Blockly ll
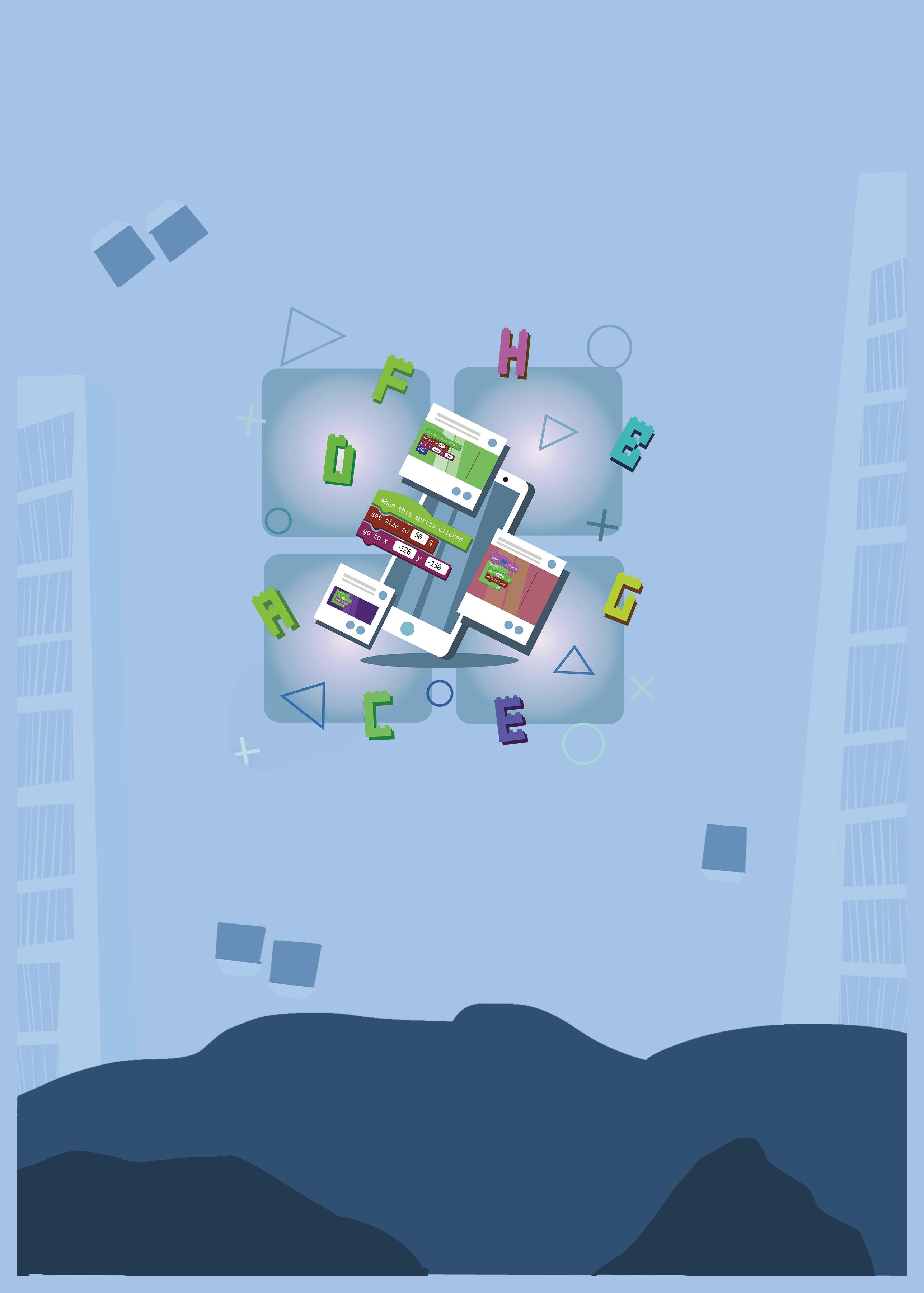
Coding: Coding is how we give instructions to computers. It is also known as computer programming.
Program: A program is a set of instructions we give to the computer to perform specific tasks. Programming is giving instructions to the computer to perform specific tasks.
Block-based Coding: In block-based coding, we use blocks to give instructions to the computer. We will give instructions in Blockly.
Print Block: The print block displays information on the screen.
Output → abc
Output → 2
Here, Blockly adds (1 + 1) and displays 2 in the output.
Text Block: The text block stores words, sentences, characters, symbols, numbers, etc.
The values stored in the text block are stored within quotes (“ ”) and are known as strings.
Printing with a text block displays the text inside the quotes “ ” as it is.
Output → Hello World
Number Block: The number block is used to store only numbers.
Printing with a number block displays the number on the screen.
Output → 123
Operator Block: Operator blocks perform mathematical operations. Printing with the operator block displays the outcome of the mathematical operation.
Output → 5
Operator Blocks
We can use the operator blocks from the ‘Math section’ to perform basic mathematical operations like addition, subtraction, multiplication, division, etc.
Operator blocks are used with the print block to display the output.
1. Addition: The addition operator block gives the sum of two numbers.
Output → 14
2. Subtraction: The subtraction operator block gives the difference between two numbers.
Output → -4
3. Multiplication: The multiplication operator block gives the product of two numbers.
Output → 45
4. Division: The division operator block gives the quotient when a number is divided by another number.
The quotient is the number obtained by dividing one number by another.
Output → 9
5. Remainder: The remainder block gives the remainder when one number is divided by another.
The remainder is the integer that is “left over” after dividing one integer by another to get an integer quotient.
Output → 1
Solved Example 1.1
Print the output of the following operations:
a) 99 + 11
Output → 110
b) 84 - 11
Output →
Output → 370
1. Even block: Even numbers, when divided by 2, give 0 as a remainder.
2, 6, 8, 10, and 12 are examples of even numbers.
The even block gives the output as true if the number is an even number, otherwise, it gives the output as false.
Output → False
2. Odd block: Odd numbers, when divided by 2, give 1 as a remainder.
1, 3, 5, 7, and 9 are examples of odd numbers.
The odd block gives the output as true if the number is odd, otherwise, it gives the output as false.
Output → True
1. Positive block: Positive numbers are numbers that are greater than zero. The positive block gives the output as true if a number is greater than 0, otherwise, it gives the output as false.
Output → True
2. Negative block: Negative numbers are numbers that are less than zero. The negative block gives the output as true if a number is less than 0, otherwise, it gives the output as false.
Output → True
Prime Numbers: A prime number is a number that can only be divided exactly by 1 and itself.
Examples of prime numbers are 2, 3, 5, 7, 11, 13, etc.
The prime block checks whether a number is prime or not. If the number is a prime number it gives the output as true, otherwise, it gives the output as false.
Output → True
Solved Example 1.2
● Check if 45 is an even number or not.
Output → False
● Check if 200-207 is a negative number or not.
Output → True
Introduction to Variables
Variables are like boxes in the computer memory, which hold information in the form of letters, words, numbers, sentences, etc.
Value/Reference
We use the ‘Create variable’ button from the ‘Variable’ section in Blockly to create a new variable and give it a name.
Creating a variable is not a big task, but naming them is. Below are a few guidelines to follow while naming variables.
a. Names of variables should be meaningful.
b. Underscore (_) is the only symbol that can be used while naming variables.
c. Variable names should not start with a number. But you can use a number in a variable name.
d. Variable names can start with an underscore.
e. For variable names having more than one word, we can follow these 3 ways to name them:
i. Camel case or camelCase: Here, the first letter of each new word is capitalised, except the first word.
‘First num’ in Camel case can be written as ‘firstNum’
ii. Snake case or snake_case: Here, each space is replaced by an underscore ( _ ) character, and the first letter of each word is written in lowercase.
‘First num’ in Snake case can be written as ‘first_num’
iii. Pascal case or PascalCase: Pascal case is similar to the camel case - the only difference being that in the pascal case, we also capitalise the first letter of the first word.
‘First num’ in Pascal case can be written as ‘FirstNum’
After creating a variable, we have to assign a value to it. Assigning value to a variable means storing a value in the variable. We use the set block to assign a value to the variable.
The set block is used to assign a value to the variable. This value can be numbers, words, or a combination of numbers and characters.
For example, here the variable is called ‘name’. We are storing the word ‘Alex’ in the variable ‘name’.
Here, the variable is called ‘num’, and we are storing the number 10 in the variable ‘num’.
After we assign a value to a variable, we can also display it on the screen using the print block.
Output → 10
Output → Alex
Note: The value stored in the variable (and not the name of the variable) will be displayed in the output.
The Change block is used to increment or decrement (increase or decrease) the value stored in a variable, by the number mentioned.
This will increase or decrease the value of the variable.
For example, if ‘num’ had the value 10 before using the change block, and you mentioned the number as 2, the new value would be (10 + 2), i.e., 12.
Similarly, you can decrease the value of the variable by mentioning the number as -1 or lower.
We can also verify that by printing the value of the variable.
Output →
There are 2 ways to change or update the variable’s value:
● We can use the change block to update the value of the variable, as we saw above.
● We can also use the operators block and set block to change the value of a variable, like this -
Example 1:
Output → 6
Here, we first set the value of the variable ‘num’ to 5.
→ num = 5
Then, we use the ‘set block’ to update the value by adding 1 to the same variable ‘num’. We set the ‘num’ value to (‘num’ + 1). In this case, Blockly will consider the value stored in ‘num’ and add 1 to it.
→ num = num + 1
num = 5 + 1
num = 6
Note: When we store a numerical value in a variable, we can perform all the mathematical operations on it.
Sam likes to write letters. He wrote 10 letters to his friends, but he discarded 3 of them due to bad handwriting. He also wrote 5 letters to his family last month. Find out how many letters he has in total.
Hint:
● Use different variables to store the letters written, the letters remaining after discarding 3 of them, and the letters written last month.
● Use the operator block to change the number of letters.
Here, we first set the letters written to 10.
→ letters_written = 10
As Sam discarded 3 of his letters, we use the subtraction operator block to find the remaining letters.
→ letters_remaining = letters_written - 3
letters_remaining = 10 - 3
letters_remaining = 7
Then, we set the letters at home to 5.
→ letters_at_home = 5
Now, the total number of letters is calculated by adding the letters remaining and the letters at home.
→ total_letters = letters_remaining + letters_at_home
total_letters = 7 + 5
total_letters = 12
A variable can store different types of values like numbers, letters, sentences, etc. These values are called data.
We classify data into 4 types:
1. Integer - These are whole numbers (non-decimal numbers).
2. Float - These are numbers with decimal values.
3. String - All values stored inside quotes (“ ”) are known as strings. These values can be words, symbols, characters, numbers, or a combination of all these.
4. Boolean - Boolean data type takes only two values - true and false. We can use them to represent conditions and also for cases that can have just two answers - yes (true) or no (false).
Comparison operators are used to compare any two values, either numbers or strings. The values being compared can be placed on either side of the operator to form a Condition.
The output of this comparison is in the form of boolean values, i.e., True or False.
There are 6 comparison operators:
1. Equal to (=) operator gives the output as true when the value in the left block is equal to the value in the right block, otherwise, it gives the output as false.
Output → false
2. Not equal to (≠) operator gives the output as true when the value in the left block is different (not equal to) the value in the right block. Otherwise, it gives the output as false.
Output → true
3. Less than (<) operator gives the output as true when the value in the left block is less than the value in the right block, otherwise, it gives the output as false.
Output → true
4. Less than or equal to (≤) operator gives output as true when the value in the left block is less than OR equals the value in the right block.
Output → true
If the value in the left block is more, it gives a false output.
Output → false
5. Greater than (>) operator gives the output as true when the value in the left block is greater than the value in the right block, otherwise, it gives the output as false
Output → true
6. Greater than or equal to (≥) operator gives output as true when the value in the left block is greater than OR equals the value in the right block.
Output → true
If the value in the left block is less, it gives a false output.
Output → false
Solved Example 1.4
Radha has 7 candies, Anna has 25 candies, and Nikit has 12 candies.
Check if:
a. Radha has an equal number of candies as Anna.
b. Nikit has fewer candies than Anna.
c. Anna has less or equal candies to the candies owned by Radha and Nikit together.
Solution:
Output → false true false
Logical operators are used to compare two or more conditions, which are formed using the comparison operators.
We get a boolean value (True or False) as output after comparing these conditions. There are 3 logical operators:
1. AND Operator: The AND operator gives a true output only if both the conditions are true.
If any one of the conditions is false, the AND operator will give a false output.
Example: The age for participating in the mental maths challenge is 10 to 15 years.
Here, the condition is that the student’s age should be between 10 and 15 years. This means that the age should be -
1. Greater than or equal to 10 AND
2. Less than or equal to 15
The condition being formed will be -
→ Now, suppose Aryan’s age is 12.
We set the value of age to 12.
Now for the conditions,
Condition 1: age ≥ 10, and as 12 ≥ 10, the condition is true.
Condition 2: age ≤ 15, and as 12 ≤ 15, the condition is true
True AND True = True
As both the conditions are true, the AND operator will give the final output as true, and Aryan can participate in the mental maths challenge.
→ Now, suppose Aryan’s friend Sam’s age is 17.
Output → false
Condition 1: age ≥ 10, and as 17 ≥ 10, the condition is true.
Condition 2: age ≤ 15, and as 17 is not less than 15, the condition is false.
True AND False = False
As one of the conditions is not true, the AND operator will give the final output as false, and Sam cannot participate in the mental maths challenge.
2. OR Operator: The OR operator gives a true output when either one or both the conditions are true.
It gives a false output only when both conditions are false. OR Operator
Example: You want to have either milk or juice with your breakfast. So, the condition will be as follows -
1. Milk for breakfast
OR
2. Juice for breakfast
→ Now, suppose your friend got you a chocolate shake for breakfast.
Here, we set the value of the variable ‘breakfast’ to ‘chocolate shake’.
Condition 1: Since chocolate shake ≠ milk, the condition is false.
Condition 2: Since chocolate shake ≠ juice, this condition is also false.
False OR False = False
Now, since both the conditions are false, the OR operator will give the final output as false and you did not get your favourite drink for breakfast.
→ Suppose another friend got you juice for breakfast.
Here, we set the value of the variable ‘breakfast’ to juice.
Condition 1: Since juice ≠ milk, the condition is false.
Condition 2: Since juice = juice, this condition is true.
False OR True = True
As one of the two conditions is true, the OR operator will give the final output as true and you get to enjoy juice with breakfast.
3. NOT Operator: The NOT operator reverses the value of the boolean input. So it changes true to false, and false to true.
Note: You can also use the ‘true’ and ‘false’ blocks from the ‘Logic’ section to set a variable to a True/False value.
The If-else statement helps us make decisions depending on whether a condition is True or False.
If the condition is True, we make a decision and perform certain actions. Otherwise, if the condition is False, we can make another decision and perform another set of actions.
If it is raining outside, then take an umbrella with you. Else, don’t take an umbrella.
To create conditions, we use comparison operators (=, <, >, etc.) or logical operators (AND and OR) that we learned before.
The ‘if do block’ from the ‘Logic’ section is used to make decisions.
The statements inside the if block get executed when the given condition is true .
Example:
Let’s say we will give chocolates only to kids who are below the age of 5. Here, the condition becomes age < 5. Only if this condition is True, we give chocolates.
We can set the age and then make a condition in the if block to check if the age is less than 5.
Then we put a ‘print’ block in the ‘do’ section, which will gets executed only when the condition is true.
Here, we have set the ‘age’ to 4. Since the age is less than 5, the condition becomes true, and the ‘print’ block inside the ‘do’ section gets executed and displays “You get a chocolate” in the output.
The else block is used to perform a certain set of actions when the condition in the if-do block is false.
To add the else block, click on the blue ‘settings’ symbol. Then drag and drop the else block from the left side to the right side, below the if block, like this:
Considering the previous chocolate example, if the age condition is not met, i.e., if age > 5, then we can add the print block in the else block to display a message like this,
Here, we have set the ‘age’ to 6. Since the age is greater than 5, the condition becomes false and the else block prints “You don’t get a chocolate”.
In this way, the if-else statement helps us in making decisions -
● When the condition is True, the code in the if block is executed.
● When the condition is False, the code in the else block is executed.
At the city water park, only children below 8 years of age are allowed to play water games.
Henry’s age is 10 years. Create a condition and check if he can play water games or not.
Here, we have set the ‘age’ to 10. As Henry’s age is greater than 8, the condition is not met, and the code in the else block is executed.
The else if block is like an upgraded version of the if-else block, which can be used when we have to check more than one condition.
To add the else if block, click on the blue ‘settings’ symbol. Then drag and drop the else if block from the left side to the right side, below the if block, like this:
The program first checks the condition in the if block. If the condition is True, the program runs the code in the if block and stops.
● However, if the condition is False, it goes to the else if block and checks another condition.
● The code in the else block runs ONLY if none of the conditions are True.
Note: The else if block should always come in between the if and else block. You can add any number of else if blocks in between if and else.
Example:
Robert High school is conducting an inter-school competition. They have organised 3 games for the day - chess, cricket, and basketball. They have created a program to help students gather at the correct venues.
Here, the game’s name will be entered by the participant.
● If the game is chess, the student is directed to the auditorium.
If the game is not chess, the program moves ahead and checks the else if condition.
● Else if the game is cricket, the student is directed to the stadium.
If the game is not cricket, the program goes ahead and directly executes the else block.
● If the game is neither chess nor cricket, the student is directed to the basketball court.
Solved Example 5.2
In the school assembly, all students are made to stand in different rows depending on their height group.
● Students of the height group 150 cm are made to stand in Row 1.
● Students of the height group 160 cm are made to stand in Row 2.
● All other students are sent to Row 3.
Alisha belongs to height group 160. Create a condition for this and then check in which row Alisha should stand.
Here, we check the following,
● If the student belongs to height group 150, she will stand in Row 1
● Else if the student belongs to height group 160, she will stand in Row 2
● Else, she will go to Row 3
Since Alisha belongs to height grade 160, the if condition is False, but the else-if condition is True, and hence the else-if block prints “Stand in Row 2”.
1. The weekly tests are scheduled every Monday. If today is Tuesday, create a condition to check whether the test will be conducted today or not.
2. In a residential society, the houses are numbered from 1 to 10. Houses numbered from 1 to 5 have 2 rooms, and those from 6 to 10 have 3 rooms each. If Reshma lives in house number 6, use suitable blocks to find the number of rooms her house has.
Try to say this statement fast and aloud 10 times.
A snake sneaks to seek a snack.
Could
As humans, we may find it challenging. However, machines can repeat tasks in the exact same way any number of times.
In coding, we can run a statement multiple times using a loop. Let us say we want to print “hello” ten times. This can be done in two ways:
1. Write the print statement ten times.
2. Write the print statement once and put it in a loop that runs ten times. The second method is more convenient because it means we have to write less code.
Loop - In computer programming, a loop is a set of instructions that are repeatedly carried out until a particular condition is met.
Iteration - Each execution of the commands in a loop is called an iteration. We can also call it the repetition of a process.
Let us take a look at the types of loops we can use in Blockly.
The repeat block runs the code in its body the specified number of times. This block is present under the Loops section.
Syntax
In the repeat part of the block, we can specify the number of times we want the body of the loop to execute. The do part of the block contains the body. The blocks inside the do part repeat the number of times specified in the repeat part.
Let us try and understand the working of the repeat loop with a few examples.
Example 1: Print “I love coding!” 5 times.
Output → I love coding!
I love coding!
I love coding!
I love coding!
I love coding!
Example 2: Print numbers from 1 to 5 using a repeat block.
Explanation:
● Here we use a variable count that is initially set to 1.
● The loop will repeat 5 times.
● The body of the loop prints the value of the count variable and then updates it by 1.
Example 3: Print odd numbers between 1 and 10 using a repeat block.
2. repeat while Block: The repeat while block iterates through one or more blocks in a loop while a condition is met. This block is present under the Loops section.
Syntax
The repeat while part of the block contains the test condition which is checked at the beginning when the loop starts. The do part of the block contains the body of the loop that executes only while the condition is true.
If the test condition is false, the body of the loop will not execute and the loop will be terminated or ended.
Let us try and understand it with the help of an example.
Example 1: Print even numbers between 1 and 10 using a repeat while loop.
Explanation:
● We use a count variable. This is also called the loop variable as it controls the number of times a loop gets executed.
● The test condition in the while part of the block specifies the condition that is tested each time the loop iterates. (Here, the condition is that the value of the count variable should be less than or equal to 10.)
● The body of the loop prints the value of the count variable and also updates it by 2 each time the body executes.
● The loop terminates when the value of the count variable becomes equal to 12 and the condition becomes false.
3. repeat until Block - The repeat while loops repeat their bodies while some condition is true. The repeat until loops are similar except that they repeat their bodies until some condition is true.
To get the repeat until block, go to the Loops component and select the repeat while block. Then, simply click on the while part of the repeat while block, and from the drop-down, select ‘until’.
The condition is somewhat opposite to the condition used in the repeat while loop.
Let us understand the difference between repeat until loop and repeat while loop with the help of an example.
Example 1: Print I love coding! three times using a
a. repeat while loop.
b. repeat until loop.
Syntaxa. Using repeat while loop
Output → I love Coding!
I love Coding!
I love Coding!
b. Using repeat until loop
Output → I love Coding!
I love Coding!
I love Coding!
Explanation:
In the repeat while block, the loop executes while the condition or the value of count is less than or becomes equal to 3.
While, in the repeat until block, the loop executes until the value of count becomes greater than 3.
The output differs when we change while to until without changing the condition in the repeat block! This is because the meaning of the statement changes.
Example 2: Print the multiples of 5 until 30.
break out Block - Most loops run until the loop condition becomes false. But we can also control the loop using a break out block. This block can be used with any type of loop. The break out of loop block provides an early exit from a loop.
Syntax
The break block terminates a loop.
While in a loop, when the program execution reaches the break loop, the loop is terminated and program execution continues with the block that follows.
To use this block, we just add it to a loop in our program.
Output → 1 2
Outside Loop 3
1. We set a count variable to 1. This is also called a loop variable.
2. The condition checks if the count value is less than or equal to 5.
3. If the condition is true, that is count <=5, it executes the do part of the block.
4. Inside the body of the loop, the value of the count variable is printed.
5. Next, the count variable is updated by 1.
6. The if statement checks if the count value becomes equal to 3. If yes, the loop terminates or ends and the control comes out of the while loop else the control goes back to step 2.
7. The loop will terminate or end when the value of the count variable becomes equal to 3.
8. The print statements outside the loop are executed.
Solved Example 1.1
Print your name 3 times using a repeat loop.
Output → NAME NAME NAME
Solved Example 1.2
Print the numbers from 1 to 5 using a repeat while loop.
Print the sum of the first 5 numbers. Use a repeat while loop.
Create a timer that starts from 10 and decreases to 1. The program should print Time Out! when the timer becomes 0. Use a repeat loop.
1. Print the multiples of 3 between 3 and 30.
2. Print a positive message 3 times.
3. Print all the numbers from 100 to 90.
Have you ever thought why does your favourite cake always taste the same, every time you buy it?
This is because your favourite baker uses/might be using the same recipe. To produce the same flavour, he uses the same ingredients in exactly the same amounts and follows the same steps.
Why do you perform your day-to-day tasks exactly the same way each time?
Why do you always tie your shoes the same way?
This is because you have conditioned your brain to work exactly the same way each time.
In programming too, we often use the same code more than once to repeat actions to execute complex tasks. Functions allow us to teach the computer how to do something once and reuse the same code later.
Functions: A function is a sequence of commands that can be reused together later in a program. They can also hold other functions and various elements of code, such as variables, conditions, and loops.
● Functions are written once and used many times, allowing us to reuse the same pieces of code.
● Functions help us break large codes into smaller chunks.
● Functions allow for the separation of codes, so we can easily find bugs in a program.
Creating a function consists of two parts.
1. Declaration
2. Definition
The function block looks something like this.
Here, the do something part of the block specifies the name of the function.
Declare - Giving the function a name is termed as declaring the function.
Define - The body of the functions contains the steps or instructions. Adding instructions inside the body of the function is termed as defining a function.
Let us try to imagine what a function looks like.
Suppose we want to prepare a pancake for breakfast. In any recipe, there are a few steps that need to be followed in a particular sequence. Let us consider the following steps for making a pancake.
Now, if we create a function to bake a pancake, it will look something like the one shown below.
You can follow the same steps to make a pancake for yourself!
Example 1: Let us create a function to add two numbers.
This looks quite simple. Now, whenever you want to add two numbers, you can use the function called SUM.
● Let us create our first function in Blockly. This will just print a message on the screen.
NOTE: This function will not produce any output if you try to run it!
We already know that a function is a piece of code that is written once and used many times. However, just declaring and defining the function is not sufficient.
This is the same, as if you do not order a cake, you will not get it! To eat a cake made by your favourite baker, you need to order it. In the same way, to use a function, we need to call it!
We call a function when we want to use it.
Let us call the PRINT function.
To do that, just drag and drop the PRINT block that we can find under the Functions component.
Run the code and we will get the output this time!
Output → I am learning functions!
Example 1: Create a function Sum that prints the sum of two numbers.
In Blockly, functions can be of four types.
Let us try and understand how inputs can be taken in a function.
In the cake-making example, can you guess which ingredients we need?
We will need ingredients like flour, eggs, milk, sweeteners, and so on. All these ingredients can also be called arguments.
Let us look at the SUM function. We need two numbers (A and B) to add them and store the result in a third variable Sum.
The numbers A and B are called inputs or arguments.
Input - The values a function requires to execute successfully are called arguments.
In the previous example, we initialised the variables and called the function. But arguments are values given directly to the function.
Let us try and create a function that accepts an input while calling the function in Blockly.
Example 1: Create a function that adds two numbers in Blockly.
1. Select the to do something block. Rename it to SUM.
2. Click on the gear (looks like a settings icon) icon on the top left of the block. A pop-up appears.
3. Drag and drop the input name x block and place it in the body of the inputs on the right-hand side of the pop-up.
4. Insert two input name x blocks and rename them to input name A and input name B as shown (these are the inputs of the function).
5. Click on the Functions component and drag the SUM with block that appears in the list.
6. Add the values to A and B (here we have added 12 and 31, respectively). These are the inputs or arguments for the function.
7. Under the Functions component, drag and drop the SUM with: A, B block.
8. Add the body of the function.
● Set the Sum to A+B
● Print the Sum
9. Run the code and get the output. This is what the entire code looks like.
Example 2: Create a function called Check. Print a message “You can vote!” if the input age is greater than 18.
Try it yourself - Create a function called MULTIPLY. It should have three arguments. The function should print the multiplication of 3 numbers.
The next two types of functions are the ones that calculate the output and return it to the calling function or a part of the program. Let us try and understand this with an example.
Suppose your teacher gave you homework and asked you to bring it to school the next day. You go home and complete the work and submit it the following day! You were assigned a specific task in this case, and after doing it, you returned the assignment to your teacher.
In coding, when a function is called to carry out a task, we might also ask it to return the result to the place from where it was called.
In the SUM function, we may want to add two numbers only to use the Sum to calculate the average of the numbers at a later stage. Here, we will not simply print the value of the Sum as we did in the previous example.
Let us see how the return statement works.
Example 1: Calculate the sum of two numbers and print it from where the function is called.
● The SUM function calculates a result and returns the value of the Sum.
● The input values of A and B are taken outside the function call as this function is one that does not have inputs.
● The SUM function is called where it returns the value of the calculated Sum and the print function prints the value that it gets.
Example 2: Calculate the average of 2 numbers. Create a function Sum that adds 2 numbers and returns the sum to the calling function.
● The SUM function has 2 inputs A and B.
● The SUM function returns the value of the calculated Sum.
● The AVERAGE function calculates the Avg of 2 numbers. It uses the value of the sum of 2 numbers that will be returned from the SUM functions.
● After calculating the Avg, it prints it.
● The AVERAGE function is called to execute it.
Output → 13.5
1. Create a function without inputs, that returns the perimeter of a square.
Hint: The perimeter of a square is 4 × side
Output → 20
2. Create a function with inputs that returns the sum of the sides of a triangle.
Output → 22
3. Create a function with inputs that returns the result. The name of the function is RADIUS. This function calculates the radius of the circle taking diameter as the input.
Hint: Radius = Diameter/2
Output → 22.5
1. Create a function named SUB that calculates the subtraction of 2 numbers and returns the result. It should take 2 numbers as input from the user.
2. Create a function called PRINT that prints the numbers between 2 numbers.
The 2 numbers should be given as input from the user.
Hint: The function does not return anything.
3. Create a function that checks if a number is positive or negative.
Let us begin with revisiting the basics of understanding a variable. Variables are containers that store values. So far, we have studied variables that take numeric values. Sometimes, these values can be sequences of letters, numbers, or special characters and they are called strings.
Strings - All values put inside double quotes (“ ”) or single quotes (‘ ’) in the text block are known as strings. These values can be letters, numbers, special characters, or a combination of these.
Syntax
The text block looks like this.
We can store numbers or characters in a text block.
We can use a print block along with the text block to print a string value.
Output → Hello
We can also store string values inside a variable.
Output → Angelina
The length of a string is the number of characters in a string. For example, the word ‘BLOCKLY’ has a length of 7 characters.
And the phrase ‘HI, THERE!’ has a length of 10 characters.
Note: Spaces and special characters are also counted.
We can use the ‘length of’ block to find the number of characters in a string.
Output → 8
A string is empty when it does not hold any value.
We use the ‘is empty’ block to check whether a string value is empty or not. It gives the output in boolean values, i.e., true or false.
Output → False
The output is false as the variable ‘Name’ holds the string value “Angelina”.
Output → True
The output is true as the variable ‘Name’ does not hold any value.
Input is the information that the human/user gives to the computer. We can ask users to enter any information, like their name, age, address, etc.
Note: You can take both words and numbers as input.
We can use the prompt block to take inputs from the user.
The message entered in the text block will be displayed to the user while entering the input. This will tell them what is to be entered in the input box, for example, their age, name, etc.
a. Text Input using the prompt block:
Output
Here, we are asking the user to enter their name. The name entered will be stored in the variable ‘Name’ and then printed in the output.
b. Number Input using Prompt block:
Here, we are asking the user to enter their age. The age entered will be stored in the variable ‘age’ and then printed in the output.
Note: You can also take inputs from the user and print them directly, like this:
The ‘Create text with’ block helps us combine multiple blocks of strings in one string, like this -
We need to enter the name when prompted. Once we do that, the output is shown as -
Output → Hello my name is Angel.
We can also add more blocks of strings by clicking on the blue symbol and adding the item block from the left to the join block on the right.
Substrings - A substring is a part of the entire string.
The ‘get substring from’ block is used to get a substring from a string by specifying the start and end positions of the substring required.
Output → Today is a cloudy day.
Here, we want the substring from the first letter (T) to the character at position 22 (full stop).
Output → I like sunny days.
Here, we want the substring from the character at position 24 (I) to the last character of the string (full stop).
1. Prompt a user to enter their name and age. Print the combined string as the output - ‘Hello!NameAge’.
Example: If the input name is Angel and the age is 12.
Output → Hello!Angel12
2. Prompt the user to input 3 numbers and print the sum of the numbers.
Output → Enter A 12 Enter B 6 Enter C 3
The Sum is 21
3. Prompt a user to take age as input and print if they are eligible to vote or not.
Hint: If age > 18, print - "You are eligible to vote!". Otherwise, print - "You are not eligible to vote!".
You are eligible to vote!
1. Prompt users to input 2 numbers and print their product with an appropriate message.
2. Prompt the user to input a sentence and print a substring between 2 positions.
3. Prompt the user with a name and get the letter at position 2.
Have you ever created a list before going shopping for groceries?
Or have you created a list of items you have to take with you to school the next day?
In Blockly, a list is an ordered collection of items, such as a “to do” list or a shopping list. Items in a list may be of any type, and the same value may appear more than once in a list.
Let us see the various ways we can create a list in Blockly.
The simplest list is the empty list, which is created with the create empty list block.
Syntax:
Let us create a list with some initial values.
● The create list with block allows one to specify the initial values in a new list. In this example, a list of three numbers is created and placed in a variable named Numbers.
● This creates a list of three colours. The values in a list are called items.
To change the number of items, click on the gear icon. This opens a new window. You can then drag item sub blocks from the left side of the window into the list block on the right side to add new items.
The value of an is empty block is true if its input is the empty list and false if it is anything else (including a non-list). The value of the following blocks would be false because the variable Colours is not empty, it has three items.
Output → false
Note: This block is similar to the is empty block under the Text component.
The value of the length of the block is the number of elements in the list used as an input. For example, the value of the following blocks would be 3 because Colours has three items.
Output → 3
Note: This block is similar to the one used under the Text component.
Consider, the Colours list -
The following blocks find the position of an item in a list.
1. in list find first occurrence of item
Output → 1
The output is 1 because the first appearance of “Red” is at the beginning of the Colours list at the first(1) position.
2. in list find last occurrence of item
Output → 3
The output is 3 because the last appearance of “Red” in Colours is at position 3.
Note: If the item is nowhere in the list, the output is 0.
Example:
Output → 0
Getting a Single Item
Consider a list -
The following blocks get items from a list
1. in list get #
Output → Blue
Blue is present at the third position of the list.
Output → Yellow
The output is Yellow because it is the third element (position 3) counting from the end of the list.
3. in list get first
Output → Red
We get the first list item of the list as the output.
4. in list get last
Output → Green
We get the last list item in the list as output.
This randomly selects an item from the list, so the output can be Red, Yellow, Blue, or Green. The output can be different every time you run the code.
A dropdown menu on the in list get block changes it to in list get and remove, which provides the same output as in list get along with modifying the original list:
Example:
Output → Red Yellow, Blue, Green
Red is fetched and removed from the list and the new Colours list will be Yellow, Blue, Green.
Selecting “remove” in the dropdown causes the plug on the left-hand side of the block to disappear.
Let us observe in the block image that follows.
Output → 6, 8, 10
This removes the first item, that is 4, from the Numbers list.
The in list get sublist block is similar to the in list get block except that it extracts a sublist, rather than a single item.
Sublist - It is a part of the larger list.
Syntax - We can change the from and to part of the in list get sub-list block.
Example: A list called Numbers is created [2,4,6,8,10].
Then, we set a SubList that contains part of the elements of the Numbers list. The part extracted from the larger list (Numbers) is from position 2 to 4, i.e., items of SubList are 4,6,8.
Output → 4,6,8
We notice that this block does not modify the original list(Numbers).
In List Set Block
The in list set block replaces the item at a specified location in a list with a different item.
Example: We create a list called Numbers with list items as [2,4,6,8,10].
The in list set block sets the 2nd position to 12. Now, when the Numbers list is printed, we see that 4 is replaced with 12.
Output → 2, 12,6,8,10 in list insert at Block
The in list insert at block is obtained by using the dropdown menu on the in list set block.
Syntax-
It inserts a new item into the list at the specified location, before the item previously at that location.
Example: This is the same example as in the previous example. We just change the set to insert at. The difference here is that 12 will be inserted at position 2 before 4. The new list will have a total of 6 elements.
Output → 2,12,4,6,8,10
The for-each block in the Loops component performs an operation on every item in a list. For example, these blocks individually print each item in the list.
Example:
Output
1. Prompt the user to input 3 names (text input) in a list called Name. Print the Name list.
The prompt will ask to give input
Input → Tia, John, Jenn
Output → Tia, John, Jenn
2. Create a list Colours which has three colour names as list items. Check if the list is empty or not. Also, print the list.
Output → false
Red, Yellow, Blue
3. Create a list called Numbers. The list has names of single digit numbers from one to six. The number four is missing from the list.
Add “four” at the correct position. Print the entire list after updating it.
Output → one, two, three, four, five, six
4. Create a list with any number names. Print the number at position 4 in your list.
Output → ten
1. Create a list of 5 items. Extract a sublist of the list from the 2nd position to the 4th position. Print the list as well as the sublist.
2. Create a list of 5 names. Extract the name at the last position and print it.
3. Create a list that contains a few grocery items. Check if it is empty or not. Also, print the length of the list.
To get started with Blockly games, type the given URL in Google Chrome. https://blockly.games/
You get a screen like the one shown below. The first game is a puzzle.
Steps to complete the puzzle
1. Click on the Puzzle icon on the first page.
2. As soon as you enter the puzzle, you get a pop-up message that contains instructions on how to complete it.
3. There is a block with the name of the animal. All you need to do is to match and attach the picture, choose the correct number of legs for that animal, and also add traits to it.
7. Observe the number of legs and traits associated with each animal.
8. Once done, click on the Check Answers button on the top right corner of the screen.
9. You get a message as shown.
10. Click OK to proceed to the next game.
In the Maze game in Blockly, a few blocks are given and a maze path is shown on the left pane.
Instructions for completing Stage 1 are given.
1. Drag and drop the move forward block two times as illustrated in the pop-up message.
3. The path is correct as the maze is a straight one. We get a Congratulations message as shown.
1. Stage 2 has a path with turns. Analyse how to move in order to reach the red balloon (destination).
2. You move forward, turn left, move forward, turn right and then move forward to reach the destination. Arrange the blocks in the same order.
3. Once you arrange the blocks, click on Run Program. You get a Congratulations pop-up message.
4. Click on OK to proceed to the next stage.
1. In this stage, there is a limit to the number of blocks you can use.
2. Use the loop block.
3. Add the block and click Run Program. You get the Congratulations popup message.
4. Click on OK to proceed to the next stage.
1. In this stage, you can use only 4 blocks.
2. Add the blocks inside the loop block. Analyse the pattern and add 4 blocks.
4. Click on OK to proceed to the next stage.
1. In this stage, you have 4 blocks. Here, you have a choice to change the player. You can do that if you click on the icon in the top right-hand corner of the screen.
2. Choosing a new player will change the background as well. Analyse the blocks and drag and drop the blocks.
3. Click on Run Program and a Congratulations pop-up message will appear.
Practice Questions
Complete the stages 6-10.
Turtle
In a maze, You need to find a path that will lead to the destination or goal. In this game, the player needs to reach the destination by following the correct path using the blocks provided.
Look at stage 1 to get clarity on how to proceed with the game.
Stage 1
1. This stage gives instructions on how to proceed with the blocks.
4. Click on OK to proceed to the next stage.4. Adjust the speed using the slider.
5. Click on Run Program and a Congratulations message will appear.
6. Click on OK to proceed to the next stage.
1. The next stage is to create a pentagon.
2. Analyse the blocks and change the blocks as per requirement.
3. A pentagon has 5 sides so we need to repeat the steps 5 times. And the turn angle also changes to 72.
We choose 72 because each angle in a pentagon measures 72 degrees. For now, let us just keep that in mind.
4. Click on Run Program and a Congratulations message will appear.
5. Click on OK to proceed to the next stage.
1. The shape of the stage is a star. A new block for colour is also added in this stage. The instructions for its use are shown in a pop-up message.
2. A Colour menu is also added in the blocks.
3. Analyse the angle and the number of times you need to run a set of blocks.
4. Choose the Set Colour to block. From the drop-down select yellow, to create a yellow star.
5. Add the blocks to create the shape of a star.
As a star has 5 sides, repeat the steps 5 times. We can try different angles here to see which angle makes the correct shapes that we want.
Note: The correct angle is 144.
6. Click on Run Program and a Congratulations message will appear. The yellow star is created.
7. Click on OK to proceed to the next stage.
1. In this stage, there is a new block added. The instructions are shown as a pop-up message.
2. Analyse the star carefully. The size of the star has decreased. The rest of the blocks remain the same. Hence, decrease the value in the move forward by block to 50.
3. The first part to create a star is done. To draw a line that is just above the star use the new Pen Up block.
4. Try to draw the line. Try out multiple options to put as the angle and see the results. If you use Pen Up you will need Pen Down to start drawing again.
5. Click on Run Program to see the result. A Congratulations message appears when the stage is passed as expected. Else, reset and try again.
6. The final blocks that create the shapes in the stage are as follows.
Note: This is not the only solution. You can use a repeat block to move forward while the Pen is Up.
7. Click on OK to proceed to the next stage.
2. You already know the steps to draw a star. There are a few important things to observe.
● The stars are arranged in a square. So, you can use turn right 90 to get to another star.
● You need to draw 4 stars so you can use a loop to do it.
● Use a loop inside a loop. Loop 1 is for the number of stars (4 stars) to be created and Loop 2 draws the star (5 lines of the star). The block of code will look something like this.
3. Click on the Run Program button. You will get a Congratulations pop-up message.
Complete stages 6 and 7.
About this book
This coding book is supplementary to the main “Mel n Conji” content book. This book represents a 21st-century approach to learning coding concepts and developing computational thinking and problem-solving skills. To prepare students for the digital age, the curriculum is interwoven with well-thought-out concept graduation with real-life examples and practice problems.
• Illustrative approach: Concepts in coding and computer science are delivered through pictorial representations and learner-friendly approaches.
• Learning through real-life examples: Age-appropriate examples that enable learners to relate to the concept and learn about the unknown from the known.
• Extensive practice: Multiple practice scenarios to reinforce learnings.
• Coding Challenges: Includes projects through which learners can demonstrate their learning outcomes in coding and computer science.
About Uolo Explore more
UOLO partners with K12 schools to bring technology-based learning programs. We believe pedagogy and technology must come together to deliver scalable learning experiences that generate measurable outcomes. UOLO is trusted by over 8,000 schools with more than 3 million learners across India, South East Asia, and the Middle East.
hello@uolo.com