Python ll
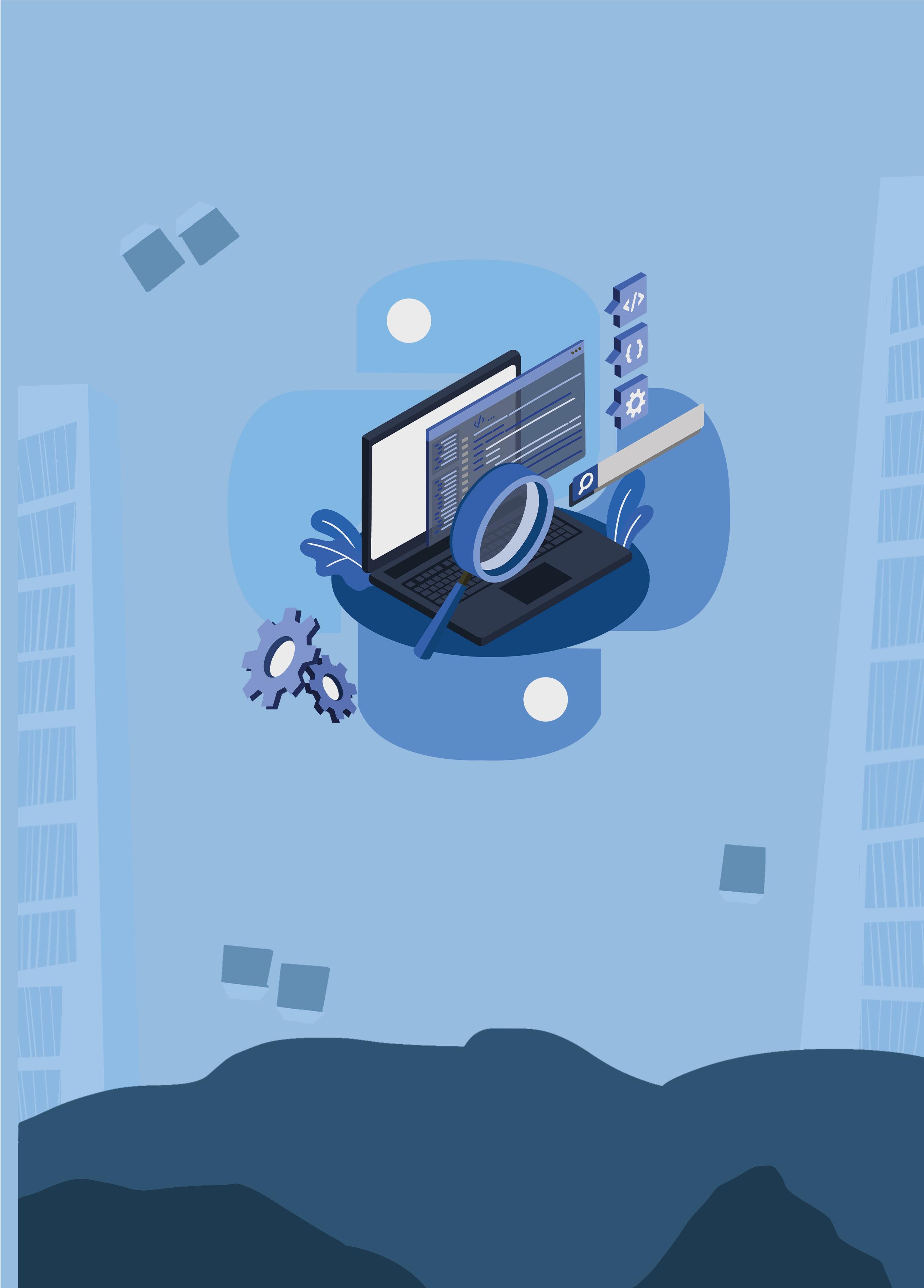
Binary Language
Introduction to Python
Variables
Comments
Assignment Operator
Data Types
Mathematical Tools in Python
Arithmetic Operators
Strings
Strings Concatenation and Repetition Input and
String
Computers are incredible and powerful machines. Today, they are everywhere, be it in a shop, a bank, a school, government offices, or malls. But they don’t do anything without our instructions. They are helpful, but deep down, they are not smart. The instructions we give to a computer are sets of commands to perform a task, which is nothing but programs.
We need a language to instruct computers to carry out a task, just as we use English to communicate with one another. Since computers are machines, they can only understand machine language. As machines are electrically powered devices, they listen to two signals: on and off, which are represented by 0s and 1s, respectively.
The language that is formed with 0s and 1s is called binary language, as it builds up on two digits. Binary language is also known as low level language.
A programming language is a language which has its own vocabulary and syntax just like we have in English, Hindi and other languages.
Programming is the process of creating a set of instructions that tells a computer how to perform a task. A task is a piece of work to be done. In a program, it may represent a section of the program.
Programming Languages are classified into two categories:
● Low-level languages
● High-level languages
Machine-friendly language is called low-level language (LLL). Low-level languages include machine and assembly languages. They communicate with the computer directly. As a result, no language translation is required.
A high-level language (HLL) is a human-friendly programming language like Java, Python, C, etc. It is intended to make computer programming simpler for programmers, as it is not feasible for programmers to remember the 0 and 1 codes for everything. Later, these high-level language (HLL) programs are translated into low-level language (LLL) programs to allow computers to understand them.
The high level language we are going to learn now is Python.
Guido van Rossum developed Python at the National Research Institute for Mathematics and Computer Science in the Netherlands in the 1990s.
Python is a high-level, interpreted, interactive, object-oriented programming language. It is the easiest of the existing programming languages and super reliable.
Python is not named after a real Python, but rather after Guido’s favourite comedy show, Monty Python’s Flying Circus.
1. Easy to Read: Python code is easy to read, just like English.
2. Quick to Learn: Python has few keywords, a simple structure, and a clearly defined syntax. This makes learning Python easy and quick.
3. Easy to Maintain: Python’s source code is fairly easy to maintain.
4. Interactive Execution Mode: Python offers script and interactive modes for running programmes. The code can be interactively tested and debugged in the interactive mode.
A three-step loop process defines how Python is run in the interactive window:
● The Python interpreter first reads the code entered at the prompt.
● Then it evaluates the code.
● Finally, it prints the outcome and awaits new input. The acronym REPL stands for read-evaluate-print loop.
5. Dynamic Typing: Python defines data type dynamically for the objects according to the value assigned. Also, it supports dynamic data type checking.
6. Portable: Python is a portable language because it can be used on a variety of hardware platforms.
7. Rich Standard Library: Python has a rich, portable and cross-platform standard library.
8. Large Database Support: Python provides interfaces to all major commercial databases.
9. GUI Application Creation: Python also supports the creation of GUI applications.
10. Scalable: Python provides better structure and support for larger programs than shell scripting. This makes it scalable.
The Python interpreter runs the code line by line and displays the results until an error occurs. It changes the HLL-written source code into bytecode, an intermediate language, which is then converted back into machine language and executed.
Every language has its own set of rules for writing. All programming languages also have rules for writing programs in them. These rules are called syntaxes.
Python also has some rules that we need to follow while writing a program.
One of Python’s rules is that it is a case-sensitive language, which means that num, Num, and nUm all refer to different things.
Syntax errors are mistakes in following the rules to write the code, such as wrong function name, missing quotes, brackets, etc.
Below are two examples of common syntax errors:
1. Missed double quotes
2. Missed symbols, such as a colon, comma, or brackets CODE
print(Learn Python SyntaxError: invalid syntax
The print() statement displays a value or information in the output.
Syntax: print("value") CODE
print("Hello kitty!")Hello kitty!
CODEOUTPUT print(25)25
We can also print multiple elements in a single print statement by separating them with a comma. While printing them, each element in the output is separated by white spaces.
Syntax: print("box1","box2")
print("Hello","everyone!") Hello everyone!
print("You","are","welcome!") You are welcome!
The sep and end are optional parameters in the print function in Python. By default, the print function has whitespace as a separator between words and ends with a newline.
With sep parameter you can replace whitespace with your choice of separator to separate words and with end parameter you can add a symbol or value at the end of the statement.
Syntax: print("box1", "box2", sep= "symbol")
CODE
print("I am fine","well","alright","tired", sep = "/") print("What are you reading", end="?")
Write a program to print the following:
180-455-2020
1*2*3*4*5*6*7 =7! 10*20*30=6000
Answer: CODE
1. print('180','455','2020',sep='-')
2. print(1,2,3,4,5,6,7,sep='*',end='=7!')
3. print(10,20,30,sep='*',end=' = ') print(10*20*30)
I am fine/well/alright/ tired
What are you reading?
A variable is a memory location on a computer which can hold any value and can also change over time as needed.
To understand it in an easy way, think of it like a box in which you can store a number and later you can replace that number with another number.
In simple words, variables are names given to the memory location where your value is stored.
The figure below illustrates three variables with their respective values. The three variables used in the figure are “name”, “winner” and “score”. The variable “name” holds the value “Bob”, the variable “winner” holds the value “True”, and the variable “score” holds the value “35”.
Therefore, whenever the variable “name” is used in the program, it refers directly to its value, “Bob”.
In Python, variable declaration and initialisation happens with a single step of assigning a value to a variable.
Syntax: variable_name = value
We can also initialise multiple variables in one line.
Syntax: var1, var2 = value1, value2
1. word, number = "hi", 5
2. print(word,number) hi 5
NOTE: If we change the value of a variable and use it again, the old value is replaced with the new value.
There are certain rules we need to follow while naming a variable. Some rules are:
1. The name of a variable should be meaningful.
2. Only the underscore ( _ ) symbol can be used while naming variables.
3. A variable name must start with a letter or the underscore character.
1. greeting_message = "Good Evening!"
2. _number_ = 205
3. print(greeting_message)
1. greeting%message = "Good day!"
2. print(greeting%message)
Good Evening! 205
SyntaxError: cannot assign to operator
4. As Python is a case-sensitive language, variable names are also casesensitive.
Example: old, Old and OLD are three different variables.
CODEOUTPUT
1. num = 25
2. Num = 30
3. NUM = 45
4. print(num)
5. print(Num)
25 30 45 8 Python II
5. A variable name can have numbers within but cannot begin with a number.
As we have case styles for words in English, we have some case styles for naming variables. Below are the case styles you can follow to name variables in Python:
A variable name can be written in camel case if it contains two or more distinct words. In camel case, we write the name of the variable as the first word in lowercase and all subsequent words with the first letter capitalised without a space in between.
Example: “Name of the company” can be written as “nameOfTheCompany”.
As this represents the camel’s hump we call it Camel-Case.
First word lowercase Capitalise All Following Words
Snake-case is the practice of writing in which each space is replaced by an underscore ( _ ) character and the first letter of each word written in lowercase.
Example: “Name of the company” in snake-case is written as “name_of_ the_company”.
It is always a good practice to document your work. In programming, we also document our projects to keep them organised and easily understandable by other programmers.
We use comments to add documentation to a Python program. Python ignores these lines of comments in the program during execution as they are not part of the code.
Comments help:
● Structure the code and make it easier for humans to read.
● Explain the thought processes and intentions behind the code.
● Find errors and debug the code.
● Reuse the code in other applications as well.
After all, there is a popular saying, “Anyone can write code that a computer can understand. Good programmers write code that humans can understand.”
In Python, we have two types of comments:
1. Single-line Comment
2. Multi-line Comment
A single-line comment starts with a hash character ( # ) and is followed by related information.
1. #using the print command
2. print("Learn Programming")
3.
4. greeting = "Learn" #defining the greeting
5. name = "Python" #defining the name
6. print(greeting, name)
Multi-Line Comment
Learn Programming Learn Python
A multi-line comment begins and ends with three single or double-quote characters (‘ ‘ ‘ or “ “ ‘) with related information enclosed.
1. '''
2. This is an example of
3. Multiline comments
4. '''
5. """
6. Printing hello world with sep command
7. Printing hello world with end command
8. """
9. print("learn", "coding", sep = "#")
Solved Examples
Example 2.1
learn#coding
11 Chapter 1 • Introduction to Programming
Answer:
side = 80 areaOfSquare=side*side print("The area is: ", areaOfSquare)
The area is: 6400
Below are the average temperatures of different places and store them in different variables. The temperature has increased by 0.5 in the afternoon and displays the updated temperature of all the places in the same format shown below.
Place Temperature Zo Hills 20
Met Town 25 Bay Area 26
Answer: CODE
Zo_Hills=20 Met_Town=25 Bay_Area=26
print("Place","Temperature") print("Zo Hills", Zo_Hills+1) print("Met_Town",Met_Town+1) print("Bay_Area",Bay_Area+1)
The ‘=’ operator is known as assignment operator, also read as ‘is set to’. It assigns the value on the right-hand side of the “=” symbol to the variable on the left-hand side.
There is a difference between the “=” we use in Maths and the one we use in Python.
In mathematics, = means to equate the left-hand side with the right-hand side. It is the sign of equality.
In python, = symbol is used to set the value of the left-hand side variable to the right-hand side variable.
Syntax: variable = value
# the value is stored in the variable variable = variable + value
# value is added to existing variable value and stored in the variable
A line of the program that tells Python to assign a value to a variable is called an assignment statement. The variable on the left of “=” holds/stores the value on the right.
Syntax: variable_name=value
1. name="Rashi"
2. roll_no=28
3. print(name)
Rashi 28
Example 3.1
Classify the following into either Animal or Tree, and then print all the Animal variables in the first line and Plant variables in the second line.
“Cat, Banyan, Monkey, Lion, Teak, Neem”
OUTPUT
Animals - Cat, Monkey, Lion
Trees - Banyan, Teak, Neem
Answer: CODE
animal_1,animal_2,animal_3 = "Cat","Monkey","Lion" tree_1,tree_2,tree_3="Banyan","Teak","Neem" print("Animals -", animal_1,animal_2,animal_3) print("Trees -", tree_1,tree_2,tree_3)
Example 3.2
A fruit shop sells 32 apples, 33 bananas, 20 strawberries, and 15 oranges. Print the percentage of fruit sold that were apples or oranges.
OUTPUT
Total Fruit sold =100 47% of fruit sold were apples or oranges.
Hint: Store the number of fruit sold in variables. Print the total number of fruit sold.
Find the percentage using the formula (apples+oranges)/total*100.
Answer: CODE
apple=32
banana=33
strawberry=20
orange=15
total_sold=apple+banana+strawberry+orange percent_apple_orange_sold=apple+orange/total_sold*100 print('Total Fruit sold =',total_sold)
Data types define what type of data a variable, a named memory location, can hold.
The default data types in Python are as follows:
Integer (int) Stores non-decimal numbers in a variable. 5, -8, 43, 54685, -5612965
Float (float) Stores decimal point numbers in a variable. 6.8, -3.2
String (str) Stores words and characters in a variable. “Hello”, ”World”
Boolean (bool) Stores two values: True and False in a variable True and False
The type() function returns the data type of the value held by the variable.
Syntax: type(variable_name)
CODE
number_ = 5
word_ = "Hi"
print(type(number_))
print(type(word_))
OUTPUT
<class 'int'>
<class 'str'>
Type casting refers to converting data type of a variable into another.
The int() function is used to typecast the value of a variable into integer. It converts the following data type into integer:
1. Float by removing the decimal point and everything after it.
2. String only if string represents a number.
3. Boolean by converting True to 1 and False to 0.
Syntax: int(variable_name)
1. str_to_int = int("8")
2. bool_to_int = int(True)
3. print(str_to_int, type(str_to_int))
4. print(bool_to_int, type(bool_to_int))
8 <class 'int'>
1 <class 'int'>
1. number=int("Python")ValueError: invalid literal for int() with base 10: 'Python'
The float() function is used to typecast the value of a variable into float. It converts the following data type into float:
1. Integer by adding a decimal followed by a zero.
2. String only if the string represents a float or an integer.
Syntax: float(variable_name)
1. num=float(65)
2. flat_no=float("101")
3. print(num)
4. print(flat_no)
1. str=float("Learn")
2. print(str)
ValueError: could not convert string to float: 'Learn'
The str() function is used to typecast the value of a variable into a string. It converts all the data types including float, integer, boolean into a string.
Syntax: str(variable_name) CODE
1. num1 = str(25)
2. num2 = str(17.2)
3. print(num1 , type(num1))
4. print(num2, type(num2))
25 <class 'str'>
17.2 <class 'str'>
The bool() function is used to typecast the value of a variable into boolean. It converts the following data type into boolean:
1. Integer and prints False if the value of the variable is 0, otherwise prints True.
2. String and prints False if the string is empty, otherwise prints True.
Syntax: bool(variable_name) CODE
1. a=bool(0)
2. b=bool(1)
3. c=bool("")
4. d=bool("Programming")
False True False True
Strings are written by enclosing a set of characters within a pair of single or double quotes.
Strings are a series of characters that form words and sentences.
Example 4.1
A boolean variable can take only one of the two boolean values represented by the words True or False without quotes.
Booleans are either 0 or 1. They are used to categorise something in either True or False, which also implies a Yes or a No.
Make 2 variables, one to store the city name and the other for the pincode. Change the pincode to string to print the city name and pin code in the format given below.
City Name Pincode
Roseville 6584796
Answer: CODE
name="Roseville"
pin=65847961
print('City Name','Pincode')
print(name , str(pin))
The Python Math Library provides common maths functions that can be used for complex mathematical computations. Math library contains functions such as ceil, floor, factorial, etc.
We need to import the math library into our program before we can use the functions.
Here is how we import the library.
Syntax: import math
Here are a few useful mathematical functions used in daily computations.
The ceil comes from the word ceiling. As the name suggests ceil() function rounds UP the number to the nearest integer (i.e., the smallest integer greater than or equal to that number) and returns the value.
Syntax: ceil(value)
As the name suggests, the floor() function rounds a number DOWN to the nearest integer (i.e., the largest integer not greater than the number) and returns the value.
Syntax: floor(value)
1. import math
2. number = 1.666666666666667
3. ceil_ = math.ceil(number)
4. floor_ = math.floor(number)
5. print("Number rounded UP to ceil is:",ceil_)
6. print("Number rounded DOWN to floor is:",floor_)
Number rounded UP to ceil is: 2
Number rounded DOWN to floor is: 1
The round() function returns a float value by rounding off the number to the decimal place that we give as input. The function takes in two values, the number we want to round off and the decimal places we want to round to (i.e., the number of digits after the decimal point).
The default number of decimals is 0, i.e. the function will return the nearest integer.
Syntax: round(float_value,digits_after_decimalpoint)
Python’s Random module is an in-built module of Python which is used to generate random numbers. The random module contains functions like random(), randint() etc.
The random() function generates random floating numbers between 0 and 1.
Syntax: random.random()
The randint() function returns an integer number within the specified range. The specified range from which we want the integer is given as input in the function.
Syntax: random.randint(start,end)
1. import random 2. print(random.randint(3,50))
Example 5.1
Prepare your report card:
Store your name and marks in 5 subjects in different variables, and calculate your percentage of marks.
Now, display the following data as well.
1. Percentage of marks scored in 5 subjects.
2. Round-up the percentage using ceil function.
3. Round-down the percentage using the floor function.
4. Round the percentage to 3 decimal places.
Name of the student: John
Percentage of the student: 79.4%
Percentage after using ceil(): 80%
Percentage after using floor(): 79%
Percentage after using round(): 79.4%
Answer:
1. import math
2. name="John" #you can write any name
3. marks1=94
4. marks2=84
5. marks3=92
6. marks4=58
7. marks5=69
8.
9. percentage=(marks1+marks2+marks3+marks4+marks5)*100/500
10.
11. print("Name of the student:", name)
12. print("Percentage of the student:",percentage,"%")
13. print("Percentage after using ceil():",math.ceil(percentage),"%")
14. print("Percentage after using floor():",math. floor(percentage),"%")
15. print("Percentage after using round():",round(percentage,3),"%")
Arithmetic operators in Python are used along with the integer or float values to perform mathematical operations on them.
There are 7 arithmetic operators in Python:
Whenever there is a mathematical expression in Python, it solves it in the following sequence:
1. Brackets (B)
2. Exponents (E)
3. Multiplication (M)
4. Division (D)
5. Addition (A)
6. Subtraction (S)
Python solves the mathematical expression in the order above from left to right of their occurrence.
But, whenever multiple exponent operators are used in an expression, Python solves the exponent part from right to left
Example:
9 + 7 * (8 - 4) / 2 - 3**2
= 9 + 7 * 4 / 2 - 3**2
(First, the brackets are solved.)
= 9 + 7 * 4 / 2 - 9
= 9 + 28 / 2 - 9
= 9 + 14 - 9
= 23 - 9
(Then, Exponent is solved.)
(Then, multiplication is solved.)
(Then, division is solved.)
(Then, addition is solved.)
= 14 (Finally, subtraction is solved.)
Solved Examples
Example 6.1
As a programmer, write a program to help Dave with the right answers to the questions given below using operators in Python.
a = 23 b = 13
a) Addition of a and b.
b) Multiplication of a and b.
c) Division of a and b.
d) Base a to the power b.
e) Modulus of a/b
Addition of a and b is: 36
Multiplication of a and b is: 299
a divided by b is: 1.7692307692307692
Base a to the power b is: 504036361936467383
Remainder, when a is divided by b, is: 10
Answer: CODE
1. a,b=23,13
2. print("Addition of a and b is:",a+b)
3. print("Multiplication of a and b is:",a*b)
4. print("a divided by b is:",a/b)
5. print("Base a to the power b is:",a**b)
6. print("Remainder, when a is divided by b, is:",a%b)
A string is a data type that represents a sequence of characters. In Python, strings are put inside double quotes (“ ”) or single quotes (‘ ’). These values can be words, symbols, characters, numbers, or a combination of all these.
A string can be categorised into two as follows:
● A single-line string
● A multi-line string
Single-Line String
A single-line string can be enclosed in either single quotes or double quotes.
1. name1="Mohit"
2. name2='Mohit'
3. print(name1)
4. print(name2)
Multi-Line String
Mohit Mohit
A multi-line string can be enclosed in either three single quotes (‘) or three double-quotes (“).
1. proverb_1= '''Don't judge
2. a book
3. by its cover. '''
4.
5. proverb_2= """
6. Beauty is
7. in the eye of
8. the beholder.
9. """
10. print(proverb_1)
11. print(proverb_2)
Don't judge a book by its cover.
Beauty is in the eye of the beholder.
When we have multiple apostrophes in a sentence, python throws an error as it is illegal.
We can avoid this with the help of an escape character. An escape character is a backslash ( \ ) followed by the character that is to be inserted.
An example of an illegal character is a double quote inside a string that is surrounded by double quotes:
print("Let us learn "Python".")SyntaxError: invalid syntax
Thus if there are multiple apostrophes and we want them to be part of the same string we use backslashes before them so that the character is included in the string.
print("Let us learn \"Python\".") Let us learn "Python".
In some cases we might need to break the string into multiple lines.
In order to add a new line, we put \n before the character where we want the string to break, the characters after the \n are printed on the next line onwards. CODE
print("Creative\nMinds") Creative Minds
Example 1.1
Teacher has asked you to write two thoughts for the day. Write a program to print the two sentences given below using ONLY ONE print.
One kind word can change someone's day. No one is perfect. That's why pencils have erasers.
Answer: CODE print("One kind word can change someone\'s day.\nNo one is perfect. That\'s why pencils have erasers.")
Concatenation
Concatenation is the technique of combining two or more strings together. There are different ways to concatenate strings.
The addition operator (+) has a different meaning in the case of strings. The + operator is used to join two or more strings into one single string.
a= "Think"
b = "Different"
c = a + b
print(c)
ThinkDifferent
The join() string function returns a string by joining all the elements of a string.
Syntax: str.join([var1, var2])
var1 = "Hello"
var2 = "World" print("".join([var1, var2]))
The (*) operator duplicates the string and combines them.
If * is used with an integer, it performs multiplication, but if it is used with strings, it performs repetition.
Syntax: repeated_string = string1*no_of_times
Multiplying a string with an integer, repeats the string that many times.
str = "Challenge" print(str*3)
ChallengeChallengeChallenge
Multiplying a string with a float gives an error. We can only repeat strings with integers.
CODE
str = "Coding" print(str*3.5)
Example 2.1
TypeError: can't multiply sequence by non-int of type 'float'
In a game app the name of a player is stored as FirstName and LastName. Now do the following tasks.
1. Create the player by concatenating the FirstName and LastName.
2. Create a temporary password by repeating the username twice.
3. Print a welcome message “Greetings, BayMax” using the join() method.
Username is: BayMax
Temporary password is: BayMaxBayMaxBayMax
Greetings, BayMax
Answer:
first_name = "Bay"
last_name = "Max"
CODE
username = first_name+last_name print("Username is:",username)
temp_password = username*3 print("Temporary password is:",temp_password)
welcome_message = "".join(["Greetings, ",username]) print(welcome_message)
The input() function in Python is used to take and store input from the user.
We can take any kind of information from the users as input. Whatever is entered as input, the input() function converts it to a string.
Syntax: input("message to be shown in input prompt")
CODE
x = input("Enter your name: ") print("Hello, " + x+"!")
OUTPUT
Enter your name: Saansh Hello, Saansh!
The islower() function returns True if all the characters in a string are in lower case, otherwise it returns False.
Syntax: string.islower()
greetings_1 = "welcome to our world!"
check_1 = greetings_1.islower()
print(check_1)
greetings_2 = "Welcome to our world!"
check_2 = greetings_2.islower()
print(check_2)
If a string contains symbols and numbers only, the function returns False.
If symbols and numbers are present along with lowercase characters, it returns True
sentence_1 = "#%@&^"
check_1 = sentence_1.islower()
print(check_1)
sentence_2 = "user@uolo.in"
check_2 = sentence_2.islower()
print(check_2)
The isupper() function returns True if all the characters are in upper case, otherwise it returns False.
Symbols and numbers are ignored by this function as well.
Syntax: string.isupper()
proverb_1 = "NOTHING IS IMPOSSIBLE"
check_1 = proverb_1.isupper()
print(check_1)
proverb_2 = "NOTHING is IMPOSSIBLE"
check_2 = proverb_2.isupper()
print(check_2)
The lower() function is used to convert all the characters of a string to lowercase.
Syntax: string.lower()
statement = "Listen, THINK and LEARN"
x = statement.lower() print(x)
listen, think and learn
The upper() function is used to convert all the characters of a string to uppercase.
Syntax: string.upper()
sentence = "Do this right!"
x = sentence.upper() print(x)
Example 3.1
DO THIS RIGHT!
Your friend has asked you to print a birthday invitation card. Write a program to accept the information entered by the user to print the card in the format below.
You are invited to Soha’s Birthday Party on 3rd May at 7pm
VENUE: Willow Springs, Gandhi Circle
Answer:
name = input("Enter your name : ")
date= input("Enter your date : ")
event = input("Enter event name : ")
time = int(input("Enter the time of the event : "))
addr = input("Enter venue address : ")
print("*"*16)
print("You are invited to")
print(name,"'s",event.upper())
print("on" ,date)
print("at",time,"pm")
print("VENUE",addr.lower())
print("*"*16)
Enter your name : Soha
Enter your date : 3rd May
Enter event name : Birthday Party
Enter the time of the event : 7 Enter venue address : Willow Springs, Gandhi Circle
You are invited to Soha's BIRTHDAY PARTY on 3rd May at 7 pm
VENUE willow springs, gandhi circle
%s reserves a place for the variables that hold the value to be inserted in the string.
Syntax: print("String %s " %variable_name)
act = "reset"
print("Every sunset is an opportunity to %s." %act)
Every sunset is an opportunity to reset.
Multiple strings can also be embedded within a single string using multiple %s operators. If there are multiple %s , the strings are replaced based on their order in the brackets.
fall = 7
stand = 8
str = 'times'
print('Fall %s %s and stand %s %s.' %(fall,str,stand,str))
Fall 7 times and stand 8 times.
The f-String formatting
f-strings are another way to use and embed variables and expressions inside a string.
We start a string with the letter f followed by quotes, and the variables or expressions we want to embed within the quotes are enclosed within curly brackets {}.
Syntax: print(f"string {variable_name}.")
name = "Vanshita"
age = 19
print(f"Hello, {name}! You are {age}.")
Hello, Vanshita! You are 19.
To embed values using the format() function, we use curly brackets in the string where we want to replace values stored in variables. Then the variables are specified in the .format() function in the sequence we want them to be inserted in the string.
Syntax: "{} {}".format(value1, value2...) CODE
var1 = "You"
You
Matter!
Solved Examples
Example 4.1
You are assigned a task to make a program to spread some joy amongst people who use your program.
Write a program to do the following:
(a) Get the name as input from the person and store it in a variable name.
(b) Using the %s and f-string embedding, produce the following output
Enter your name: Shnaya
Hey Shnaya! We learned string formatting today. Are you ready for some practice, Shnaya?
Answer: CODE
name=input("Enter your name: ") print("Hey %s! We learned string formatting today." %name) print(f"Are you ready for some practice, {name}?")
Every character in a string is given a number that is called an index. We can access each character of a string with help of these indices. The first character has index 0, the second character has index 1, and so on.
Syntax: string_name[index number] CODE
greeting = "Happy Journey!"
# H=0 a=1 p=2 p=3 y=4 white_space=5 J=6 o=7 u=8
print(greeting[8])
The index() function is used to get the index number of a particular character in a string.
If the character occurs more than once in the string, the index of the first occurrence of that character is returned.
Syntax: string.index(value, start, end)
#value - The character to search for #start (Optional): Index number to start the search from. Default is 0.
#end (Optional): Index number to end the search at. Default is to the end of the string.
str = "Crunchy Leaves"
Python numbers the characters of a string in two ways, forward and backward. While numbering backward, Python gives the characters a negative index starting from -1 for the last character in the string.
quote = 'Embrace the journey!'
print(quote[-1])
print(quote[-4])
Python does not allow you to change any character in a string with help index. So we cannot change the value or a character within a string unless we reassign the entire string to the variable again.
msg = "Hey, Lio!"
msg[0] = "C"
print(msg)
TypeError: 'str' object does not support item assignment
In addition to indexing the strings, Python allows us to extract a part of a string. This extraction of strings is known as slicing and the extracted part of the string is known as a substring.
We slice a string by providing the starting index and 1 more than the ending index in square brackets [] after the variable.
Syntax: string_name[starting index:ending index]
s = "Embrace the journey!" print(s[2:7]) brace
Python can also skip letters in between while slicing. We use two colons for that.
Syntax:
String_name[start:end:step]
#If step is 3, python will print every third character
s = "Glorious journey!" print(s[4:16:2])
#python prints every second character
By not specifying the ending index, Python automatically takes the last letter of the string as the endpoint.
Syntax: string_name[starting index:]
word = 'helipad'
print(word[2:]) lipad
By not specifying a starting index, Python takes the start index of the first letter in the string.
Syntax: string_name[:ending index] CODE
word = 'helipad'
print(word[:6]) helipa
Python counts and returns the number of characters in a string using len() function.
Syntax: len(string_variable)
Example 5.1
word = 'helipad'
print(len(word)) 7
Store your first name as a string in the variable first_name and your last name as a string in the variable last_name.
1. Print the length of the string.
2. Select the first letter of the variables first_name and last_name and store them in variables first_initial and last_initial respectively.
3. Now, print the name and the first and last initials on 2 separate lines.
Enter your first name: Shreya
Enter your last name: Sharma
Entered name is: Shreya Sharma
Length of the name string is: 13
The first initial is: S
The last initial is: a
Answer:
first_name=input("Enter your first name: ")
last_name=input("Enter your last name: ") name=first_name+" "+last_name
print("Entered name is: ",name)
print("Length of the name string is: ",len(name))
first_initial=first_name[0]
print("The first initial is: ",first_initial)
last_initial=last_name[-1]
print("The last initial is: ",last_initial)
The reversal of strings means the output shown would be a string from last to first. For example, take a string “Malayalam” and the reversal of it would be “malayalaM”.
1. Reverse the following string: ”Good Day”.
2. Now using string slicing, reverse each word separately and join them back together.
(i.e., Change ”Hello world” to “olleH dlrow”)
Good Day
Reverse of the entire string is: yaD dooG
Word 1: Good
Word 2: Day
Reverse of both words individually: dooG yaD
Answer: CODE
string_1="Good Day"
print(string_1)
string_1_rev = string_1[::-1]
print("Reverse of the entire string is:",string_1_rev)
word_1 = string_1[:4]
print("Word 1:",word_1)
word_2 = string_1[5:]
print("Word 2:",word_2)
string_2_rev = word_1[::-1]+" "+word_2[::-1]
print("Reverse of both words individually:",string_2_rev)
The find() function returns the index of the first occurrence of the searched character or substring in a string.
The find() function returns -1 if the searched character or substring is not found within the string.
Syntax: string.find(<letter / substring to be searched>)
CODE
line = 'Kate on her skateboard!'
print(line.find('ate'))
#there are 2 ""ate"" substrings in the variable line, #but python shows the starting index of the first occurrence.
line = 'Kate on her skateboard!' print(line.find('Katie'))
#As 'Katie' is not present in the string, the output will be -1.
The replace() function replaces a specified character or substring with another in a string. The part of the string you wish to replace and the new string to replace it with are specified inside the replace() function along with the number of times you want to replace.
Syntax:
string.replace('oldWord','newWord',count)
oldWord: word you want to replace
newWord: word you want to replace with
count: the number of times you want to replace oldWord with newWord
line = "bo bo bo! Merry Christmas!" print(line.replace('bo', 'ho', 2))
ho ho bo! Merry Christmas!
If a count is not specified python replaces all the occurrences.
line = "bo bo bo! Merry Christmas!" print(line.replace('bo', 'ho'))
Example 6.1
ho ho ho! Merry Christmas!
You are given a string str1= “Hello , Have a nice day!”.
Take your name as an input and insert it between the string (using replace function) as shown below.
Hint: Replace the first space (“ “) with your name and spaces before it.
Enter your name: Riya
Hello Riya, Have a nice day!
Answer:
str1= "Hello, Have a nice day!"
name = input("Enter your name: ")
str2 = str1.replace(' '," "+name,1) print(str2)
We have a string str1=”plant trees, save earth”. You have to perform the following tasks in the specified sequence:
1. Replace all occurrences of the letter “e” with the letter “x”.
2. Find and print the index of the first and the last occurrence of the letter “x”.
3. Find and print the number of letters between the first and the last “x”. OUTPUT
The string after replacing e with x is: plant trxxs, savx xarth
Index of first x is : 8
Index of last x is: 18
Number of letters in between the first and the last x is: 9
Answer:
str1="plant trees, save earth" str1=str1.replace("e","x")
print('The string after replacing e with x is:',str1) firstx=str1.find("x")
str1_reverse=str1[::-1]
xtemp=str1_reverse.find("x")
lastx=len(str1_reverse)-1-xtemp
print('Index of first x is :',firstx)
print('Index of last x is:',lastx)
no_of_letters=lastx-firstx-1
print('Number of letters in between the first and the last x is:',no_of_letters)
Decision-making, as the name suggests, is making decisions based on certain conditions. To write these conditions we need to use comparison and logical operators.
Comparison operators compare the value on the left-hand side with the value on the right-hand side and return either True or False as a result of the comparison.
These operators are also known as relational operators. Below are the six comparison operators in Python.
== Equal to Returns True if both operands are equal x==y
!= Not Equal to Returns True if operands are not equal x!=y
> Greater than Returns True if left operand is greater than the right x>y
< Less than Returns True if left operand is less than the right x<y
>= Greater than or equal to Returns True if left operand is greater than or equal to the right x>=y
<= Less than or equal to Returns True if left operand is less than or equal to the right x<=y
# Using the inequality operator
print(3!=5)
print(2!=2)
# Using the greater than operator
print(1>3)
print(10>8)
# Using the less than operator
print(10<12)
print(10<9)
# Using the greater than or equal to operator
print(16>=16)
print(14>=15)
# Using the less than or equal to operator
print(16<=18)
print(15<=10)
Example 1.1
Johnny has to check whether the number of balls in box A is equal to, less than or greater than the number of balls in box B. Box A contains 453 balls and box B contains 454 balls respectively. Using conditional statements print whether the situations are True or False.
Are balls in box A equal to box B? False
Are balls in box A less than box B? True
Are balls in box A greater than box B? False
Answer:
box_A=453 box_B=454
cond1=box_A==box_B
print("Are balls in box A equal to box B?\n",cond1) cond2=box_A<box_B
print("Are balls in box A less than box B?\n",cond2) cond3=box_A>box_B
print("Are balls in box A greater than box B?\n",cond3)
Joe’s mother gives him Rs. 350 and asks him to get some groceries from a nearby shop. He plans to buy tomatoes for Rs. 50, onions for Rs. 60, and a jar of Peanut Butter for Rs. 100.
(a) With the help of a conditional statement print True or False to help him find out if the money he has will be enough to buy the planned items or not.
(b) Joe plans to buy 2 more jars of Peanut butter with the remaining money. Help him decide if he has enough money for doing so.
The total amount of money with Joe is: 350
The total bill will be: 210
Is the money adequate to buy groceries? True
The remaining amount left with Joe is: 140 Can Joe buy 2 more jars of Peanut butter?
False
Answer:
price_tomato=50
price_onion=60
price_butter=100
total_money=350
print("The total money with Joe is: ",total_money) total_bill=price_tomato+price_onion+price_butter
print("The total bill will be: ",total_bill)
cond=total_money>total_bill
print("Is the money adequate to buy groceries?\n",cond)
remaining_money = total_money - total_bill
print("The remaining amount left with Joe is: ",remaining_money)
cond2=remaining_money>(price_butter*2)
print("Can Joe buy 2 more jars of Peanut butter?\n",cond2)
Logical operators are used to compare two or more conditions. These conditions can be formed using the comparison operators. We get a boolean value (True or False) as output after comparing these conditions.
The logical operators include “and” and “or”.
The and operator compares two conditional statements and returns True only if BOTH are true, else returns False in all other cases.
Syntax: Statement1 and Statement2
Following is the truth table for the AND operator:
Condition_1 Condition_2
and Condition_2
print(4<9 and 10==10) print(8>3 and 10==12)
The or operator compares two conditional statements and returns True if any one of the statements is true. It returns False if both conditional statements are false.
Syntax: Statement1 or Statement2
Condition_1 Condition_2
Condition_1 or Condition_2 TRUE TRUE TRUE TRUE FALSE TRUE FALSE TRUE TRUE FALSE FALSE FALSE
We can form complex conditional statements in Python using multiple and/ or operators. In such cases where we have multiple and/or conditional statements, and has precedence over or. (i.e., the conditions with and are solved first and then the conditions with or are solved)
Syntax:
Statement1 or Statement2 and Statement3
#First we solve the AND operator, and then the OR
print(4>7 or 5==5 and 7>5)
Example 2.1
David needs to select students for his upcoming project based on two criteria. The students should have 60% in their academics, and they should have a score of 7 in their analytical tests.
Now, Ray has a score of 65% in his academics and 8.5 in analytical tests. Similarly, Joe has a score of 55% in his academics and 8 in analytical tests.
So display True or False based on the condition each for Ray and Joe whether they pass David’s criteria or not.
Ray's scores:
Academics: 65%
Analytical test: 8.5
Does Ray meet David's criteria? True
Joe's scores:
Academics: 55%
Analytical test: 8
Does Ray meet David's criteria? False
Answer:
ray_academics=65
ray_analytical=8.5
print(f"Ray's scores:\nAcademics: {ray_academics}%\nAnalytical test: {ray_analytical}")
ray_condition = ray_academics>=60 and ray_analytical>=7
print("Does Ray meet David's criteria?\n",ray_condition)
joe_academics=55 joe_analytical=8
print(f"Joe's scores:\nAcademics: {joe_academics}%\nAnalytical test: {joe_analytical}")
joe_condition = joe_academics>=60 and joe_analytical>=7 print("Does Ray meet David's criteria?\n",joe_condition)
Ben is the captain of the school’s football team. He needs defensive players who are tall and strong and offensive wingers who are short and agile.
For defense, he needs players taller than 6 feet and with a weight of more than 68 kg.
For offense, he needs players shorter than 5.5 feet and with a weight of less than 57 kg.
Take your height and weight as float inputs, and with the help of Comparison and Logical operators, print True or False for the combined condition.
Enter your height in feet: 5
Enter your weight in Kg: 55
Do you satisfy any of Ben's criteria for the football team?
Answer:
height=float(input("Enter your height in feet: "))
weight=float(input("Enter your weight in Kg: "))
condition = (height>6 and weight>68) or (height<5.5 and weight<57)
print("Do you qualify any of Ben's criteria for the football team?\n ",condition)
Indentation is a fixed number of spaces added at the beginning of the code, grouping a set of statements into a particular block of code. So Python runs a particular block of code at a time.
Below you can see how the spaces or Indentation divides the code into various blocks of code.
Flowchart is a diagram and a graphical representation of an algorithm or program.
It is the blueprint of the program and it shows steps in sequential order.
Certain shapes are used to draw flowcharts, and these shapes have the meaning as follows:
Purpose Represents a start or end point
Represents the connection between two shapes and the flow of program Represents input or output
Represents the process or block of code
Represents the decision or conditional statement (Output is usually True or False)
A conditional statement is a statement that tests a criterion and performs the defined action if it becomes true.
The if-else statement in Python is the most simple decision-making statement. It is used to decide if a certain condition or set of conditions is True or not.
If the condition after the “if” keyword is True, the code block after the if condition is executed, and if the condition is False, the code block after the “else” keyword is executed.
It is not necessary to have another condition.
Syntax: if condition_1:
#Statements to execute if condition_1 becomes True else:
#Statements to execute if condition_1 becomes False
age = 21
if age >= 18:
print("Eligible to vote.")
else: print("Too young to vote!")
Eligible to vote.
Nesting is the process of adding an if-else statement within another if-else. This will help us evaluate the second condition only when the first condition is True.
The first if statement is commonly called “outer if” and the block within it is called “nested / inner if”.
Syntax:
if condition_1:
if condition_2:
Statement_A
else:
Statement_B
else:
if condition_3:
Statement_C
else:
age=10
height=160
if age >= 10:
if height > 150:
Statement_D
You get a roller-coaster!
print('You get a roller-coaster!') else:
else:
print('You get water rides!')
print('Sorry, no rides for you!')
In situations where we have multiple conditions to evaluate the elif statement is used. This in simple terms means “else if”. The elif statement lets you add more than one conditional statement after the if statement or between the if and else statements in the program.
FlowchartIn multiple elif conditions, Python checks for the next one if the previous ones are False.
Syntax: if condition_1:
Statement_1
elif condition_2:
Statement_2
elif condition_3:
Statement_3 .. .. ..
else:
age=10
height=160
if age >= 10 and height > 150: print('You get a roller-coaster!')
elif age >= 10 and height <= 150: print('You get water rides!')
else: print('Sorry, no rides for you!')
Flowchart
You get a roller-coaster!
Example 3.1
Take a number as an input from the user and write a program to check whether the given number is a positive number or a negative number. Also, take another number as a reference in the variable ref_number and display if the input number is on the left or right-hand side of the reference number when put on the number line.
Enter a number: -5.2
The input number -5.2 is negative
Enter a reference number: -9 -5.2 is on the right-hand side of the number -9.0
Answer:
num=float(input("Enter a number: ")) if num>0:
print(f"The input number {num} is positive")
else:
print(f"The input number {num} is negative")
ref_number = float(input("Enter a reference number: ")) if num>ref_number:
print(f"{num} is on the right-hand side of the number {ref_ number}")
else:
print(f"{num} is on the left-hand side of the number {ref_ number}")
Write a program to get today’s day as the input and check if it is a “weekend” or a “weekday”.
If it is a day from the weekend, check if the next day is a weekend or a weekday.
Enter today's Day: Monday
Monday is a weekday.
Enter today's Day: Saturday Saturday is a weekend.
Next day is Sunday, it is the weekend.
Answer:
current_day = input("Enter today's Day: ")
if current_day.lower()=="Monday" or current_day.lower()=="Tuesday" or current_day.lower()=="wednesday" or current_day.lower()=="Thursday" or current_day.lower()=="Friday":
print(f"{current_day} is a weekday.")
elif current_day.lower()=="Saturday":
print(f"{current_day} is a weekend.")
print("Next day is Sunday, it is the weekend.")
elif current_day.lower() == "sunday":
print(f"{current_day} is a weekend.")
print("Next day is Monday, it is a weekday.")
1. Write a program to print a bill as given below using sep.
2. Below are the marks of a student named Max in different subjects. Store the scores in variables to print the total and average.
3. There are three trains. Train 1 covers 25 km per hour. Train 2 covers 50 km per hour. Train 3 covers 80km per hour. How far do the trains go in 2.5 hours?
Train 1 covers 62.5km
Train 2 covers 125km
Train 3 covers 200km
4. In a quiz competition, for each correct answer you get 2 points and for each incorrect answer 1 point is deducted. There are 12 questions in total. What are the maximum and minimum marks that you can score in the competition? Convert those marks into the string and the boolean values and print them as well.
Maximum marks=24
Float value= 24.0
Minimum marks=-12
Float Value= -12.0
5. Create a program that uses the input of the game’s name. Display a boolean value to see whether the entered name is lowercase or not. Also, it would look messy to store the game names if everyone used a different case so, convert the input to uppercase.
Enter a game you like to play: Football
Entered Game name is: FOOTBALL
The input is in lowercase: False
6. It is the start of the school year and you are at a notebook store. The shopkeeper asks your help to print the bill. The rate of the note books are as follows:
Print the total bill of a customer who purchases 3 blank, 4 ruled, and 1 grids notebooks.
7. Input a sequence of vegetables in a single string.
For example veggies = “Carrot Radish Tomato Beans Okra”
You have to display this in a vertical manner using the replace function and the newline character.
Enter the name of vegetables : Carrot Radish Tomato Beans Okra
The list with each item on a new line as follows: Carrot Radish Tomato Beans Okra
8. Write a program to take a year as input from the user and calculate whether the year is a leap year or not.
Hint: Leap year is the year which is divisible by 4 and not by 100 or which is directly divisible by 400.
Enter a year: 2004
The year 2004 is a leap year
Enter a year: 1999
The year 1999 is not a leap year
While programming, we will often come across situations where we need to use a piece of code over and over again. However, writing the same line of code multiple times does not make sense. This is where loops come in handy. Loops help us repeat a set of instructions again and again until a particular condition remains True. As soon as the condition becomes False, the loop stops.
Python provides mainly two types of loops, the While loop and the For loop. But the steps remain loosely the same as below.
A looping process has mainly 4 steps:
1. Creating a condition variable and storing an initial value in it
2. Providing a test condition that runs the loop (This should be carefully stated in order to run the loop for a desired number of times)
In some cases, the body of the loop gets executed over and over as the test condition never becomes false, and the execution never stops. Such a loop is called an Infinite loop.
The simplest looping statement is the while statement. It allows a programmer to repeat a set of instructions when the condition is evaluated as True, hence it is also known as a conditional loop.
It verifies the condition before executing the loop. The code inside the while loop is repeated as long as the given condition remains True.
A while loop can be written using:
(a) The while keyword
(b) A condition which is either True or False
(c) A block of code, which is nothing but the set of instructions that is supposed to be repeated
Syntax: while condition:
Statement 1
Statement 2 ... ...
(Incrementing the value if required)
Proper indentation needs to be provided for the block of code present inside the while loop. It is also called the body of the loop.
Here, count is the condition variable, which starts with 1, and when Python checks the condition (count < 6), it comes out to be True. So, the loop starts. In line 3, the value of count is displayed. Then in line 4, i is increased by 1 (count = count + 1 = 1 + 1 = 2). After that, Python checks the condition again. As 2 < 6 is True, the loop runs again. This continues till the value of count is less than 6. When count becomes 6, the condition becomes False, and the loop stops.
The for loop is another looping statement. It is also known as a counting loop because we can use it to count up to a number or go through a sequence of values.
We use a variable to create the for loop called the loop variable.
The two ways to create a for loop:
1. With the range() function
2. Without using the range() function.
Using range() function, we can specify a starting number and an ending number. So, the for loop will start from the starting number and continue up to (ending number - 1), increasing the number by 1 after each loop.
Syntax:
for loop_variable in range(start, end, steps):
<statements>
#the loop runs from start to end-1
start Optional. An integer number specifying at which position to start. If the start number is not specified, Python takes 0 by default.
stop Required. An integer number specifying at which position to stop (not included).
step Optional. An integer number specifying by what value to increase the value of loop variable. By default Python increases the value by 1.
Here, the starting number is 0 and the ending number is 5. So, the for loop starts counting from 0 and continues till (ending number - 1) which is 4. And after each loop, the value of 1 increases by 1.
Without using the range function, a for loop can iterate over letters in a string, values in a list or a dictionary as well.
Syntax: for loop_variable in string_variable: <statements>
Here the loop starts with the first letter, i.e., letter = ‘a’. And in each loop, the value of the variable changes to the next letter in the string. So in the second loop, letter = ‘d’, in the third loop, letter = ‘v’, and so on. It continues up to the last letter in the string.
In for loop, we initialise, test and update the loop variable during writing a loop in one single line, whereas the while loop requires more lines.
For loop is also called a counting loop since it counts up to a number. for i in range(start, stop, increment):
//step1 //step2
In a while loop, the condition is tested first and the loop gets executed when the condition is met. In case, if we forget to update the loop variable, there are chances we may end up in an infinite loop which doesn’t happen in the case of for loop.
While loop is also called a conditional loop because it has a condition.
i=1
while(i<=10):
//step1 //step2
A nested loop is a loop inside another loop. The first loop we start off with is called the outer loop. All the loops that are written inside the outer loop are called inner loops. The outer loop can contain more than one inner loop. The inner or outer loop can be any type, such as a while loop or a for loop.
The entire “inner loop” gets executed every time the “outer loop” runs.
If the outer loop runs 5 times, and the inner loop runs 10 times. Then for the 1st iteration of the outer loop, the inner loop will run 10 times, for the 2nd iteration of the outer loop, the inner loop will run 10 times, and so on.
So in total, the inner loop will run 5 (outer loop count) * 10 (inner loop count) = 50.
rows = 6 for num in range(rows): for i in range(num): print(num, end=" ") # print
while i<6: for j in range(0,i): print(i,end="
Loops run the same set of code for a defined number of times or until the test condition becomes False, but sometimes we might wish to stop the loop even before the test condition becomes False or skip an iteration.
In those cases, we can use the break and continue statements.
The break keyword is used to break out / get out of the loop and execute the next statement.
Syntax:
while expression:
if condition: break
#breaks out of the loop
#executes statement outside the loop
word = input('Enter a word: ') for letter in word:
if letter in 'aeiou': print('Vowel found!') break
Enter a word: Success Vowel found!
Here, the loop runs for all the letters in the word entered by a user. Inside the loop, Python checks the condition if the letter is a vowel or not (i.e. a, e, i, o, u). If the condition becomes True, Python runs the break statement in line 5, which forces it to stop the loop.
The continue keyword is used to skip the execution of statements ahead in the loop. Python skips the statements after the continue keyword in the current iteration/cycle of the loop and does not stop the loop.
#skips next set of statements and starts with next loop
Here, the for loop starts counting from 1. In each loop, it increases the value of num by 1, and when num is equal to 12, 15, or 19 (i.e. the condition in line 2) is True, Python runs the continue statement, which forces it to skip the lines below it. That is why the numbers 12, 15, and 19 are not displayed in the output.
Practice Problems:
1. Write a program to print the table to any number using a while loop.
2. Create a program to print multiples of a number in the range 1 to 100.
3. Write a program to calculate the sum of the first 10 natural numbers using a for loop.
4. Write a program to display following pattern using nested loop:
5. Print all the numbers between 1 to 50 except the multiples of 5 using the continue statement.
In our day-to-day routine, we use a lot of machines for various purposes such as washing machine, oven, fan, tubelight, air conditioner, stove, chimney and a lot more. All these machines serve some specific purposes.
Let us take the example of a vending machine. A vending machine is an automated machine used to purchase items such as snacks, juices and soft drinks.
To purchase an item from a vending machine:
● Use buttons or the digital panel provided to select items and pay for them.
● Then, collect the items from the dispenser once the payment is done.
A vending machine does the above tasks every time it is used, which is an example of a function.
Every day, we repeat certain tasks over and over again. Therefore, if we automate them—that is, if we create them only once and reuse them as necessary—it becomes simple.
In programming, we come across situations where we need to repeat a particular set of lines for a task. So, instead of rewriting it, wouldn’t it be better to write it once and then use it as many times as we want without repeating ourselves?
But we can solve this with functions. We can create them once and call them whenever needed.
A function is a reusable block of code which can be used over and over again to perform a particular task.
The following are the two main categories of function:
1. Built-in functions
2. User-defined function
Built-in Functions: The functions that are already available in the library of python for our usage are known as built-in functions. These functions can be used anywhere in the program just by importing the specific library.
For example : print("Hello World!")
User-defined function : The functions that we create for achieving a particular task are known as user-defined functions. For defining a function in Python we use the def keyword followed by the function name.
For example : def my_function():
print("Hello, this is my first function");
Syntax for defining a function: In Python a user-defined function can be defined using def keyword. A function can have n number of statements.
Syntax: def function_name(): Statement1 ; Statement2; ….. ….. Statement n;
Example: def my_function: print("Hello! This is my first function");
Calling a Function: A function can be called by simply using the function name.
Example: for calling the above defined function my_function();
Output: Hello! This is my first function
Parameters are the variables that we pass while defining a user-defined function.
Whereas arguments are the variables that we pass while calling a function.
Example :
Output: 7
Explanation: Here, the parameters are x and y, and the arguments are 3 and 4 for the respective parameters, i.e., x = 3 and y = 4.
Types of Arguments
Python functions can have two types of arguments:
1. Positional arguments
2. Keyword arguments
Positional Arguments
Positional arguments need to be passed in the same order used in function definition.
Example:
Output: Daisy Brown
Explanation: Here, Daisy and Brown will be taken by the parameters according to their position.
first_name = Daisy
last_name = Brown
Keyword Arguments
Keyword arguments are the arguments that are preceded by a keyword and an equal to sign (i.e. Key = Value), here the order of the arguments does not matter as the values are being assigned explicitly.
Example:
def name (first_name , last_name):
print (first_name + last_name);
name (last_name = “Brown”, first_name = “Daisy”);
Output: Daisy Brown
Explanation: Here, the arguments are passed with their respective keywords, such as last_name = Brown and first_name = Daisy.
A function can return a value as a result , which makes it generalised. To get a function to return a value we can use the ‘return’ statement.
These functions can also be used to perform calculations or transformations on the input data and return the result. It makes the code more readable and maintainable. Such functions can be used in almost any programming scenario where a specific task needs to be performed repeatedly or where a calculation or data processing needs to be done.
Example:
Output: 16
Explanation: Here x = 4, So the function will return (4*4) i.e. 16.
You can even catch the result returned by the function in a variable.
Example:
Output: 16
Explanation: Here the variable square is catching the result from the function number_square and then printing the result.
Recursion is a common programming and mathematical concept. When a function calls itself is known as recursion. Recursion is a very efficient way to write a program.
The above image shows the working of a function recurse() which is calling itself in its definition.
Example:
Output: The factorial of 3 is 6.
Explanation: Here the factorial() is a recursive function created to calculate the factorial of a number.
When we call this function with a positive integer it will recursively call itself by decreasing the number.
Our recursion ends when the number reduces to 1. This is called the base condition.
Every recursive function must have a base condition to stop the recursion otherwise it will run infinitely.
Practice Questions:
1. Create a program to find the product of two numbers using a function with parameters.
2. Create a program to find if a number is prime or not using a function that returns the result.
3. Create a program that counts down from a specified number to zero using a recursive function.
4. Create a program to generate a fibonacci sequence using a recursion function.
5. Create a program to calculate the square of any number using a function that accepts parameters and returns the result.
Lists are used to store multiple values in a single variable. They are one of the most used data types that allow us to work with multiple values at once. To create a list, we store multiple values in between the square brackets. Each value is given a number called an index and the index starts from 0. The values inside the loop can be accessed using these index numbers.
Example: num_list = [1, 2, 3, 4, 5, 9, 6, 2]
Features of Lists
● They are ordered, i.e., Python always maintains the order in which you store the values in the list
● They have no fixed size or limit
● We can modify a list, i.e., we can add, update, or delete any value from a list
● The values in a list can be of the same or different data types
Syntax:
list_name = [value1,value2,value3,value4,................,valuen]
animals = ["lion", "tiger", "horse","cat","dog","rabbit"] print(animals)
['lion', 'tiger', 'horse', 'cat', 'dog', 'rabbit']
Each element in a list can be accessed by its index number.
Indexing like strings can happen in two ways,
● Positive indexing (Used to access elements in forward direction)
● Negative indexing (Used to access elements in backward direction)
animals = ["lion", "tiger", "horse","cat","dog","rabbit"] print(animals[0]) print(animals[-1])
lion rabbit
Lists can be sliced again in the same way as strings. We get a slice of the list or sublist by giving the starting index, ending index and an index jump. Python starts slicing the list at the starting index and continues till (ending index - 1). We use a simple slicing operator (:) to slice a list.
Syntax:
List_name[starting_index : ending_index : index_jump]
where,
starting_index: Starting point for slicing.
ending_index: Ending point for slicing. Python slices the list till (ending_ index - 1)
index_jump: Number of elements skipped. If the value is 2, then Python skips one value after slicing an index number. If the value is 3, then Python skips 2 values after slicing an index number.
NOTE: The default value for index_jump is 1. If we don't specify index_jump value , 1 will be considered as the value for index_jump. In that case, Python does not skip any value in the middle. CODE
vowels = ['a', 'e', 'i', 'o', 'u'] print(vowels[2:5])
['i', 'o', 'u']
Here, Python starts slicing the list at index number 2 (i.e., ‘i’) and continues till the index number 4 (i.e., ‘u’) .
vowels = ['a', 'e', 'i', 'o', 'u'] print(vowels[1:5:2])
['e', 'o']
Here, Python starts slicing the list at index number 1 (i.e. ‘e’). As the value of index_jump is 2 here, Python skips one value after index 1 and jumps to index 3 (i.e. ‘o’). Then, it skips index 4 and jumps to index 5, which does not exist in the list, so Python stops there. Hence, the final output is [‘e’, ‘o’].
Lists can be iterated over by using a loop in the same way as strings. Each time the looping variable holds the value of each element in the list.
Syntax:
list_name=[item1,item2,item3,...] for item in list_name: print(item)
vowels = ['a', 'e', 'i', 'o', 'u'] for letter in vowels: print(letter)
To check if a specific element is present in a list or not, we can use the in keyword to form the condition. This condition will return True if the element is in the list and False if it is not in the list.
Syntax:
list_name=[item1,item2,item3,...] print(item_name in list_name)
vowels = ['a', 'e', 'i', 'o', 'u'] print('b' in vowels)
As ‘b’ is not present in the list, Python displays False as output.
In lists, items can be changed by specifying their index. We just need to use the assignment operator to assign the value on the right at the specified index. This cannot be done in strings.
Syntax:
list_name[index] = value
numbers = [1,2,3,4,5] numbers[0] = "111" print(numbers)
In Python, we can join two or more lists into one list. We can use the + operator to merge the contents of two lists into a new list.
list_1 = [1, 2]
list_2 = [3, 4]
print(list_1 + list_2)
The elements inside a list can be repeated within the list multiple times using the * symbol. All the elements in a list are repeated in the same order for the number of times mentioned after the * operator. We can only use an integer value after the * symbol. Using a float value will show an error.
Syntax: list2 = list1 * integer_number
list_1 = [1, 2, 3]
print(list_1 * 2)
nested_list = [1, 2, [3, 4]]
print(nested_list * 2)
A list can contain another list as a value which in turn can contain sublists themselves, and so on. This method of building a list within another list is known as nesting of lists. These inner lists are called "sub-lists".
Syntax:
list_name = [item1, [item2_1, item2_2, item2_3], item3, item4]
Here, [item2_1, item2_2, item2_3] is the nested list.
If we want an item within the nested list or sublist of the list, we access it using the following 2 indices.
1. We first specify the index of the sublist
2. We then specify the index of the element inside the sublist that we want to access
Syntax:
list_name[index of sublist containing the item][index of the item]
= [1, 2, [3, 4]] print(num_list[2][0])
Explanation
Here, num_list[2] first accesses the sublist, i.e., [3, 4]. Then, num_list[2][0] accesses the first value inside the sublist, i.e., 3.
= [1, 2, [3, [4, 5]]] print(list_num[2][1][1])
Explanation
Here, num_list[2] first accesses the sublist, i.e., [3, [4, 5]]. Then, num_list[2][1] accesses the second value inside the sublist, i.e., [4, 5]. Finally, num_list[2][1][1] accesses the second value inside the innermost sublist, i.e., 5.
The len() function is used to get the number of elements in a list.
Syntax: len(list_name)
The append() method can be used to add elements to the end of the list.
Syntax: list.append(element)
CODE
list_1 = ['Mathematics', 'chemistry', 1997, 2000]
list_1.append(20544)
print(list_1)
['Mathematics', 'chemistry', 1997, 2000, 20544]
The insert() method helps us to insert an element at a specific index or location in a list. We specify the position of the index at which we want to insert the element and then the element separated by a comma.
Syntax: list.insert(position_index, element) CODE
List = ['Mathematics', 'chemistry', 1997, 2000]
List.insert(2,10087)
print(List)
Here, Python inserts 10087 at index 2 inside the list.
['Mathematics', 'chemistry', 10087, 1997, 2000]
The remove() method removes the specified item from a list. We specify the element we wish to remove from the list in the brackets. The method removes the 1st occurrence of the element from the list.
Syntax: list.remove(element)
Here, Python removes the first 3 from the list.
The del keyword removes the element at a specified index and can also delete the list completely.
Syntax: del list[index]
The min() method returns the minimum value from the list. In case of numbers, the number with least value is returned, in case of strings, the string with the lowest alphabet is returned.
Syntax: min(List)
The max() method returns the maximum value from the list. Same as min(), in case of strings, the string with the greatest alphabet is returned.
Syntax: max(List)
The sum() function returns the sum of all items in a list.
Syntax: sum(List)
1. Write a program to check whether a number exists in a list or not?
2. Write a program to calculate the sum and average of all numbers in a list.
3. Write a program to count even and odd numbers in a list.
4. Write a program to count occurance of a number in a list.
5. Create a list of marks in mathematics of 10 students and display maximum and minimum marks.
Dictionary is a data type that is used to store data in key:value pairs. It is an unordered collection of elements. A dictionary can be created by placing a sequence of elements within curly {} brackets. A dictionary can be created by placing a sequence of elements within curly {} brackets, separated by ‘comma’.
Example :
dict = { 'Name': 'Daisy', 'Age': 12, 'Grade': 7 }
Features of a Dictionary:
● Each element is accessed through its key.
● Values of a dictionary are mutable or changeable.
● Each key in the dictionary must be unique as it does not allow duplicate data.
NOTE: Dictionary keys are case sensitive, the same name but different cases will be treated distinctly.
Example:
dict = { 'a': 1, 'A': 2 }
Here a and A will be considered unique.
The name of the dictionary can be used to access all of its elements and the key name is used to access a particular element of the dictionary.
dict = { 'Name': 'Daisy', 'Age': 12 , 'Grade': 7 }
print(dict)
{'Name': 'Daisy', 'Age': 12, 'Grade': 7}
dict = { 'Name': 'Daisy', 'Age': 12, 'Grade': 7 }
print(dict['Name'])
dict = { 'Name': 'Daisy', 'Age': 12 , 'Grade': 7 }
print(dict['Age'])
You can update a dictionary by :
● Adding a new item in it using key:value pair
● Modifying the existing item
● Deleting any item from it
dict = { 'Name': 'Daisy', 'Age': 12, 'Grade': 7
# Adding a new item dict['LastName'] = 'Brown' print(dict)
{ 'Name': 'Daisy', 'Age': 12, 'Grade': 7, 'LastName': 'Brown'}
dict = { 'Name': 'Daisy', 'Age': 12, 'Grade': 7
# Updating/Modifying an existing item dict['Grade'] = 8 print(dict)
{ 'Name': 'Daisy', 'Age': 12, 'Grade': 8, 'LastName': 'Brown'}
The del keyword can be used to delete a particular item from the dictionary. You can even delete the entire dictionary using the same.
dict = { 'Name': 'Daisy', 'Age': 12, 'Grade': 7, 'LastName': 'Brown'
# Deleting an item del dict['LastName'] print(dict)
{'Name': 'Daisy', 'Age': 12, 'Grade': 7}
dict = { 'Name': 'Daisy', 'Age': 12, 'Grade': 7, 'LastName': 'Brown' }
# Deleting the entire dictionary del dict print(dict)
<class
Note: you can use clear() to remove all the entries from the dictionary.
Example: dict.clear();
Dictionary is a commonly used data type in Python to store multiple values using the key: value pair.
Although before using the dictionary you must understand the properties of dictionary keys:
1. No duplicate keys allowed: More than one entry for a particular key is not allowed. In such cases the last assignment for the key will be considered .
dict = { 'Name': 'Daisy', 'Age': 12, 'Grade': 7, 'Name': 'John' }
print(dict['Name'])
Explanation: Here the key ‘Name’ was repeated so the last assignment i.e. ‘John’ will be considered.
2. The values of a dictionary are mutable, whereas keys are not. That is why lists are not allowed as keys of a dictionary. Because lists are mutable.
dict = {
TypeError: unhashable type: 'list'
'Name': 'Daisy', 'Age': 12, ['Grade']:7
print(dict)
A dictionary can contain dictionaries, this is called a nested dictionary.
Syntax:
nested_dict = {
'dict1' : {'key_A' : 'value_A'}, 'dict2' : {'key_B' : 'value_B'}
students = { 1: {
"Name": "Daisy", "Grade": 7, "Roll_number": 5 }, 2: {
"Name": "John", "Grade": 7, "Roll_number": 10
print(students)
{1: {'Name': 'Daisy', 'Grade': 7, 'Roll_number': 5}, 2: {'Name': 'John', 'Grade': 7, 'Roll_ number': 10}}
CODE OUTPUT
details = {
'Name': 'Daisy', 'Age': 12, 'Marks': { 'Eng': 89, 'Math': 95, 'Computer': 98 }
{'Name': 'Daisy', 'Age': 12, 'Marks': {'Eng': 89, 'Math': 95, 'Computer': 98}}
Python has various methods to work with dictionaries. Following are the methods of Python dictionary:
Method Description Syntax clear() Removes all the items from the dictionary.
copy() Returns a copy of the dictionary.
dict.clear()
dict.copy()
fromkeys() Creates a dictionary from a given sequence. dict. fromkeys(sequence,value) get() Returns the value of a given key.
items() Returns a list with all the keys and values.
keys() Returns all the keys of the dictionary.
values() Returns all the values of the dictionary.
dict.get(key,default = None)
dict.items()
dict.keys()
dict.values()
setdefault() Inserts a key with default value to the dictionary.
update() Updates an existing dictionary item.
pop() Removes and returns the specified element from the dictionary.
popitem() Removes the last inserted key-value pair.
sort() Sorts the dictionary in reference with keys.
len() Shows the total number of items in the dictionary.
Practice Questions:
dict.setdefault(key, default_ value)
dict.update([other])
dict.pop(key)
dict.popitem()
dict.sort()
len(dict)
1. Create a dictionary to store prices of fruit and display the prices of all fruit.
2. Create a Python program to store details of a student (Name, Grade, Address, PhoneNumber, Favourite_Subject) using a dictionary data type and display all the values.
3. Create a phone book program using a nested dictionary and store details of your 3 friends and display them.
4. Create a dictionary to store your marks of all your subjects and display them.
5. Create a nested dictionary to store the capital and currency of any 5 countries.
Create a dictionary that stores student details. Add new keys in that dictionary by taking inputs from the user.
About this book
This coding book is supplementary to the main “Mel n Conji” content book. This book represents a 21st-century approach to learning coding concepts and developing computational thinking and problem-solving skills. To prepare students for the digital age, the curriculum is interwoven with well-thought-out concept graduation with real-life examples and practice problems.
• Illustrative approach: Concepts in coding and computer science are delivered through pictorial representations and learner-friendly approaches.
• Learning through real-life examples: Age-appropriate examples that enable learners to relate to the concept and learn about the unknown from the known.
• Extensive practice: Multiple practice scenarios to reinforce learnings.
• Coding Challenges: Includes projects through which learners can demonstrate their learning outcomes in coding and computer science.
About Uolo Explore more
UOLO partners with K12 schools to bring technology-based learning programs. We believe pedagogy and technology must come together to deliver scalable learning experiences that generate measurable outcomes. UOLO is trusted by over 8,000 schools with more than 3 million learners across India, South East Asia, and the Middle East.
hello@uolo.com