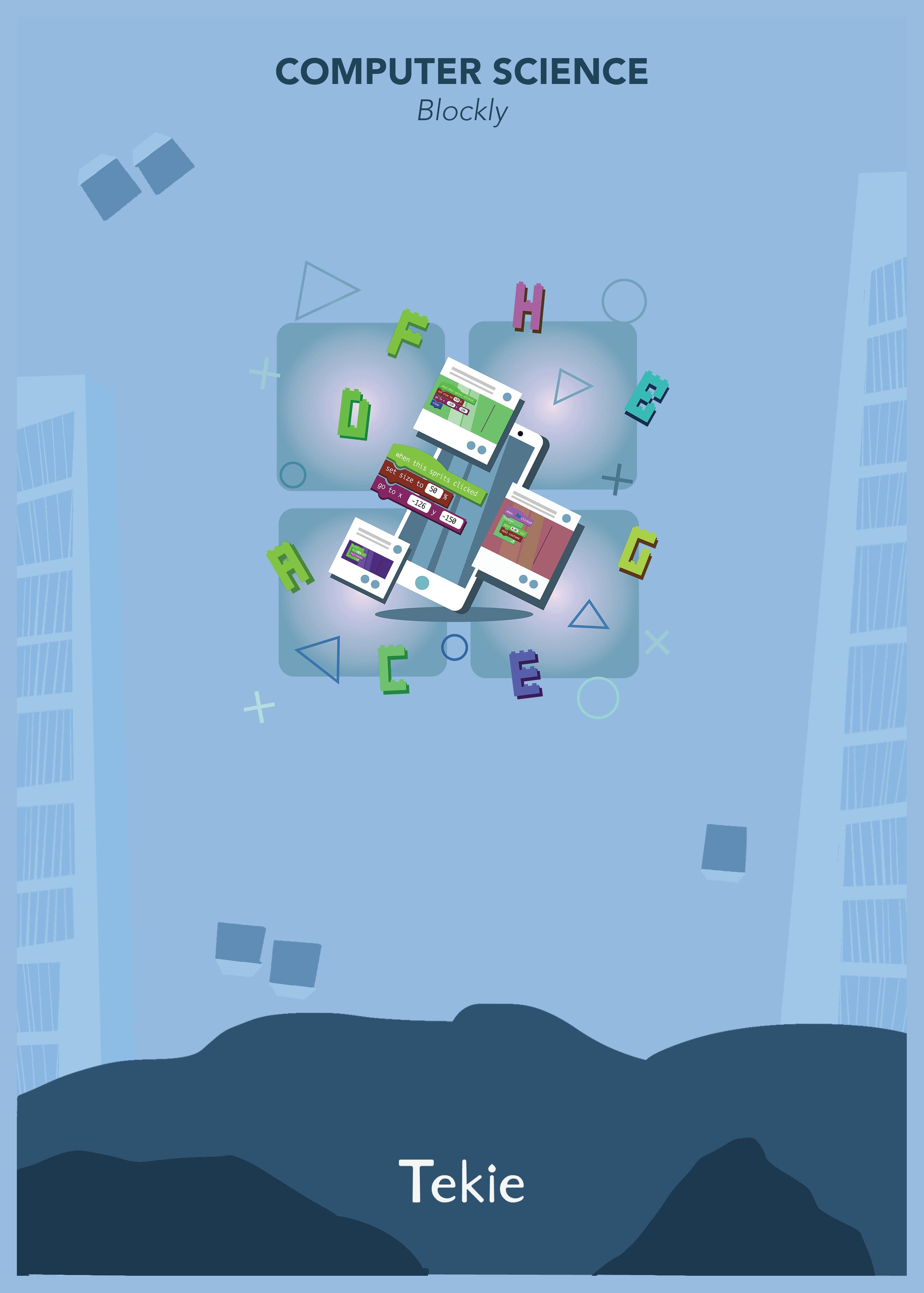
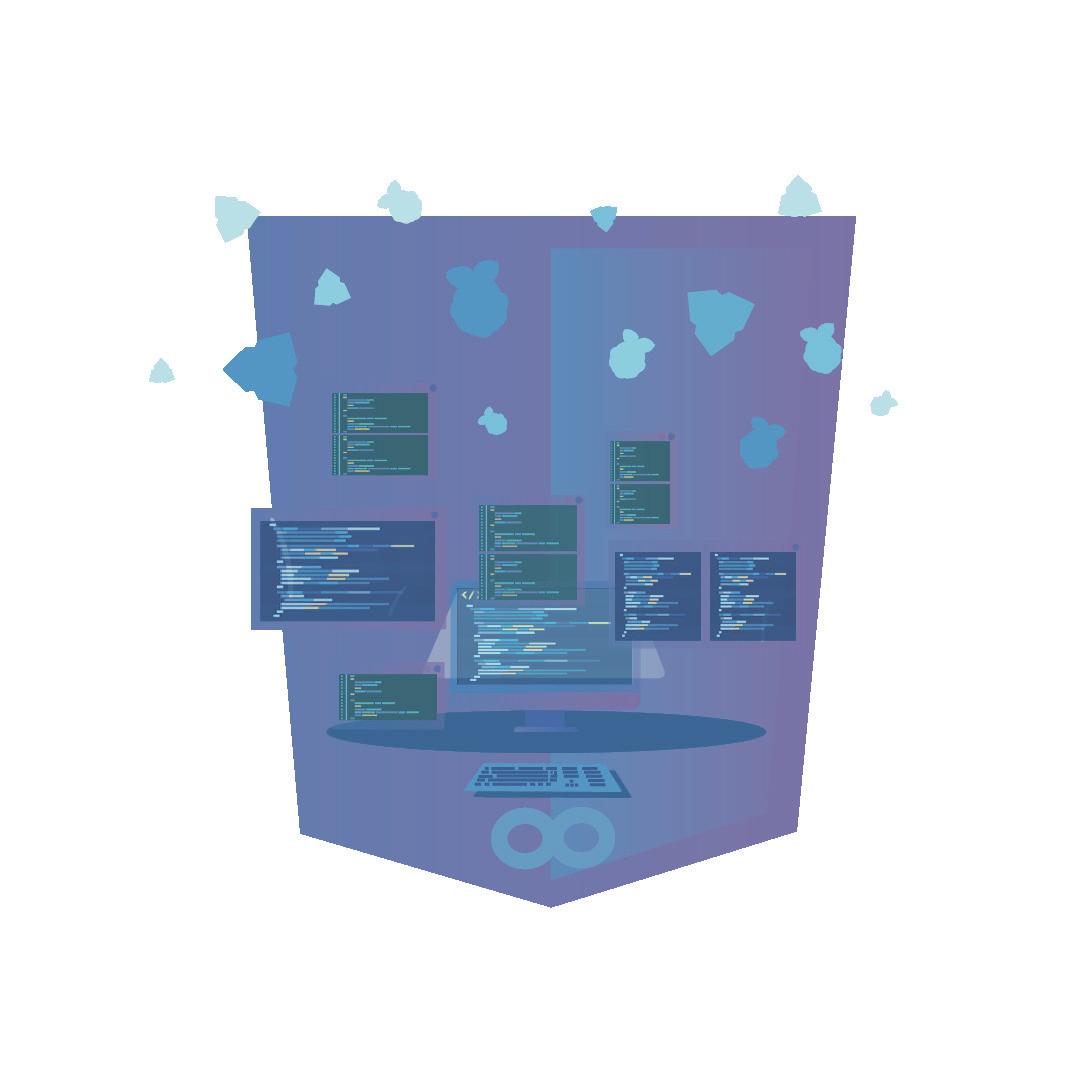
1 Fundamentals of Java 1
What is Programming? 1 What is Java? 2 Why Learn Java? 2
2 Decision Making 25 User Inputs 25 Conditions and Flowcharts 27
3 Loops with Java 39
Introduction to Loops 39 Jump Statements 44 Appendix A 47
Computers are incredible and powerful machines. Today, they are everywhere, be it a shop, a bank, a school, government offices, or malls. But they don’t do anything without our instructions. They are helpful, but deep down, they are not smart. The instructions we give the computer are sets of commands to perform a task, which is nothing but a programme.
Programming is the process of creating a set of instructions that tells a computer how to perform a task. A task is a piece of work to be done. In a program, it may represent a section of the program.
We need a language to instruct computers to carry out a task, just as we use English to communicate with one another. Since computers are machines, they can only understand machine language. As machines are electrically powered devices, they listen to two signals: on and off, which are represented by 0s and 1s, respectively.
The language that is formed with 0s and 1s is called binary language, as it builds up on two digits. Binary Language is also known as Low-Level Language.
A high-level language (HLL) is a humanfriendly programming language like Java, Python, C, etc. It is intended to make computer programming simpler for programmers, as it is not feasible for programmers to remember the 0 and 1 codes for everything. Later, these high-level language (HLL) programs are translated into low-level language (LLL) programs to make computers understand them.
Java is a widely used programming language and software platform that runs on billions of devices, including notebook computers, mobile devices, gaming consoles, medical devices, and many others. It is used to develop mobile apps, web apps, desktop apps, games, and much more.
Java was developed by Sun Microsystems (which is now a part of Oracle) in 1995. James Gosling is known as the father of Java. Before Java, its name was Oak. Since Oak was already a registered company, James Gosling and his team changed the name from Oak to Java.
Any hardware or software environment in which a program runs is known as a platform. Since Java has a runtime environment (JRE) and API, it is called a platform.
Below are the reasons why we should learn Java:
1. Java is based on the “Write Once, Run Anywhere” principle, which makes it a platform-independent language.
2. Java is beginner-friendly and gives you a competitive edge as it’s one of the leading programming languages in the industry.
3. Java has strong memory management, which makes it robust.
4. Java takes less execution time as it is an interpreted language.
5. Java is secure and dynamic.
6. Java is used on a large scale around the world, covering different sectors.
7. Java offers a variety of job roles, expanding career options such as Software Developer, Web Developer, Application Developer, etc.
Java is a high level language, so any programme written in it must first undergo a two-step conversion to a machine level language.
Any Java programme is initially translated into bytecode, a type of machineindependent code. This bytecode is then executed on the machine-specific JVM (Java Virtual Machine).
A compiler is a computer program that converts high-level program instructions into machine language. It reads the source code, decodes it instruction by instruction into machine language, and then provides a machine-independent Java file that can run on any machine, like Windows, MacOS, Android, etc., with the help of the JVM.
Java compilers include the Java Programming Language Compiler (javac), the GNU Compiler for Java (GCJ), the Eclipse Compiler for Java (ECJ), and Jikes.
The JVM acts as a run-time engine to run Java applications. The JVM is the one that actually runs the Java code. It provides a runtime environment in which Java bytecode (a compiled Java program) can be executed. JVMs are available for platforms like Windows, MacOS, Android, etc.
So basically, the Java compiler first compiles the program into machine independent byte code, which is then executed by the JVM, a machine dependent platform, to produce the final output.
Generally, in programming, we follow sequential procedures to perform a task. But there are situations where we cannot solve a problem using a sequential method. A sequential method doesn’t allow us to go back to some set of statements to repeat or perform some different operation on different or similar types of data. When we need to solve a problem with real-life scenarios, we need a procedure that is more relatable and reusable.
OOP is one such programming paradigm. OOP stands for Object-Oriented Programming which deals with objects and classes to solve problems. Consider the scenario shown in the picture below. With OOP, we can make a class called Dog, which includes ATTRIBUTES of a dog such as HEIGHT, WEIGHT and FOOD. We can have related methods for a dog as well, such as RUN, PLAY and EAT. We can use the DOG class to add details to any number of dogs by creating their objects once we’ve created it.
1. OOP is faster and easier.
2. OOP gives the programmes a clear structure.
3. OOP helps to keep the code easier to maintain, modify, and debug.
4. OOP makes it possible to create fully reusable applications with less code and a shorter development time.
Java is an object oriented programming language. With an object-oriented programming technique, we design a program using objects and classes.
Objects are nothing but any real-world entity or item. They can be non-living objects like cars, phones and computers, or living entities like dogs, cats, plants, etc.
All objects have some features, like a car has wheels, engine, gears, accelerator, brake, doors, windows, etc. We call these features states. Similarly, a car has various functionalities like it should accelerate when the accelerator is pressed, it should stop when the brake is pressed, switching on the engine should turn on the car. We call these functionalities behaviours.
Similarly, a java object also has states and behaviours. States are stored in variables and behaviours are implemented using methods.
A class represents a group of objects of the same kind. For example, around us we see different kinds of vehicles, like bikes, scooters, cars, trucks, etc. But all these vehicles have some common features like wheels, engine, power, and they are all used for transportation. So, in terms of objects and classes, bikes, scooters, cars, and trucks are objects, but all of them are members of the class Vehicle.
Similarly, if we consider the class Animal, different animals like dogs, cats, tigers, etc., will be the members of this class.
In Java, the class is a blueprint from which we can create objects. We store all the common features and functionalities of the objects inside the class using variables and methods. Once a class is created, we can create any number of objects from the class that share the common features and functionalities. That is why an object belonging to any class is called an instance of that class.
In the above example, we have a class called Car and from that class different car objects, like Car 1, Car 2, and Car 3 are created.
In Java we write programs using classes and objects. A typical structure of a Java program includes the following:
//Program to print “Hello World!”. Import java.util.Scanner; class Welcome{
public static void main (String args[])
{ System.out.printIn(“Hello, Java!”);
{ }
Class Import Statements
Class Definition
Main Method
We start a Java Program with a Documentation statement which tells what the program is about. We use comments to add documentation about a program.
In Java, we have three types of comments:
● Single-line Comment
● Multi-line Comment
● Documentation Comment
Single-line comments are added using a pair of forwarding slashes (//). A multi-line comment starts with /* and ends with */. A documentation comment starts with /** and ends with */.
Next, we can add any package or import an existing class to the program. We can also create our own class in a Java program using the keyword class.
In the example above, we have created the class named Welcome. We call this statement a class definition. The opening curly braces at the end of the line indicates the start of the class.
Inside the class, we have the main method which is the execution point of the program when we run it. A Java program must have a main method else it will not execute. The name of the program should be the same as the name of the class which has the main method.
Here, the class Welcome has the main method, so the name of the program should be Welcome.java.
A main method can be public, static, and void.
public : It indicates that the main method can be called from anywhere outside or inside the class. Calling a method is nothing but asking Java to execute the program written inside a method.
static : It indicates that the main method will remain the same in all the objects that are created from the class. For example, let us say we create the objects Welcome1, Welcome2, and Welcome3 from the class Welcome, the main method will remain the same in all the three objects, that is, it will have only one instruction (line 3) inside it.
void : It indicates that the main method will not return any result other than executing the instruction present inside it.
The open curly bracket at the end indicates the beginning of the main method.
The instruction System.out.println() is a print instruction in Java that will display the message written inside the parentheses, which is Hello, Java! We end an instruction with a semicolon at the end.
The first closing curly bracket indicates the end of the method, and the second closing curly bracket indicates the end of the class.
A java compiler will convert this Welcome.java program into Welcome.class, which will be the bytecode. Then, a JVM will execute the main method inside this Welcome.class bytecode, where it will run the System.out.println() statement to display the message Hello, Java! Main.Java
Example 1.3 Program to display the product of two numbers.
Program to display “Welcome to the world of Java!” and “Hope you are having fun!” in a single line.
Whenever we display a message using System.out.println(), it first displays the message in the output then moves the cursor in the output to a new line. That is why in Example 1.1 on the previous page, the messages are displayed in two separate lines. But System.out.print() does not move the cursor to a new line. That is why in Example 1.3 above, the messages are displayed in the same line.
Example 1.4 Main.Java
Program to calculate the area of a rectangle whose width is 5 and height is 10.
Main.Java
Syntax refers to the set of rules defining how a program should be written. Every programming language has its own syntax. Similarly, the syntax of Java also defines all the main rules, commands, and constructions to write programs that the Java compiler will understand.
A few of the syntax rules in Java are:
● Every Java code has to be written inside a class, as it is one of the main principles of object-oriented programming that Java strictly follows.
● The main() method should be defined inside a class.
● In Java, we end any instruction with a semicolon (;). That is, each individual statement should be ended with a semicolon. It shows the compiler where an instruction ends and where the next instruction begins.
● Java is case sensitive, which means the names “showData” and “showdata” are two different names.
Syntax errors are mistakes in the code, such as spelling mistakes, punctuation errors, etc. It indicates that the programmer has written an invalid statement.
If the programme contains any syntax errors, the compiler will display them after compiling it.
Some common syntax errors are:
● Missing double quotes in printed statements
Main.Java
Output
● Leaving out the semicolon symbol at the end of any instruction.
Main.Java
Output
A variable is a memory location on a computer which can hold any value and can also change over time as needed.
To understand it in an easy way, think of it like a box in which you can store a number and later you can replace that number with another number.
In simple words, variables are names given to the memory location where your value is stored.
The figure below illustrates three variables with their respective values. The three variables used in the figure are “name”, “winner” and “score”. The variable “name” holds the value “Bob”, the variable “winner” holds the value “True”, and the variable “score” holds the value “35”.
Therefore, whenever the variable “name” is used in the program, it refers directly to its value, “Bob”.
Declaring a variable means creating a variable. If we want to use a variable in Java we need to create it in advance. To create or declare a variable we need to specify the data type of the variable along with the variable name.
Data type refers to the type of value the variable is going to hold.
Let us say that we want to create a variable to store a count. As the value of count will be a number, we use the keyword int, which means integer (a nondecimal number), to declare the type of the variable, and we can use count as the name of the variable.
String name; int count;
String is the data type used if the value of the variable stores characters or words within it. The data type is int if the variable is used to store an integer value.
In the example above, the variable “name” should hold characters or words within it, and the variable “count” should hold an integer value.
initialising a variable means giving a value to the declared variable for the first time. The syntax for initialising a variable is as follows.
Syntax: <data type> <variable name> = <value>;
Initialisation of the variable includes the following three components:
Data type : Type of data the variable will store.
Variable name : Name of the memory location where the value will be stored.
Initial Value : The value the variable will be initialised with.
int x; x=10;
In the above example, the first line, “int x”, is declaring the variable and specifying that the value stored in the variable is of integer type.
The second line, “x = 10”, gives the initial value to the variable x, which is of datatype integer.
Therefore, whenever the variable x is used in the program, it refers to the value stored within it, which is 10.
Data types allow us to define what type of data a memory location can hold. The main data types in Java are as follows:
byte 1 byte Stores whole numbers from -128 to 127 short 2 bytes Stores whole numbers from -32,768 to 32,767 int 4 bytes Stores whole numbers from -2,147,483,648 to 2,147,483,647 long 8 bytes Stores whole numbers from -9,223,372,036,854, 775,808 to 9,223,372,036,854,775,807 float 4 bytes Stores fractional numbers. Sufficient for storing 6 to 7 decimal digits double 8 bytes Stores fractional numbers. Sufficient for storing 15 decimal digits boolean 1 bit Stores true of false values char 2 bytes Stores a single character/letter or ASCII values
Aside from these d ata types, the most commonly used data type is String. It helps us store multiple characters in a variable, like “John”, “4 kilograms”, “$ 50”, etc. Program to store the number 5 in a variable and display it.
Example 1.6
In the program above, we have initialised the number variable with 5 in line 3, and in line 4, we display the value of the variable using the print instruction. We can group Java variables into the following categories based on the data types we learned:
Fundamentals of Java
● String variables : Store letters and numbers within double quotes.
● Integer variables : Store numbers without decimal values.
● Float variables : Store numbers with decimal values.
● Character variables : Store a single character such as “a”, “b”, etc.
● Boolean : Stores only true or false values.
Example 1.7
Program to store three numbers 5, 6, and 10 in different variables and display their sum.
Main.Java
Output
In the program above, three integer variables number1, number2, and number3 are declared in line 3. In lines 4, 5, and 6, the variables are initialised with their respective values using assignment statements. Then, the sum is calculated in line 7, and line 8 displays the sum.
The following are the types of variables in Java:
1. Local Variable
2. Instance Variable
3.
A variable that is defined and created within a method, constructor, or function is called a local variable. These variables are created when the function or
method is created and destroyed once the control comes out of the block. Therefore, the scope of the local variable lies within the function or method for which it has been created. The variable cannot be used outside the block it has been initialised to.
Main.Java
Output
A variable declared inside the class but outside the body of the methods, is called an instance variable. It is not declared as static.
Example
Main.Java
Java
A variable that is declared as static is called a static variable. It cannot be local. You can create a single copy of the static variable and share it among all the instances of the class. Memory allocation for static variables happens only once when the class is loaded into memory.
Variables are one of the fundamentals of the Java language. A few more fundamentals are:
Java tokens are the smallest element of a Java program. These are the basic building blocks, which can be put together to make a program. Java tokens can be identifiers, keywords, constants, variables, punctuators (like {, ;), or operators (like +, *).
An identifier is any name given to different components of a Java program. Identifiers are used to name classes, methods, and variables. Identifiers can be short names, like x and y, or more descriptive names, like age, sum, choice, result, etc. It is better to use descriptive names in order to make your programs easy to understand and maintain.
Keywords are reserved words in Java that have a special meaning and can only be used for that purpose. We cannot use the keywords to name any variable, class, or method in Java, as doing so will show an error. A few keywords in Java are public, static, void, class, int, float, char, if, while, for, etc.
They are the fixed values that do not change during the execution of a program. Constants can be numeric constants or character constants.
Numeric constants: 12, -5, 2334, -786, 1.55, 0.756, etc.
Character constants: They can be single character constants, like “P”, “a”, “B”, etc., or multi-character (string) constants, like “Kips”, “Welcome”, etc.
The general rules for naming a variable in Java are:
● Names can contain letters, digits, underscores, and dollar signs.
● Names must not begin with a number.
● Names cannot contain blank spaces.
● Names are case sensitive, so “myVar” and “myvar” are two different names in Java.
● Java keywords, such as int or boolean, cannot be used as names.
● Names in Java can be of any length.
● Variable names can also begin with $ and _. A few examples of valid variable names are count, name, _num, num1, num_1, $name, etc. However, names like 1num, tile count, and value #1 are invalid variable names.
When we have two or more distinct words in our variable name we can write them without spaces in between and capitalise the first letter of each new word.
For example - “Name of the company” can be written as “nameOfTheCompany”. As this represents the camel’s hump we call it Camel-Case
Words first word lower
Operators are special symbols that are used to perform calculations. We can use operators with variables and constants to form expressions.
Arithmetic operators in Java are used along with the integer or float values and variables to perform mathematical operations on them.
Operator Name Description Example + Addition Adds two values together X + y - Subtraction Subtracts one value from another X - y * Multiplication Multiplies two values X * y / Division Divides one value by another X / y % Modulus Returns the division remainder X % y
They are used to compare two values (or variables). This is important in programming, because it helps us to find answers and make decisions. The result of a comparison is either true or false.
Operator Name Example == Equal to x == y != Not equal x != y > Greater than x > y < Less than x < y >= Greater than or equal to x >= y <= Less than or equal to x <= y
They are used to compare the result of two or more comparisons in Java and give the result as true or false.
Operator Name Description Example && Logical and Returns true if both statements are true x < 5 && x < 10 || Logical or Returns true if one of the statements is true x < 5 || x < 4 ! Logical not Reverse the result, returns false if the result is true !(x < 5 && x < 10)
These operators work on a single variable or constant. They are used to increase or decrease the value stored in a variable by 1.
Operator Name Description Example ++ Increment Increases the value of a variable by 1 ++x Decrement Decreases the value of a variable by 1 --x
Java performs the following operations in order to solve a mathematical expression:
(B)
(E)
Example
9 + 7 * (8 - 4) / 2 - 3
= 9 + 7 * 4 / 2 - 3 (First, the brackets are solved.)
= 9 + 28 / 2 - 3 (Then, multiplication is solved.)
= 9 + 14 - 3 (Then, division is solved.)
= 23 - 3 (Then, addition is solved.)
= 20 (Finally, subtraction is solved.)
Example 1.11 Main.Java
Output
In the above program, when num1++ is used, Java first displays the value of num1 and then increases its value by 1. That is why line 5 displays 5 as num1 is 5, then Java increases the value of num1 to 6 (5 + 1) and displays the new value in line 6. However, when ++num2 is used, Java first increases the value by 1 and then displays the value of num2. So in line 10, Java increases num2 to 6 (5 + 1) and displays the new value.
To get input from the user, we have to use the Scanner class. The Scanner class can be imported from the java.util package, as shown below. To use any of the Scanner class’s methods, we must first create an object of the class. Below is an example of how to create and use an object of the Scanner class to call a method from the class.
The decision statement in Java allows you to make a decision based on the outcome of a condition.
Consider a real-life scenario where you are cycling and suddenly a puppy runs in front of you. What would you do? You will surely stop cycling. However, you would have kept going if the puppy hadn’t crossed the road. This is how we make decisions in real life.
Generally, a Java program runs the code in sequence. But we can control the order in which the program executes and run specific code based on the outcome of a condition. This is accomplished through the use of control flow statements, such as if else.
Control flow statements allow us to specify a set of instructions that should be executed only if certain conditions are met.
In this chapter, we will learn the following control flow statements for decisionmaking in Java:
● If Statement
● If-else Statements
● Nested-if Statements
● If-else-if Statements
The if statement is used to execute a statement or a block of statements only when the given condition becomes true.
Consider a scenario where you need to check if someone is eligible to vote or not. How would you know if someone is eligible to vote?
One can vote if he or she is over the age of 18. Right!
Syntax:
if (condition)
{
}
//Task to be performed if the condition becomes true.
If the condition becomes true, then the statements inside the block will be executed; otherwise, they will not. The control goes to the next statement after the closing braces.
Example 2.3
A program to check which of the two variables x and y has the greater value.
Main.Java Output
A program to check whether the number is even.
The if-else statement has two sets of codes to be executed in either case if the given condition is true or false.
Let us consider the example of eligibility to vote again to understand how ifelse works. Take a look at the flowchart below. If the condition is met, the person is eligible to vote; otherwise, they are not eligible to vote.
Syntax: if (condition) { //Task to be performed if the condition becomes true. } else{ //Task to be performed if the condition becomes false. }
If the condition becomes true, then the statements inside the if block will be executed; otherwise, the statements inside the else block will be executed. A program to print “Good day” if the time is less than 18 or else print “Good evening.” Main.Java
“Nest” means within. In Java, an “if statement” is referred to as being “nested” if it is stated inside another “if statement” or an “if-else statement.” Another if statement may also be present inside the if statement. In fact, there is no limit to how deep a nest can go. Nested-if statements are very common in Java programming. Let’s compare three numbers with values of 12, 30, and -15 as an example. Check which number is the largest by looking at the flowchart below.
Syntax: if (condition1) { if (condition2)
{ //Task to be performed if condition1 and condition2 becomes true.
} } else{ //Task to be performed if condition1 becomes false. }
Assume that a = 30, b = 12, and c = 15. First, the outer if condition is examined. Control goes to the nested if block since the outer if condition is true. Now that the Nested-if condition has been checked and found to be true as well, the output is “30 is the biggest number.”
The output would have been “Either 12 or -15 is the largest” if the outer if condition had been false. In that case, the control would have moved to the outer else block.
Example 2.7
A program to check the greatest of three numbers. Main.Java Output
Example 2.8
A program to check the values of a and b. Main.Java
An addition to the if-else statement is the if-else-if ladder statement. It is used in situations where multiple cases need to be checked. If one of the conditions in an if-else-if ladder statement is true, the statements defined in the if block will be executed. If some other condition is true, the statements defined in the else-if block will be executed. Eventually, the statements defined in the else block will be executed if none of the conditions are true.
Several else-if blocks are possible. If none of the conditions match, the default statement is executed. Let’s look at the example below to understand better.
Syntax: if (condition1) {
//Task to be performed if condition1 becomes true. } else{ if (condition2) {
//Task to be performed if condition1 becomes false and condition2 becomes true. } else{
//Task to be performed if condition1 and condition2 both become false. } }
Example 2.9
A program to print “Good Morning”, “Good Day”, and “Good Evening” depending on the time.
Main.Java
Output
Example 2.10
A program to check the value of a variable.
Main.Java
1. Write a program to check whether the student has passed the exam or not.
2. Write a program to assign a number within 1, 2, and 3 to a variable and print it in words as per the integer number.
3. Write a program to check whether 2023 will be a leap year or not.
In programming, a loop executes a set of instructions until a termination condition is reached. Loops are also known as iteration statements.
In real-life, we usually play our favourite songs on a loop. Here, the word loop represents playing that favourite song repeatedly.
In programming, we can run a statement multiple times using a loop. Let’s say we want to print “hello” ten times. This can be done in two ways:
1. Write the print statement ten times.
2. Write the print statement once and put it in a loop to run ten times. Of course, the second alternative is always more convenient because it means we have to write less code.
There are three iteration statements in Java.
1. while
2. do-while
3. for In this chapter, we will learn about while and for loops.
A while loop repeats a statement until the looping condition is true.
variable initialization condition task
keyword decrement
System.out.printIn(i);
Syntax: //variable initialization while (condition) { //Task to be performed until the condition is true. //increment/decrement statement } i=5; while (i>1){ i--1; }
The condition can be an expression which returns a boolean value. i.e. true/ false. The body of the loop will run only until the condition is true. Once the condition returns false, the loop terminates.
Example 3.1
A for loop needs a loop control variable which acts as the counter. Syntax: for(initialization;condition;increment/decrement){ //body of the loop }
Observe that there are 3 sections inside the parentheses separated by semicolons.
● The initialisation section runs when the loop starts. This section sets the initial value of the loop control variable.
● Then, we have a termination condition. The loop runs until this condition is true.
● The last portion is an expression which increments or decrements the loop control variable.
For every iteration, the condition is checked. If the condition is true, the body of the loop is executed. Then, the loop control variable is incremented/ decremented. This is repeated until the condition is false. We can skip the curly brackets if the body of the loop contains only one line.
Output
Factorial of 4:24
Jump statements are control statements that transfer control of the execution of a program from one place to another. There are two jump statements in Java: break and continue.
The break Statement is used to exit a loop. Syntax: break;
Example
Consider the same program which we wrote to print the first 10 natural numbers. Let’s add a break statement in this program.
In this program, the for loop runs until the condition i==7 is met. When i==7 is true, the break statement is executed and it exits the loop. Output
The continue Statement is used to skip a particular iteration. Syntax: continue; Example – Consider the same program which we wrote to print the first 10 natural numbers. Let’s add a continue statement to this program.
In this program, the for loop runs until the condition i==7 is met. When i==7 is true, the continue statement stops running the statements inside the loop for that iteration. The loop resumes from the next iteration.
Chapter 3 Loops with Java
1. Write a program to find the sum of the following series: 1 + 4 + 7 +. . . . . . + 28.
2. Write a program to determine if a number is prime or not.
Project: Rock Paper Scissors
Create a Rock, Paper, Scissors game in Java where one player will be the computer and the other player is the user.
Rock, Paper, Scissors is a hand game usually played between two people where both people form one of three objects (a rock, a piece of paper, or a pair of scissors) with their hands. The winner is decided based on the following:
● Rock defeats scissor (Rock annihilates scissor)
● Scissors cut paper (cut paper with scissors)
● Paper beats rock (or paper wraps rock).
The game will be considered over if both players make a similar hand formation.
2. Program to assign a number within 1, 2, and 3 to a variable and print it in words as per the integer number.
Solution Main.Java Output
3. Write a program to check whether 2023 will be a leap year or not.
Solution
Main.Java Output
1. Write a program to find sum of the following series: 1 + 4 + 7 +. . . . . . + 28 Output Output
2. Write a program to determine if a number is prime or not.
Create a Rock, Paper, Scissors game in Java where one player will be the computer and the other player is the user.
Rock, Paper, Scissors is a hand game usually played between two people where both people form one of three objects (a rock, a piece of paper, or a pair of scissors) with their hands. The winner is decided based on the following:
● Rock defeats scissor (Rock annihilates scissor)
● Scissors cut paper (cut paper with scissors)
● Paper beats rock (or paper wraps rock).
The game will be considered over if both players make a similar hand formation.
Solution