Introduction to PHP Framework Development
PHP frameworks have revolutionized web development by providing efficient tools and libraries for building robust and scalable applications In this article, we will explore the world of PHP framework development and delve into the process of creating modern web applications
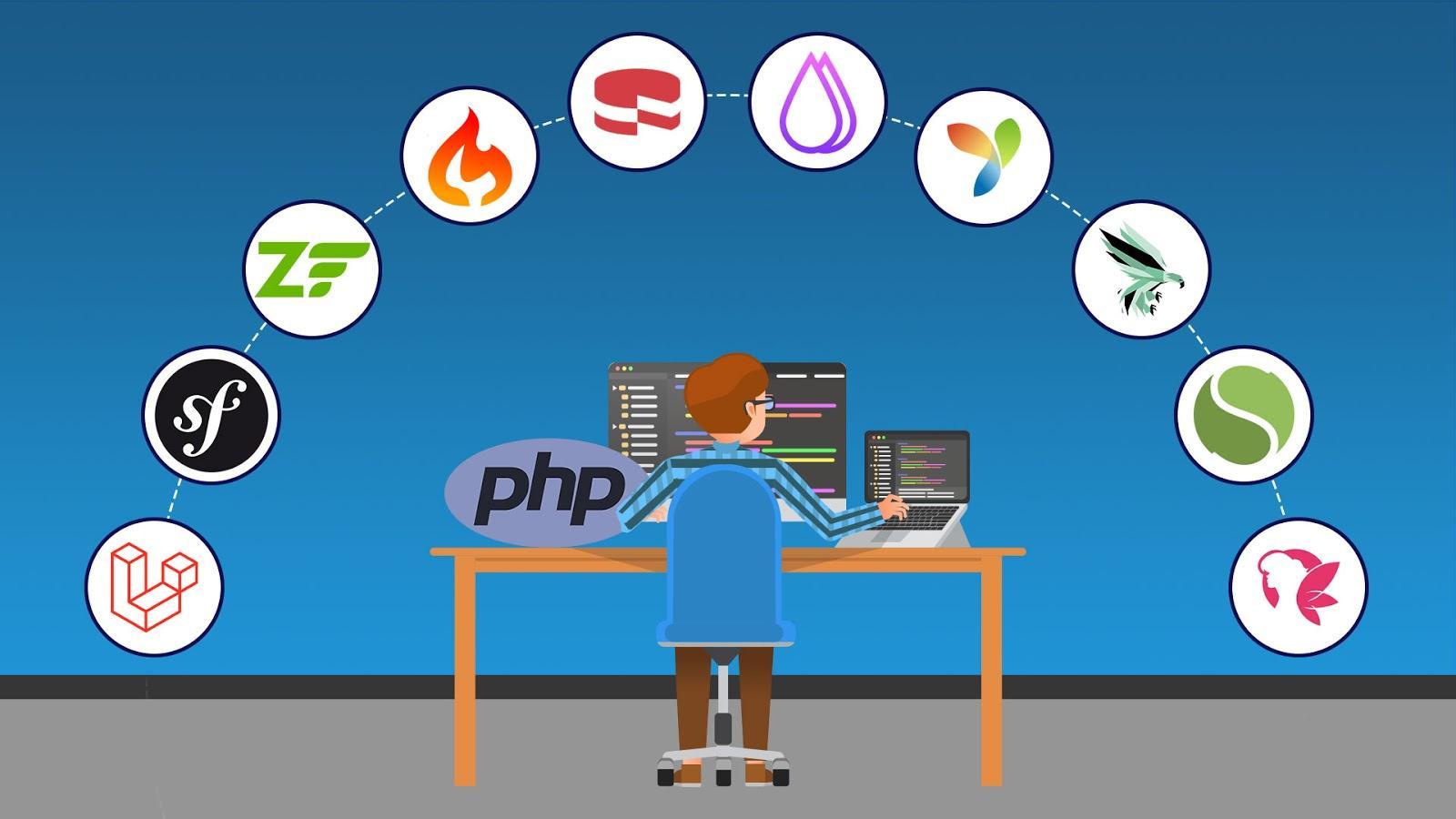
Choosing the Right PHP Framework
Before diving into PHP framework development, it's crucial to select the right framework for your project Consider factors such as project requirements, community support, documentation, and the learning curve. Let's take a closer look at some of the popular PHP frameworks and their key features
Getting Started with PHP Framework Development
To begin your PHP framework development journey, you need to set up the chosen framework. We will walk you through the installation process, including any necessary prerequisites Additionally, we'll explain
the basic directory structure and configuration files you'll encounter in a typical PHP framework project Understanding these fundamentals will help you navigate and organize your code effectively
Understanding MVC Architecture
Model-View-Controller (MVC) is a widely adopted architectural pattern in PHP framework development. MVC provides a structured approach to separate the application's data (Model), user interface (View), and application logic (Controller) By understanding the roles and interactions of these components, you can build scalable and maintainable applications
The Model represents the data layer of your application. It handles data retrieval, manipulation, and storage You'll learn how to define models and interact with databases to perform CRUD operations efficiently
The View component focuses on presenting data to the users. It encompasses the user interface and handles rendering HTML templates, displaying data, and handling user input You'll explore how to create dynamic views and leverage template engines for cleaner and modular code.
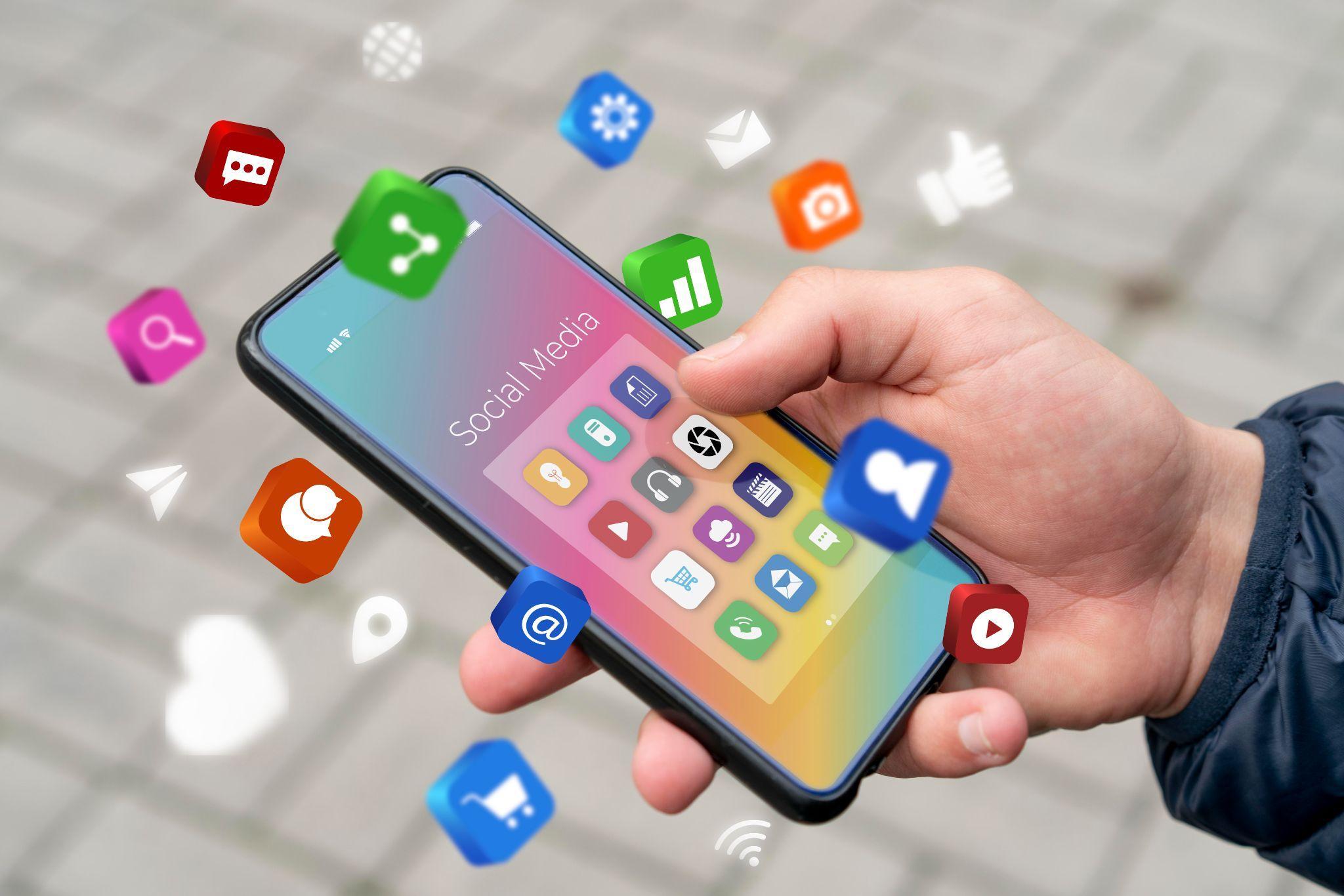
It receives user requests, processes them, and orchestrates the appropriate actions You'll discover how to define routes, handle user input, and invoke the corresponding actions in the controllers.
Building a CRUD Application with a PHP Framework
A common task in web development is creating CRUD (Create, Read, Update, Delete) applications. With a PHP framework, this process becomes streamlined and efficient. Here are the key steps to building a CRUD application:
Define the database structure: Design and create the necessary database tables to store your application's data.
Set up routing: Configure routes in your framework to map URLs to specific controllers and actions
Create models: Define models that represent your data entities and define relationships between them
Implement controllers: Write controller methods to handle user requests and interact with models to perform CRUD operations
Design views: Create user interface templates using HTML and the framework's templating system Use dynamic data from the models to populate the views
Handle forms: Implement form handling in your application to capture user input and validate it before performing operations on the database.
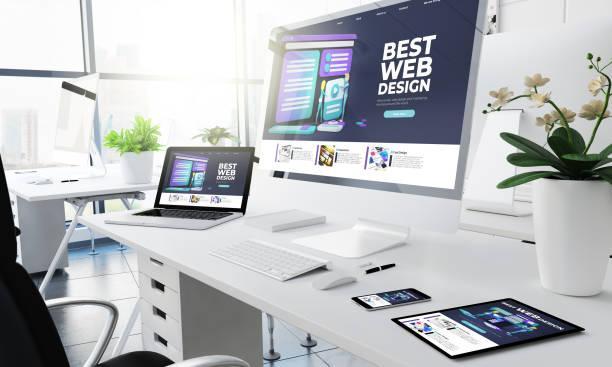
Perform CRUD operations: Use the framework's APIs and conventions to insert, retrieve, update, and delete data from the database.
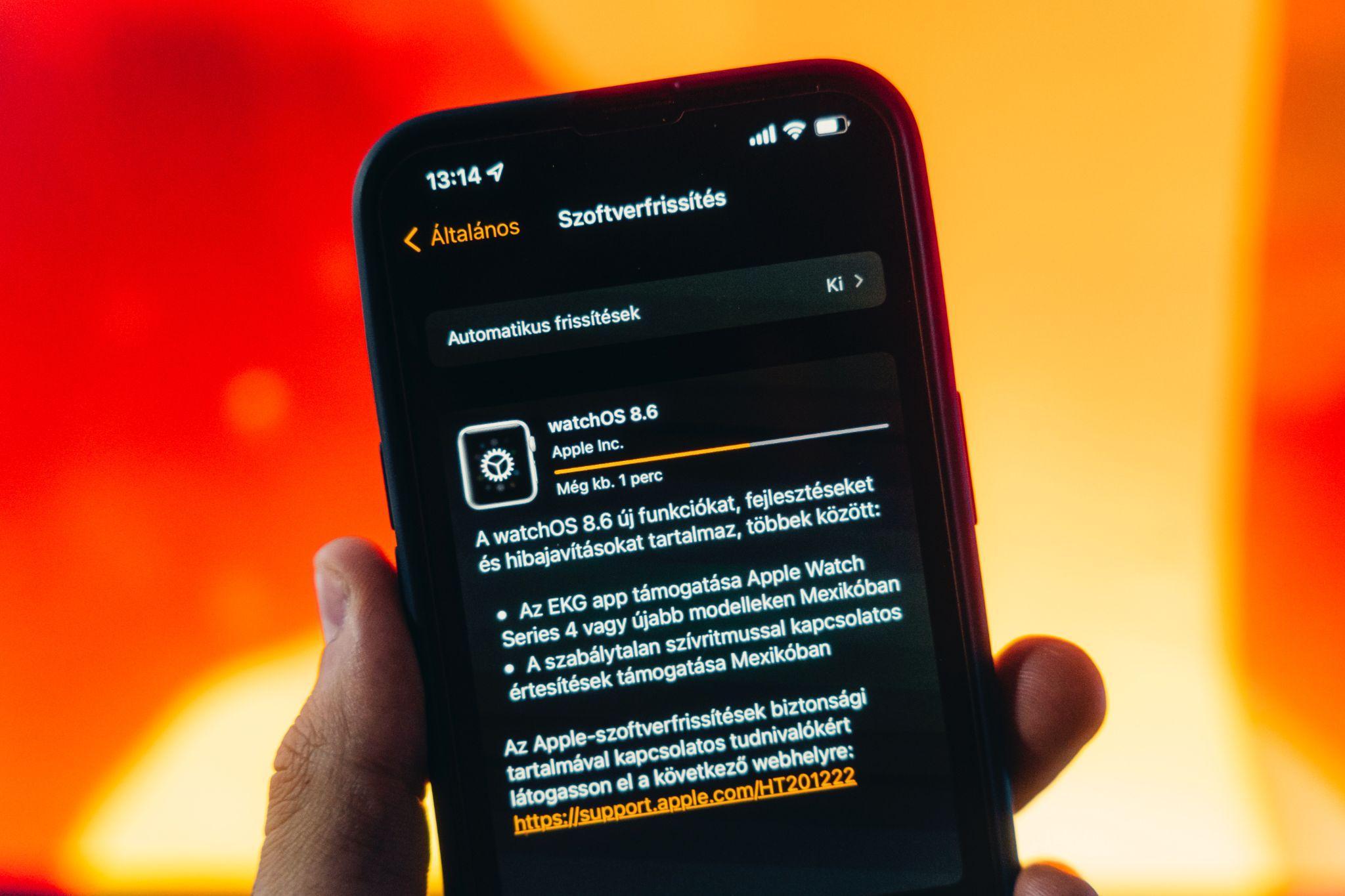
Optimizing Performance in PHP Frameworks
To ensure your PHP framework-based applications deliver optimal performance, consider the following:
Caching: Implement caching mechanisms to store frequently accessed data, reducing database queries and improving response times
Code optimization: Review and optimize your code for performance bottlenecks, such as eliminating redundant database queries and optimizing loops and conditionals
Database query optimization: Optimize your database queries by using indexes, optimizing joins, and avoiding unnecessary queries.
Benchmarking and profiling: Use benchmarking tools to measure your application's performance and identify areas for improvement Profile your code to pinpoint bottlenecks and optimize critical sections.
Security Considerations in PHP Framework Development
Ensuring the security of your PHP framework-based applications is of utmost importance. Here are some essential security considerations:
Input validation: Validate and sanitize all user inputs to prevent common security vulnerabilities like SQL injection and cross-site scripting (XSS) attacks.
Output encoding: Properly encode and escape output to prevent HTML injection and other forms of attacks when displaying user-generated content.
Authentication and authorization: Implement secure authentication and authorization mechanisms to protect sensitive data and restrict unauthorized access
Protection against CSRF attacks: Apply CSRF tokens and techniques to prevent Cross-Site Request Forgery attacks
By following these security best practices, you can mitigate potential security risks and ensure the integrity and confidentiality of your PHP framework-based applications
In conclusion, PHP frameworks provide a powerful foundation for efficient web development. By choosing the right framework, understanding MVC architecture, building CRUD applications, optimizing performance,
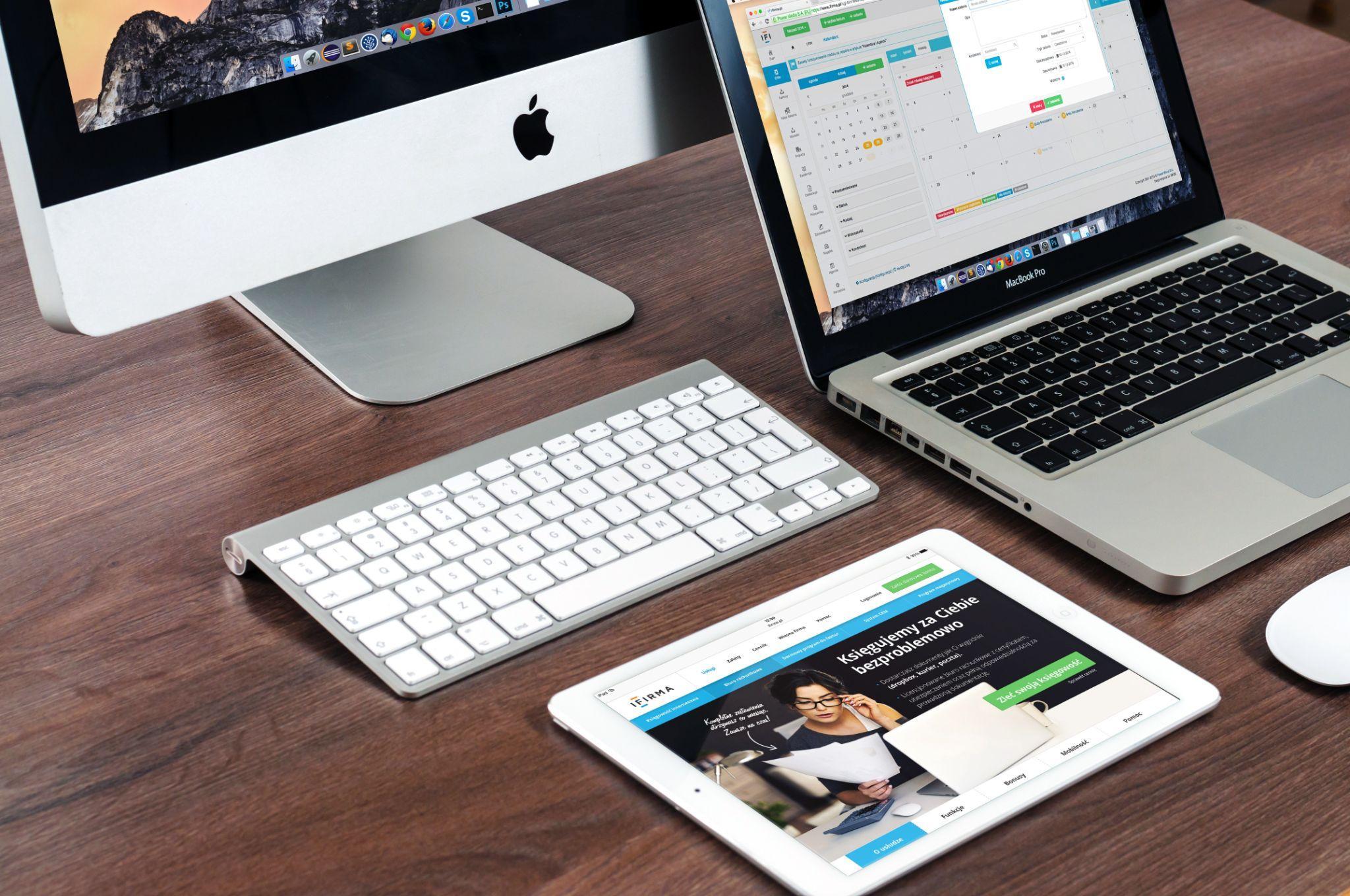