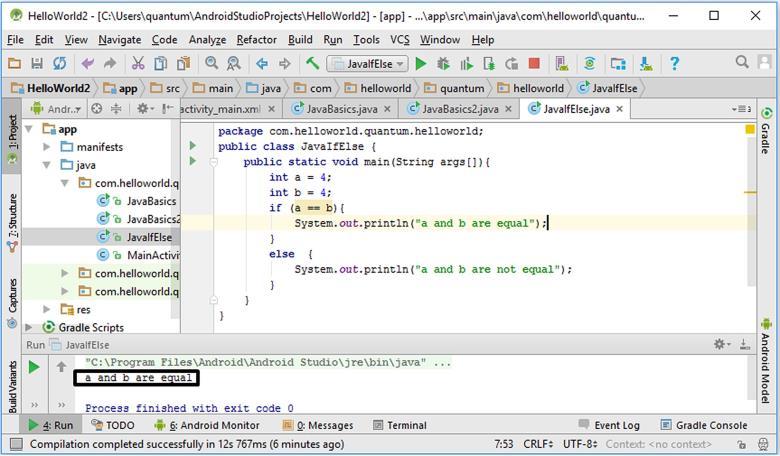
3 minute read
4.4. Logical Decision Making Statements in Java
Our next subsection is about the logical decision making structures in Java, let‟s have a coffee break and then continue with if-else and switchcase statements.
4.4. Logical Decision Making Statements in Java
Advertisement
Decision making is a widely in programming as in daily life problems. We frequently make logical decisions in daily life such as:
“If their coffee is tasty I‟ll get another one, else I‟ll grab a tea”.
“If it‟s rainy I‟ll take my umbrella, else I‟ll not”.
In a programming language, decision making statements controls if a condition is met or not as in real life. There are two decision making statements in Java: if–else and switch–case blocks.
If–else structure: In this conditional, if the condition is satisfied, the code inside the if block is executed. If the condition isn‟t satisfied, then the code in the else block is executed. Hence, if we need tell the rainy –not rainy example using an if–else block, we do it as follows:
if it‟s rainy {
I‟ll take my umbrella.
else {
I‟ll not take my umbrella.
Let‟s see how we can check if two numbers are equal in Java using an if-else statement:
package com.helloworld.quantum.helloworld; public class JavaIfElse { public static void main(String args[]){ int a = 4; int b = 4; if (a == b){
System.out.println("a and b are equal"); } else {
System.out.println("a and b are not equal"); }
}
Code 4.17 (cont‟d from the previous page)
Let‟s analyse this code:
In this code, two integer type variables, a and b, are created and initialized to 4. In the next line, the if statement checks if a and b are equal. The comparison operator == is used to check the equality. If the result of this comparison is true, the statement inside the if
block System.out.println("a and b are equal"); is executed which prints “a and b are equal” on the terminal screen. If the result of this comparison is false, the statement inside the if
block System.out.println("a and b are not equal"); is executed which prints “a and b are not equal” on the terminal screen.
Since we initialized both a and b to 4, they are equal and the Java compiler executes the code inside the if block as follows:
Figure 4.18. if –else example in Java
When we change one of the numbers to something other than 4, the code inside the else block is executed as shown in Figure 4.19.
Figure 4.19. if–else statement in Java when the condition not satisfied
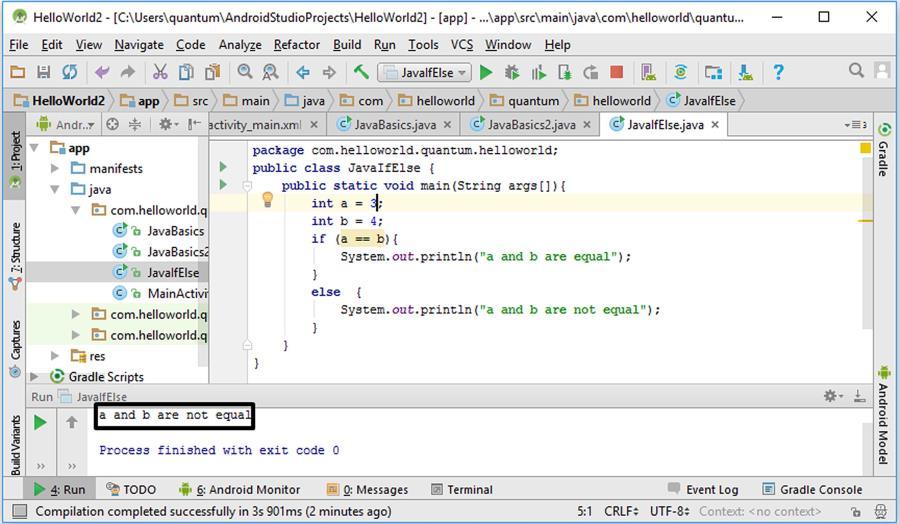
If–else statements can also be used in nested forms as in Code 4.17. In nested statements, the conditions are checked from top to down. When a condition is satisfied, then the code inside its block is executed and the program ends. If none of the conditions are true, then the final else block is executed. In other words, the statements in the last else block is executed if none of the conditions above it are satisfied. The screenshot of this nested code in the playground is given in Figure 4.20.
package com.helloworld.quantum.helloworld; public class JavaNestedIfElse { public static void main(String args[]){ int a = 3; int b = 4; if (a == b){ System.out.println("a and b are equal");
} else if(a > b) { System.out.println("a is greater than b");
} else { System.out.println("a is lower than b");
}
Code 4.18 (cont‟d from the previous page)
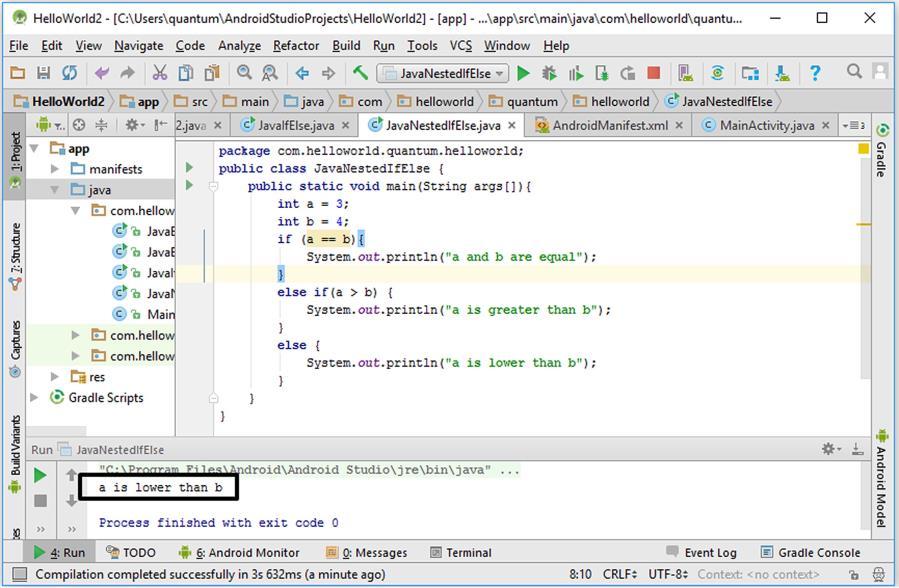
Figure 4.20. Nested if–else statements in Java
Nested if–else decision making statements become error-prone as more and more conditions are added. In order to check a lot of conditions easier, switch–case statements are used. Switch–case statements work similar to nested if–else statements but they are easier for checking multiple conditions. A switch–case statement for assessing the grade of a student is shown in Code 4.18.