Low TW, Fadeyi MO, and Low MYH (2021). Guidance for performing automatic verification of building information modelling (BIM) files using Python. Built Environment Applied Research Sharing #07 (Technical Note). ISSUU Digital Publishing.
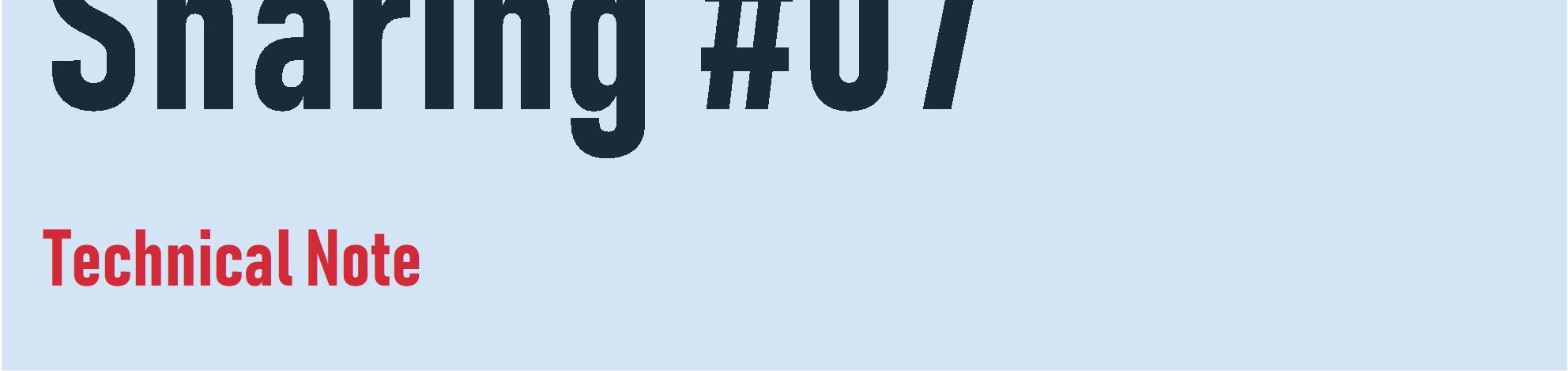
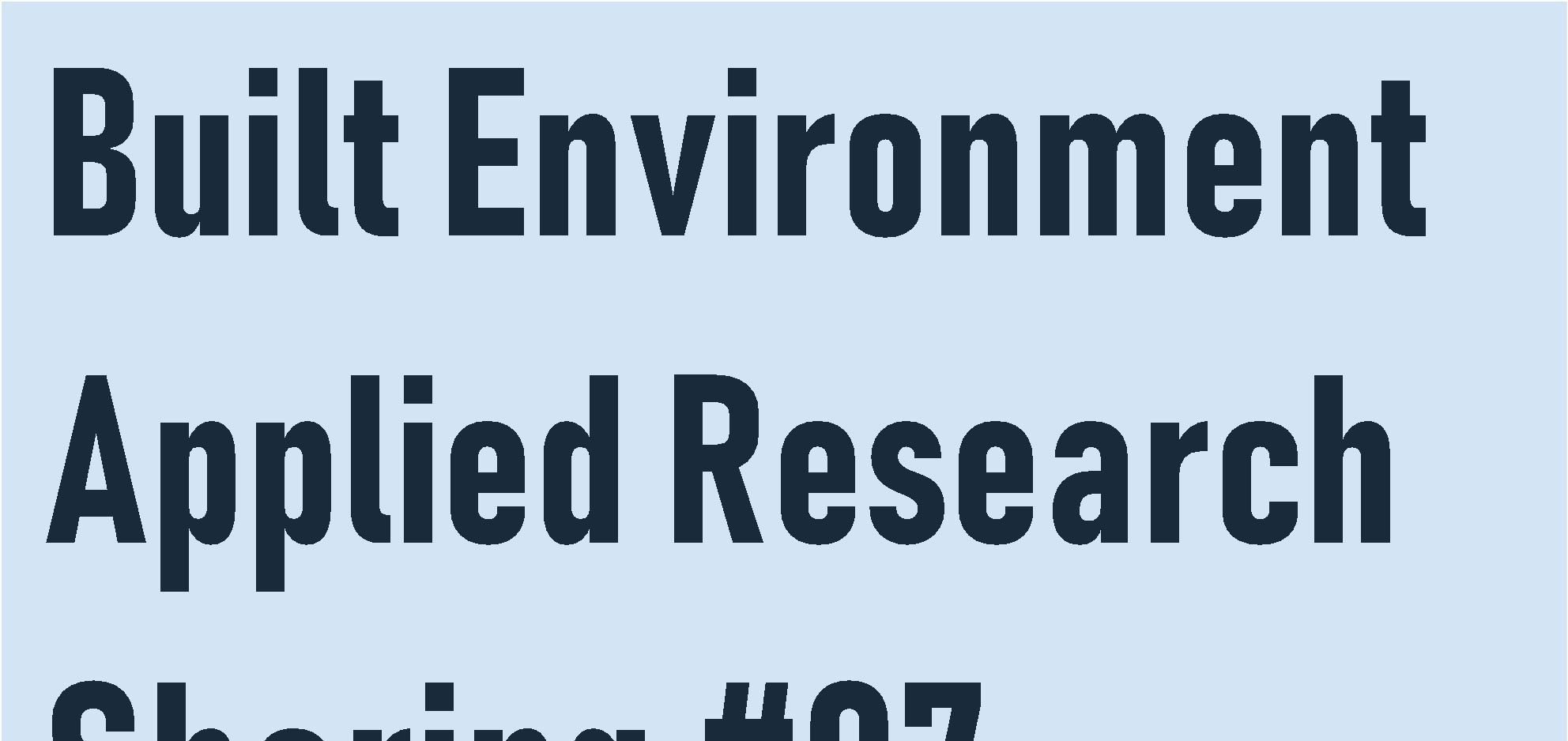
Low TW, Fadeyi MO, and Low MYH (2021). Guidance for performing automatic verification of building information modelling (BIM) files using Python. Built Environment Applied Research Sharing #07 (Technical Note). ISSUU Digital Publishing.
Teck Wei Low1, Moshood Olawale Fadeyi,2* , and Malcolm Yoke Hean Low1
1Infocomm Technology Cluster, Singapore Institute of Technology, 10, Dover Drive, Singapore 138683, Singapore
2Sustainable Infrastructure Engineering (Building Services) Programme, Singapore Institute of Technology, 10, Dover Drive, Singapore 138683, Singapore
*Corresponding author’s email: fadeyi.moshood@singaporetech.edu.sg
This technical note provides guidance on how python can be used to automate the process of verifying building information modelling (BIM) files. The research conducted and reported in this paper is a follow up to the Chua and Fadeyi (2021) study in which dynamo was used to automaticallyverifyBIMfiles Theguidingquestionsforthispresentstudywere:(i) Would the excel outputs from dynamo and python be the same? (ii) Can the use of python provide better customisation of the verification process and the derived building information than dynamo? Excel outputs from python and dynamo were found to be the same or similar when compared. For verification functions where the outputs are similar, python provided conciseinformationneededbetterthanthedynamobecauseofthebettercustomisationbenefits python provides. Python also provided better customisation of the process of doing the automatic verification. The automatic verification benefits of python, like in dynamo, can significantly optimise the process and productivity of validating building information modelling (BIM) files.
Keywords: Building information modelling, Digital facility management, Productivity, Python, Computational thinking
This technical note contains steps for developing an automatic verification of BIM files using python as an alternative to existing published automatic verification solution using dynamo reported in Chua and Fadeyi (2021) in the hope of better usability and performances. All the solutions reported in this paper have been tested against the building models in Chua and Fadeyi (2021) and results obtained therein. Sample video recordings of the developed solution using python versus that of dynamo can be found in the Supplementary Information A section of this technical note. Installation guides for the Autodesk Revit and PyRevit can be found in Supplementary information B and C sections, respectively. The guiding questions for this present study were: (i) Would the excel outputs from dynamo and python be the same? (ii) Can the use of python provide better customisation of the verification process and the derived building information than dynamo?
Autodesk Revit will be used as the main BIM software to host the BIM files. It also provides an API for consumers and developers to develop custom scripts using three primary programming languages: C#, Visual Basic, and VB.NET. More information about the Revit API can be found in the following link: https://www.revitapidocs.com/.
PyRevit is an extension utility written in Python to provide consumers and developers with an easy way to extend functionalities into Autodesk Revit to improve workflow by acting as an intermediary and serving as a transpiler to convert python scripts into usable C# scripts for interfacing with the Revit API. PyRevit scripts follow similar namespace patterns in terms of package declarations, function names, and variable naming conventions to provide developers with better clarity when referring to the Revit API documentation. An example can be seen in Figure 1 and Figure 2 below.
Figure 1: DB Namespace defined in Revit Documentation
PyRevit also provides developers with a convenient way to add GUI elements to Autodesk Revit’s toolbar through simple naming and folder organizations. More details can be found in the following link: https://pyrevit1.readthedocs.io/en/latest/creatingexts.html. The following information serves to highlight its ease of use. The scripts' organisation and the naming of the folders define and correlate to the navigation structure when accessed through the extension tab. A folder named “lib” can be placed in the top level to allow its content to function as a library that can be imported into the other GUI scripts. A directory structure example can be seen in Figure 3.
From Figure 4 below, a “_layout” file can be defined in each bundle folder to allow some level of customisation and enforce ordering of the menu items.
Figure 4: Sample Layout file under BIMValidation.panel
Figure 5 shows the reflected extension GUI placements in reference to the sample directory structure in Figure 3.
Figure 5: Extension reflecting Directory Structure
In Revit API, most of the required classes can be found in the DB namespace. The Revit API is structured to involve a mixture of inheritance hierarchies and compositions of information for dynamic, variability, and flexibility. The following classes are more commonly used for the developed solution in this paper. More information can be found in the following link: https://www.revitapidocs.com/.
FilteredElementCollector: This built-in class provides an interface to collect and iterate through all elements in the BIM files using filters.
BuiltInCategory: This class contains a set of named constants that are used to categorise elements, which will be one of the main ways to identify elements.
Element: This class is one of the base classes that the solutions in this paper will be interacting with.
ParameterMap: This object can be found in each element and serves to hold parameterized values.
For interfacing with Microsoft Excel Sheets, Microsoft COMObject API will need to be used. Figure 6 is an example showing how one might link the COMObject API into the script. Like the code structure for PyRevit to interface with Revit API, the package declarations, function
names, and variable naming conventions are close to how one might code it in VBA. More information can be found in the following link: https://docs.microsoft.com/en-us/office/clientdeveloper/excel/excel-home.
Figure 6: Import Excel COMObject API
The solution is broken up into two main components. All the core solution procedures can be found in the “lib” folder. All the scripts existing in the extension navigation structure contain enough necessary information to provide GUI support and invoke the correct routines from the “lib” folder. This allows clear separation of concerns between mangling with the GUI code and performing the actual task required.
This script contains common routines that might be used across the whole or part of the solution. In reference to Figure 7, CategoryOption class and ClassOption class will be used as a wrapper to interface with PyRevit’s form dataset. These two classes exist as some elements can only be selected through filtering by class instead of “BuiltInCategory”.
In reference to Figure 8, some methods were created to facilitate uses with Revit’s parameter class, as some repeated additional type and error checking need to be done.
In reference to Figure 9, Octree spatial data structure was implemented to facilitate indexing of elements by their logical boundaries to provide logarithmic search.
In reference to Figure 10, this function is implemented to reduce repetitive typing for sorting elements by their element id. Figure 10: Sorted elements function
This script mainly contains functions that help to reduce the repetition of common excel routines for the solutions. Figure 11 depicts two functions that act as a wrapper that automatically opens the workbook, forward relevant objects to the handlers, and finally close the workbook and clean up any resources, even when there are errors or exceptions raised so that memory leak can be avoided.
Figure 11: Wrapper functions
Figure 12 depicts a function that helps to format the entire excel sheet in terms of horizontal alignment and cells' resizing to autofit its content.
Figure 12: Format function
Figure 13 depicts a function that helps to set borders by specifying boundaries using the top left cell and the bottom right cell as the corner indicator.
Figure 13: Set border function
Under the lib folder, attempts were made to further isolate logical components for better
readability and maintainability if any unexpected changes were required, excel related logic was shifted into a separate file, suffixed with “_excel” shown in Figure 14a below.
Under the extension folder, Figure 14b shows the general outline of the navigation structure for this solution. The functions are grouped logically and placed under each pulldown folder as a button.
Figure 14b: General navigation structure
In this section, a total of 13 functions will be covered. Only information pertaining to the core of the solution will be discussed in this section. Sections 3.4.1-3.4.4 cover room specific functions. Sections 3.4.5-3.4.8 cover shared implementation for mechanical and electrical equipment. Sections 3.4.9-3.4.13 cover shared implementation for both room and equipment related functions. Excel inputs and outputs algorithms will not be covered here as they are considered visual output and formatting. Excel inputs and outputs algorithms for the verification functions can be found in the sample video recordings provided in the Supplementary Information A section.
The following picture depicts an activity diagram describing the solution.
Figure 15: Check room information w/o existing information activity diagram
In reference to Figure 16, room elements can be collected using the “FilteredElementCollector” class and filter by category using the BuiltInCategory “OST_Rooms”. Figure 17 depicts an example to filter by level id. Room name can be accessed using the room element’s ParametersMap. Figure 18 depicts an example to obtain information from the room element through direct reference and the ParametersMap property.
Figure 16: FilteredElementCollector Code Snippet
Figure 17: ElementLevelFilter Code Snippet
The following picture depicts an activity diagram describing the solution.
The idea of this solution is to keep multiple sets of rooms indexed by their room number. In Figure 20, if a set has more than one room in it, there will be duplication of the room number. Sets were used instead of a simple integer counter to eliminate potential repetitions of elements. For the collection of rooms and retrieval of room numbers, refer to Figures 16-18 in section 3.4.1.
Figure 20: Filter for duplicated room numbers
The following picture depicts an activity diagram describing the solution.
Figure 21: Check room boundaries activity diagram
In reference to Chua and Fadeyi (2021), a room has an invalid boundary if its calculated area is 0 or invalid. For the collection of rooms and retrieval of room area, refer to Figures 16-18 in section 3.4.1.
The following picture depicts an activity diagram describing the solution.
Figure 22: Check room information w/ existing information activity diagram
In reference to Chua and Fadeyi (2021), the information involves applying symmetric difference (See Figure 23 below) between the input information and information in the BIM file. For the collection of rooms, refer to Figure 16 in section 3.4.1.
Figure 23: Symmetric difference code snippet
The following picture depicts an activity diagram describing the solution.
Figure 24: Check equipment information w/o existing information activity diagram
This solution is like Section 3.4.1, with the only main changes being the “BuiltInCategory” involved (See Figure 25), specifically “OST_MechanicalEquipment” and “OST_ElectricalEquipment”, including the parameters to check for, depending on the requirements.
Figure 25: Collect equipment code snippet
The following picture depicts an activity diagram describing the solution.
For the collection of equipment, refer to Figure 25 in section 3.4.5. For a brief overview of why the set subtraction works, refer to Figure 27 for the code snippet and Figure 28 for a Venn diagram-based explanation.
Figure 27: Set subtraction code snippet
Figure 28: Venn diagram explanation
The following picture depicts an activity diagram describing the solution.
Figure 29: Check for duplicated equipment label activity diagram
This solution is like section 3.4.2. Rather than “OST_Rooms”, the BuiltInCategory argument
is either “OST_MechanicalEquipment” or “OST_ElectricalEquipment”, and instead of room numbers, the value of interest is under the parameter “Mark”.
The following picture depicts an activity diagram describing the solution.
Figure
This solution is like section 3.4.4. Rather than “OST_Rooms”, the BuiltInCategory argument is either “OST_MechanicalEquipment” or “OST_ElectricalEquipment”, and instead of room numbers, the value used as the index is under the parameter “Mark”.
The following picture depicts an activity diagram describing the solution.
Figure 31: Check for critical interference activity diagram
This solution consists of multiple phases and can be applied to both rooms and equipment. The Option classes mentioned in section 3.2.1 are also responsible for the retrieval of its elements. Octree was chosen as it helps to reduce the time complexity when looking for overlapping or colliding elements, from O(mn) to potentially O(m log(n)), where m refers to the main set and n referring to the elements to test against. Referring to the Chua and Fadeyi (2021), this solution has been tested against elements in the order of thousands. Using the critical interference report by Revit under the collaboration tab took approximately 1 hour and more. With this solution, it only took about 8.5 minutes. Results from this solution were cross referenced with the exported result through Revit’s GUI exposed function and reported result in Chua and Fadeyi (2021).
Figure 32 shows the method uses to determine if it is intersectable. In reference to Figure 33, the bounding box of each intersectable element is used for the Octree spatial data structure. After which, the broad detection (See Figure 34) is simply running through each element against the Octree to obtain its potentially overlapping or colliding nearest neighbors. This function produces a dictionary whose key is the main element, and its value being a set of elements to test against.
As this solution utilises multiple sets of elements, there is no guarantee that there will not be any element with multiple occurrences. Hence it is beneficial to perform the removal of duplicated test cases to speed up the interference checks. Figure 35 illustrates the concept as such. This algorithm aims to remove elements from other element’s set of test cases where duplicated test cases are observed.
A test case is considered equal when symmetric relation exists in the form of (A, B) and (B, A), where A and B are elements. Using mathematical constructs, the algorithm is as follows. Let D be the dictionary, K be the key, D[K] be the value, and Vn be each element in D[K]. For each K in D, for each Vn in D[K], D[Vn] – K. Finally, the narrow detection phase is performed by iterating through the resulting dictionary, using the set of test cases as the main pool for the “FilteredElementCollector” and passing it through the built in “ElementIntersectsElementFilter” using the main element. (See Figure 36).
Figure 32: Intersectable Check Code Snippet
Figure 33: ElementLeaf Code Snippet
Figure 34: Broad Detection Code Snippet
Figure 35: Removal of Duplicated Test Cases Code Snippet
Figure 36: Narrow Detection Code Snippet
The following picture depicts an activity diagram describing the solution.
For the collection of elements, refer to Figure 16-17 in section 3.4.1. Figure 38 shows a map function to extract parameter values from each element. More details of the “get_param_value” function can be found in section 3.2.1.
Figure 38: Parameter Extraction code snippet
The following picture depicts an activity diagram describing the solution.
All modification to the BIM file using scripts requires the process to be wrapped within a transaction object. (See Figure 40) More details about “compare_parameter_value” and “set_parameter_value” functions can be found in section 3.2.1.
Figure 40: Import element code snippet using transaction
The following picture depicts an activity diagram describing the solution.
As mentioned in section 3.4.11, all modification to the BIM file using scripts requires the process to be wrapped within a transaction object. Figure 42 illustrates the underlying code for the deletion of elements. This solution gave rise to the two option classes, “CategoryOption” and “ClassOption”. Due to type constraints, some elements are not tagged to any BuiltInCategory, and can only be referenced and filtered by class. Figure 43 depicts an example usage of the options. It can also be seen that to differentiate between CAD Imports and CAD
Links, the property “IsLinked” needs to be checked, which further complicates the requirements, such that it is now required for the option to cater for category types, class types, and property differentiated types.
Figure 42: Deletion of element code snippet
Figure 43: Usage of option classes code snippet
The following picture depicts an activity diagram describing the solution.
Figure 44: Lighten BIM files overall activity diagram
As mentioned in section 3.4.11, all modification to the BIM file using scripts requires the process to be wrapped within a transaction object. In this solution, the need to click through multiple times using Revit’s Purge function can be removed. Unfortunately, there is no direct way to automate this section. Thus, the solution will have to step through its own. As this solution runs through multiple routines, the following contains more detailed descriptions for each of them.
The following picture depicts a closer view of the activity diagram describing this routine.
Figure 45: Purge unused families routine activity diagram
This solution makes use of Revit’s internal class “PerformanceAdviser” (See Figure 46) with a fixed rule guid: “e8c63650-70b7-435a-9010-ec97660c1bda”. (See Figure 47) This rule guid specifically instructs the “PerformanceAdviser” to detect unused elements.
Figure 46: Performance adviser code snippet
The following picture depicts a closer view of the activity diagram describing this routine.
Figure 48: Purge unused materials routine activity diagram
In this section, the deletion of materials is done through trial and error. The first few activities only serve to reduce the number of materials to test. At the time of writing, there are no other known methods to look for used materials.
The transaction group is used to wrap the main transaction due to two constraints. Firstly, to be notified of any propagated modifications, the transaction needs to be committed. Secondly, a transaction alone cannot be rolled back, and thus removing materials will be persisted, which is undesirable if the materials are used. Hence, the transaction itself is used to perform the commits to detect modifications, while the transaction group is used to perform the rollback if anything goes wrong.
Due to context limitation, the listener used to watch for changes (See Figure 49-51) needs to be bounded to a context to be properly registered and notified.
Figure 49: Modification listener code snippet
Figure 50: Attach listener code snippet
Figure 51: Detach listener code snippet
The following picture depicts a closer view of the activity diagram describing this routine.
This solution utilises exhaustive trial and error to look for used materials over 4 areas. Figure 53 shows the first area, which is to investigate the “GetMaterialIds” method. It contains a Boolean parameter that toggles between what to check. Hence the method is invoked twice, with the two possible Boolean values, true and false.
Figure 54 shows the second area, which investigates the layers by assuming the element has a compound structure. The goal is to collect the material used for each of these layers.
Figure 54: Compound Structure Layers Code Snippet
Figure 55 shows the third area, which is to investigate the parameters of the element. The solution checks for parameters whose name contains the word “Material”. Although this may yield false positives that might not be materials, it does not affect the initially intended outcome, which is to subtract away any used materials from all the materials available indexed in the BIM file.
Figure 56 shows the last area, which investigates the element’s category, collecting any material tied to it, if any.
Figure 56: Checking of Element’s Category Code Snippet
The following picture depicts a closer view of the activity diagram describing this routine.
This section utilises set subtraction to identify unused assets. Structural Asset and Thermal Assets are instances of “PropertySetElement”. Hence (See Figure 58), by obtaining all the property set elements and subtracting all the structural asset and thermal assets used, unused property set elements can be obtained. Appearance Assets belongs to another class “AppearanceAssetElement”. Hence (See Figure 59), a similar concept is applied here, gathering all the appearance assets and subtracting the used appearance assets to obtain unused
appearance assets. With these unused assets, they can be sent for deletion.
Figure 58: Unused property set code snippet
Figure 59: Unused appearance asset code snippet
This technical note explored how python could be used to do automatic validation of building information modelling (BIM) files. The guidance for doing the automatic verification was documented in this technical note to improve digital facility management practice and productivity of building information verification. The applied research effort reported in this paper is a continuation of similar work conducted by Chua and Fadeyi (2021) with the use of dynamo. Python was explored to examine its potential in providing better customisation of the process of conducting the automatic verification and providing concise output of building information needed.
Excel inputs and outputs algorithms for the verification functions are provided in the Supplementary Information A section in video format. As evident in the sample video recordings, the developed automatic verification solution using python suggests excel outputs from python (on the left-hand side) and dynamo (on the right-hand side) from Chau and Fadeyi (2021) are the same or similar when compared. For automatic verification functions where the outputs are similar, python provided concise information needed better than a dynamo because of the better customisation benefits python provides. Nevertheless, python and dynamo can significantly optimise the process and productivity of validating building information modelling (BIM) files.
The Singapore Institute of Technology funds this project through a SEED grant (R-MOEA403-G008). Mr. Low Teck Wei did the project work and writing of this technical note in his position as a research assistant. Dr. Moshood Olawale Fadeyi and Dr. Malcolm Low designed the study and guided the development of the developed automated verification solution. Dr. Fadeyi and Dr. Malcolm Low also contributed to the development of this technical note. The
support of Ms. Camille Chua during the development of the automated verification solution is gratefully acknowledged.
Chua C and Fadeyi MO (2021). Identification and rectification of errors in BIM models using dynamo. Built Environment Applied Research Sharing #04. ISSUU Digital Publishing.
Supplementary A – Automated Solution
The video recordings of the developed solution can be downloaded from the links provided below. The authors reserved the right to the videos.
Check Room Information w/o Existing Information
https://www.dropbox.com/s/f64sm9ukgr9iha9/1.4%20Check%20Info%20Fields%20WO%20existing %20Info%20%28Archi%29%20%28PyRevit%29.mp4?dl=0
Check for Duplicated Room Numbers
https://www.dropbox.com/s/jevj7pufrytyk4n/1.2%20Check%20for%20Duplicated%20Room%20Num bers%20%28PyRevit%29.mp4?dl=0
Check Room Boundaries
https://www.dropbox.com/s/g56qkg548gff4pk/1.1%20Check%20Room%20Boundaries%20%28PyRe vit%29.mp4?dl=0
Check Room Information w/ Existing Information
https://www.dropbox.com/s/3tv6qd9dohm9x7d/1.4b%20Check%20Info%20Fields%20W%20existing %20Info%20%28Archi%29%20%28PyRevit%29.mp4?dl=0
Check Equipment information w/o Existing Information
https://www.dropbox.com/s/d7b8rek4jpfdfik/1.8a%20Check%20Info%20Fields%20WO%20existing %20info%20%28MEP%29.mp4?dl=0
https://www.dropbox.com/s/aeo78jr6aa8rfo5/1.5%20Check%20for%20Extra%20or%20Missing%20E quipment%20%28PyRevit%29.mp4?dl=0
Check for Duplicated Equipment Label
https://www.dropbox.com/s/hvqfu66vrki2woj/1.6%20Check%20for%20Duplicated%20Equipment%2 0%28PyRevit%29.mp4?dl=0
Check Equipment information w/ Existing Information
https://www.dropbox.com/s/l0hs5faaxjl7nw2/1.8b%20Check%20Info%20Fields%20W%20Existing% 20Info%20%28MEP%29.mp4?dl=0
Check for Critical interference (Archi)
https://www.dropbox.com/s/pelfslz6vymq0ro/1.3%20Check%20for%20Critical%20Interferences%20 %28Archi%29%20%28PyRevit%29.mp4?dl=0
Check for Critical interference (MEP)
https://www.dropbox.com/s/8opdhn3gf3qi0dr/1.7%20Check%20for%20Critical%20Interferences%20 %28MEP%29.mp4?dl=0
Delete Unwanted Items
https://www.dropbox.com/s/7f0j9rxp1ki9tj0/2.2%20Removal%20of%20Unwanted%20Items%20%28 PyRevit%29.mp4?dl=0
Lighten BIM Files
https://www.dropbox.com/s/w6uyy0sk3j2c7xk/2.3%20Lighten%20BIM%20Model%20Files%20%28 PyRevit%29.mp4?dl=0
Update Information (Archi)
https://www.dropbox.com/s/kua2e809z04zwez/2.1a%20Update%20Information%20%28Archi %29%20%28PyRevit%29.mp4?dl=0
Update Information (MEP)
https://www.dropbox.com/s/lzhq71cjuuwaqc9/2.1b%20Update%20Information%20%28MEP %29%20%28PyRevit%29.mp4?dl=0
1. Go to the following website:
https://www.autodesk.com/education/edu-oftware/overview?sorting=featured&page=1
2. Click the “Get started” button.
Figure S1: Product page, student and educators
3. Sign in or create a new account as needed.
Figure S2: Login page
4. Present identification document to request access.
Figure S3: Get started page, identification
5. Follow any further instructions to download and unlock the license key, as instructed by Autodesk Revit.
1. Installation of PyRevit
a. https://github.com/eirannejad/pyRevit/releases
b. The installer should be run after the installation of Revit. If another version of Revit is installed after PyRevit is installed, PyRevit needs to be reinstalled to appear on the new version.
2. The following tab should appear in the Autodesk Revit.
Figure S4: PyRevit extension tab
1. In the PyRevit tab, click this button to open a sub-window
Figure S5: PyRevit extension tab
2. Next, select the settings button
Figure S6: Configuration sub window
3. The extension folder should be added here
Figure S7: Settings window
4. Lastly, relaunch Autodesk Revit.
5. This tab should appear
Figure S8: New extension tab