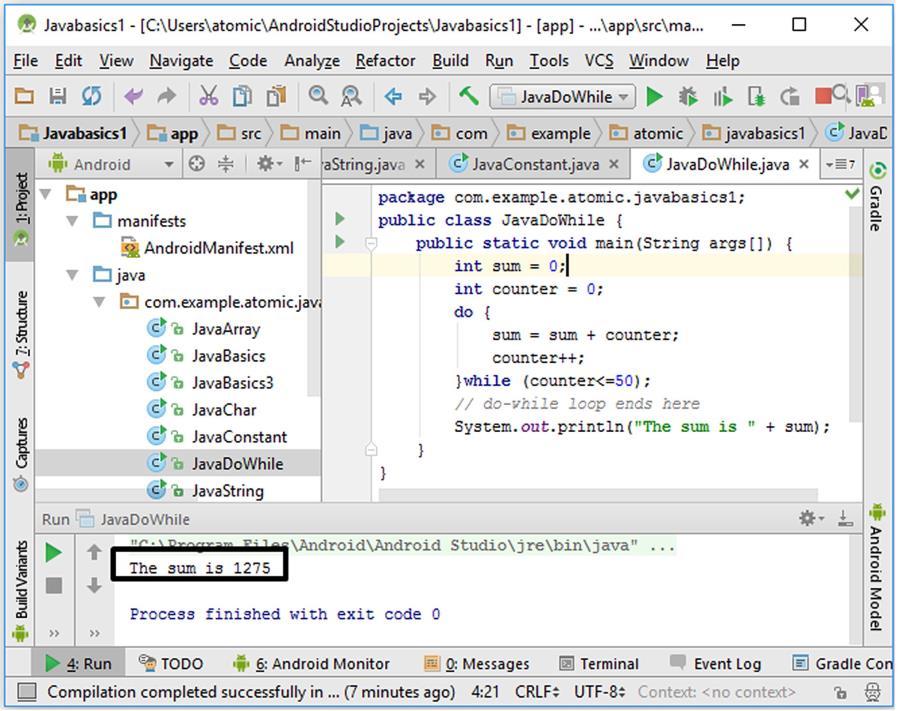
4 minute read
4.6. Methods in Java
comment line in Java. The compiler ignores anything written next to //. Comments are used for increasing the readability of the code.
The output of the do–while program is shown below:
Advertisement
Figure 4.23. do–while loop example in Java
Note: There are two important keywords that are used to further control loops: break and continue statements.
These commands are usually used together with an if statement. The break command breaks the loop; it means the program quits the current loop before the loop condition expires. On the other hand, continue command makes the loop continue with the next iteration
4.6. Methods in Java
Methods are subroutines that are used for performing an operation. Methods are similar to functions in other programming languages but the difference is that methods are always associated with classes and objects.
Because of this, methods are always defined inside classes. The general form of a function is as in Code 4.24.
(public) (void) (return type) methodName (arguments){ ......... (code inside the method) (return output_values;)
}
Code 4.25
A method declaration has the following parts:
The name of the method (methodName). Method identifiers like public and static (optional). Arguments (input values) of the method (optional). The type of return values (optional). The void keyword–used if the method won‟t output any value (optional). return keyword for outputting return values (optional). The statements that will perform the operation.
Let‟s write a method that adds two integers and prints the sum on the terminal:
static void addNumbers(int a, int b){ int sum; sum = a + b;
System.out.println("The sum is " + sum);
}
Code 4.26
In this method:
The static keyword is used that means this method can be called without creating an object of its class. void keyword is used because the method won‟t output any values; it will just print on the terminal screen. Inputs (arguments) of the method has two input variables a and b which both are of the int type.
The sum is calculated inside the function and assigned to the sum variable. Please note that the variables which are defined inside the function cannot be accessed outside the function. Finally, the sum is printed on the terminal screen with the usual
System.out.println() method.
When we define a method, it doesn‟t run automatically. We need to call it with its input arguments. We do this by writing its name and arguments as follows:
addNumbers(2, 5);
Code 4.27
When we call this method, the input arguments are 2 and 5. The method will add these numbers and print the result on the screen. The complete code of the method definition and its call is as follows:
package com.example.atomic.javabasics1; public class JavaMethodAdd1 {
public static void main(String args[]){ addNumbers(2, 5);
} static void addNumbers(int a, int b){ int sum; sum = a + b;
System.out.println("The sum is " + sum);
}
Code 4.28
When we run this program in Android Studio, we get the terminal output shown in Figure 4.24.
This function didn‟t have return values. Let‟s modify it so that the sum of the input values will be given as a return value. We can do this modification by just adding the following line instead of the
System.out.println():
return sum;
Code 4.29
We also have to replace the void keyword to int keyword as shown in Code 4.29 because the function will output an int type variable (sum).
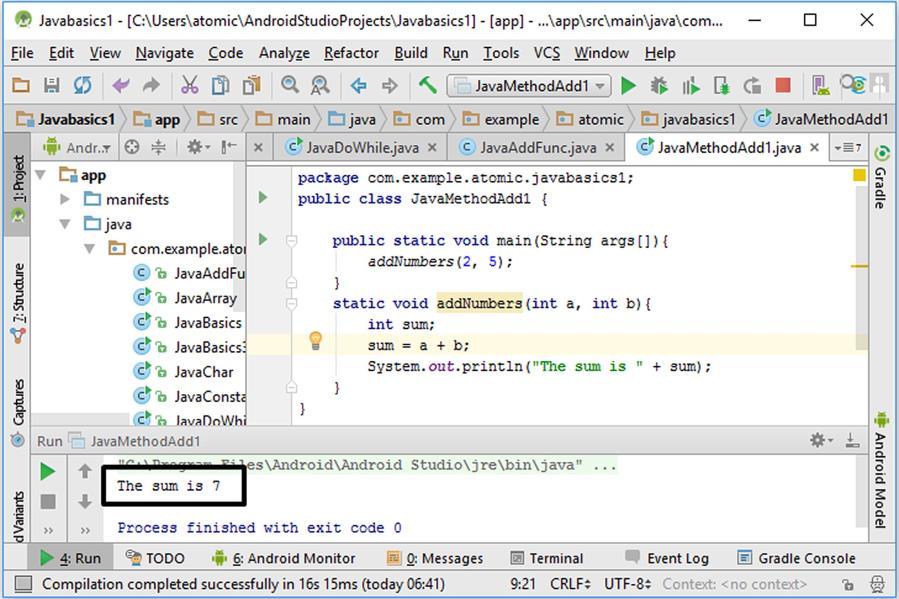
Figure 4.24. Method definition and calling in Java
package com.example.atomic.javabasics1; public class JavaMethodAdd2 {
public static void main(String args[]){ addNumbers(2, 5);
} static int addNumbers(int a, int b){ int sum; sum = a + b; return sum;
}
Code 4.30
When we run the code above, nothing happens because we removed the printing code from the method and it only outputs the sum. We can print the output of the method as follows:
package com.example.atomic.javabasics1; public class JavaMethodAdd2 {
public static void main(String args[]){
System.out.println(addNumbers(2, 5));
} static int addNumbers(int a, int b){ int sum; sum = a + b; return sum;
}
Code 4.31 (cont‟d from the previous page)
We have written the method call inside the System.out.println() method therefore the return value of the method will be printed as shown below:
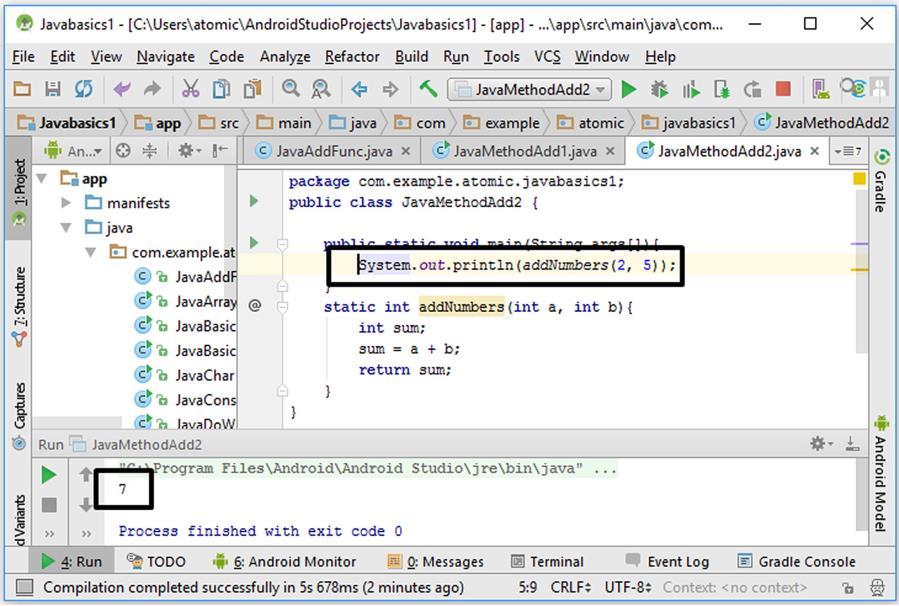
Figure 4.25. Using a method that returns a value
Methods provide us a good way of shortening our code and making it compact as we can see from these simple examples. Of course their usage range is not only simple mathematical operations, a Java or an Android application contains a lot of user defined and ready methods available from the Android SDK or Java SDK. Android SDK includes thousands of methods which makes the developers‟ lives easier.