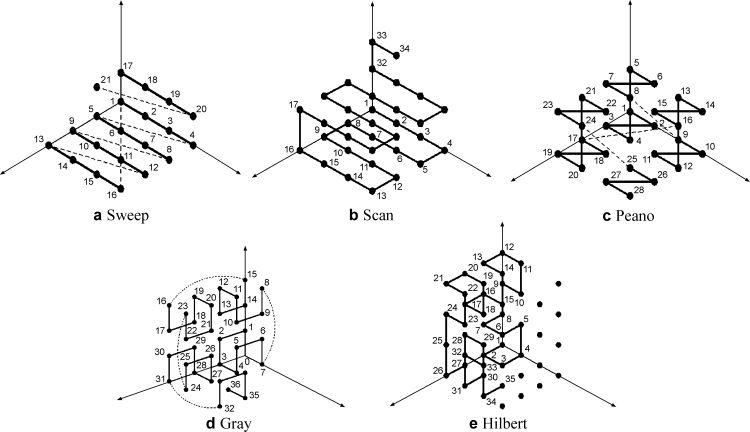
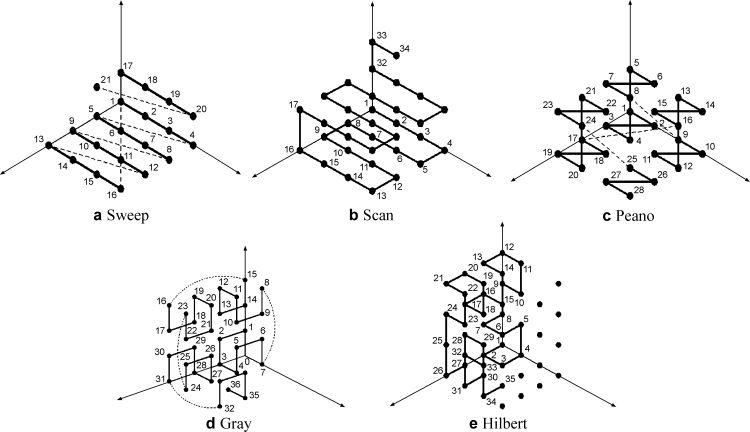
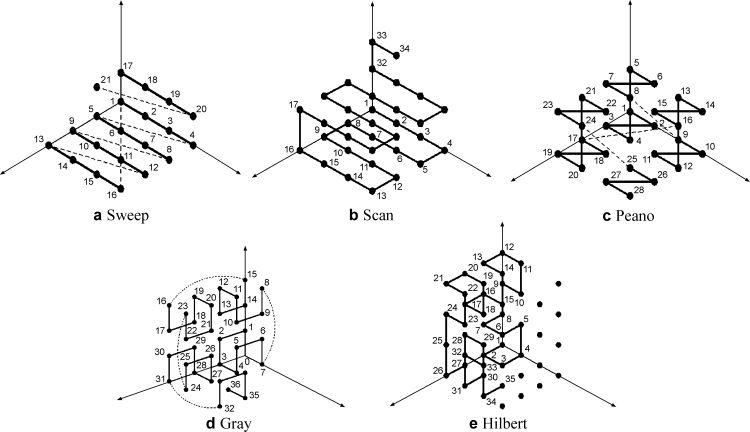
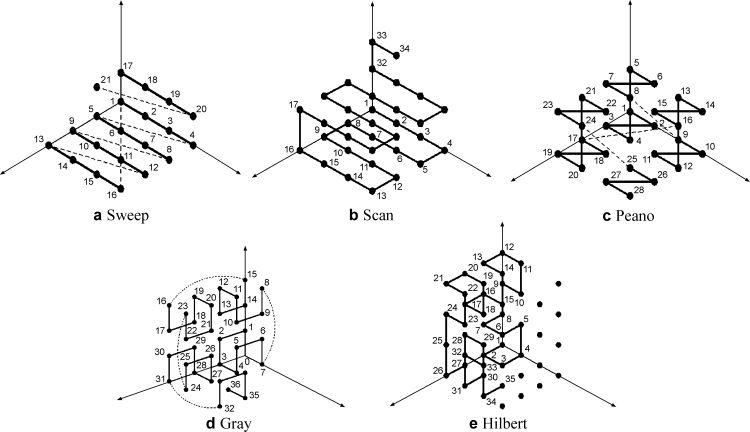
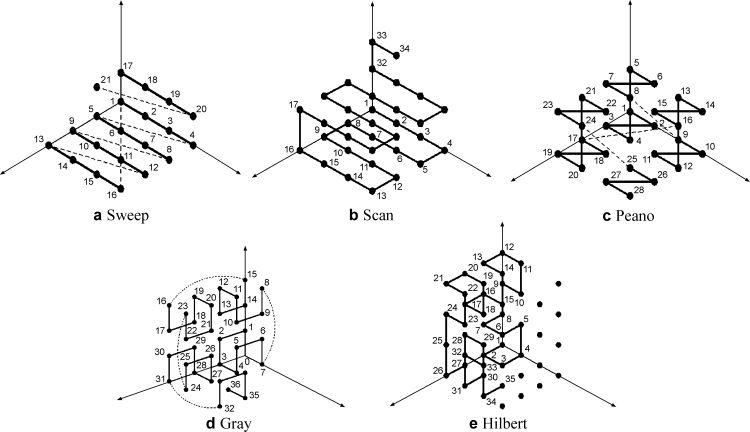
2-dimensional to 3-dimensional
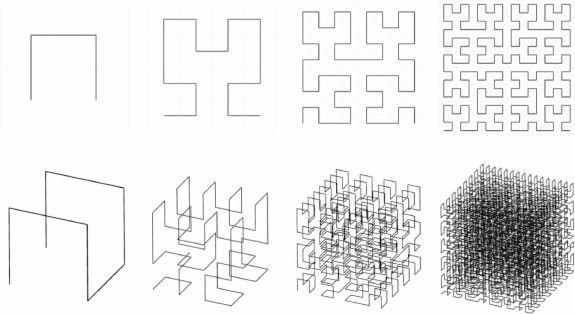
HILBERT CURVE
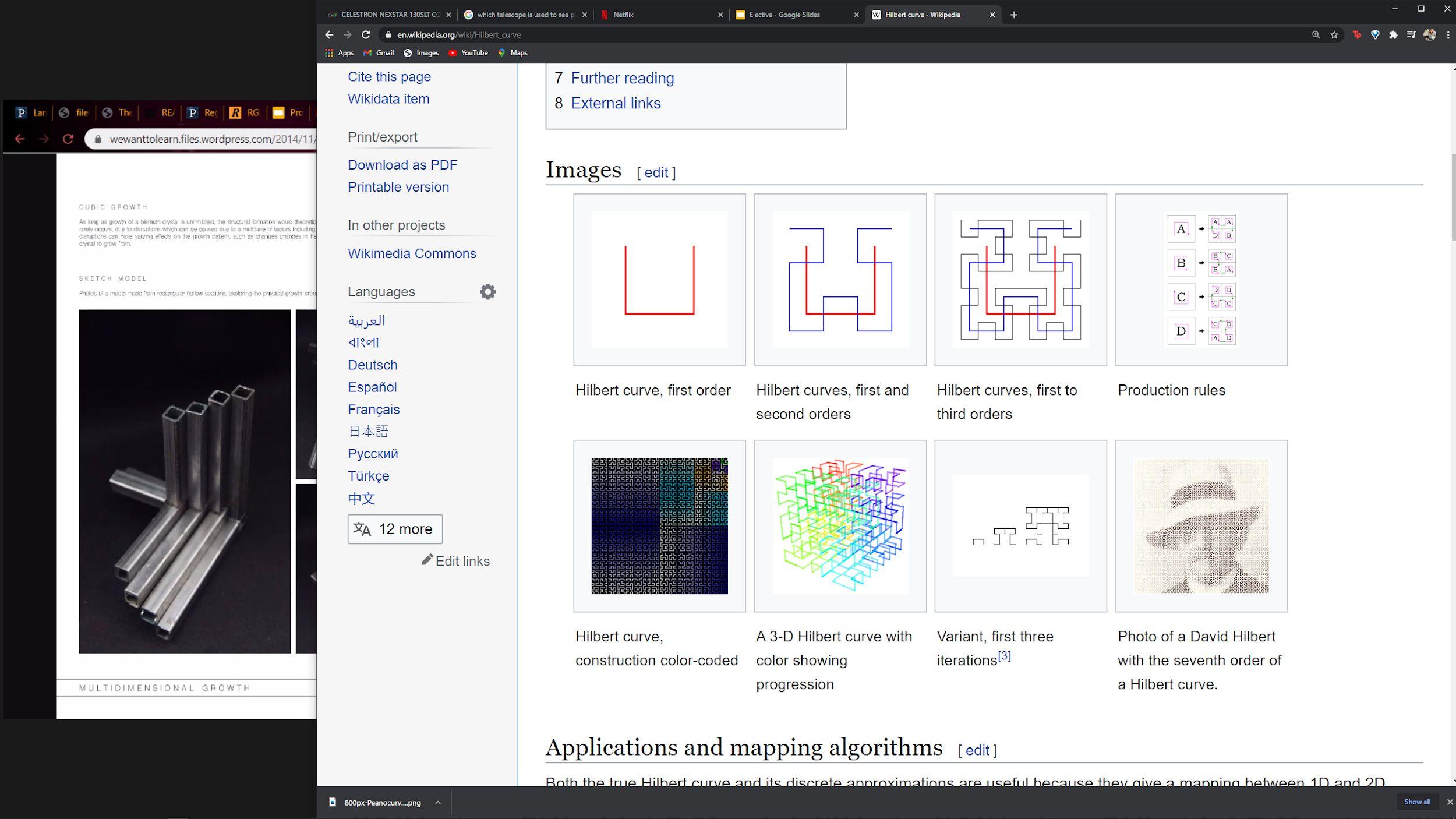
//convert (x,y) to d int xy2d (int n, int x, int y) { int rx, ry, s, d=0; for (s=n/2; s>0; s/=2) { rx = (x & s) > 0; ry = (y & s) > 0; d += s * s * ((3 * rx) ^ ry); rot(n, &x, &y, rx, ry); } return d; } //convert d to (x,y) void d2xy(int n, int d, int *x, int *y) { int rx, ry, s, t=d; *x = *y = 0; for (s=1; s<n; s*=2) { rx = 1 & (t/2); ry = 1 & (t ^ rx); rot(s, x, y, rx, ry); *x += s * rx; *y += s * ry; t /= 4; } }
//rotate/flip a quadrant appropriately void rot(int n, int *x, int *y, int rx, int ry) { if (ry == 0) { if (rx == 1) { *x = n-1 - *x; *y = n-1 - *y; } //Swap x and y int t = *x; *x = *y; *y = t; } }
First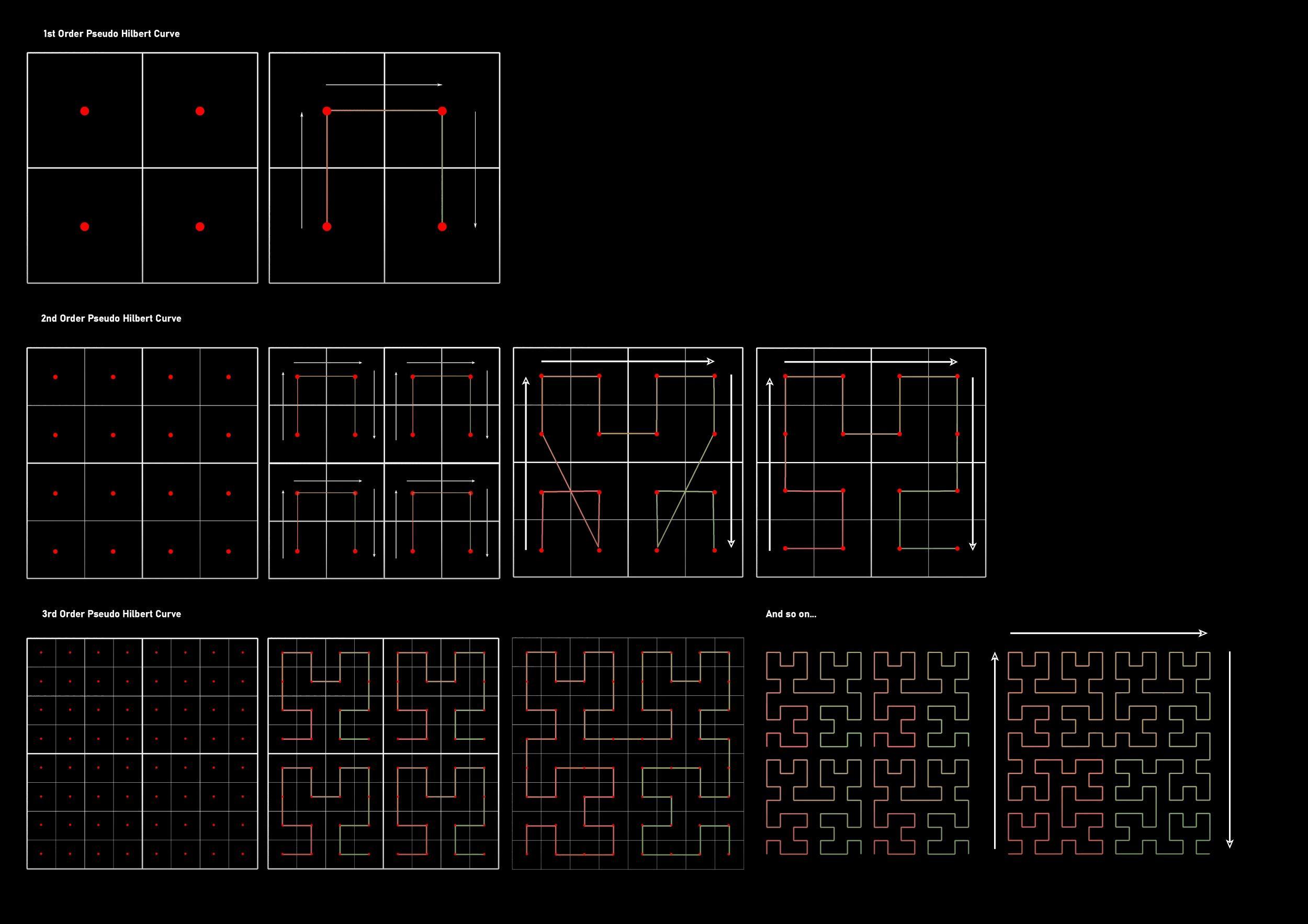
APPROACH
●
Pixel
Updating pixels. ○
Parameters for not updating intermediate pixels.
●
Line Segment/ Vertex
Establish points as possible vertices. ○ Join vertices with line segments. ○
Parameters to give a vector to the line segment. ○
Parameters for maintaining intermediate distance.
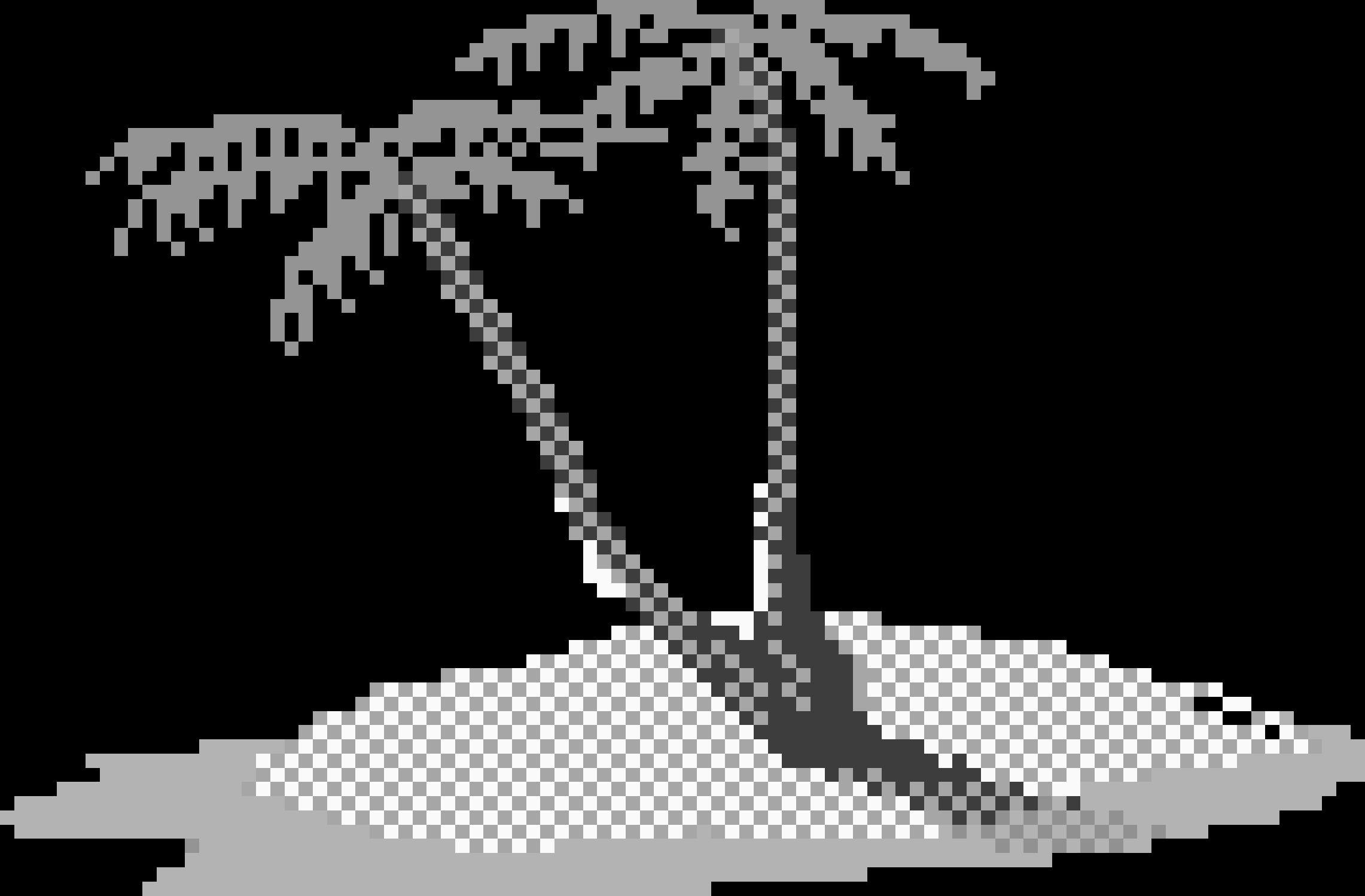
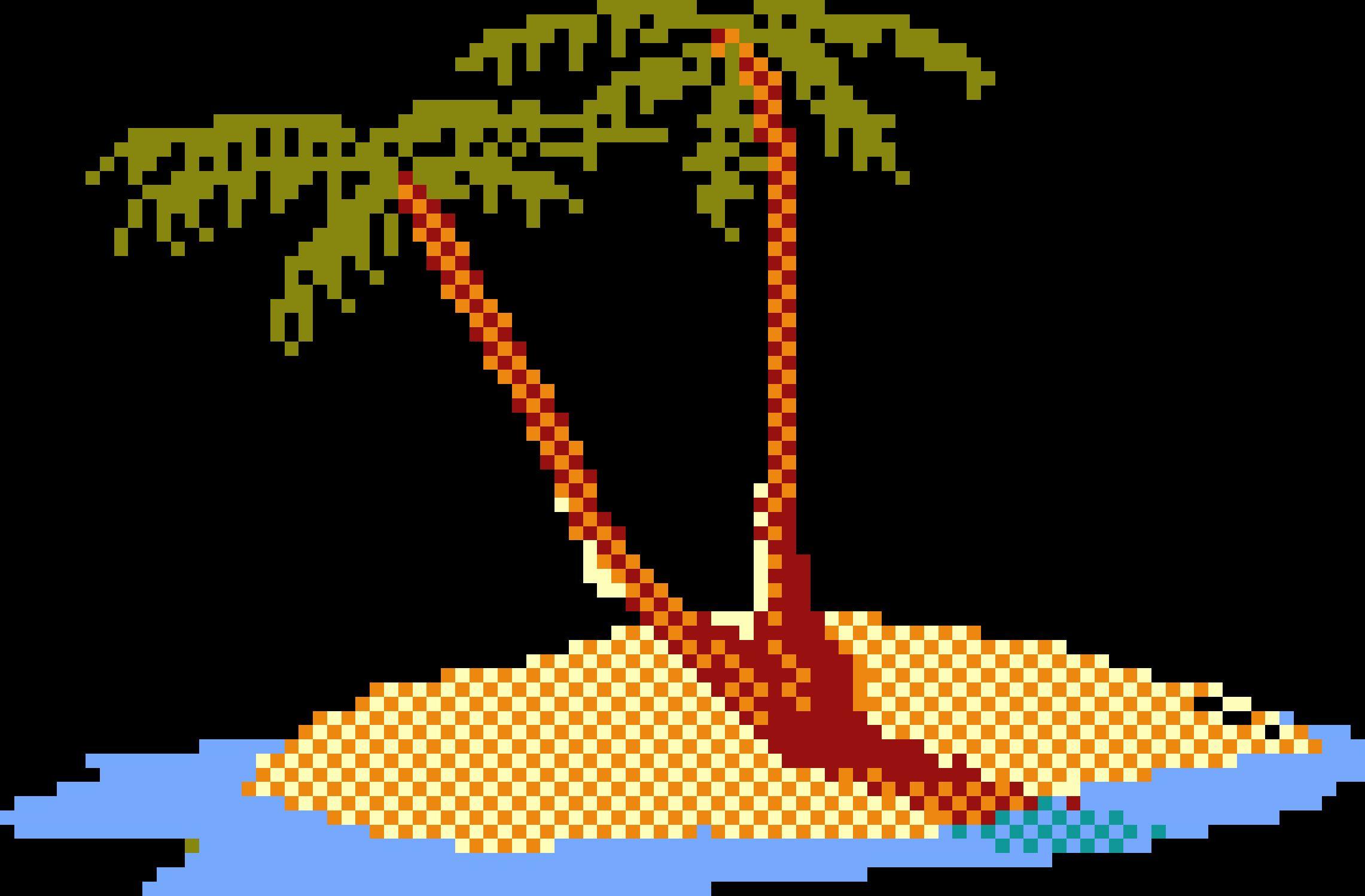
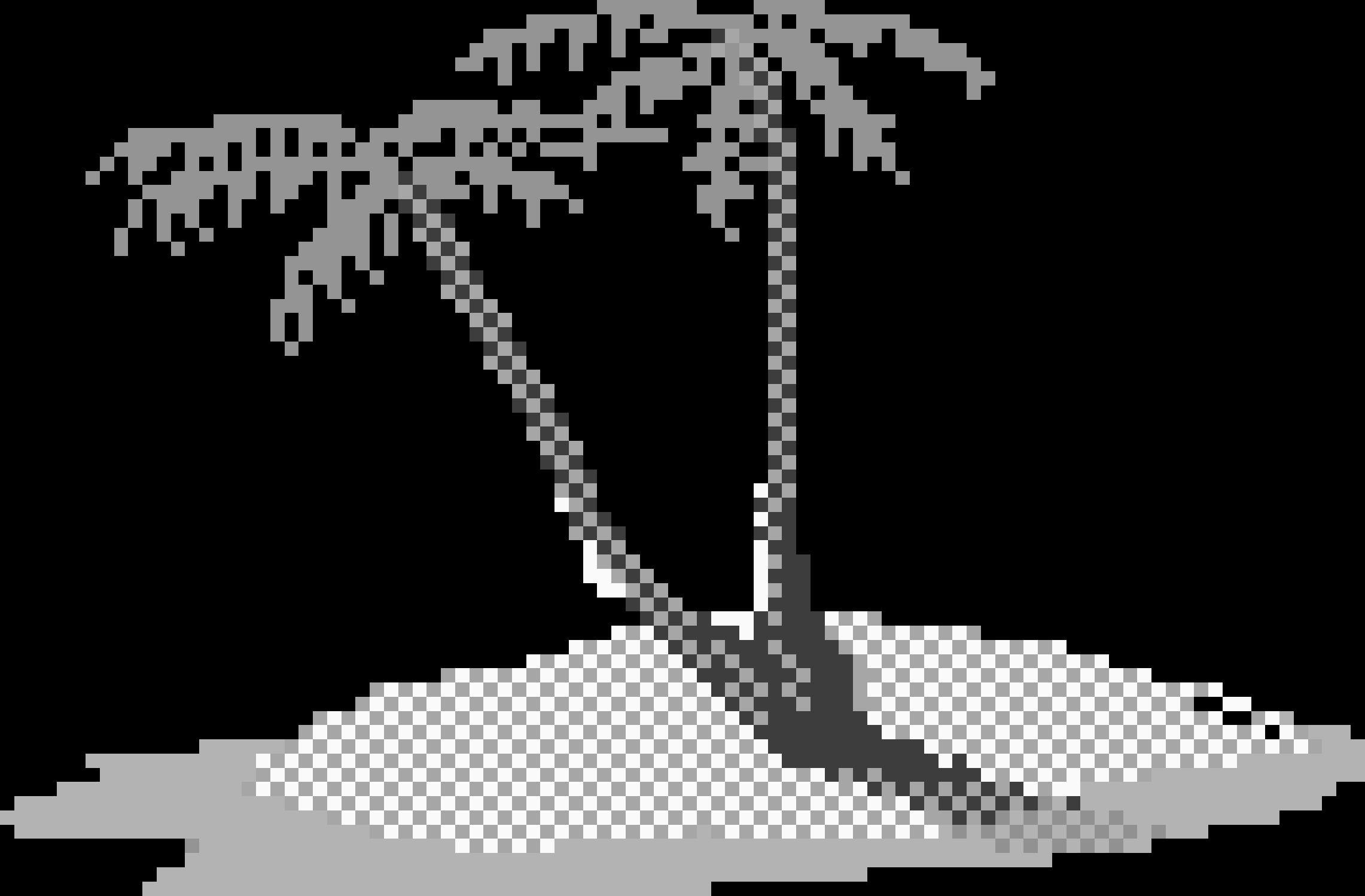
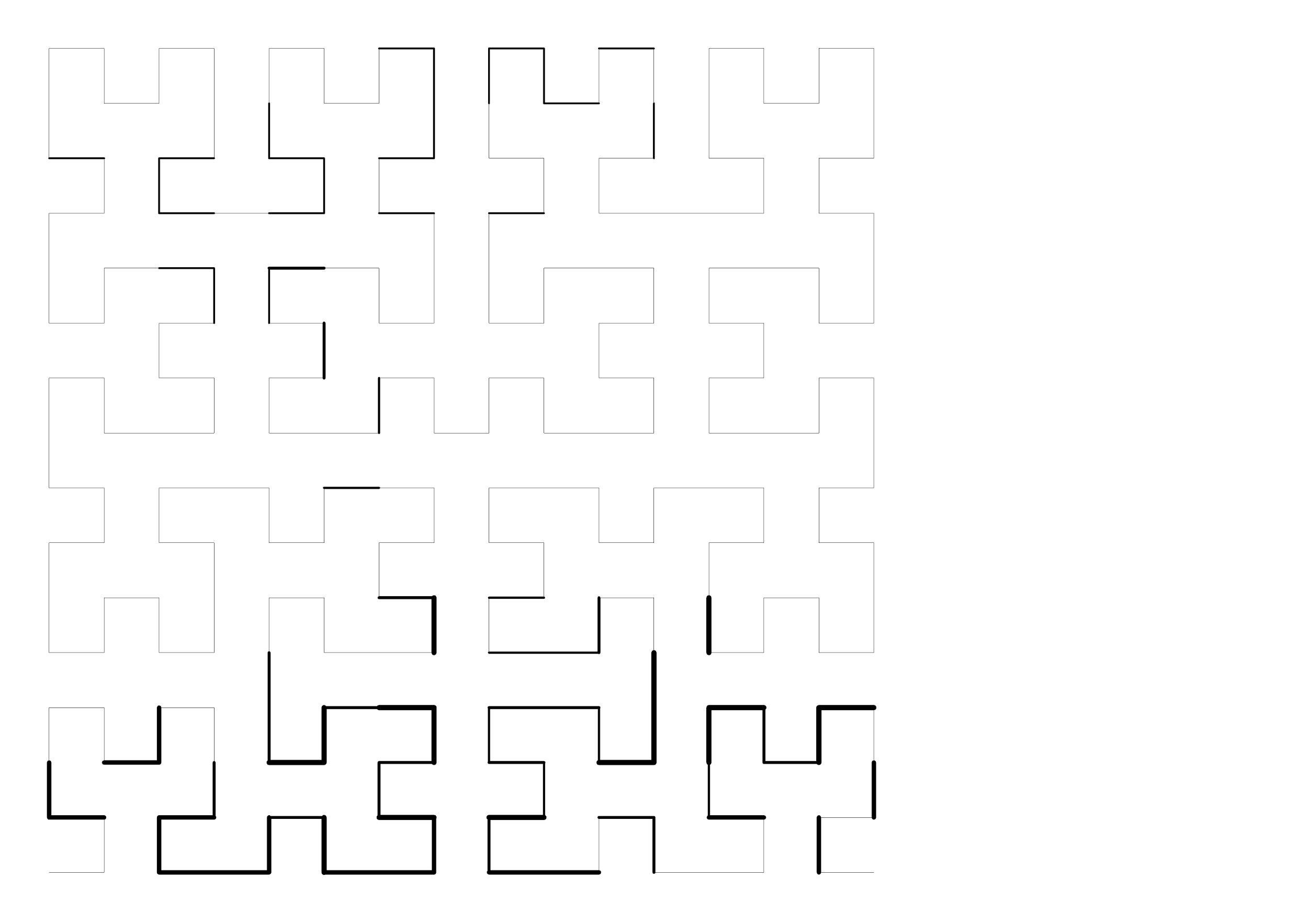
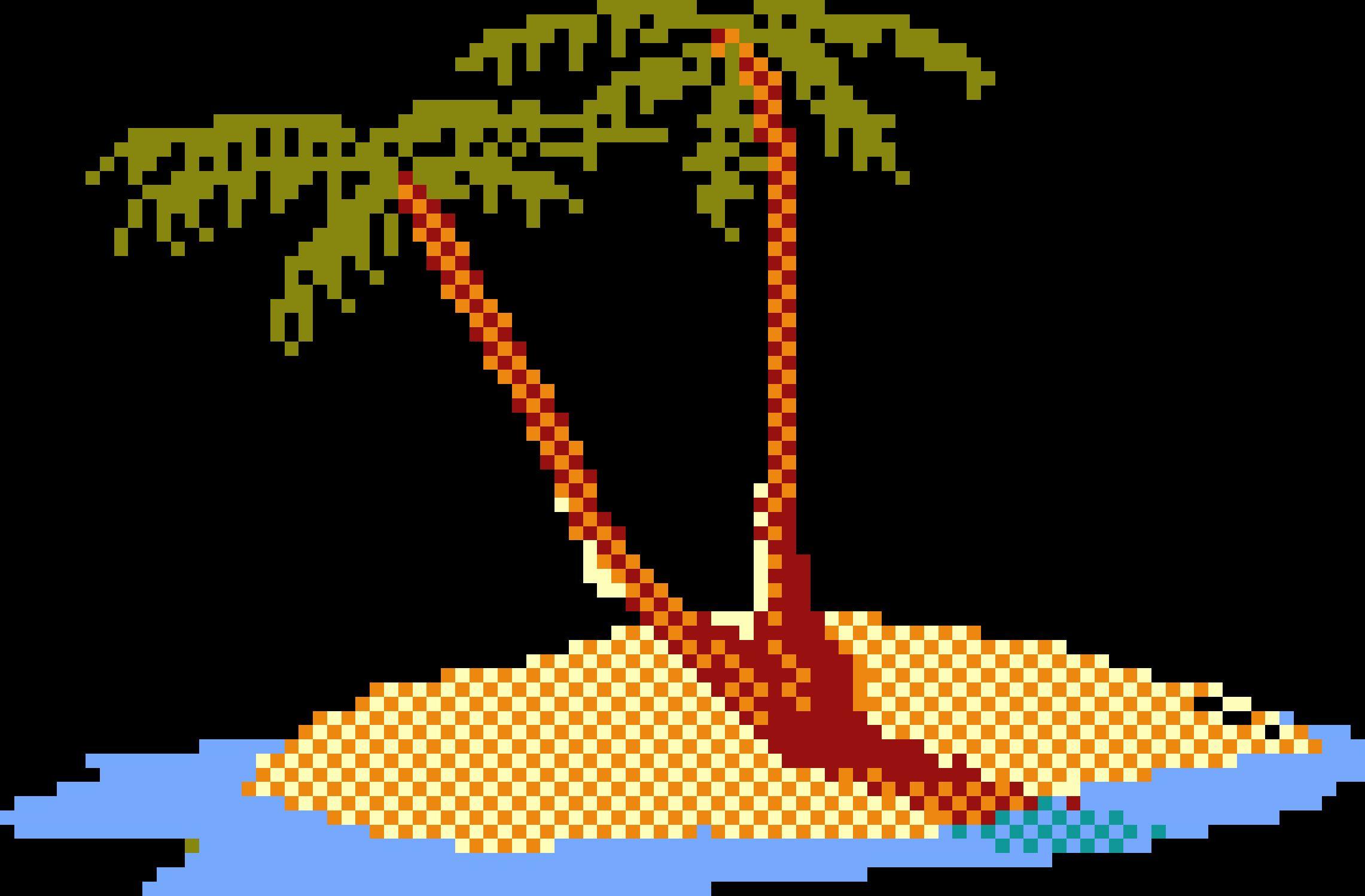
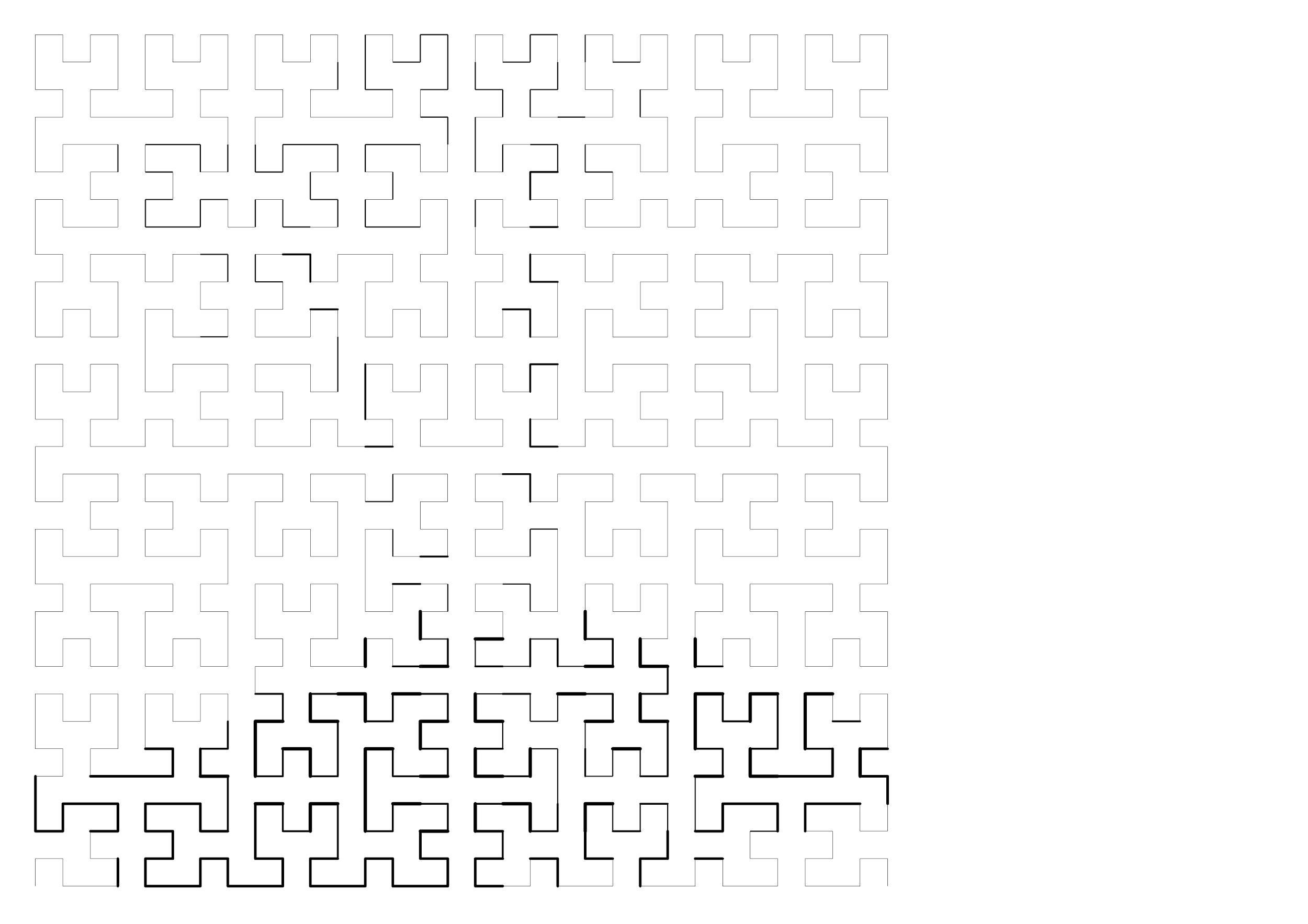
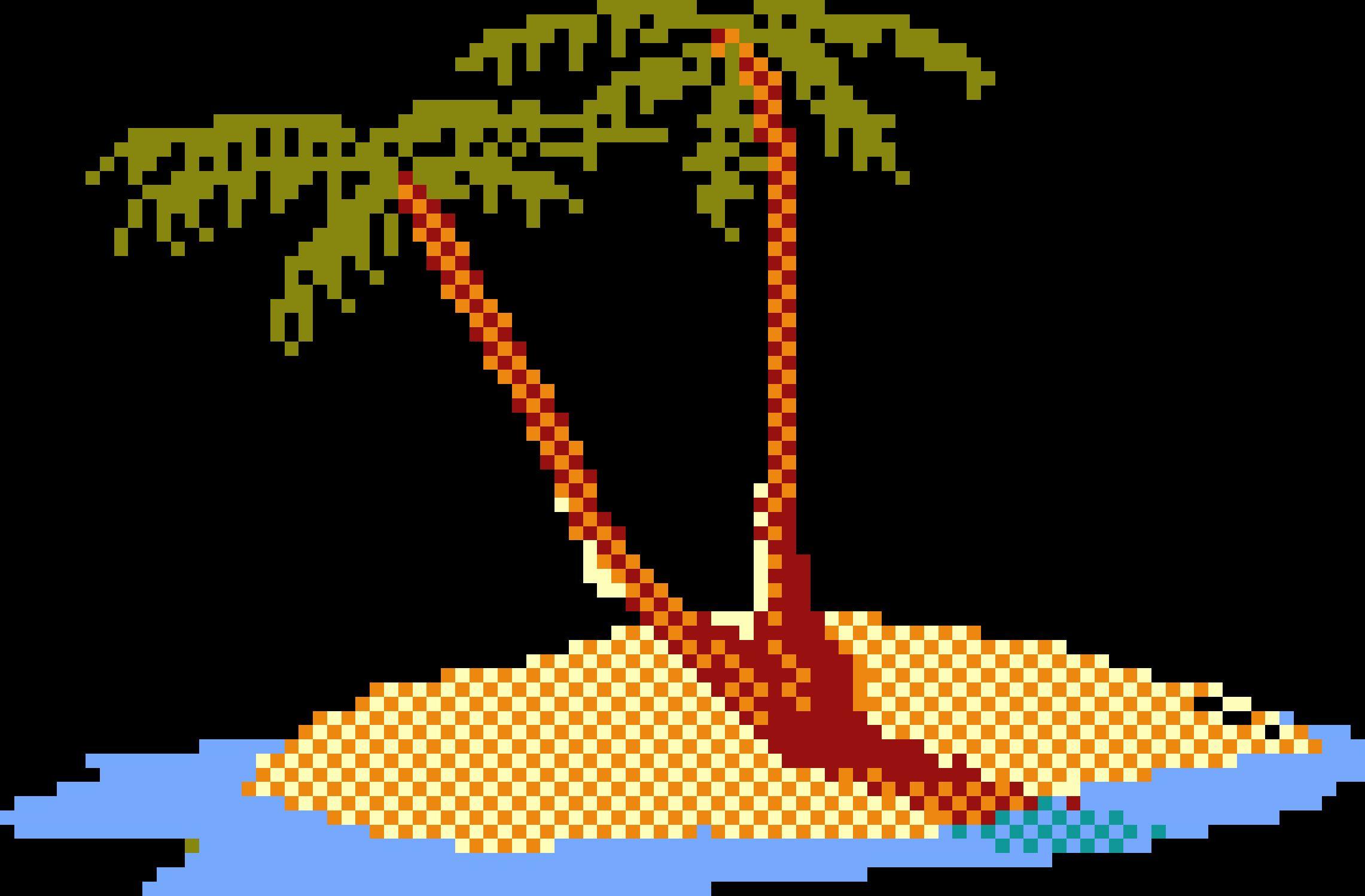
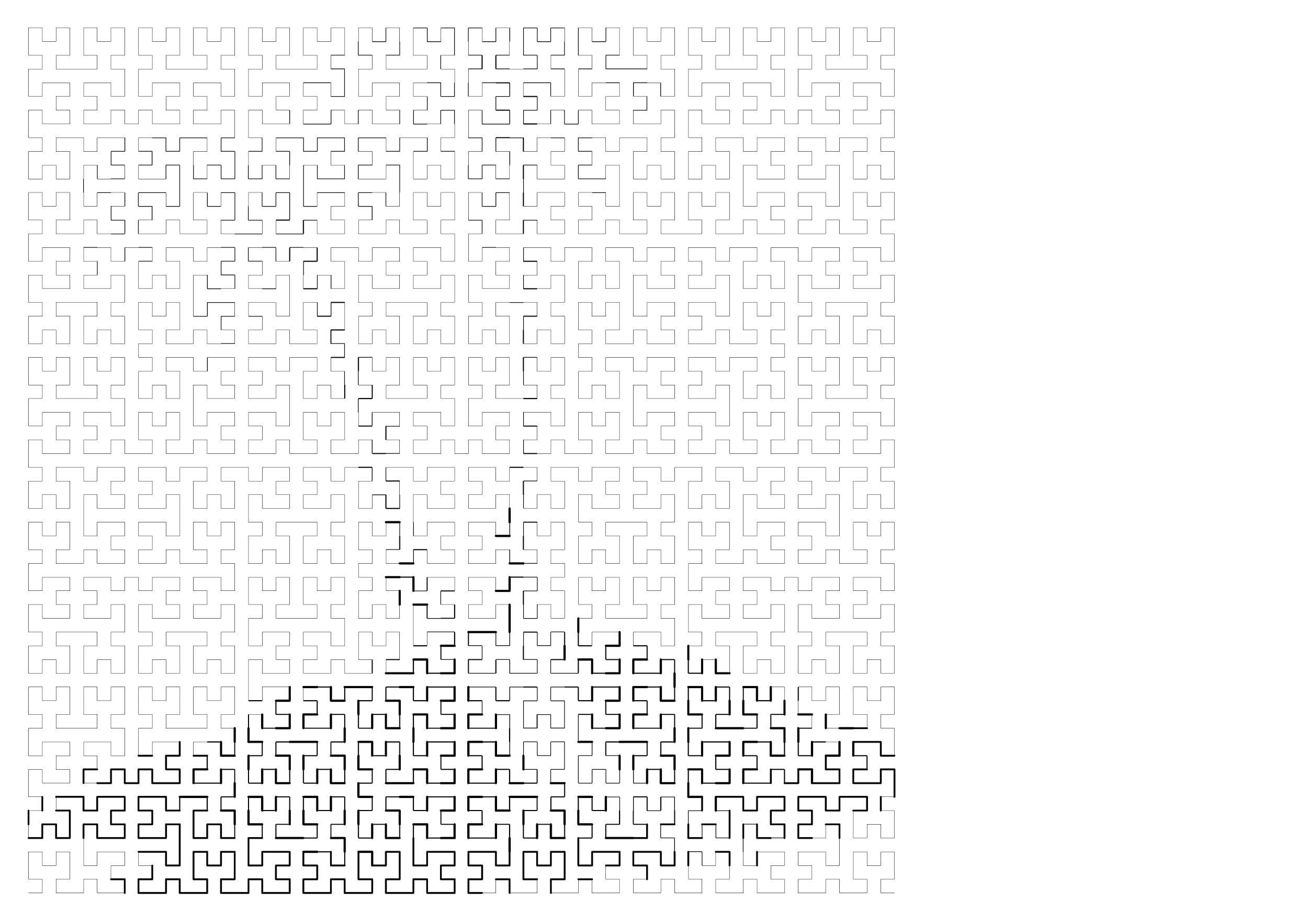
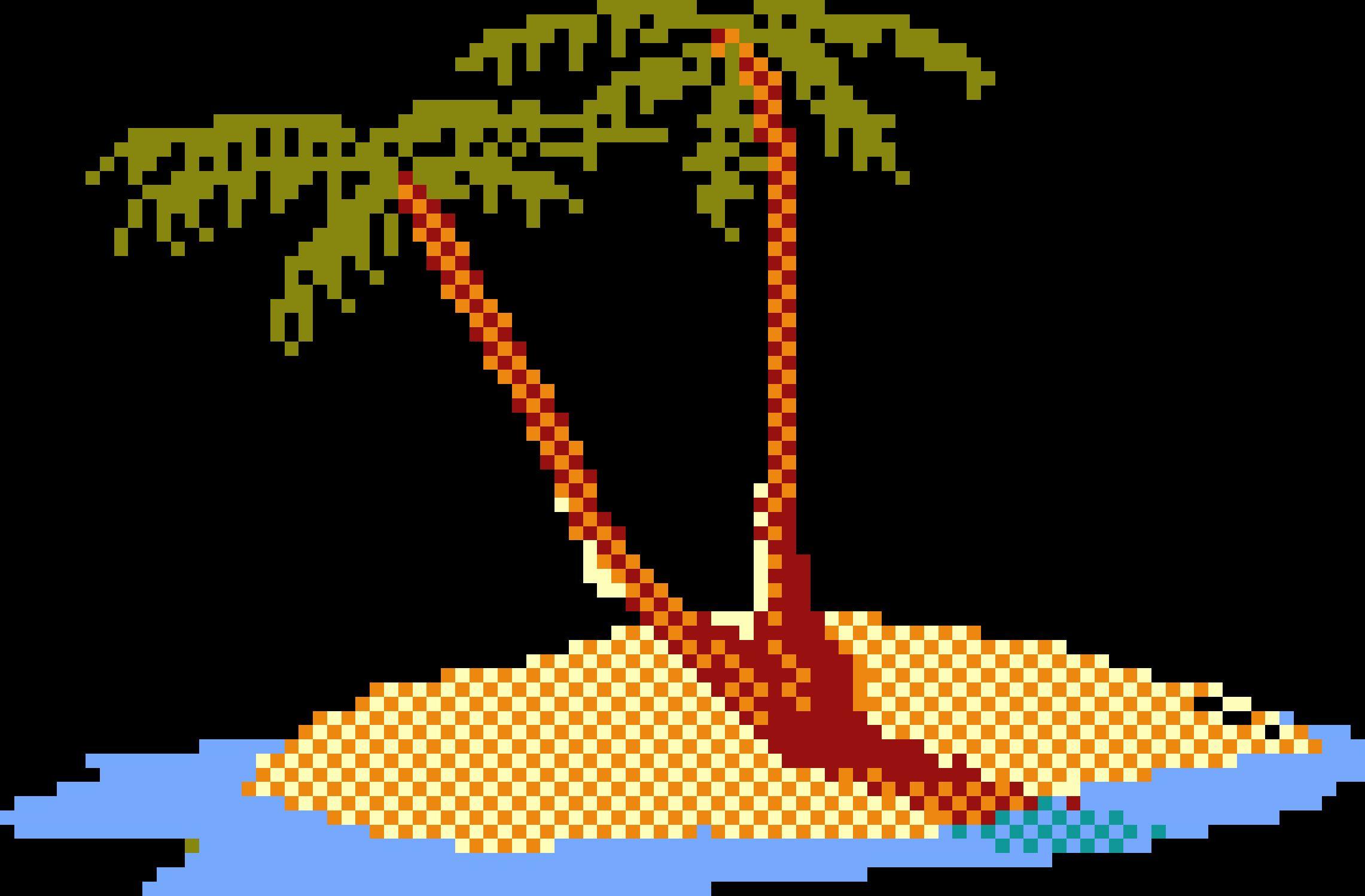
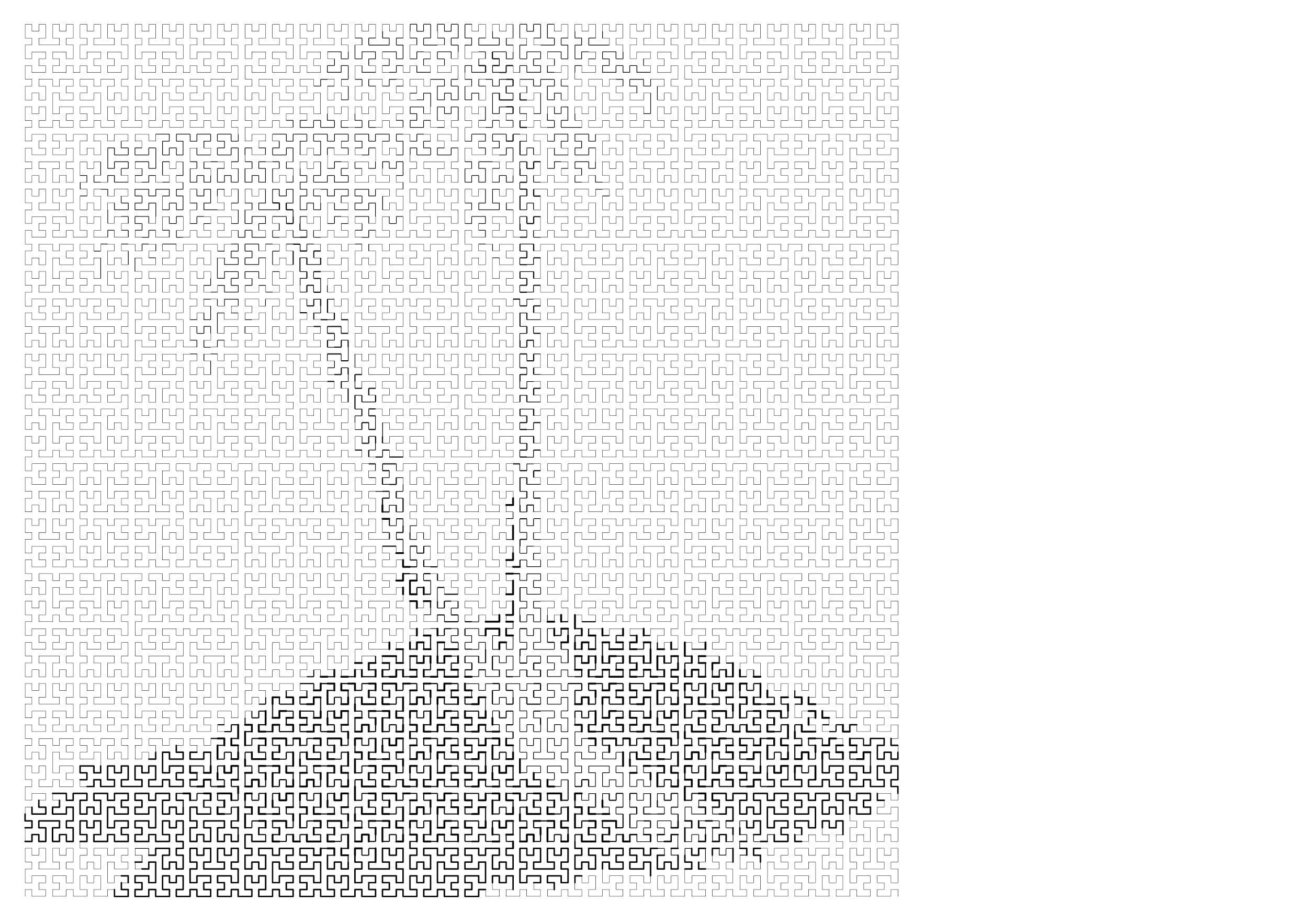
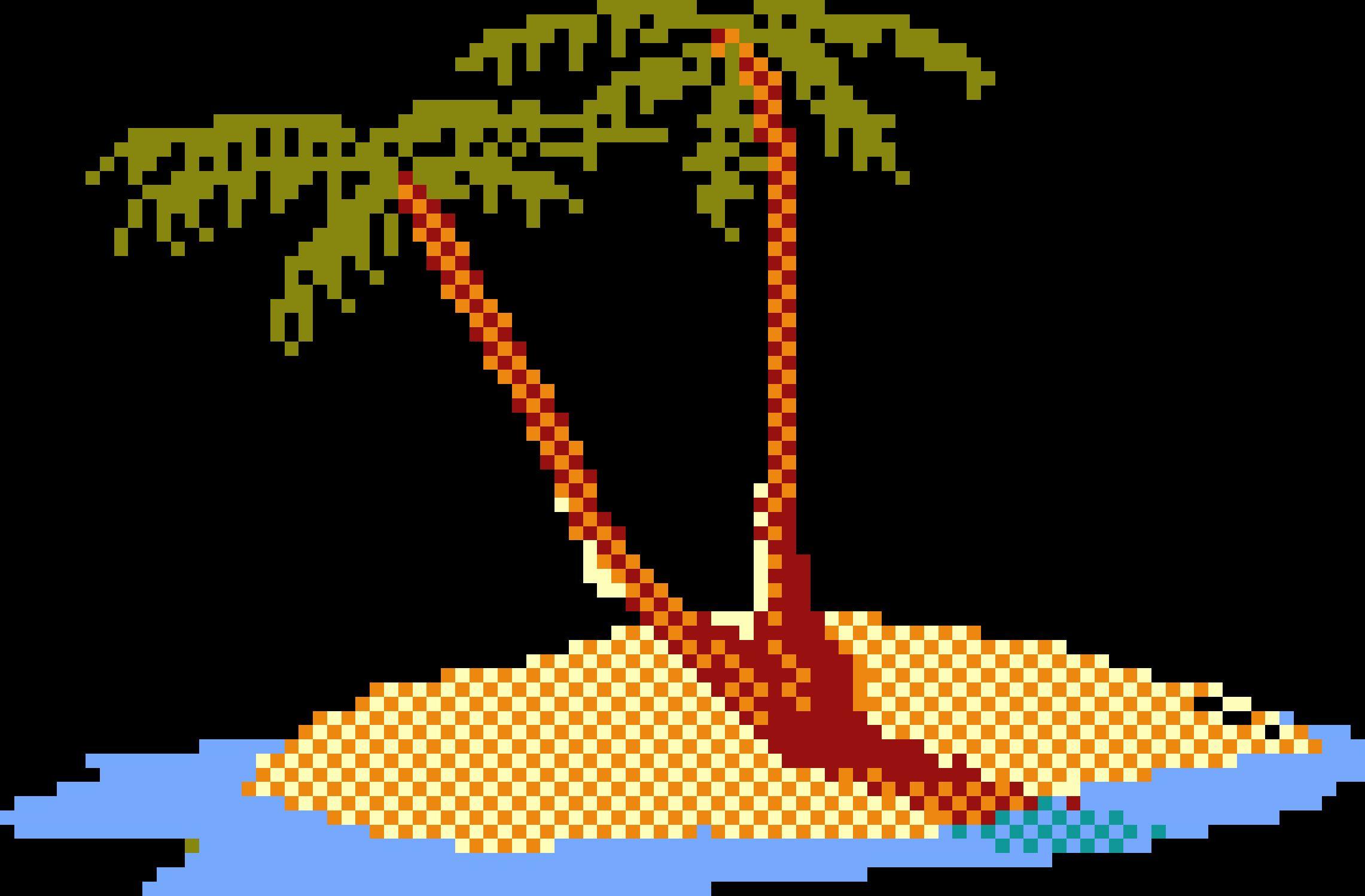
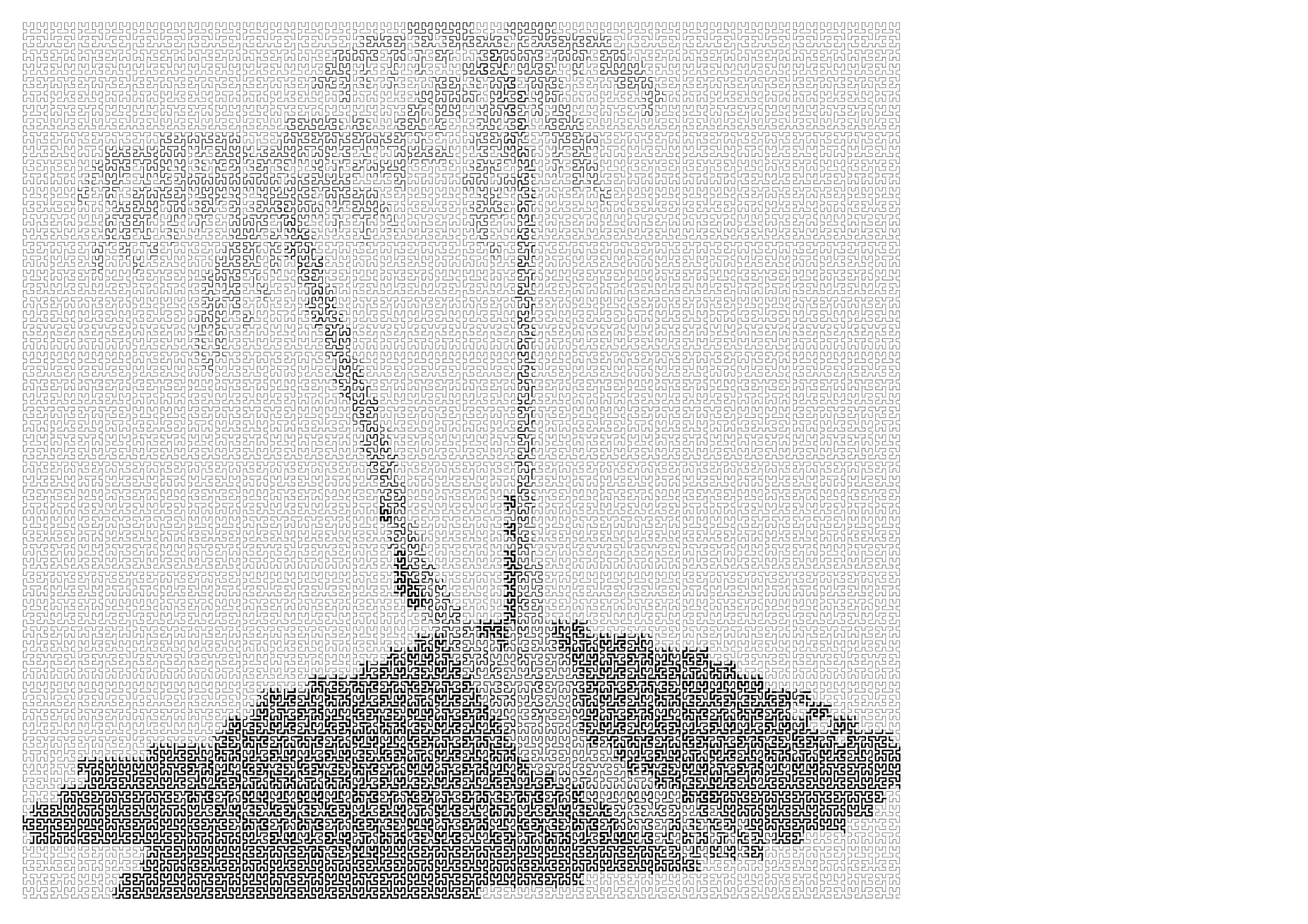
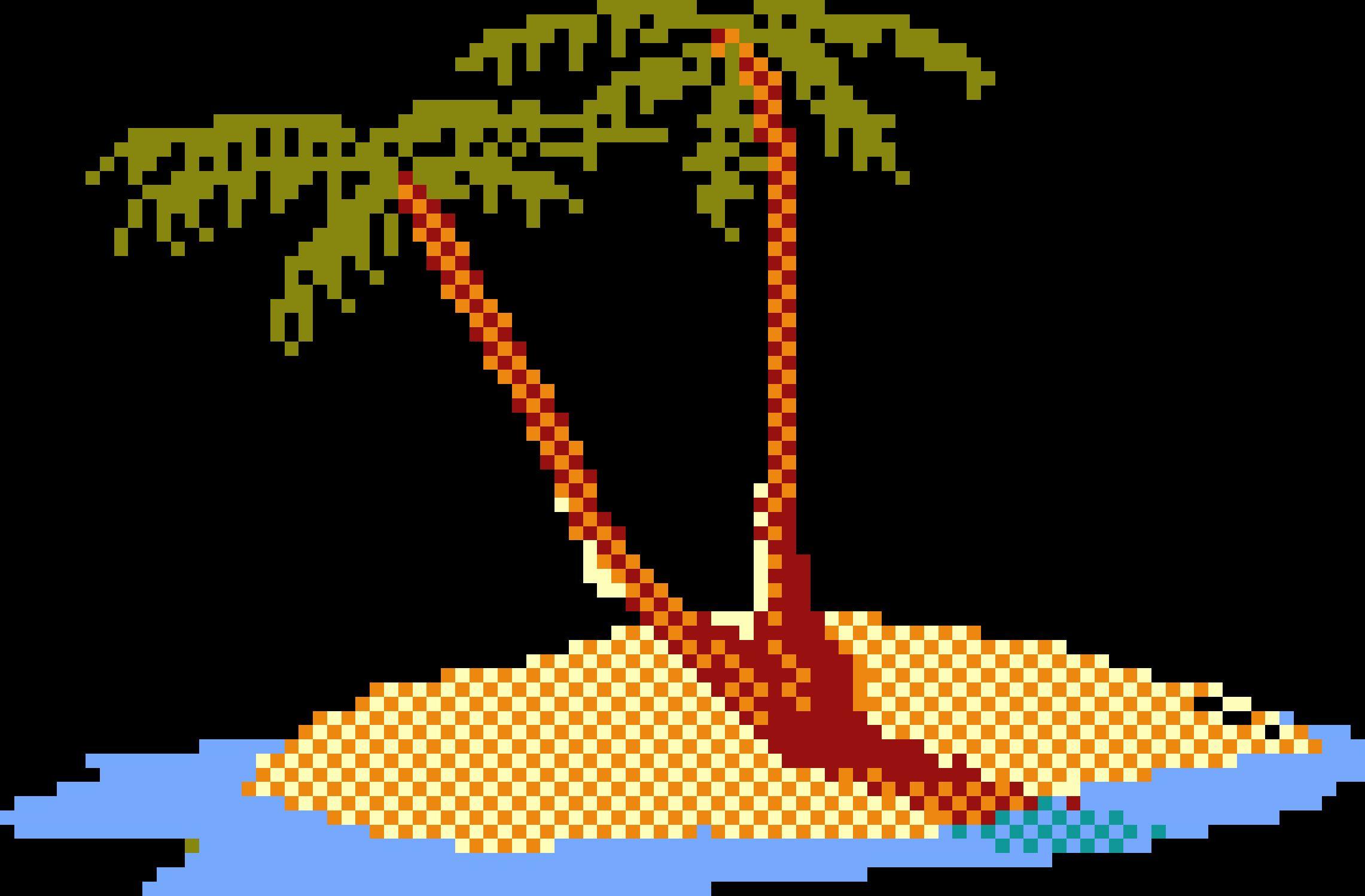
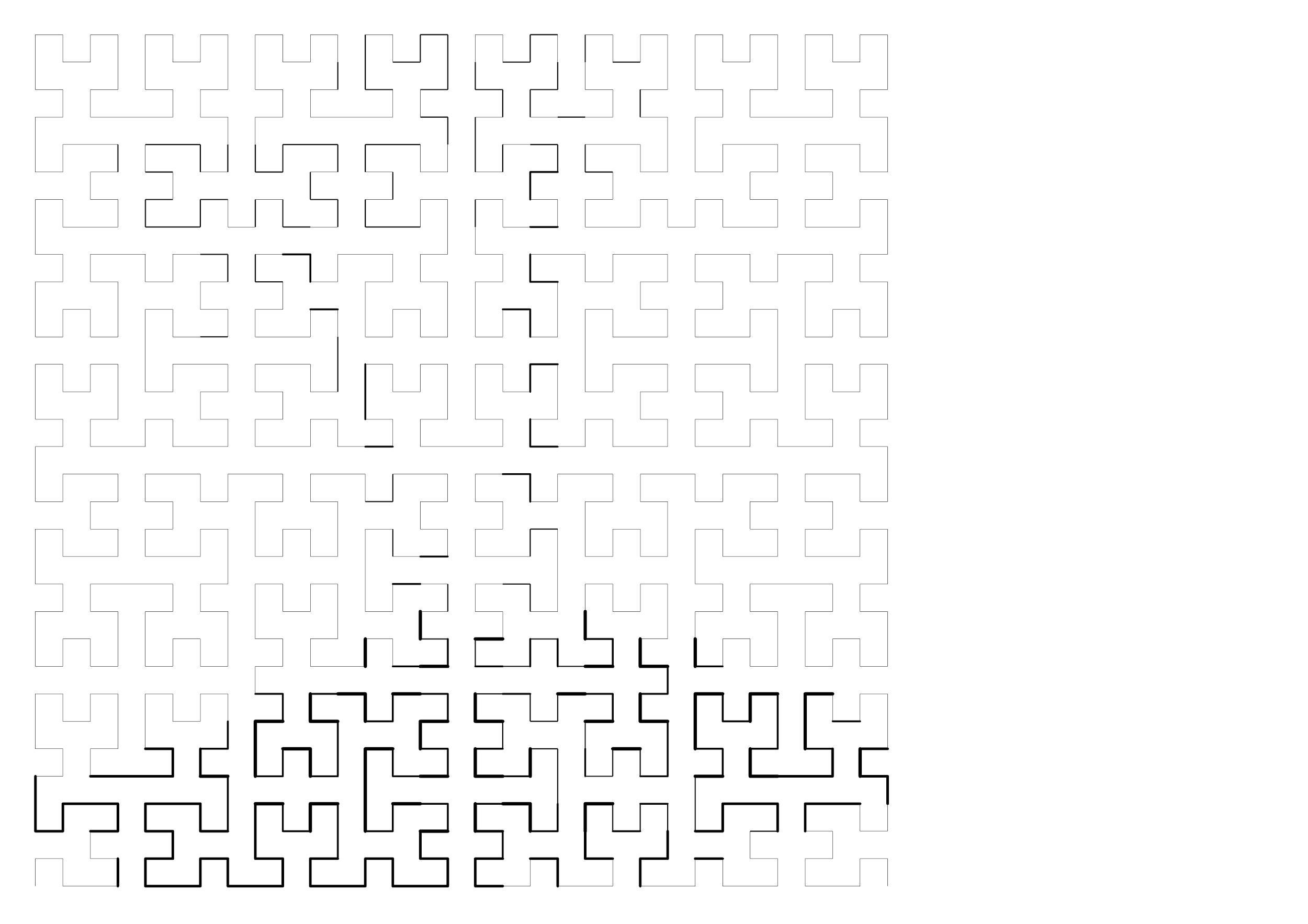
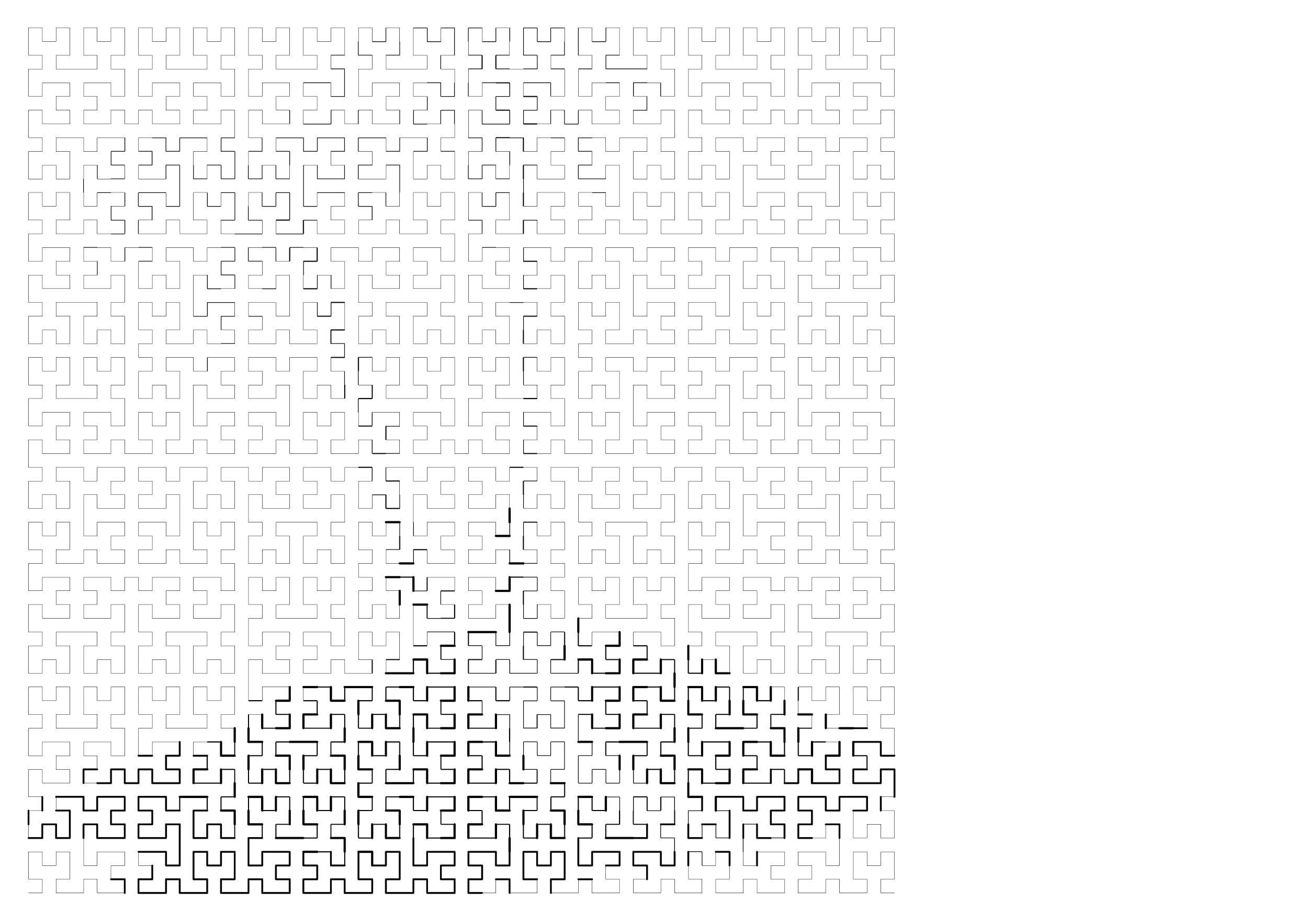
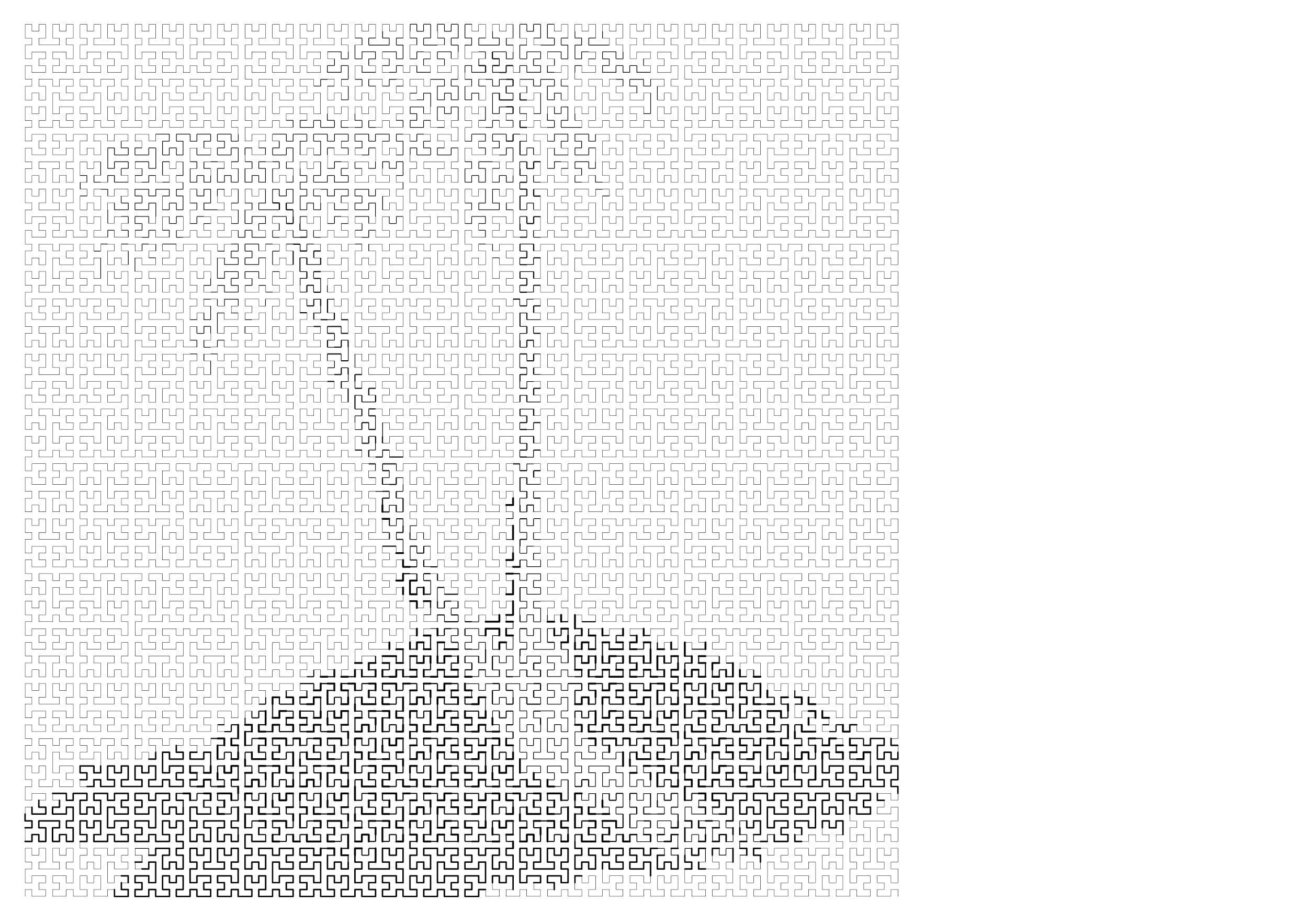
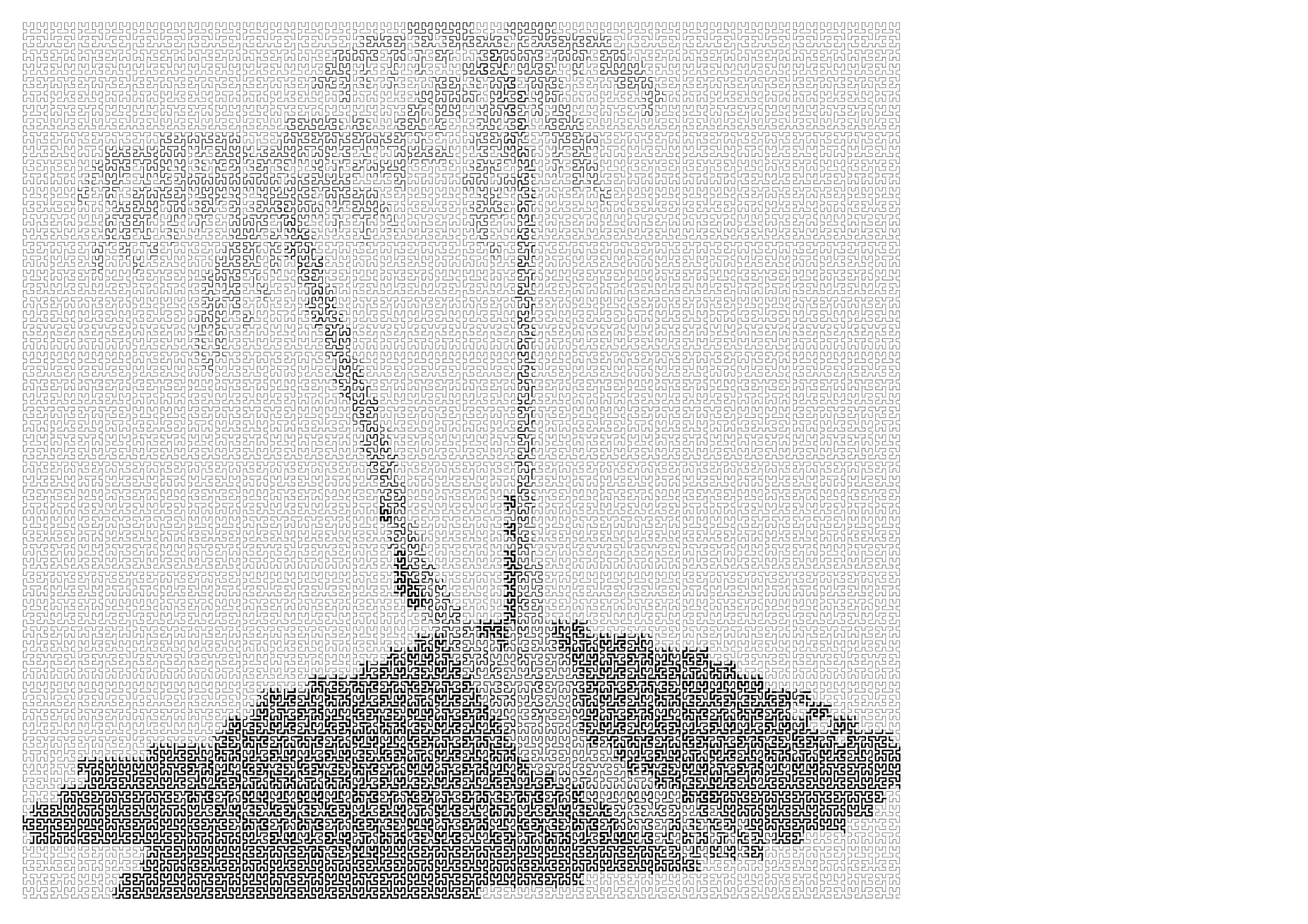
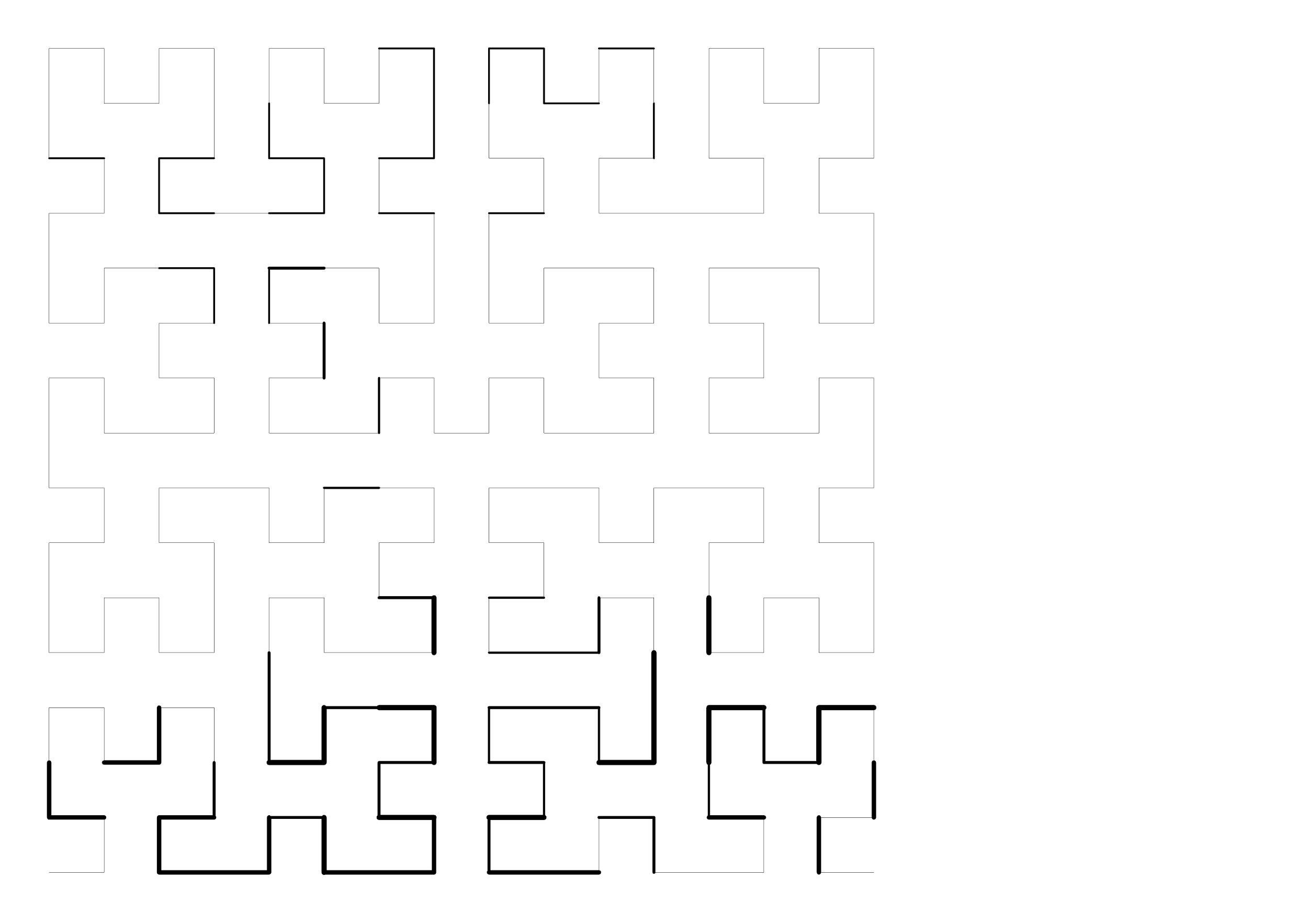
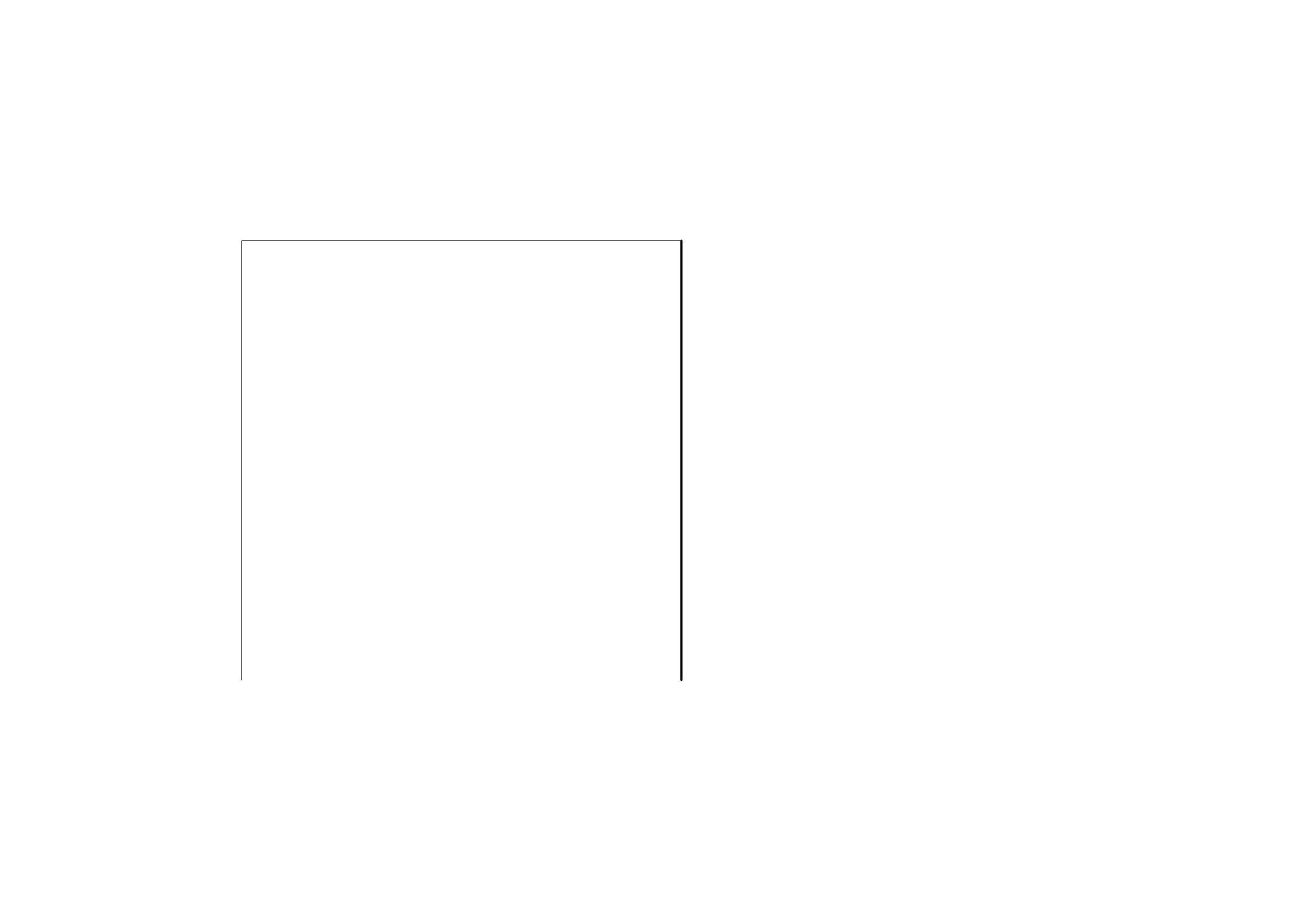
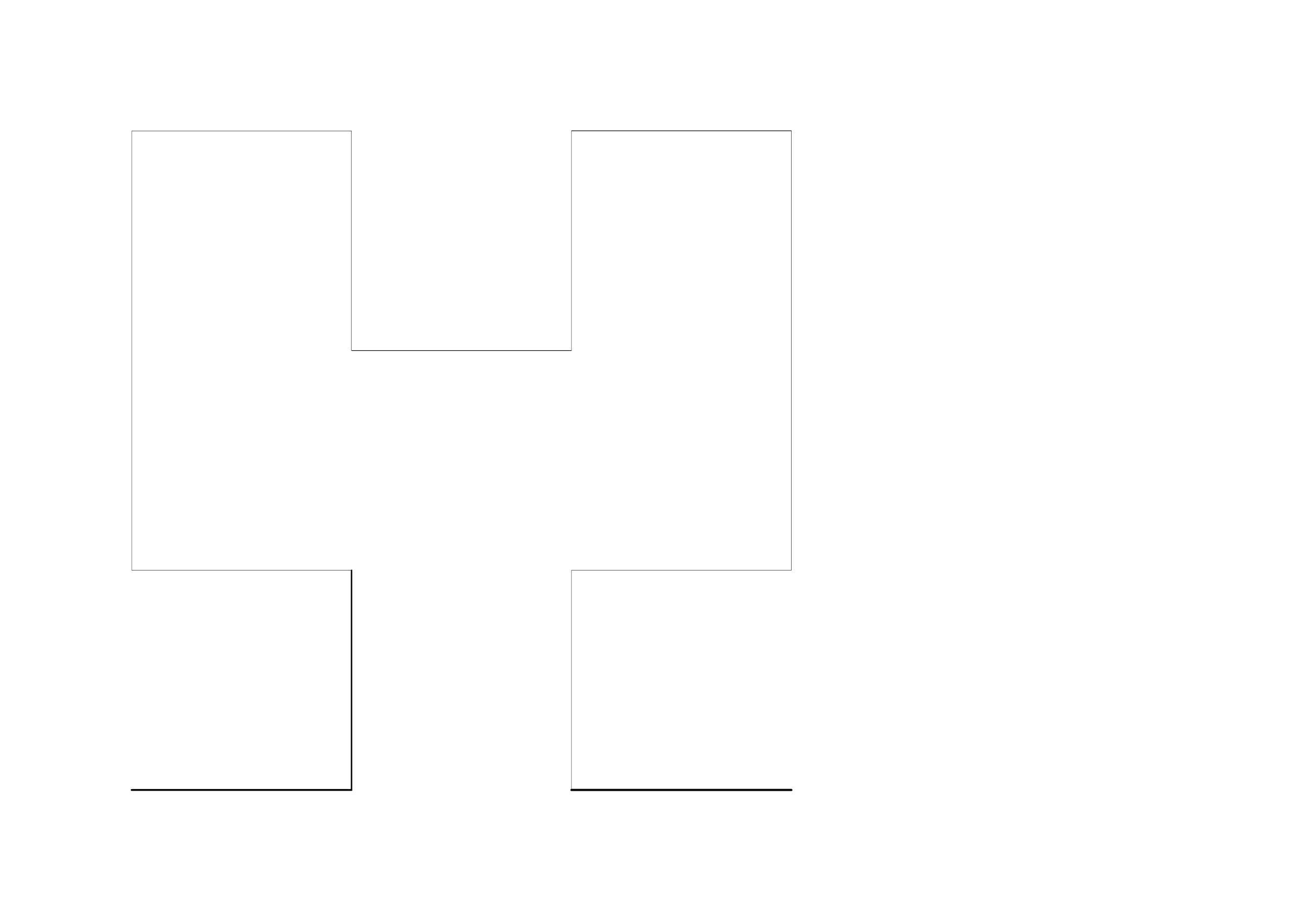
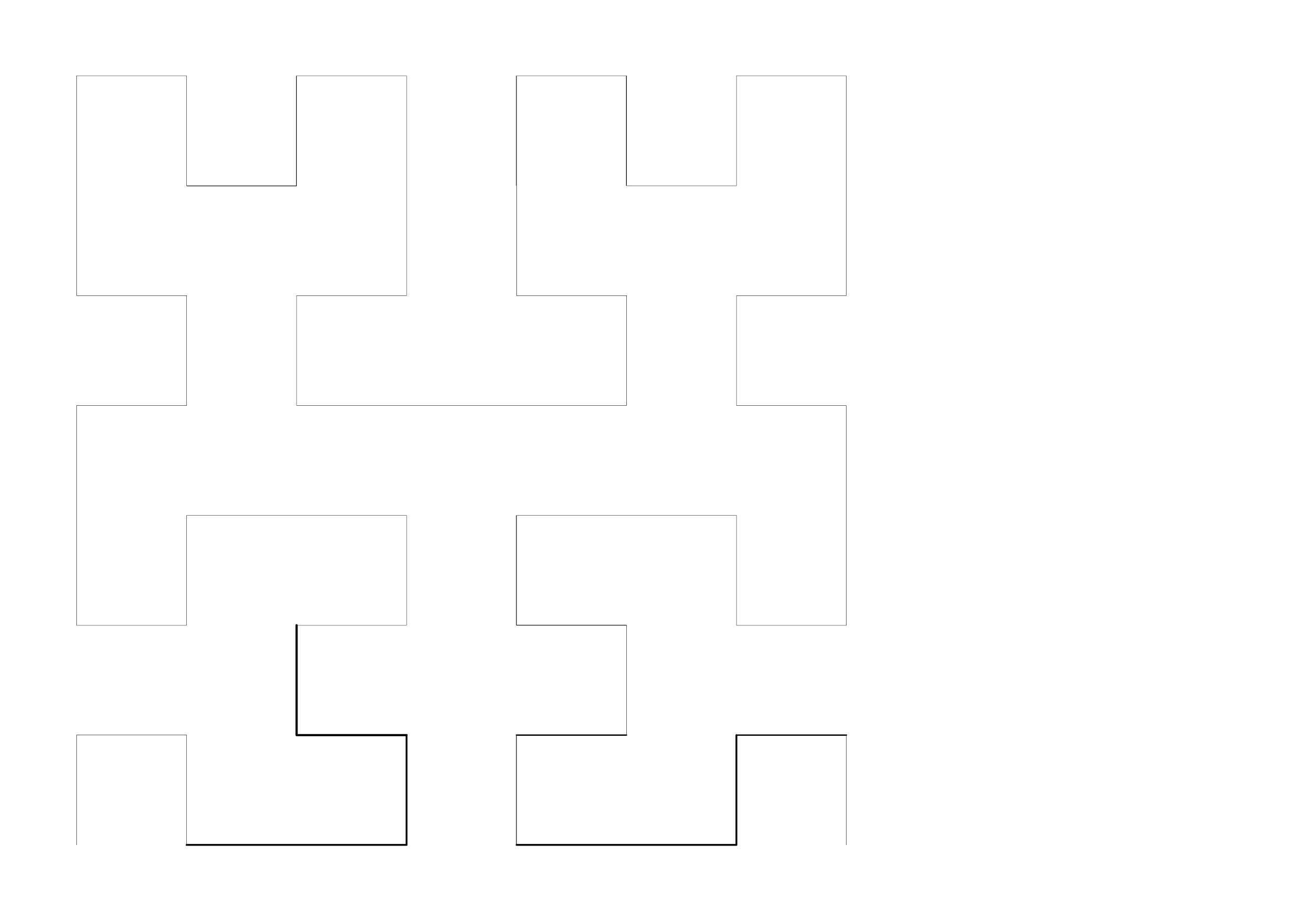
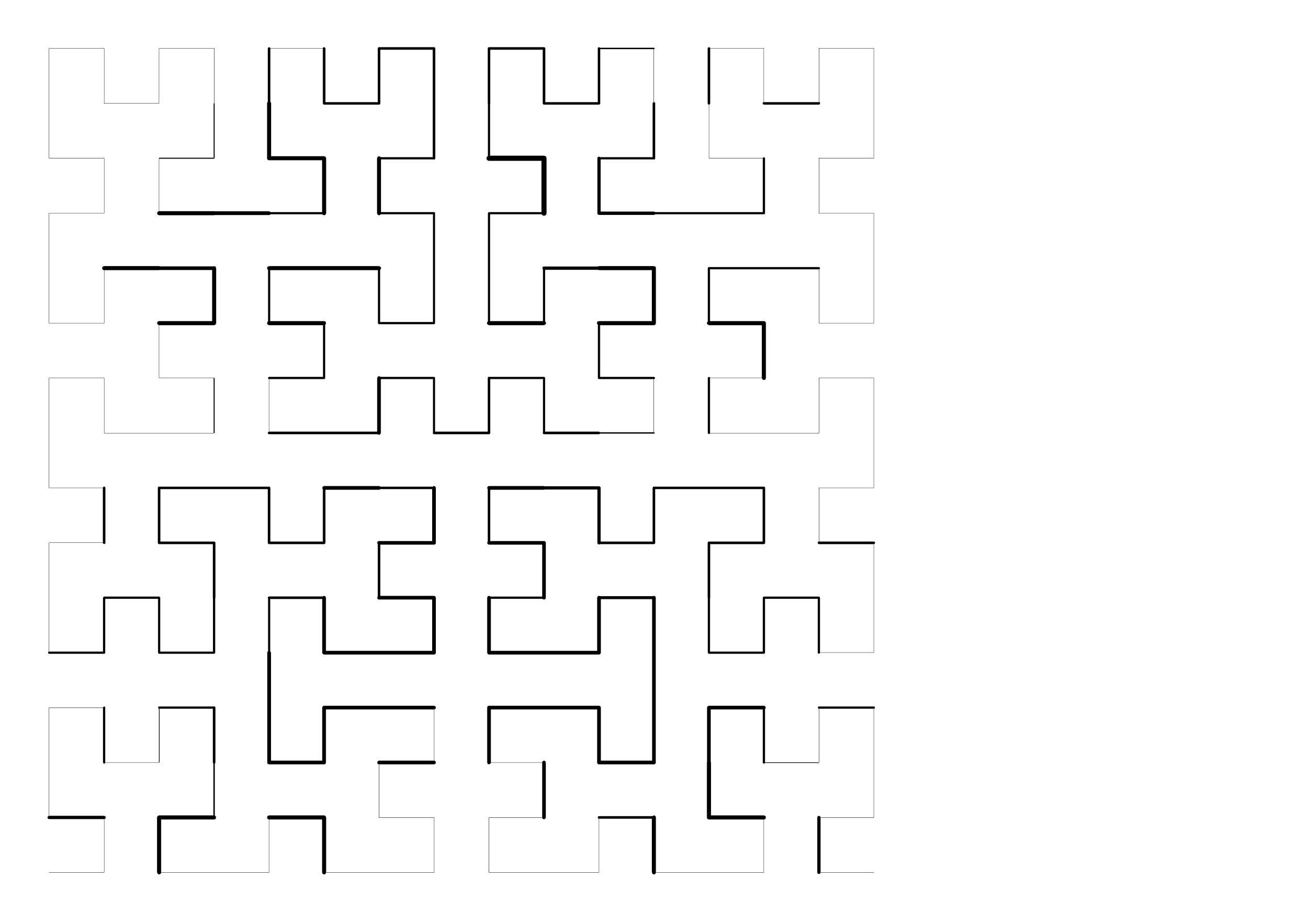
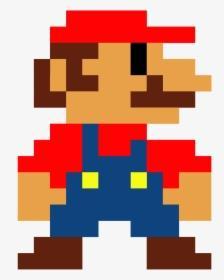
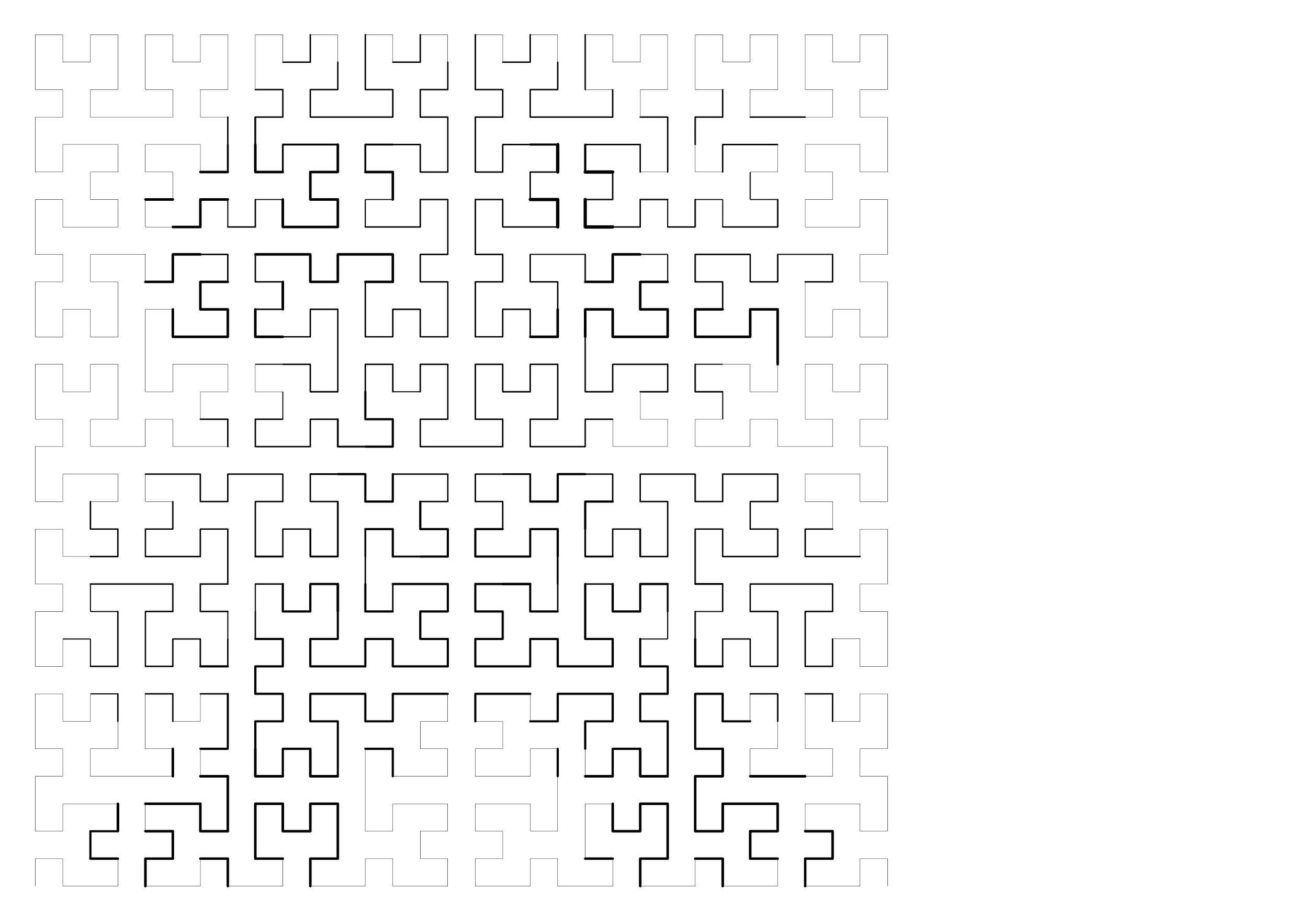
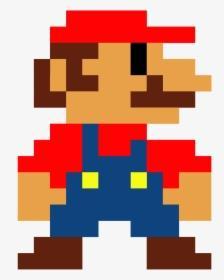
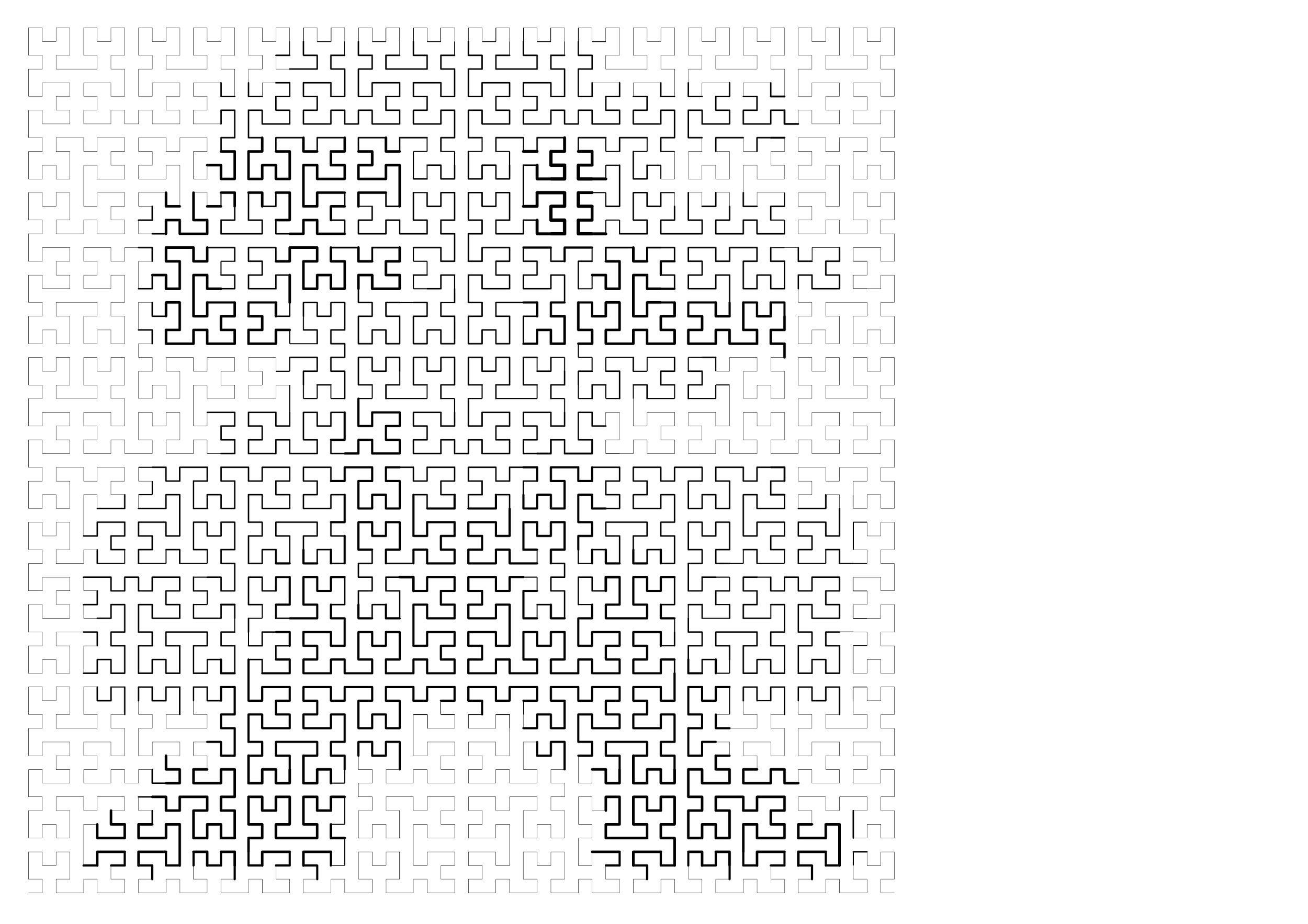
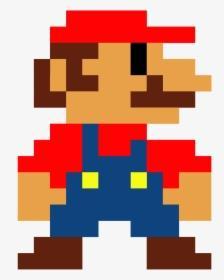
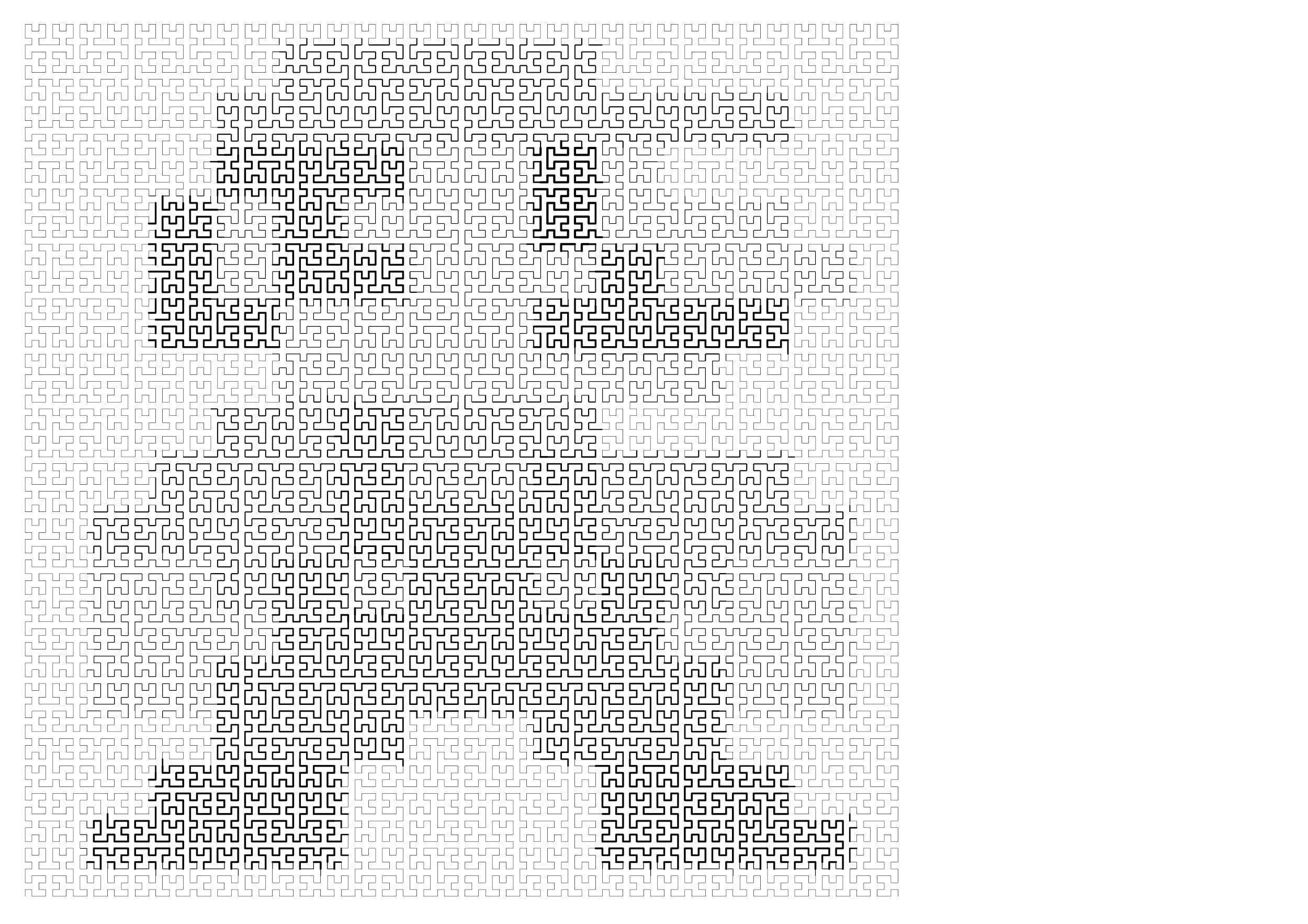
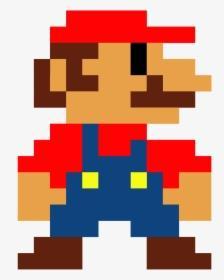
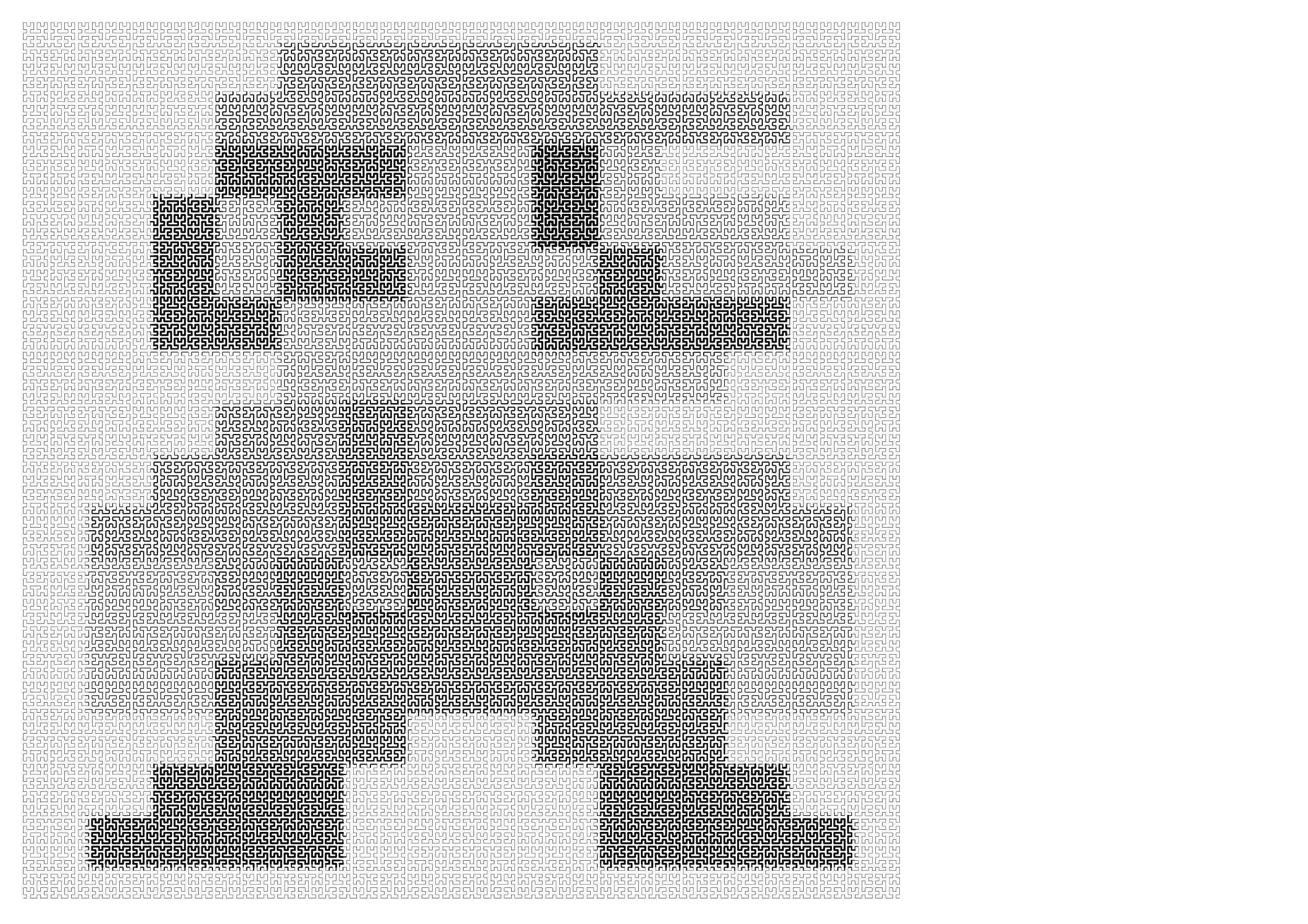
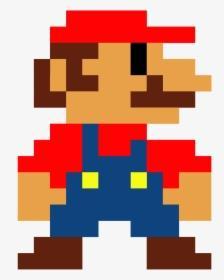
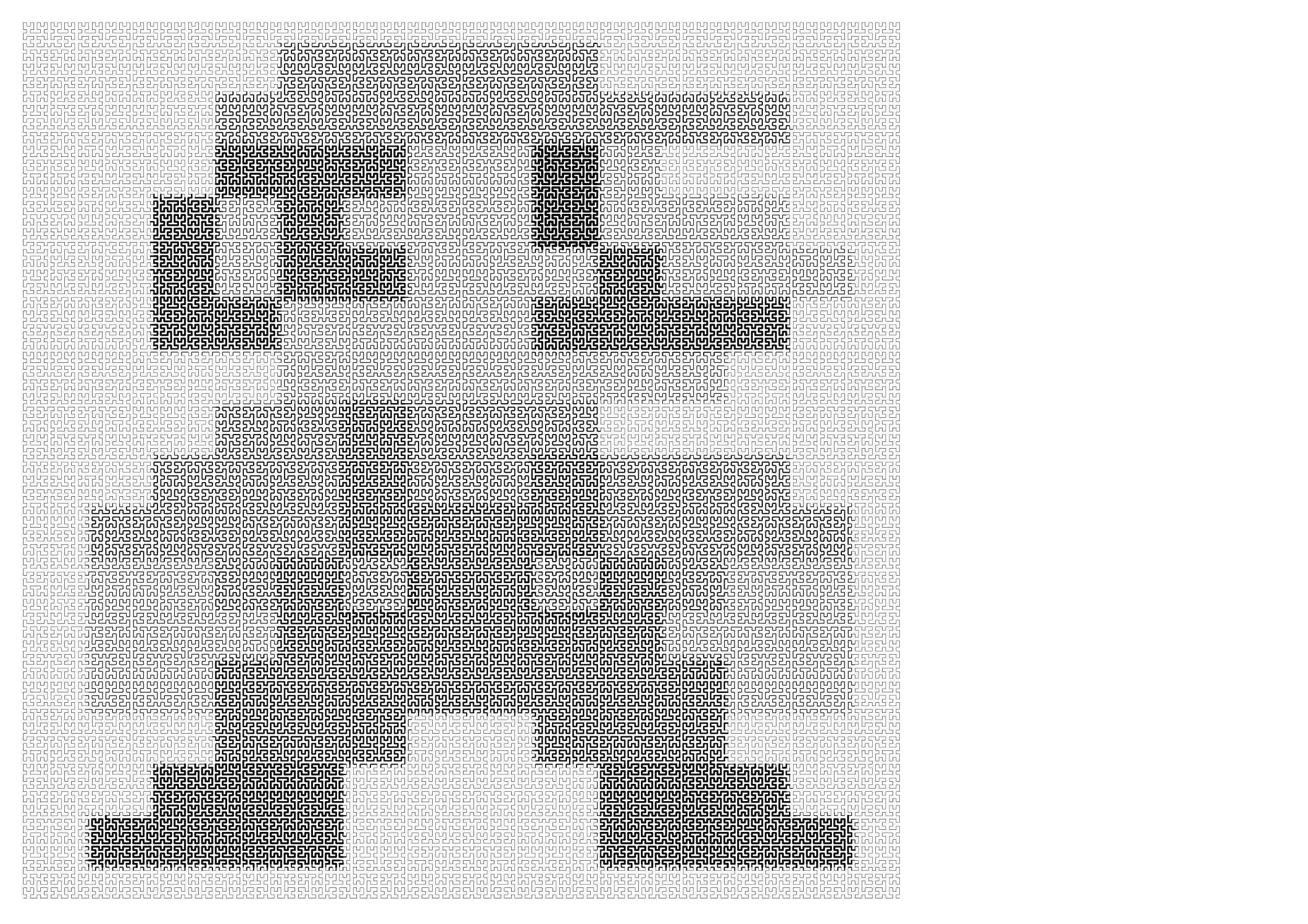
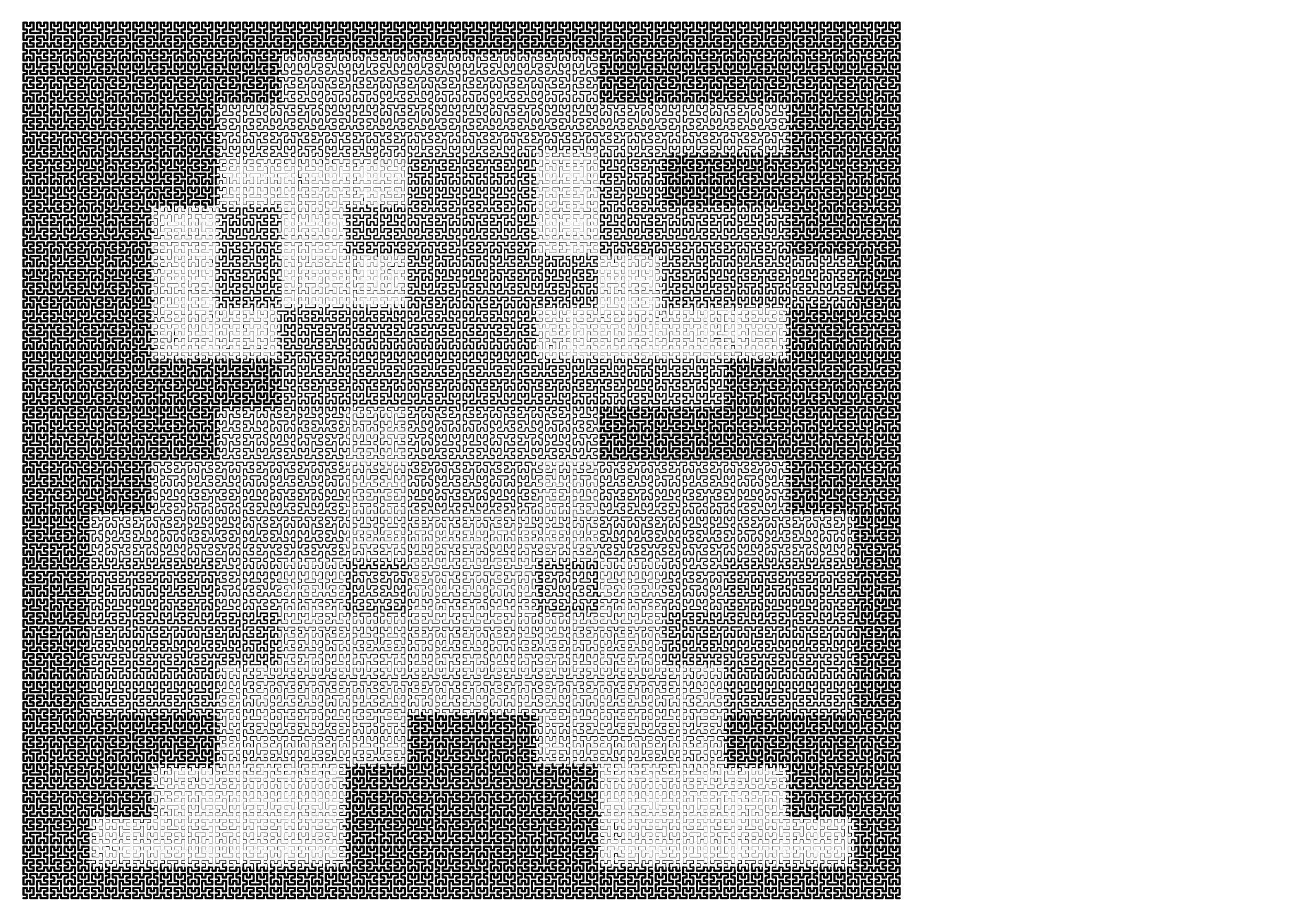
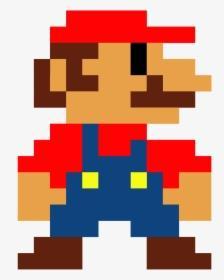
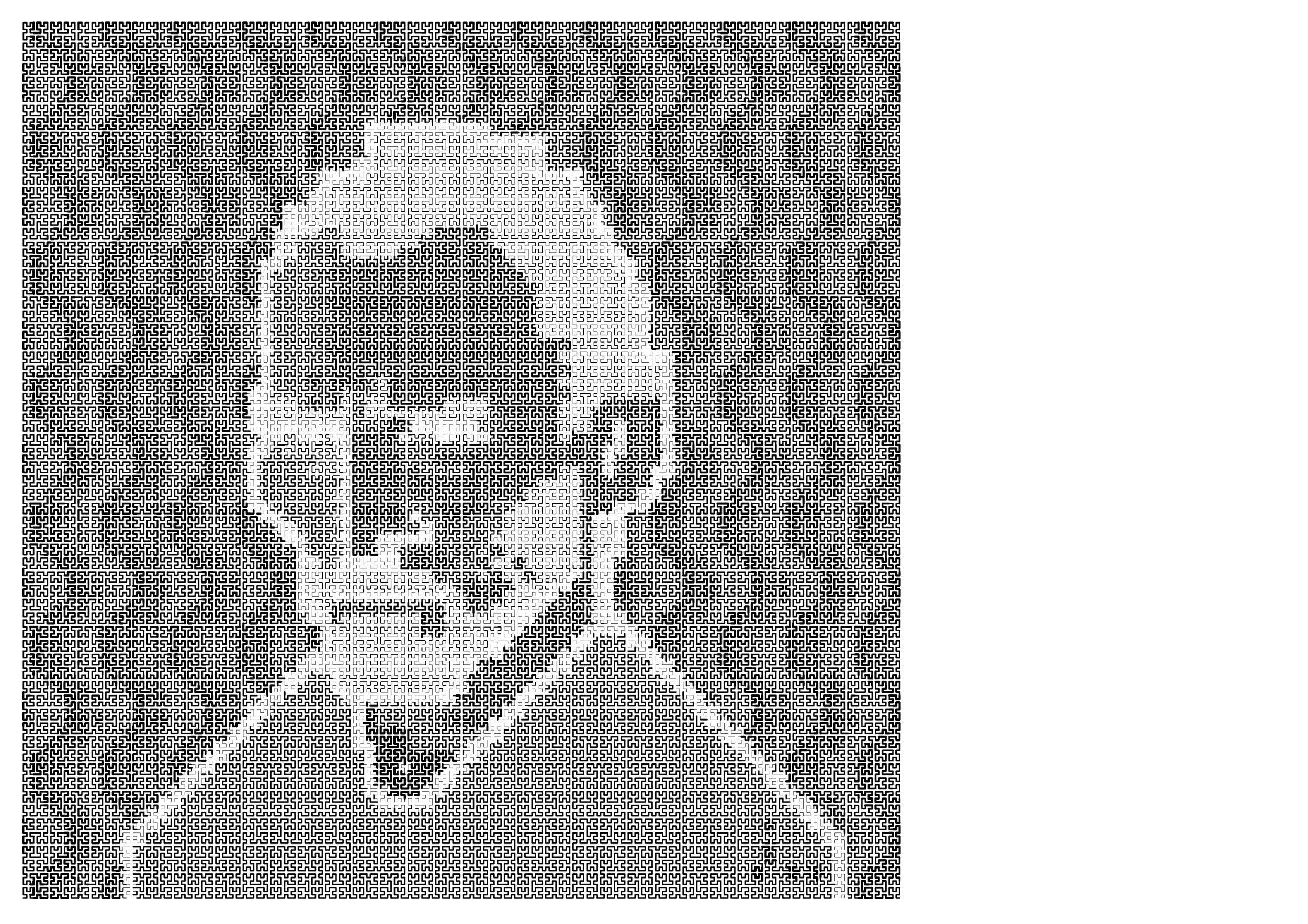
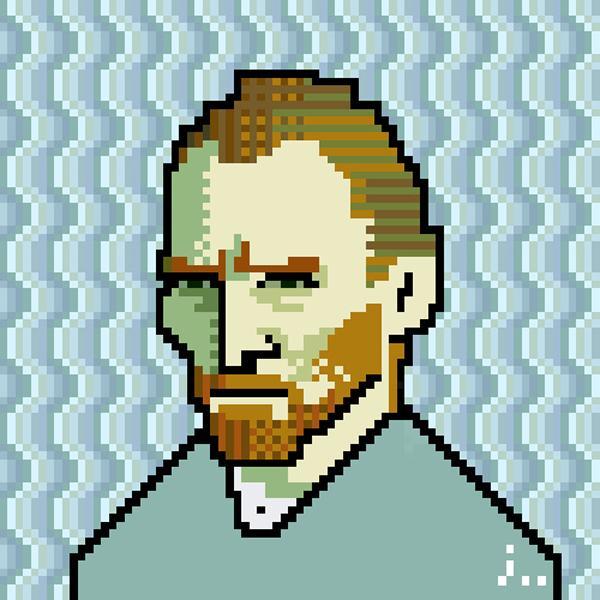
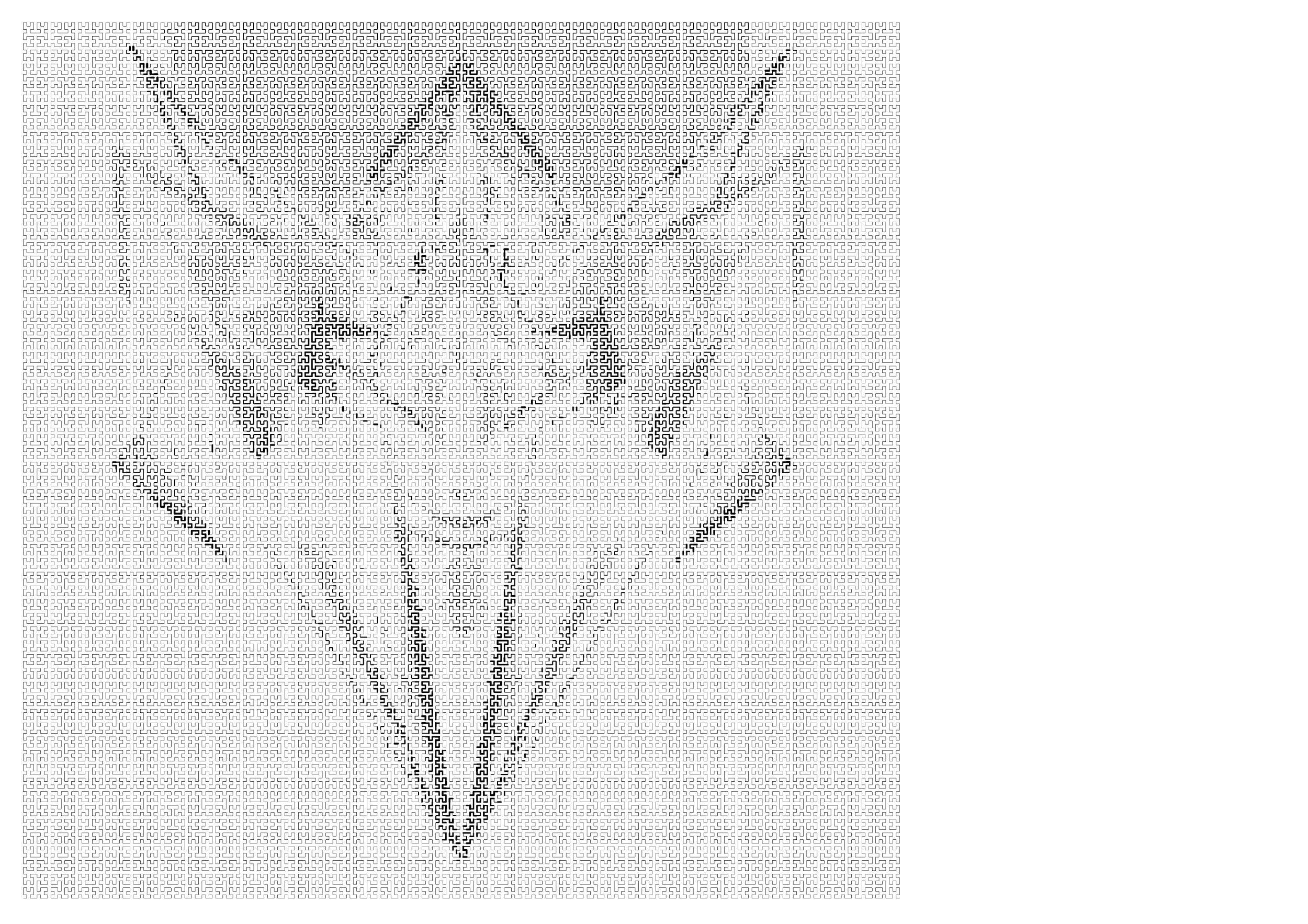
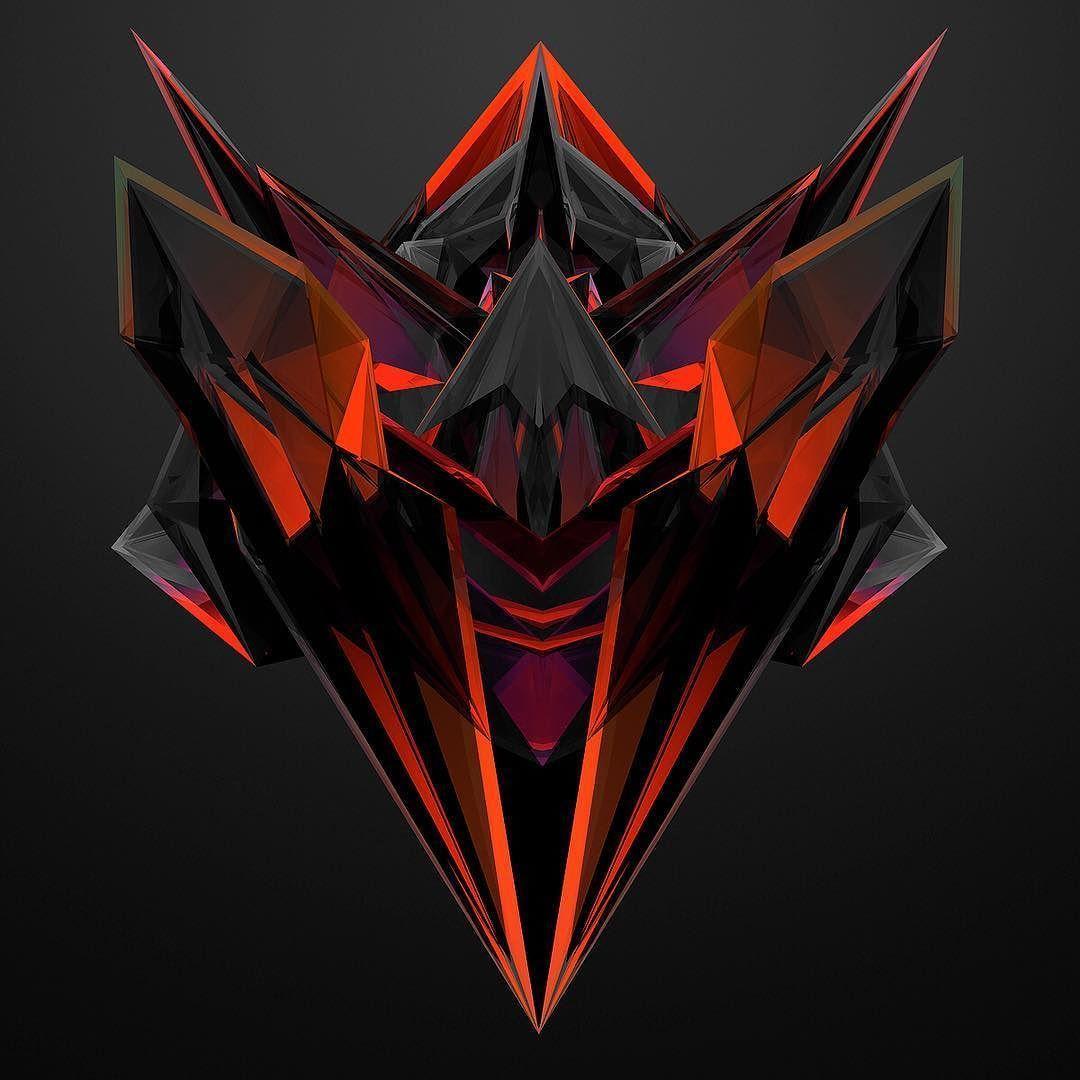
MORE EXAMPLES
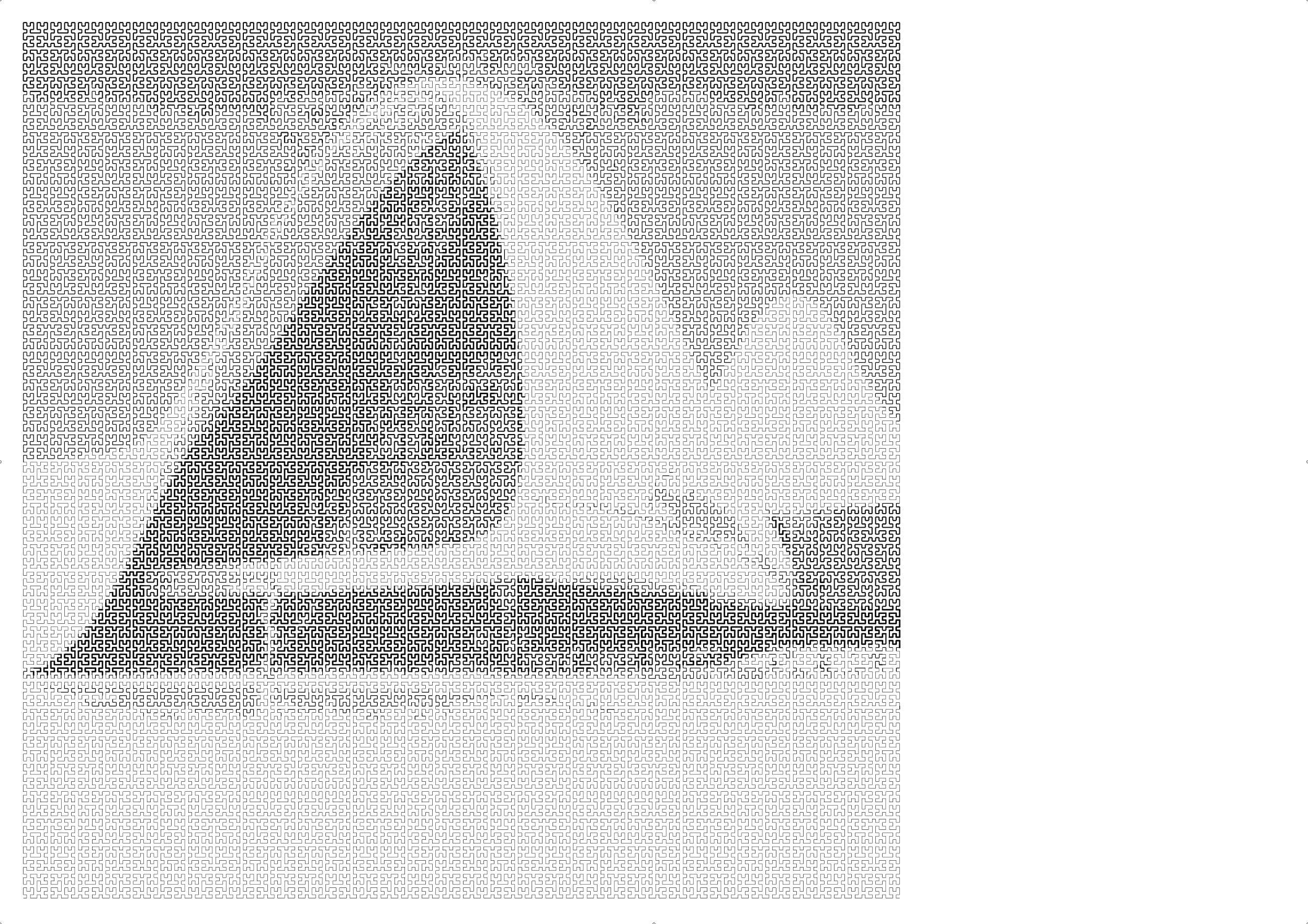
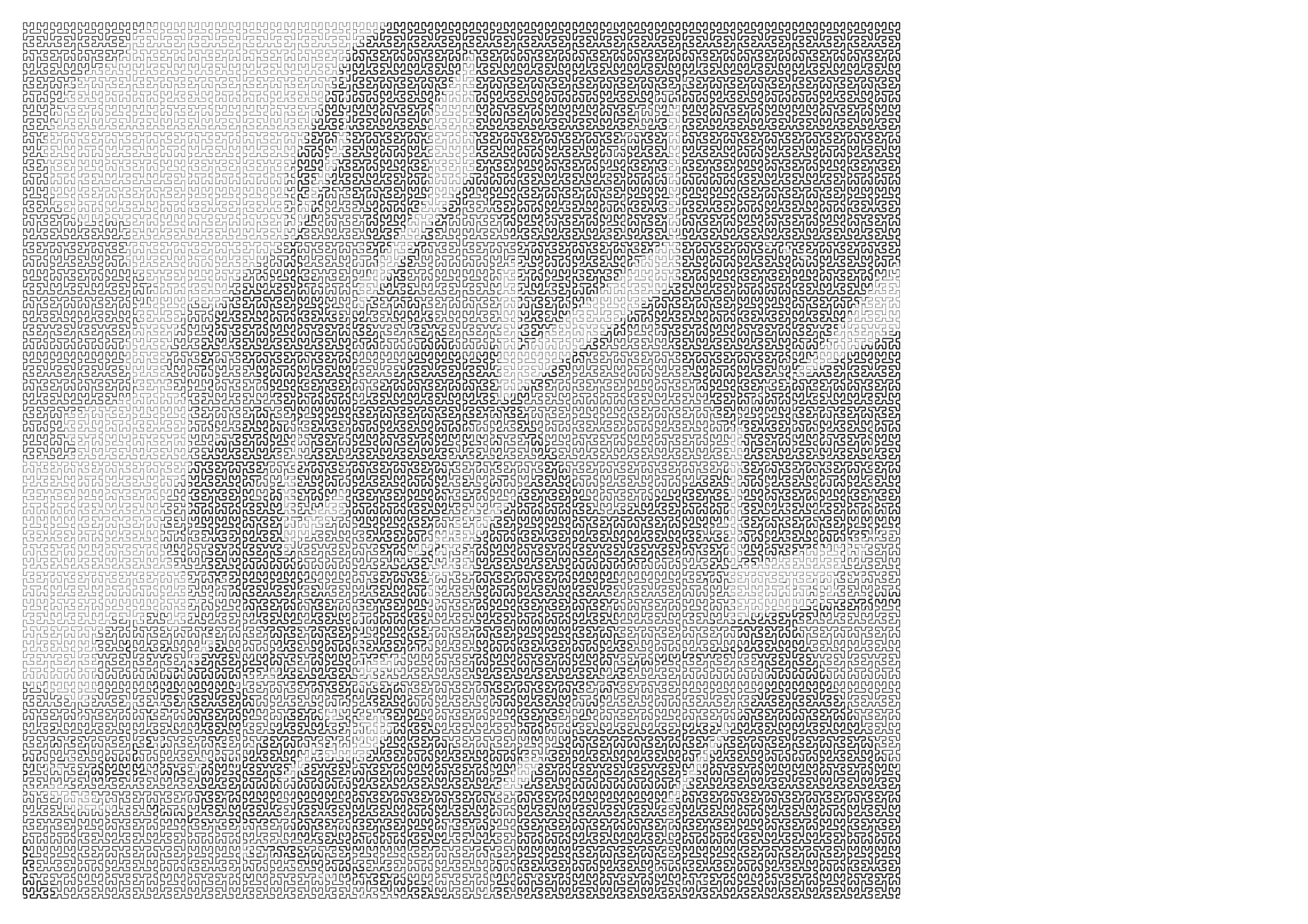
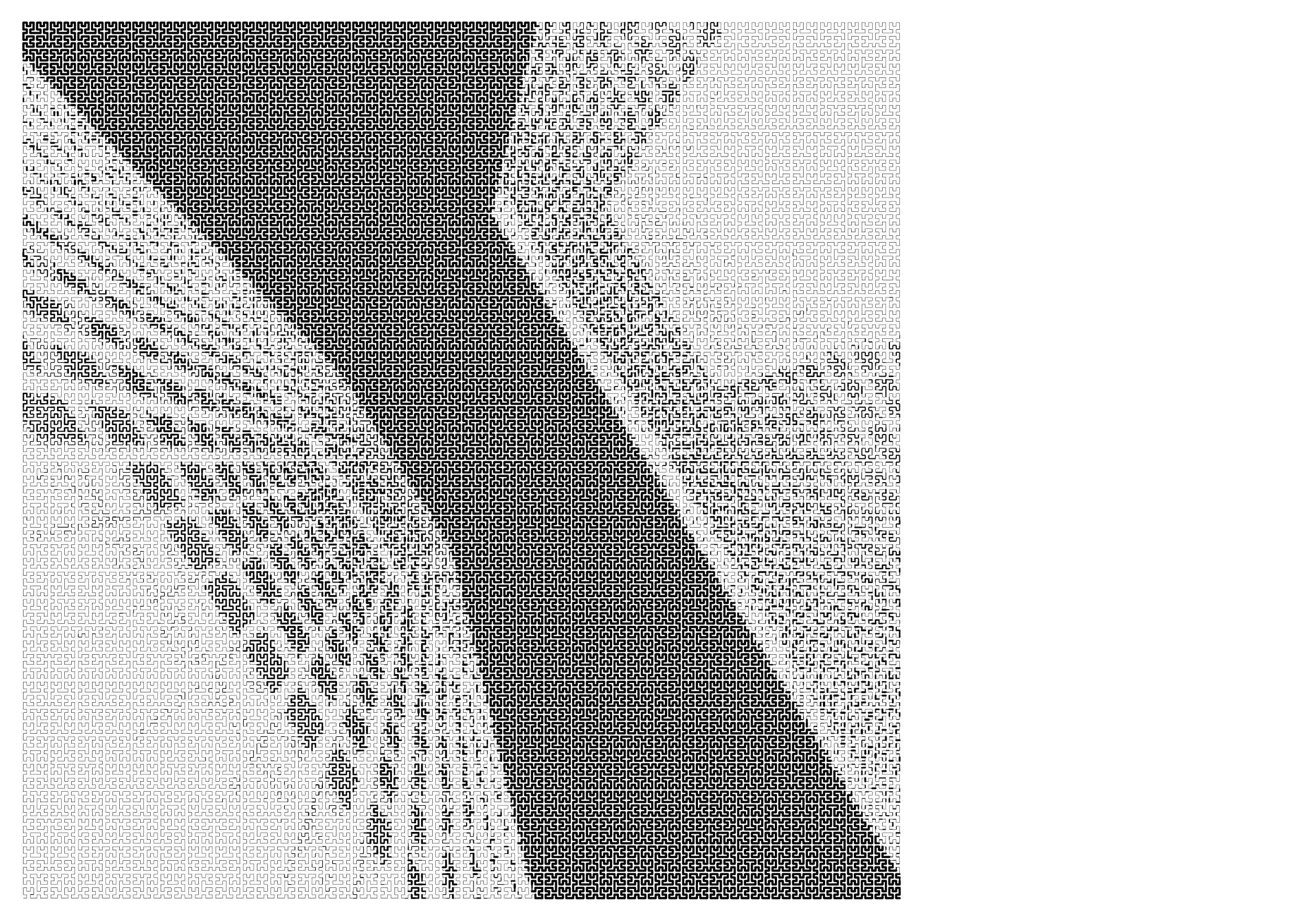
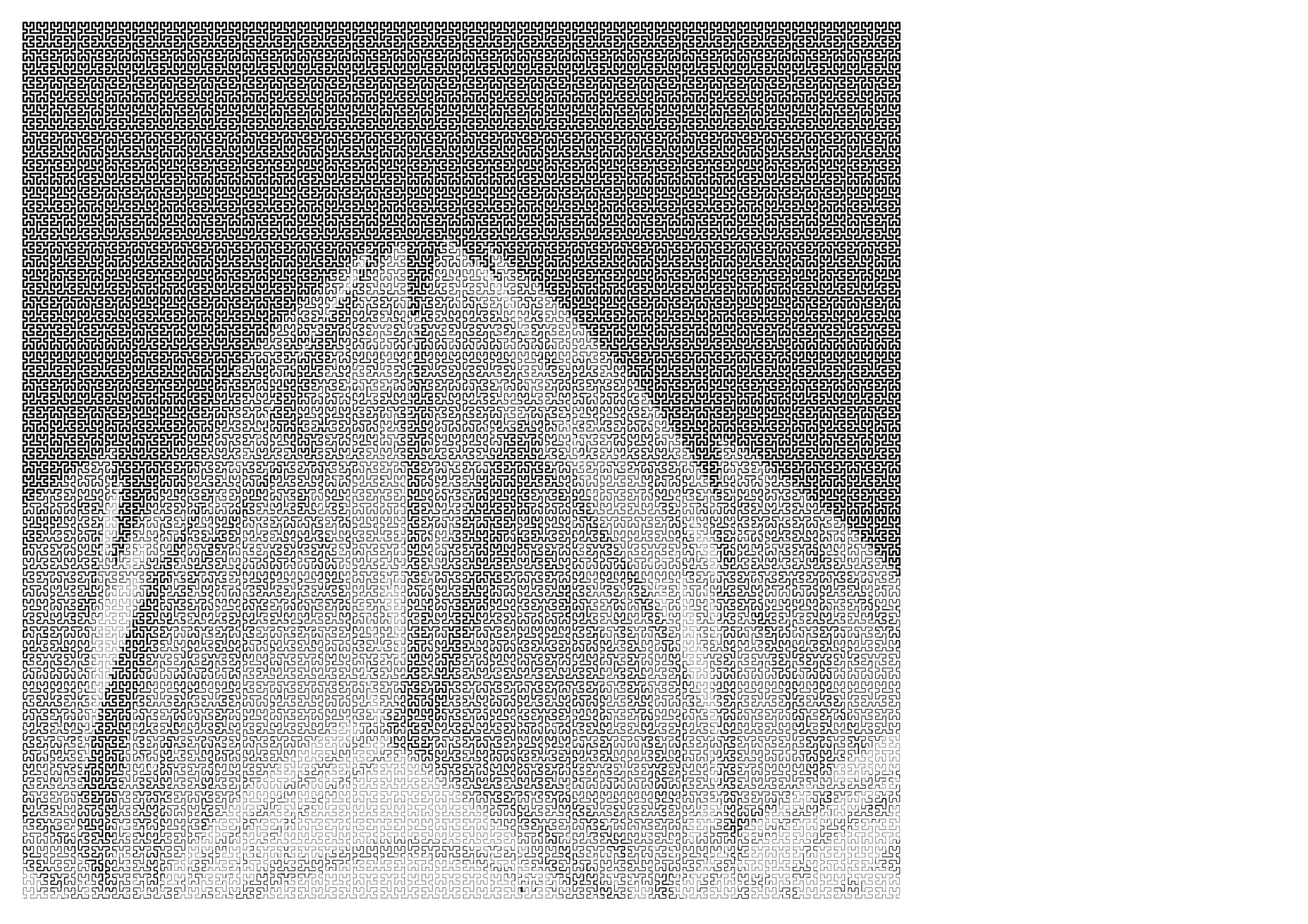
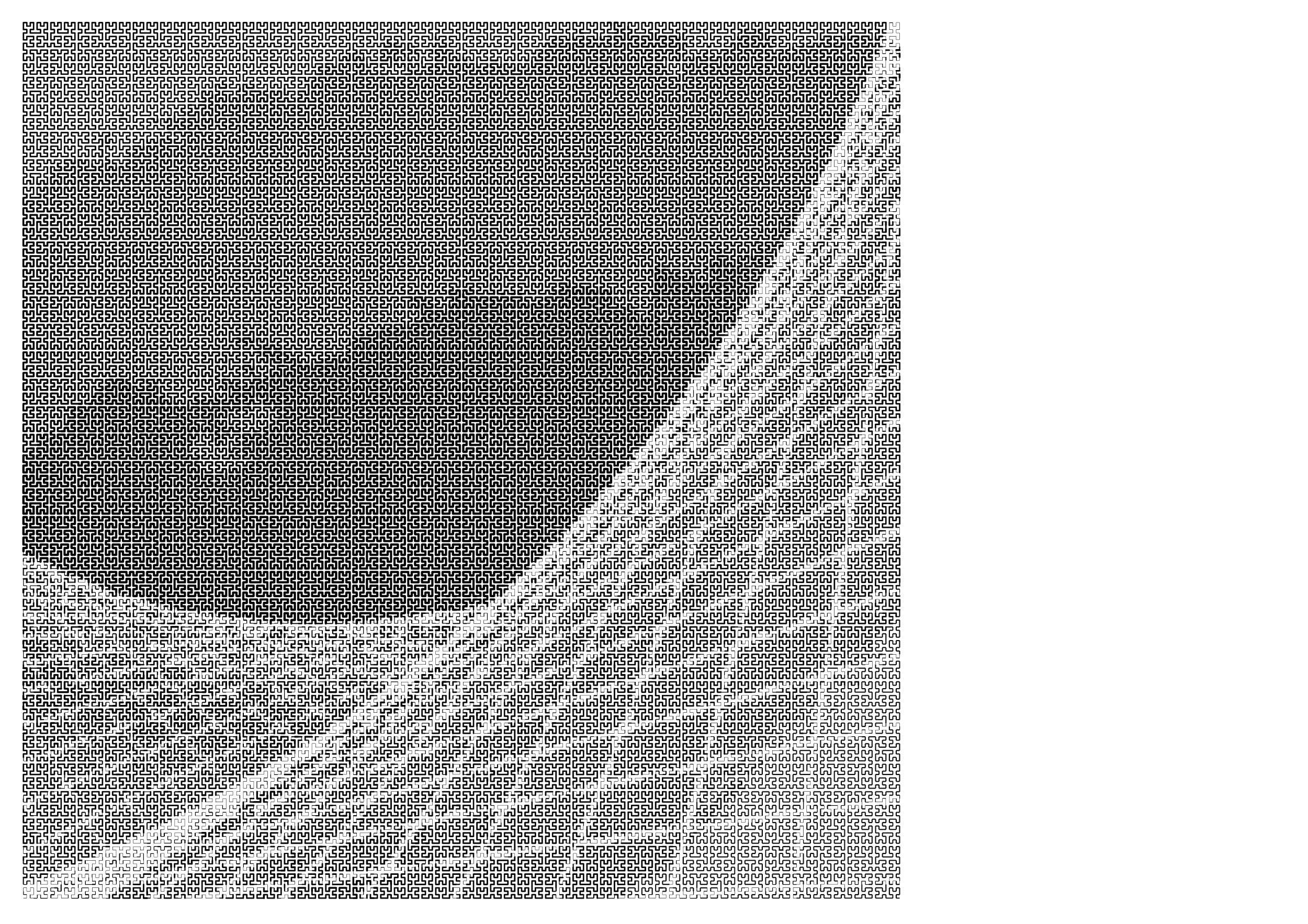
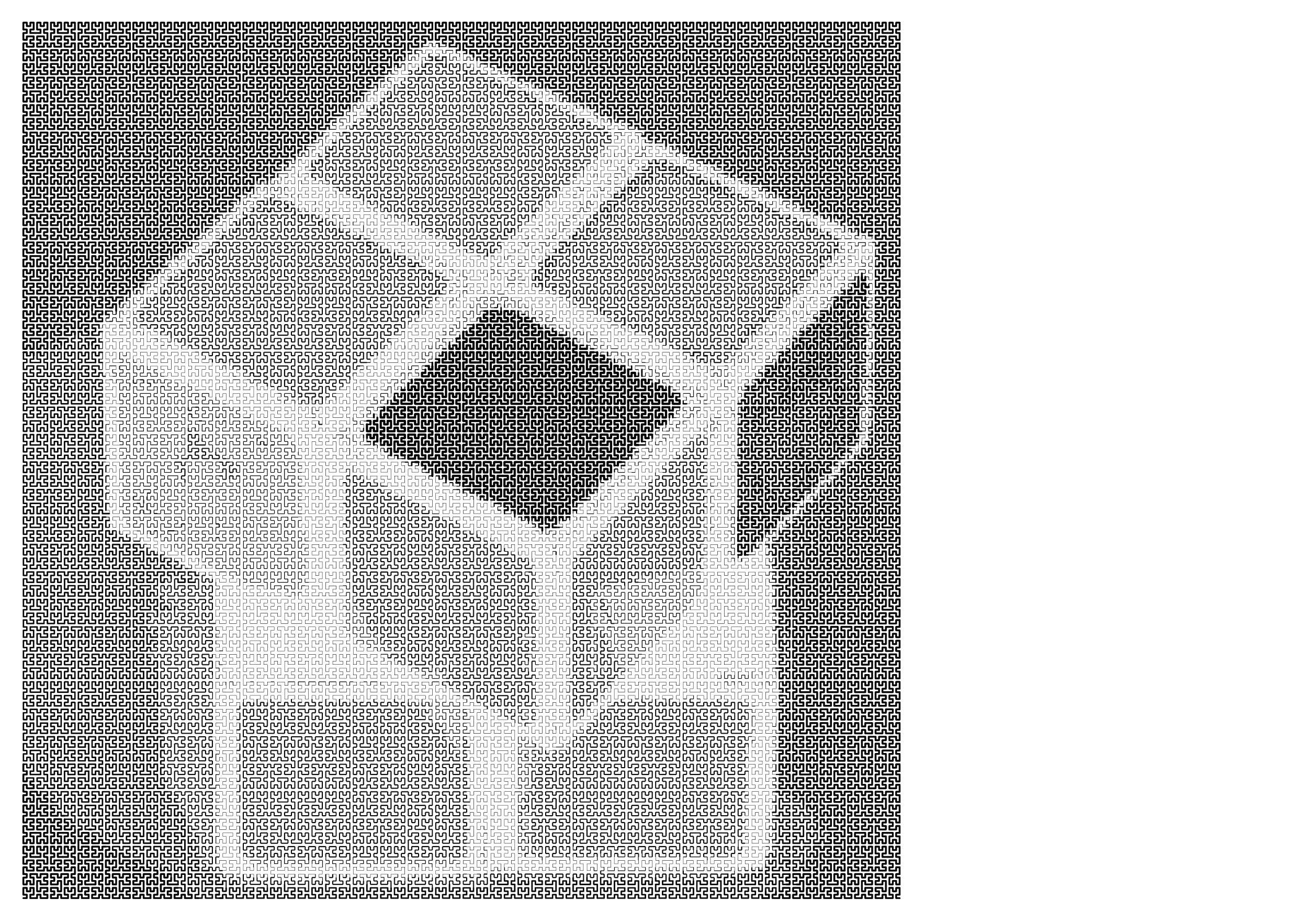
[ZOOM IN]
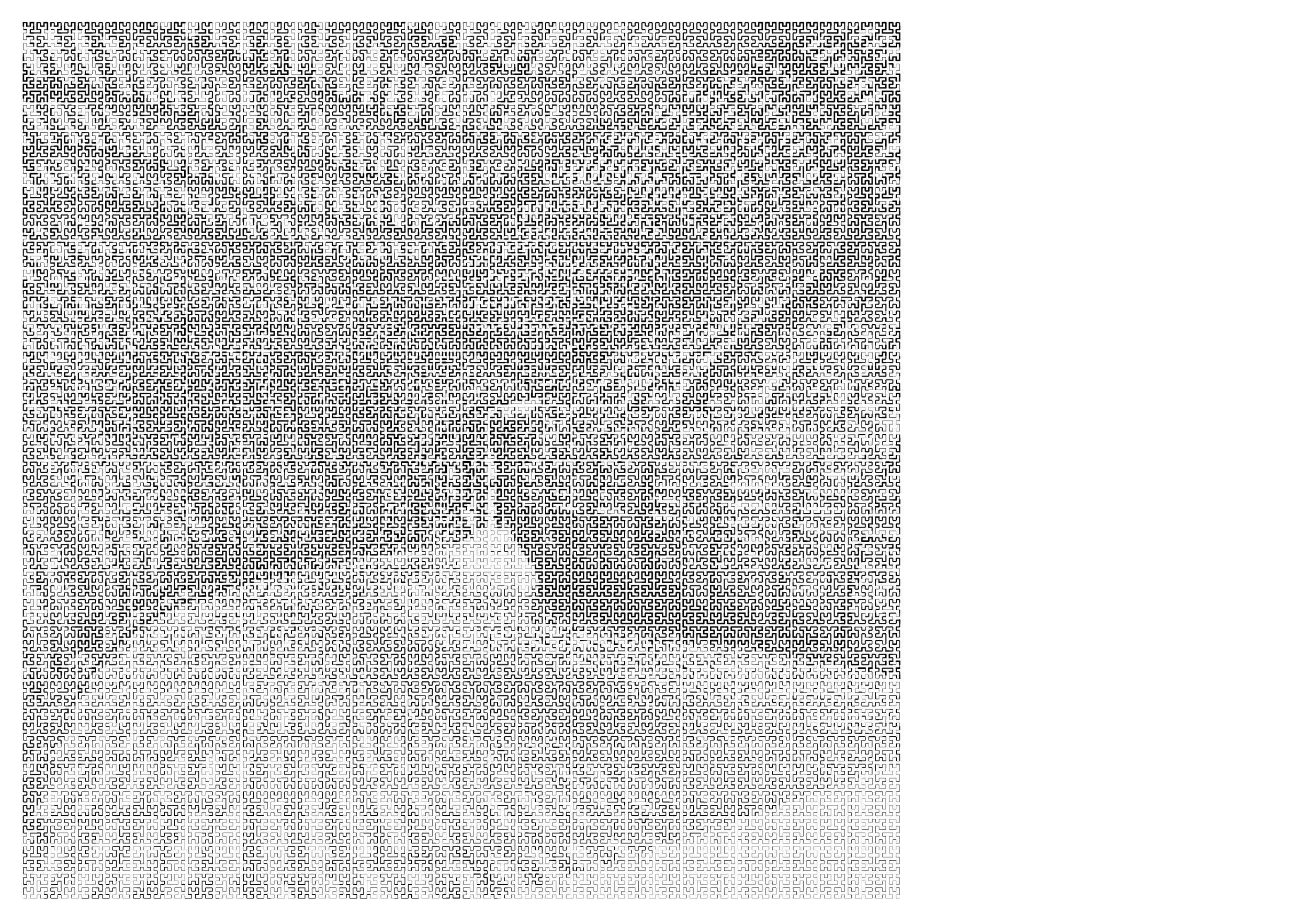
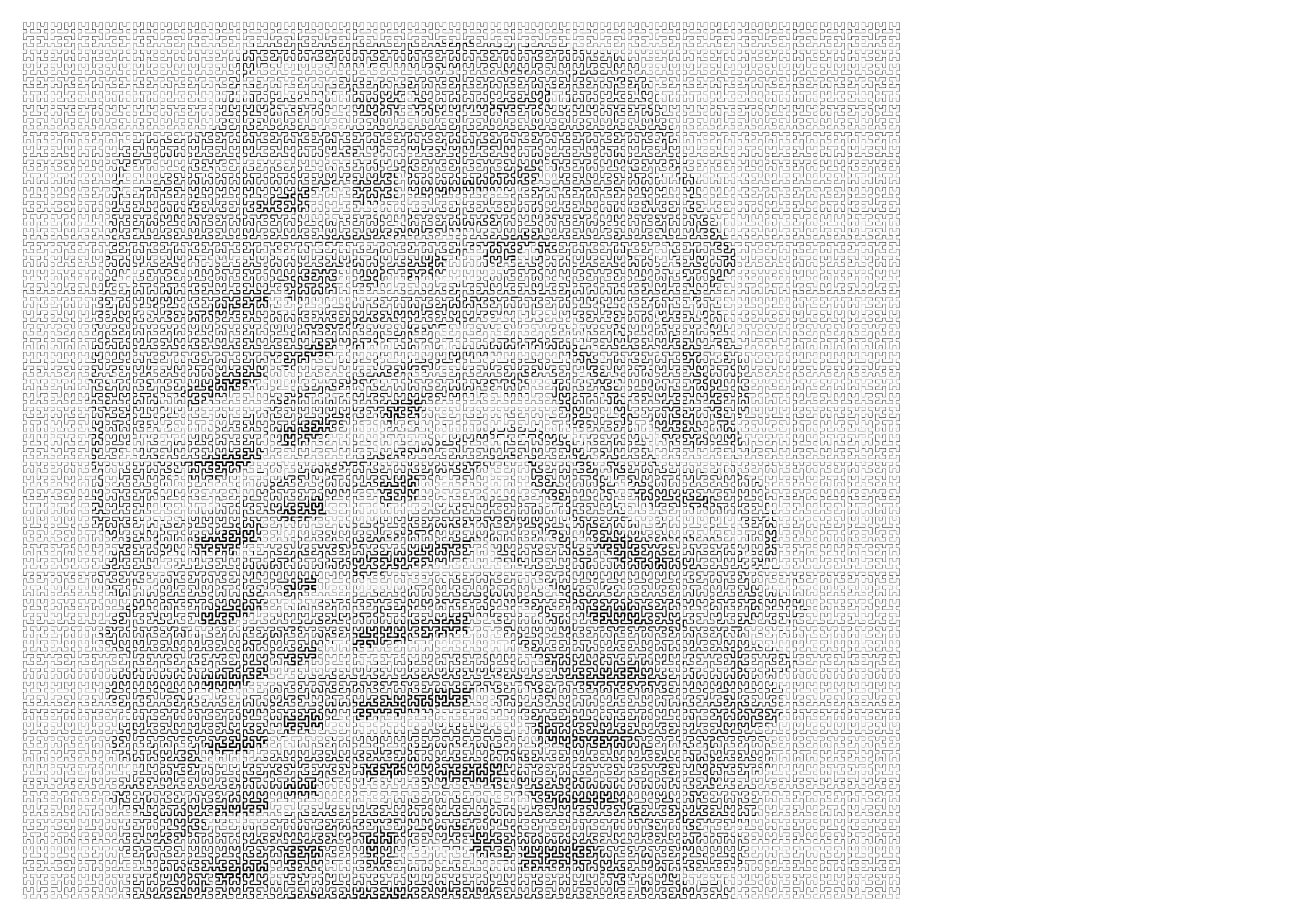
THE SCRIPT
C# Code for Hilbert Curve
Generated Points Converted to Polyline and Each Line Segment Extracted
Domain Creation for Maximum and Minimum Lineweight Values
n = number of recursion integer bigger than 0 and less than 10 d = normalized distance between 0 and 1
Hilbert Curve
Function that transform a normalized distance "d" (0 to 1) to a coordinate on xy plane with x also normalized https://en.wikipedia.org/wiki/Hilbert_curve
*/ int n_rec; if (n < 1) n = 1;
if (n > 10) n = 10;
//Power of 2 => (int) Math.Pow((double) 2, (double) n); n_rec = 1 << n;
if (d < 0.0) d = 0.0;
if (d > 1.0) d = 1.0;
double d_d = d * ((double) n_rec * (double) n_rec - 1.0); double d_min = Math.Floor((d * ((double) n_rec * (double) n_rec - 1.0)));
double d_max = Math.Ceiling((d * ((double) n_rec * (double) n_rec - 1.0)));
//int d_int = (int) d_double;
Image Sampler to Extract Image Pixel Data
Piping of Line Segments as an alternative for Lineweight
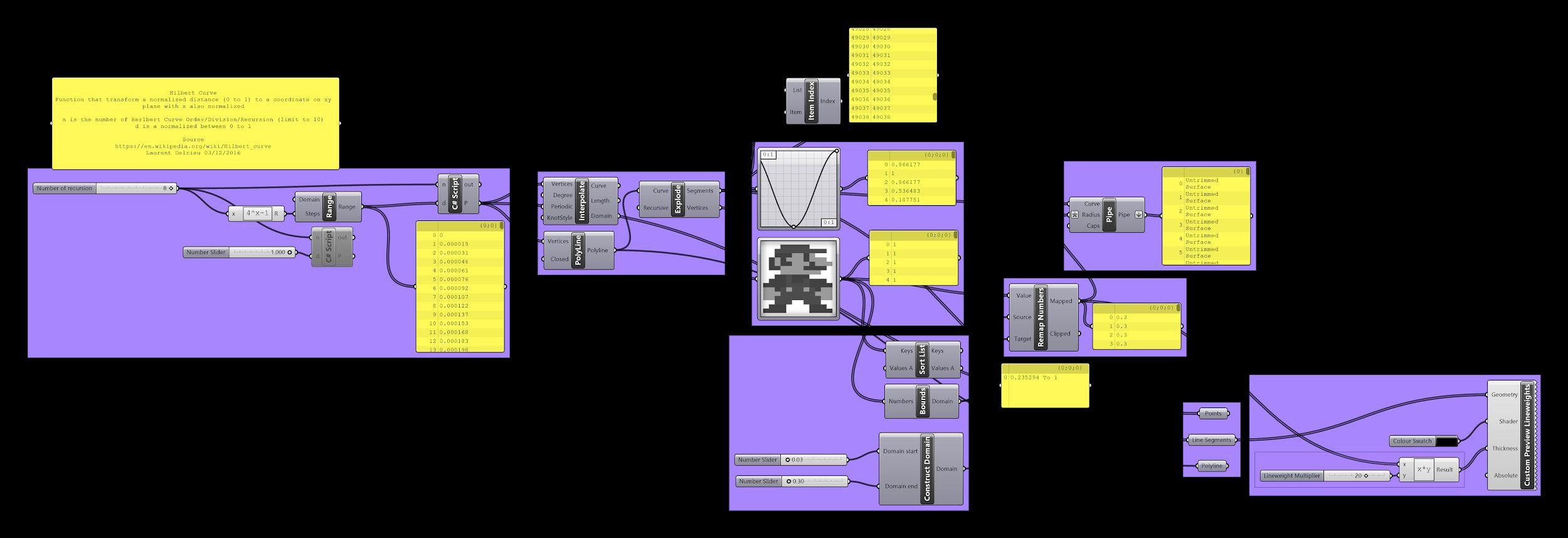
Pixel Data Used as Factor for Lineweight
Adding Lineweight to Line Segments
int x_min , y_min; int x_max , y_max; x_min = y_min = x_max = y_max = 0; d2xy(n_rec, (int) d_min, ref x_min, ref y_min); d2xy(n_rec, (int) d_max, ref x_max, ref y_max); double x, y; x = y = 0.0; if (d_min < d_max) {
x = (double) x_min + (double) (x_max - x_min) * (d_dd_min) / (d_max - d_min); y = (double) y_min + (double) (y_max - y_min) * (d_dd_min) / (d_max - d_min); } else { x = (double) x_min; y = (double) y_min; }
double shift; shift = 1 / Math.Pow((double) 2, (double) (n + 1)); P = new Point3d(x / (double) n_rec + shift, y / (double) n_rec + shift, 0.0); }
void d2xy(int n, int d, ref int x, ref int y) { int rx, ry, s, t = d; x = y = 0; for (s = 1; s < n; s *= 2) { rx = 1 & (t / 2); ry = 1 & (t ^ rx); rot(s, ref x, ref y, rx, ry); x += s * rx; y += s * ry; t /= 4; } }
//rotate/flip a quadrant appropriately void rot(int n, ref int x, ref int y, int rx, int ry) { if (ry == 0) { if (rx == 1) { x = n - 1 - x; y = n - 1 - y; }
//Swap x and y int t = x; x = y; y = t; } } }
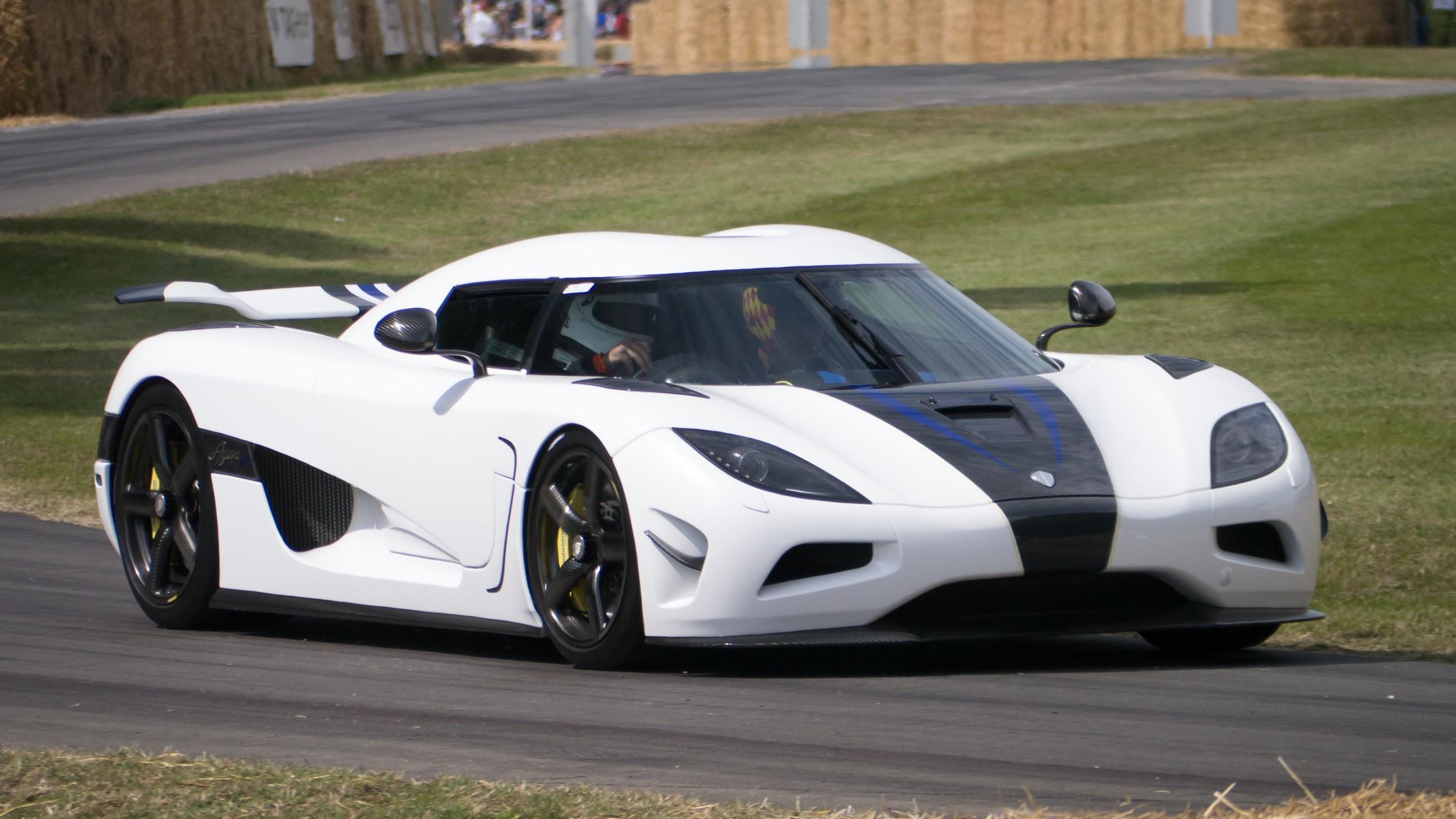
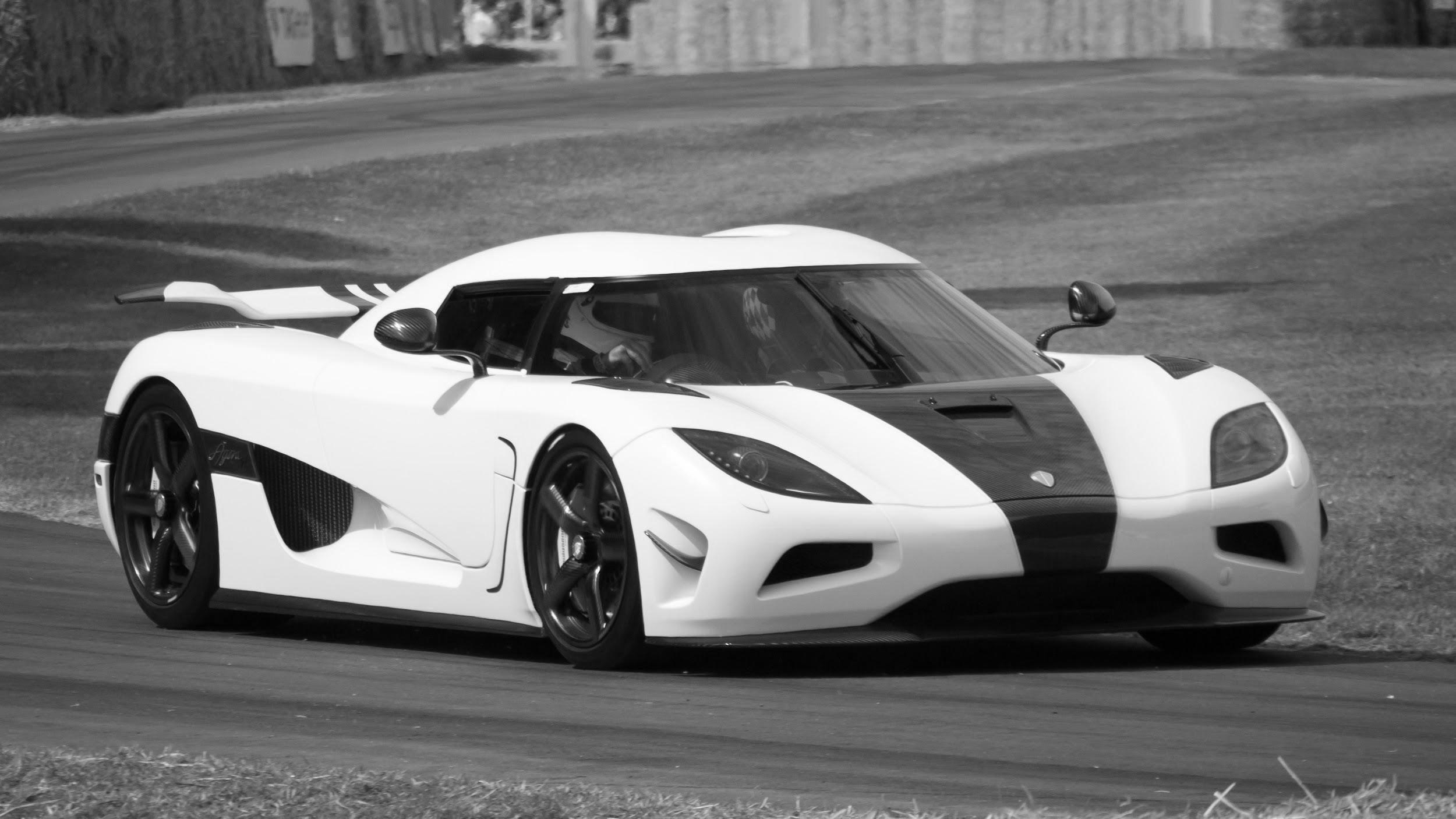
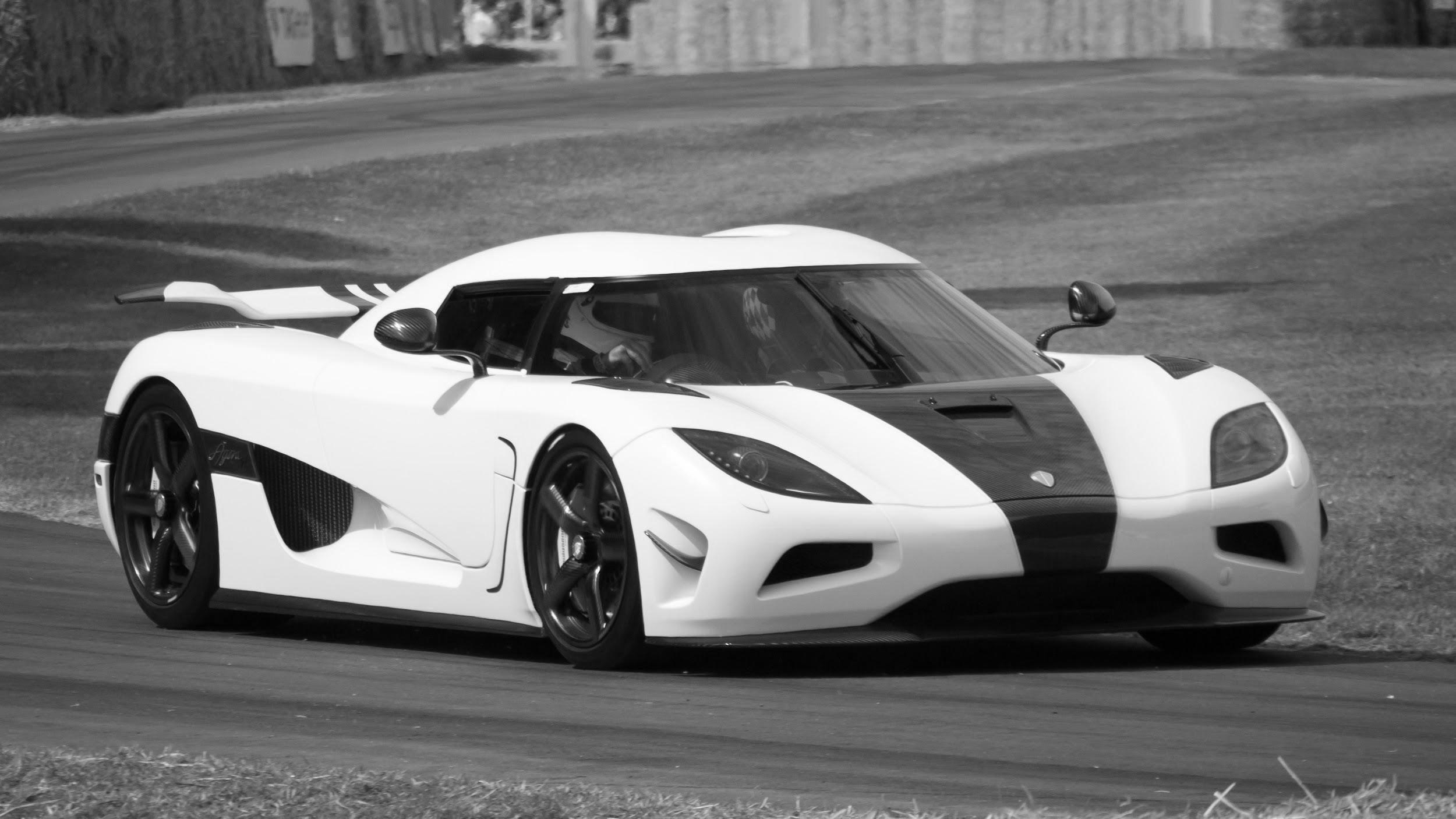
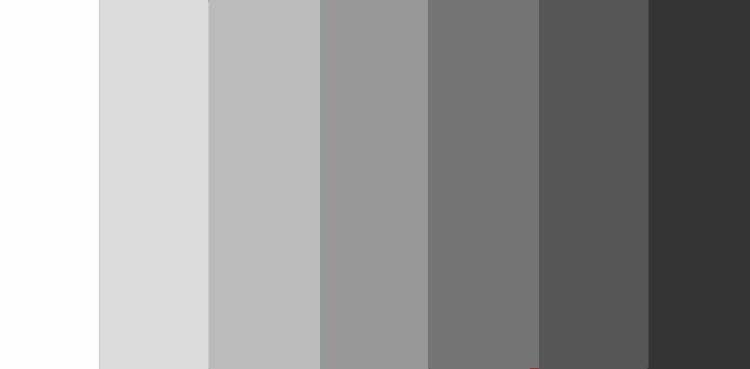
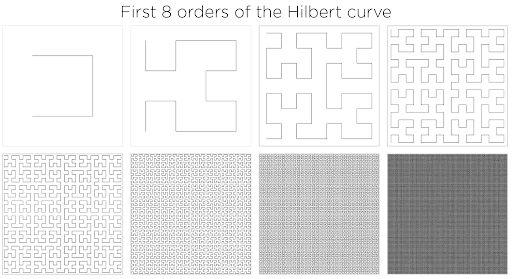
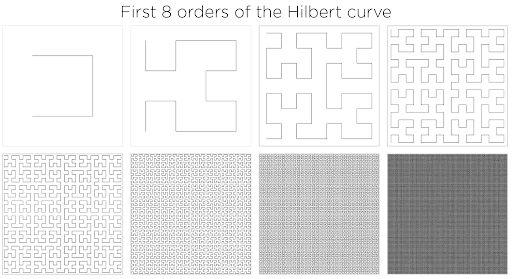
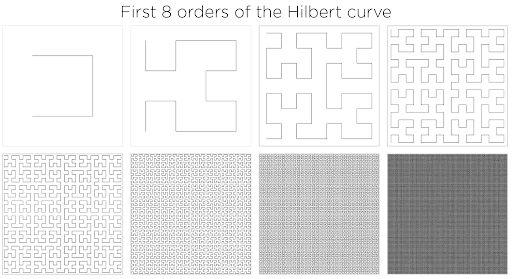
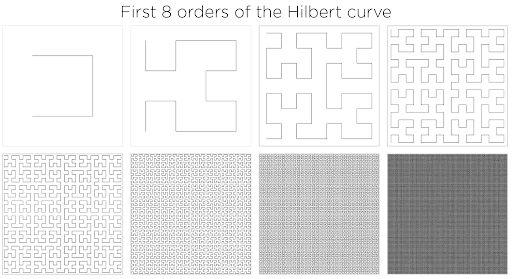
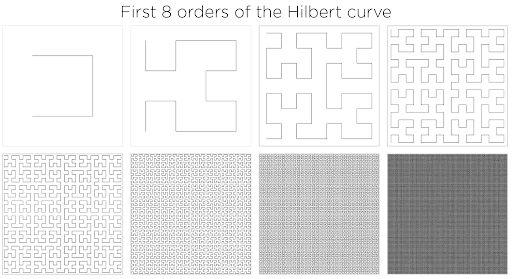
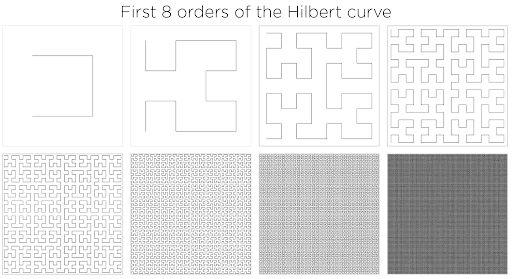
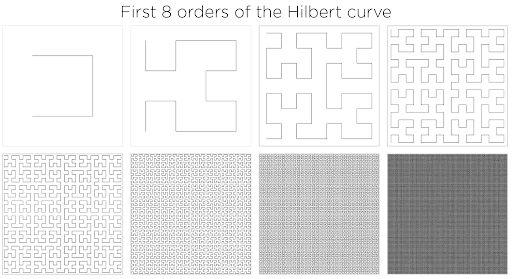
MANIFESTATION TO 3D
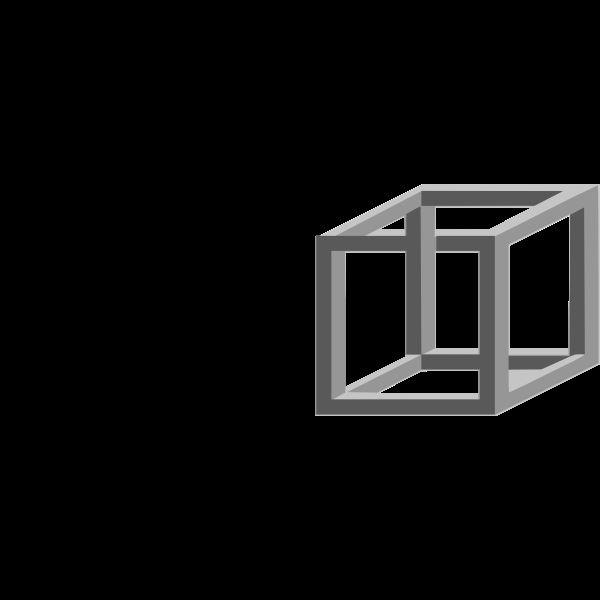
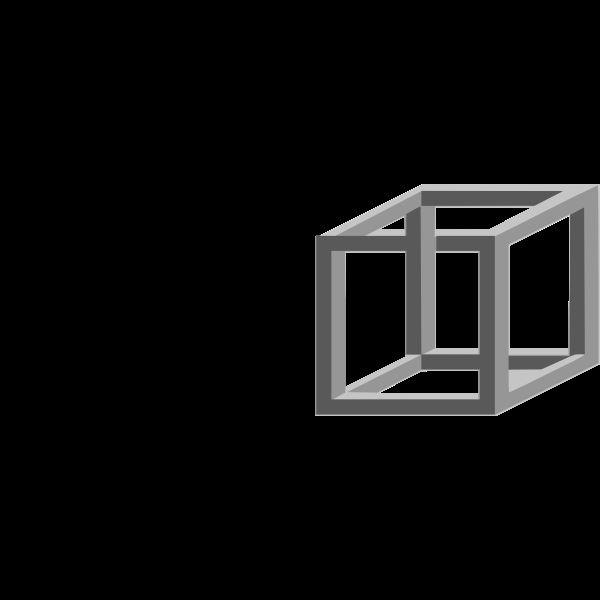
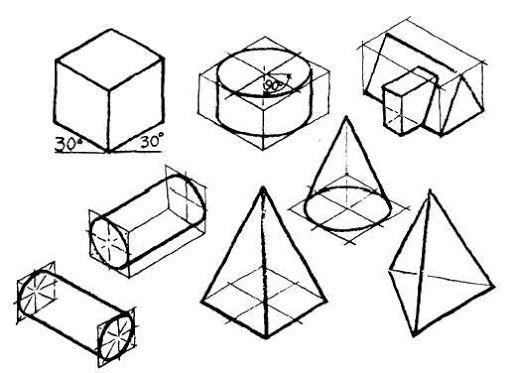
● For a 2-dimensional Curve: ○ Magnitude And Form Of Volume ○ Number Of Lines ○ Initiation Vector Of Line
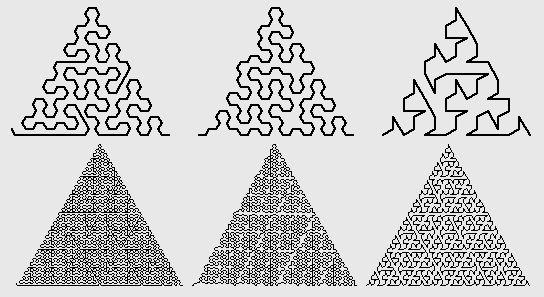
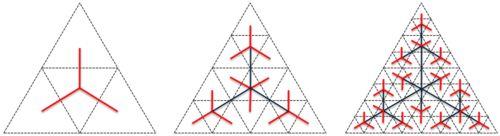